Mastering Java: Sort a List of String-Integer Pairs!
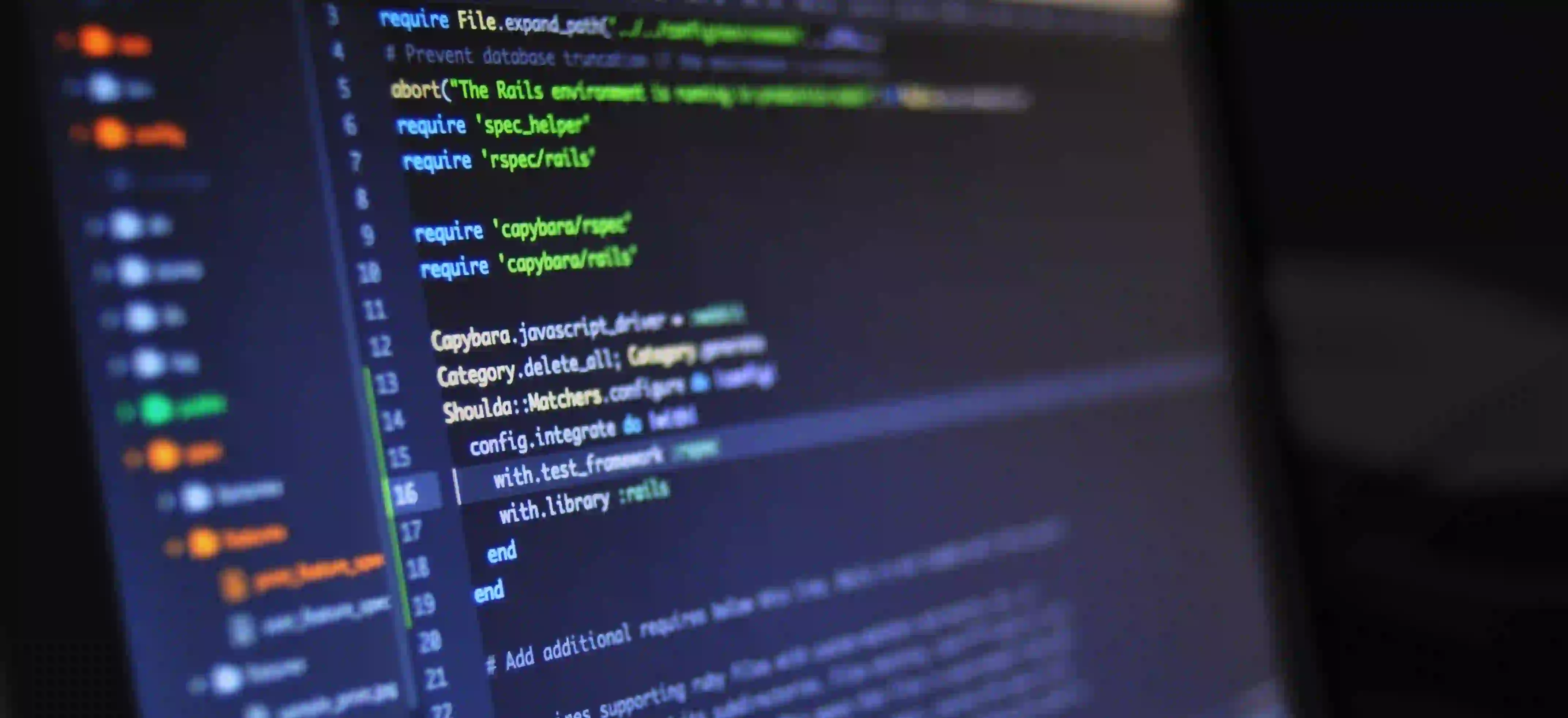
Mastering Java: Sort a List of String-Integer Pairs!
In the world of programming, mastering data structures and algorithms is key to writing efficient code. One common task is sorting a collection of data. Today, we will explore how to sort a list of pairs, specifically that of strings and integers. This operation presents numerous applications in real-world scenarios, such as sorting user data, organizing product information, and more.
In this blog post, you will learn:
- How to create a list of string-integer pairs.
- Different methods to sort that list.
- Best practices for working with collections in Java.
Let's dive in!
Setting Up Your Java Environment
Before we start coding, ensure you have a Java Development Kit (JDK) installed on your machine. If you haven't set it up yet, you can download the latest version from the Oracle website.
