Unlocking Java 9: Common Module System Pitfalls to Avoid
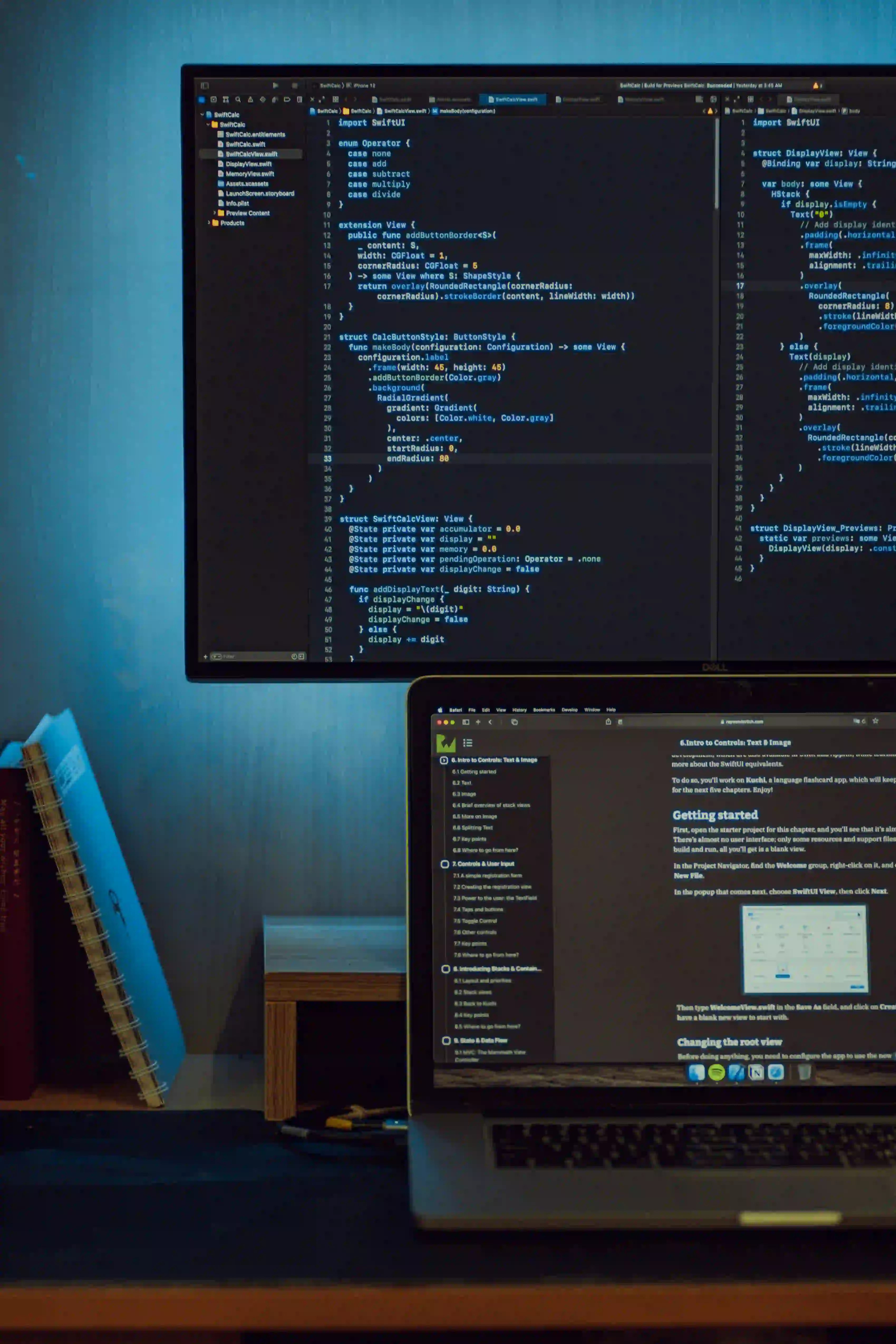
Unlocking Java 9: Common Module System Pitfalls to Avoid
Java 9 introduced a significant overhaul to the Java platform with the introduction of the Java Platform Module System (JPMS), also known as Project Jigsaw. With the promise of improved encapsulation, better performance, and more maintainable code, the module system can be an edge in developing Java applications. However, migrating to a modular system has its challenges. This blog post aims to explore common pitfalls developers encounter and how to overcome them.
