Mastering Java 8: Overcoming Common Coding Challenges
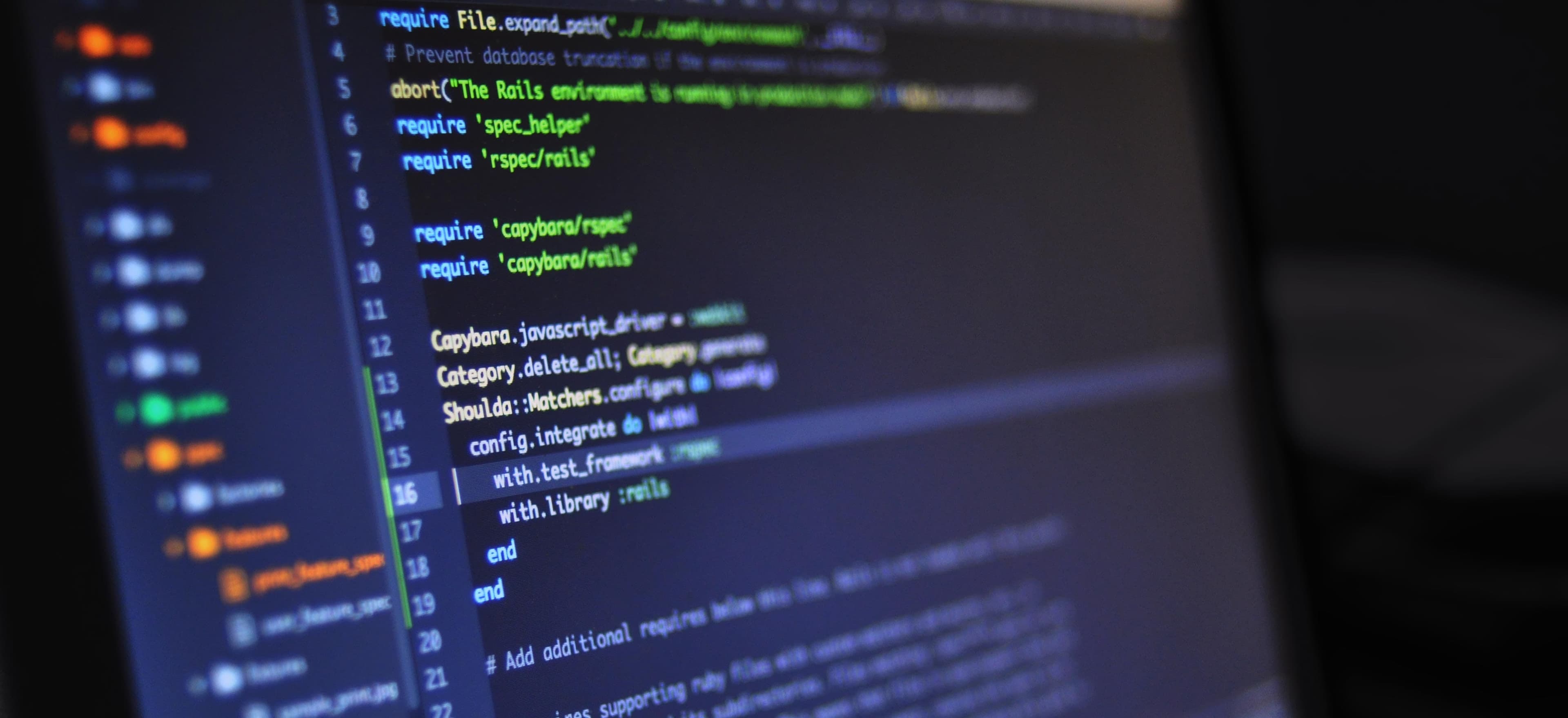
- Published on
Mastering Java 8: Overcoming Common Coding Challenges
Java is known for its versatility, but with every technology comes its unique set of challenges. Java 8 introduced a plethora of new features that not only enhance performance but also change the way we approach coding in Java. In this blog post, we will explore common coding challenges faced by Java developers and how to overcome them using Java 8 features.