Example Code Structure
We will begin by creating a simple Java program that creates a list of string-integer pairs. Here's the basic structure of our code:
import java.util.*;
public class StringIntegerSorter {
public static void main(String[] args) {
// Your code will go here
}
}
Creating a List of String-Integer Pairs
First, let's define our list of pairs. In Java, a good way to represent a pair of values is through a simple class or using a built-in class. For simplicity, we will use AbstractMap.SimpleEntry
, which allows us to create pairs easily.
Here’s how you can create a list of string-integer pairs:
List<Map.Entry<String, Integer>> pairs = new ArrayList<>();
pairs.add(new AbstractMap.SimpleEntry<>("Apple", 30));
pairs.add(new AbstractMap.SimpleEntry<>("Banana", 20));
pairs.add(new AbstractMap.SimpleEntry<>("Orange", 25));
Why This Approach?
Using AbstractMap.SimpleEntry
offers a concise way to handle pairs without creating an entirely new class. Nonetheless, if you require additional functionality, it may be worth implementing your own class.
Sorting the List
Java provides several ways to sort collections, primarily through the Collections
class and the Comparator
interface. Here, we'll sort our list based on the integer values (the second item in the pair).
Using Lambda Expressions
Java 8 introduced lambda expressions that allow for more concise code. We can sort our list using a lambda expression as follows:
Collections.sort(pairs, (pair1, pair2) -> {
return pair1.getValue().compareTo(pair2.getValue());
});
Why Use compareTo
?
The compareTo
method is part of the Comparable
interface and is used for comparing two objects. This method returns:
- A negative integer if the first object is less than the second.
- Zero if they are equal.
- A positive integer if the first is greater than the second.
Complete Code Snippet with Sorting
Putting it all together, here’s a full code snippet that demonstrates the above functionalities:
import java.util.*;
public class StringIntegerSorter {
public static void main(String[] args) {
// Create a list of string-integer pairs
List<Map.Entry<String, Integer>> pairs = new ArrayList<>();
pairs.add(new AbstractMap.SimpleEntry<>("Apple", 30));
pairs.add(new AbstractMap.SimpleEntry<>("Banana", 20));
pairs.add(new AbstractMap.SimpleEntry<>("Orange", 25));
// Print the list before sorting
System.out.println("Before sorting:");
printPairs(pairs);
// Sort the pairs by integer value
Collections.sort(pairs, (pair1, pair2) -> pair1.getValue().compareTo(pair2.getValue()));
// Print the list after sorting
System.out.println("After sorting:");
printPairs(pairs);
}
// Helper method to print the pairs
private static void printPairs(List<Map.Entry<String, Integer>> pairs) {
for (Map.Entry<String, Integer> pair : pairs) {
System.out.println(pair.getKey() + ": " + pair.getValue());
}
}
}
Output Explanation
When you run the above code, you should see the output as follows:
Before sorting:
Apple: 30
Banana: 20
Orange: 25
After sorting:
Banana: 20
Orange: 25
Apple: 30
This output demonstrates that our list of pairs has been sorted according to the integer values associated with each string.
Sorting in Different Orders
While the previous code snippet showed how to sort in ascending order, you can easily modify the comparator for descending order:
Collections.sort(pairs, (pair1, pair2) -> pair2.getValue().compareTo(pair1.getValue()));
Sorting by String Values
Often, you may want to sort based on the string values instead. You can do this easily by replacing the integer value in the comparator:
Collections.sort(pairs, (pair1, pair2) -> pair1.getKey().compareTo(pair2.getKey()));
Why Are Comparators Important?
Comparator
s provide a flexible mechanism for sorting collections in Java. By using custom comparator logic, we can define multiple sorting criteria, dramatically increasing the versatility of our code.
Final Considerations
In this blog post, we learned how to handle sorting a list of string-integer pairs in Java. We explored creating pairs using AbstractMap.SimpleEntry
, sorting the list with Collections.sort()
using both default and custom comparators, and examined various sorting scenarios.
Sorting is an essential skill in programming, and Java makes it relatively straightforward and flexible. The examples provided demonstrate how you can tailor your code to meet specific requirements, improving efficiency and clarity.
If you're interested in diving deeper into Java collections and advanced sorting techniques, consider visiting the Java Tutorials. As always, happy coding!
Additional References
Feel free to reach out with any questions or insights on your journey to mastering Java!