Overcoming Retry Challenges in Web Service Operations
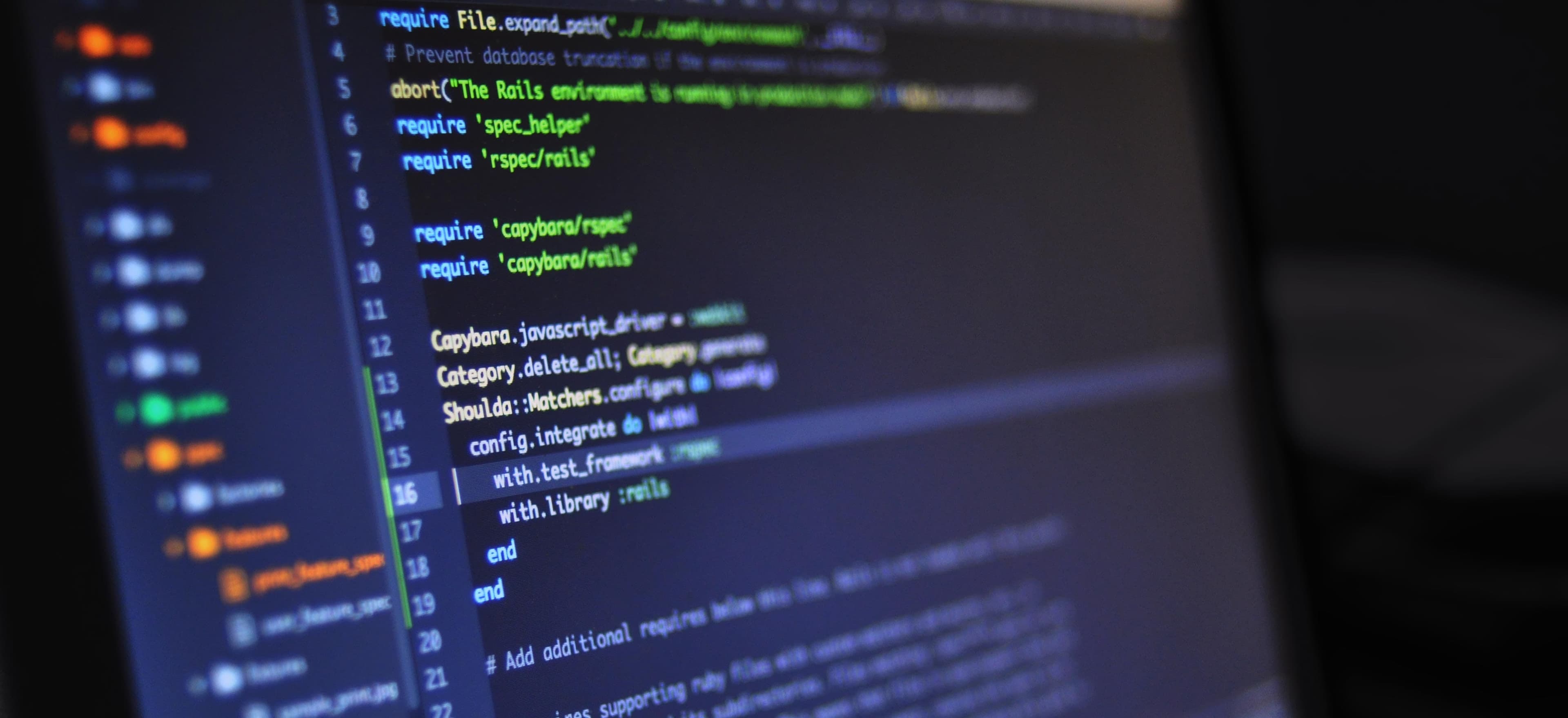
- Published on
Overcoming Retry Challenges in Web Service Operations
In the world of web services, network communication is often unreliable. When a system is under load or when there are intermittent connectivity issues, requests can fail. One common strategy to handle these failures is implementing retry logic. In this blog post, we will discuss how to effectively implement retry mechanisms in Java applications that consume web services, the challenges that come with it, and how to overcome those challenges.
The Need for a Retry Mechanism
When interacting with web services, failures can occur due to several reasons:
- Transient Network Issues: Temporary loss of connectivity or timeout.
- Service Overload: The server is under heavy load and cannot process requests.
- Rate Limiting: Some APIs limit the number of requests to prevent abuse.