1. Stream API
The introduction of the Stream API in Java 8 revolutionized how we handle collections of data. It provides a way to process sequences of elements (such as collections) in a functional style.
Challenge: Managing Large Data Sets
When managing large data sets, traditional for-loops can be inefficient and hard to read.
Elegant Solution: Using Stream API
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Using Stream API to filter names starting with 'C'
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("C"))
.toList(); // Java 16+ alternative to collect()
System.out.println(filteredNames); // Output: [Charlie]
}
}
Why Use Stream API?
The code above demonstrates how the Stream API simplifies the process of filtering data, making it more readable and concise. You no longer need to worry about incrementing indices in loops; the logic is clear and declarative.
For more details on Java Streams, you can check the official Oracle documentation.
2. Lambda Expressions
Java 8 introduced lambda expressions, allowing you to implement functional interfaces concisely.
Challenge: Writing Anonymous Classes
Before Java 8, writing anonymous classes for simple functionality often cluttered the codebase.
Solution: Use Lambda Expressions
import java.util.ArrayList;
import java.util.List;
public class LambdaExample {
public static void main(String[] args) {
List<String> items = new ArrayList<>();
items.add("Apple");
items.add("Banana");
items.add("Cherry");
// Using a lambda expression to print items
items.forEach(item -> System.out.println(item));
}
}
Why Use Lambda Expressions?
Lambda expressions reduce boilerplate code significantly. The forEach
method accepts a lambda that makes it very easy to read what the code does. As a result, you maintain the clarity of your operations without lengthy class definitions.
For more on lambda expressions and their applications, explore this Java Developer Guide.
3. Optional Class
One of the most prevalent issues in Java has been handling null
values, which can lead to exceptions during runtime.
Challenge: Null Pointer Exception
Developers often face Null Pointer Exceptions when navigating through objects without ensuring they aren't null.
Solution: Use Optional
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
String value = null;
// Using Optional to handle possible nulls safely
Optional<String> optionalValue = Optional.ofNullable(value);
// Use ifPresent to handle the value if it's present
optionalValue.ifPresent(val -> System.out.println("Value: " + val));
System.out.println("Value is absent: " + optionalValue.isEmpty());
}
}
Why Use Optional?
The Optional class helps avoid issues related to null references by providing a container that either holds a value or indicates absence of a value. By using methods like ifPresent()
, you can write cleaner and safer code.
4. Default Methods in Interfaces
Another hefty challenge has been adding new methods to interfaces without breaking existing implementations.
Challenge: Versioning Interfaces
When needing to add new functionality, modifying existing interfaces could disrupt all implementing classes.
Solution: Use Default Methods
interface Animal {
void makeSound();
// Adding new functionality with default method
default void eat() {
System.out.println("This animal eats food.");
}
}
class Dog implements Animal {
@Override
public void makeSound() {
System.out.println("Bark");
}
}
public class DefaultMethodExample {
public static void main(String[] args) {
Animal dog = new Dog();
dog.makeSound(); // Output: Bark
dog.eat(); // Output: This animal eats food.
}
}
Why Use Default Methods?
Default methods allow you to extend interfaces without forcing changes to every implementation. This is particularly beneficial for larger codebases and libraries where backward compatibility is crucial.
To Wrap Things Up
Java 8 has equipped developers with powerful tools to tackle common coding challenges effectively and elegantly. By leveraging features like the Stream API, Lambda Expressions, Optional class, and Default Methods, you can significantly improve your coding practice, enhance code readability, and avoid common pitfalls like Null Pointer Exceptions.
Remember, while these new features do provide an elegant solution, your programming approach should still reflect the requirements of your project. Strive for performance, maintainability, and readability.
If you're eager to dive deeper into Java 8's new features, consider exploring the Java Tutorials provided by Oracle. Happy coding!