Solving Hot Deploy Challenges in Java Enterprise with Docker
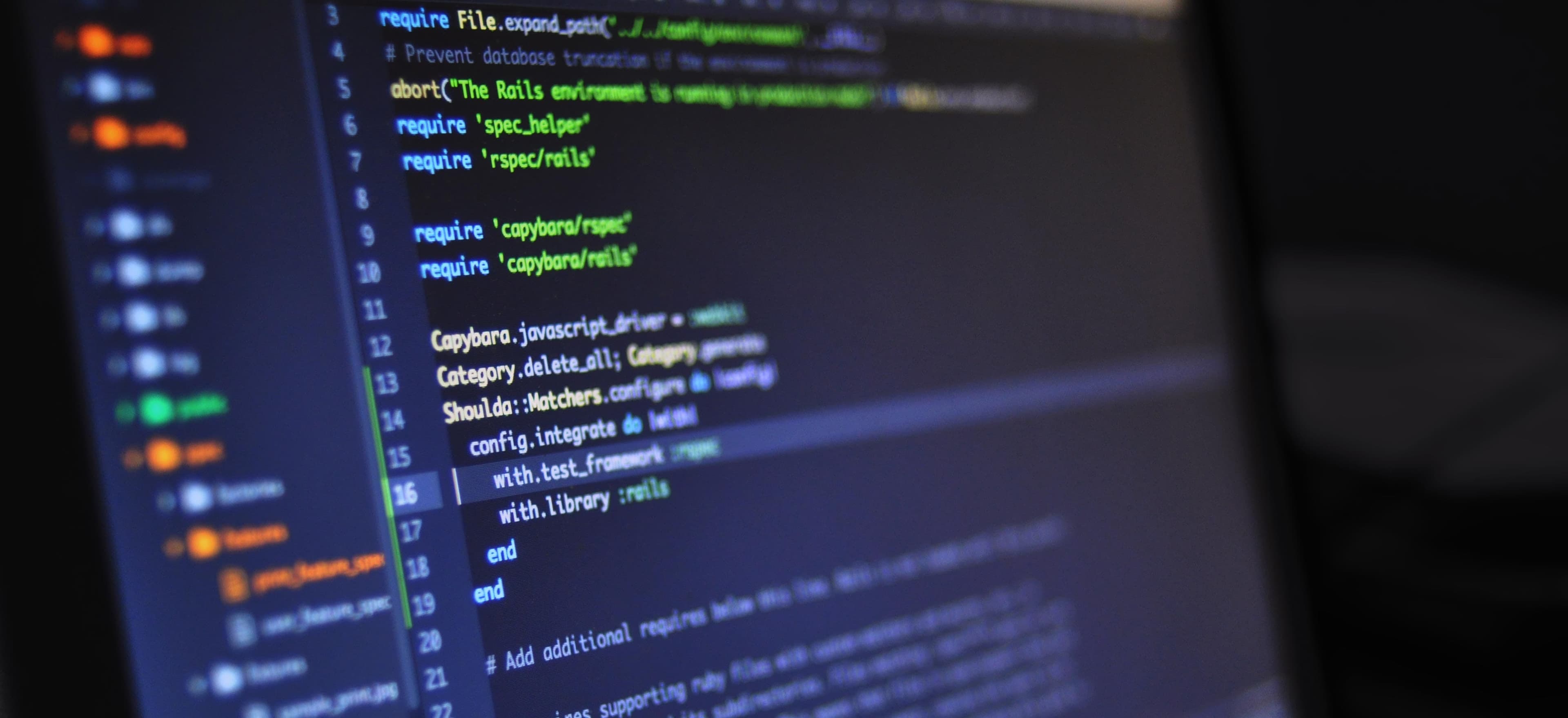
- Published on
Solving Hot Deploy Challenges in Java Enterprise with Docker
In the world of Java Enterprise (Java EE), the concept of hot deployment is crucial. Developers aiming for agility often find themselves struggling with deployment times and the inability to see real-time changes without a restart. This is where Docker comes into play. In this blog post, we'll explore how Docker can help streamline the hot deployment process, ultimately enhancing developer productivity.
Understanding Hot Deployment
Hot deployment, also known as hot swapping, allows developers to update a running application without having to restart it. This can be immensely beneficial for large applications where restart times can be lengthy. However, there are challenges:
- Complexity in Configuration: Ensuring that all components work seamlessly during hot deployment can be daunting.
- State Management: Preserving the application state while deploying new changes can lead to unexpected errors.