Understanding the Module System
At its core, JPMS divides the Java runtime into a set of modules, which are essentially groups of packages. Each module defines its dependencies and explicitly declares which packages it exports. This modular design encourages cleaner architecture and aids in reducing the classpath complexity that often tormented developers with earlier Java versions.
Basic Structure of a Java Module
A Java module is defined using a module-info.java
file, which includes the module's name and its dependencies. Below is a simple example:
module com.example.myapp {
requires java.base; // this is by default for all modules
exports com.example.myapp.service;
}
- Module Name:
com.example.myapp
is the name of the module. - Requires: The
requires
statement indicates dependencies on other modules. - Exports: The
exports
statement controls which packages are accessible to other modules.
Pitfall 1: Ignoring the Module Dependencies
One of the first common pitfalls is neglecting to properly manage module dependencies, leading to runtime errors.
For instance, if a module tries to access a class from another module without declaring it, a javac
or java
command will fail with an error indicating that the module does not have access to the package.
Example of a Missing Dependency Error:
Imagine you have two modules: moduleA
and moduleB
. If moduleA
relies on a class from moduleB
but does not include the requires
statement, you would encounter an error.
// In moduleA
module moduleA {
requires moduleB; // Ensure you declare this to access moduleB's classes
}
Tip: Always review dependencies in your module-info.java
files. Using tools like jdeps can help identify weak points in your module structure.
Pitfall 2: Over-Exporting Packages
Over-exporting packages may seem more convenient initially, but it can lead to a lack of encapsulation, one of the fundamental goals of the module system. You may inadvertently create tight coupling between modules, making your system less maintainable and more challenging to evolve.
Example:
module com.example.myapp {
exports com.example.myapp.service; // This is fine
exports com.example.myapp.internal; // Risky if used widely
}
In the second exports
statement, exposing internal
may disrupt the intention behind modular encapsulation.
Tip: Export only what is necessary. This will reduce the risk of unintended access and violations of encapsulation principles, enhancing the maintainability of your code.
Pitfall 3: Confusing Module and Package Names
Another frequent confusion arises from the naming of modules and packages. Developers may often use package names in modules, leading to potential clashes and ambiguity. The module names should clearly reflect their functionality without being tightly coupled with specific packages.
Good Naming Example:
module com.example.authentication {
exports com.example.authentication.service;
}
Poor Naming Example:
module com.example.authentication.service { // This leads to ambiguity
exports com.example.authentication.service;
}
Tip: Use a clear, hierarchical naming scheme for your modules and packages. Modular naming reflects the purpose and area of responsibility cleanly, making maintenance and collaboration easier.
Pitfall 4: Failing to Support Non-Modularized Code
It is crucial to recognize that not all existing libraries and frameworks have migrated to the module system. If your application uses such a library, it may create runtime exceptions if your application is fully modularized.
Example:
If a project relies on a legacy library that doesn't have modular support, referencing it as a module might break the build.
// Module attempts to use a non-module
module com.example.myapp {
requires old.library; // This will cause issues
}
Tip: Use the --patch-module
option when compiling or running your code to add non-modularized libraries to your module path. This can help mitigate issues during the transition phase.
Additional Resources for Further Reading
- JPMS Documentation
- Modular Programming in Java
Lessons Learned
In summary, while the Java Platform Module System presents an opportunity to enhance code quality and modularity, it comes with its own set of complexities. Awareness of common pitfalls ensures you can navigate the complexities of Java 9 with better foresight and preparation.
Avoiding these pitfalls involves careful planning and understanding of module dependencies, proper encapsulation, coherent naming conventions, and managing compatibility with non-modularized code.
By effectively leveraging these insights, developers can unlock the full potential of Java 9 and ensure that their applications are not only robust but also more maintainable in the long run.
Happy coding!