Adding a retry mechanism can enhance the robustness of your application, allowing it to recover gracefully from temporary issues.
Why Retry Logic Matters
Retry logic can ensure your application performs optimally, minimizes disruptions, and improves user experience. However, the implementation of retry mechanisms is not as straightforward as it sounds. Poorly designed retries can lead to issues like:
- Increased Load: Excessive retries might overload the service.
- Unpredictable Response Times: Infinite loops can lead to poor performance.
- Violation of API Policies: Some APIs have strict rate limits.
Implementing Retry Logic in Java
Let's explore a simple, yet effective, way to implement retry logic in a Java application using an example of a RESTful web service client.
Step-by-Step Implementation
- Basic Retry Logic: Start with a simple attempt to call the web service.
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
public class WebServiceClient {
private static final int MAX_RETRIES = 3;
public String callService(String serviceUrl) {
for (int attempt = 0; attempt < MAX_RETRIES; attempt++) {
try {
URL url = new URL(serviceUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
if (connection.getResponseCode() == 200) {
// Successful response
return readResponse(connection);
} else {
// Log or handle non-200 responses as needed
System.out.println("Received non-200 response: " + connection.getResponseCode());
}
} catch (IOException e) {
if (attempt == MAX_RETRIES - 1) {
// Log the exception for the final attempt
System.err.println("Failed to call service after " + MAX_RETRIES + " attempts: " + e.getMessage());
}
// Brief sleep before retrying
try {
Thread.sleep(1000); // basic backoff
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
}
}
}
return null; // or handle as needed
}
private String readResponse(HttpURLConnection connection) {
// Logic to read from InputStream and return response
return "response"; // placeholder
}
}
Why This Code Works
- Max Retries: The constant
MAX_RETRIES
defines how many attempts the application will make before giving up. This avoids infinite retry loops. - Error Handling: Catching
IOException
ensures that the application can respond to transient issues. - Delay between Retries: Implementing a
Thread.sleep()
call introduces a simple backoff mechanism, reducing the load on the service.
Enhancements to Retry Logic
While the basic implementation is acceptable, there are many enhancements we can make:
- Exponential Backoff: Instead of a fixed delay, increase the wait time after each failed attempt.
private void exponentialBackoff(int attempt) throws InterruptedException {
Thread.sleep((long) Math.pow(2, attempt) * 100); // 100ms, 200ms, 400ms, etc.
}
- Jitter: Add randomness to the delay to avoid the "thundering herd" problem.
long delay = (long) Math.pow(2, attempt) * 100;
long jitter = (long) (Math.random() * 100);
Thread.sleep(delay + jitter);
- Circuit Breaker Pattern: Use a circuit breaker to prevent continuous overload of the service when it is down.
Example of Circuit Breaker Implementation (Pseudo-Code)
class CircuitBreaker {
private boolean isOpen = false;
public boolean allowRequest() {
return !isOpen;
}
public void recordFailure() {
// Logic to track failures and open circuit if threshold is reached
}
public void reset() {
isOpen = false;
}
}
public String callServiceWithCircuitBreaker(String serviceUrl) {
CircuitBreaker circuitBreaker = new CircuitBreaker();
if (!circuitBreaker.allowRequest()) {
System.err.println("Circuit is open! Request rejected.");
return null;
}
try {
return callService(serviceUrl);
} catch (Exception e) {
circuitBreaker.recordFailure();
// Handle failure
}
}
Best Practices for Handling Retries
- Limit Retries: Ensure that you do not set too many retries that might overwhelm the service.
- Monitor and Log: Keep track of retry attempts for diagnostics.
- Integrate with Existing Libraries: Frameworks like Spring Retry or Resilience4j provide more robust implementations of retry logic.
- Test Thoroughly: Simulate different failure scenarios to ensure that your retry logic performs as expected.
Final Thoughts
Implementing a well-structured retry mechanism can significantly improve the robustness of your Java applications interacting with web services. However, it's essential to handle retries with care—balance between resilience and avoiding service overload, and be mindful of the intricacies of error handling.
By utilizing the techniques and code patterns provided in this post, you can build a fault-tolerant application that successfully communicates with web services even when issues arise. For more advanced patterns, consider exploring frameworks that enhance fault tolerance and response handling.
For further reading, check out:
- Spring Retry Documentation
- Resilience4j Documentation
By understanding and implementing these strategies, you'll be well-equipped to tackle the challenges of retry mechanisms in web service operations. Happy coding!
Checkout our other articles