- Dependency Resolution: New changes might introduce conflicts with existing dependencies.
Enter Docker
Docker can significantly mitigate these challenges. By providing a lightweight, consistent environment, Docker enhances the hot deployment process. Let's delve into how it does this.
1. Consistent Environment
One of Docker's standout features is its ability to create consistent environments through images. This consistency means you can run the same container in development and in production without worrying about environment discrepancies. Let’s take a look at a simple Dockerfile for a Java application:
# Choose a base Java image
FROM openjdk:11-jre-slim
# Set the working directory
WORKDIR /app
# Copy the executable .jar file into the container
COPY target/myapp.jar /app/myapp.jar
# Run the application
ENTRYPOINT ["java", "-jar", "myapp.jar"]
Commentary on the Dockerfile
- OpenJDK Image: We’re starting with a slim base image of OpenJDK to keep our application lightweight.
- Work Directory: Setting a working directory allows us to avoid potential path issues when running commands inside the container.
- COPY Command: This ensures that our application is encapsulated within the container for portable deployment.
- ENTRYPOINT: This directive specifies the command that should run when the container starts.
By leveraging this Docker architecture, you minimize the risks associated with deploying a Java EE application in inconsistent environments.
2. Simplified Deployment Process
Docker simplifies the deployment process through container orchestration tools like Docker Compose and Kubernetes. By bundling all necessary components, developers can replace or update containers with new builds. For instance, using Docker Compose, you can define and run multi-container applications with a single file. Here's a sample docker-compose.yml
for a Java application and its database:
version: '3.8'
services:
app:
build: .
ports:
- "8080:8080"
depends_on:
- db
db:
image: postgres:13
environment:
POSTGRES_DB: mydatabase
POSTGRES_USER: user
POSTGRES_PASSWORD: password
ports:
- "5432:5432"
Commentary on the Compose File
- Services Declaration: We define multiple services (the Java app and a Postgres database).
- Build Command: The app service uses the Dockerfile in the current directory to build an image.
- Port Mapping: Ports are mapped from the host to the container, allowing for external access.
- Dependency Management: The
depends_on
directive helps manage service dependencies cleanly.
3. Modifying Code Without Restarts
A major advantage of integrating Docker into Java EE development is that you can modify source code and instantly rebuild your Docker image. By optimizing the build process, you can even change your code without losing the state of your application. Below is a revised workflow for developers:
- Modify your Java code.
- Build the Docker image:
docker build -t myapp:latest .
- Redeploy the container:
docker run -d -p 8080:8080 myapp:latest
Automation for Increased Efficiency
Automation can further enhance this process. Tools like Jib can build Docker images directly from Maven or Gradle without requiring Docker to be installed. Here's a basic pom.xml
snippet for Maven users:
<build>
<plugins>
<plugin>
<groupId>com.google.cloud.tools</groupId>
<artifactId>jib-maven-plugin</artifactId>
<version>3.1.4</version>
<configuration>
<to>
<image>myapp:latest</image>
</to>
</configuration>
</plugin>
</plugins>
</build>
Commentary on Jib Configuration
- Plugin Configuration: The Jib plugin allows you to easily specify the target image.
- No Dockerfile Needed: Jib automatically handles image building, bypassing conventional Dockerfile requirements.
4. Stable State Management
Another critical aspect of hot deployment is managing application state. In a traditional setup, you often lose the current state during restarts. Docker simplifies state preservation by allowing you to use volumes. For instance, you can persist database data even if you restart your app. Here’s how you can modify your docker-compose.yml
to add a volume:
db:
image: postgres:13
volumes:
- db_data:/var/lib/postgresql/data
Commentary on Docker Volumes
- Volume Declaration: This ensures database entries will persist irrespective of container restarts, addressing one of the prominent worries with hot deployment.
The Last Word
Docker emerges as a powerhouse tool in overcoming the complexities of hot deployment in Java Enterprise applications. Its ability to create consistent environments, simplify deployment processes, enable real-time code updates, and manage application state efficiently makes it an invaluable asset.
By implementing Docker, developers can minimize downtime, significantly improving productivity. As the Java ecosystem continues to evolve, it's crucial to leverage tools like Docker to stay competitive.
For further reading, check out the official Docker documentation and consider exploring advanced techniques like CI/CD for streamlined development cycles.
Embracing a modern deployment strategy is not just an option; it's essential for today's fast-paced development world. By harnessing the power of Docker, you can focus more on coding and less on deployment headaches. Happy coding!