How to Avoid Common Pitfalls in TDD Sanity Checks
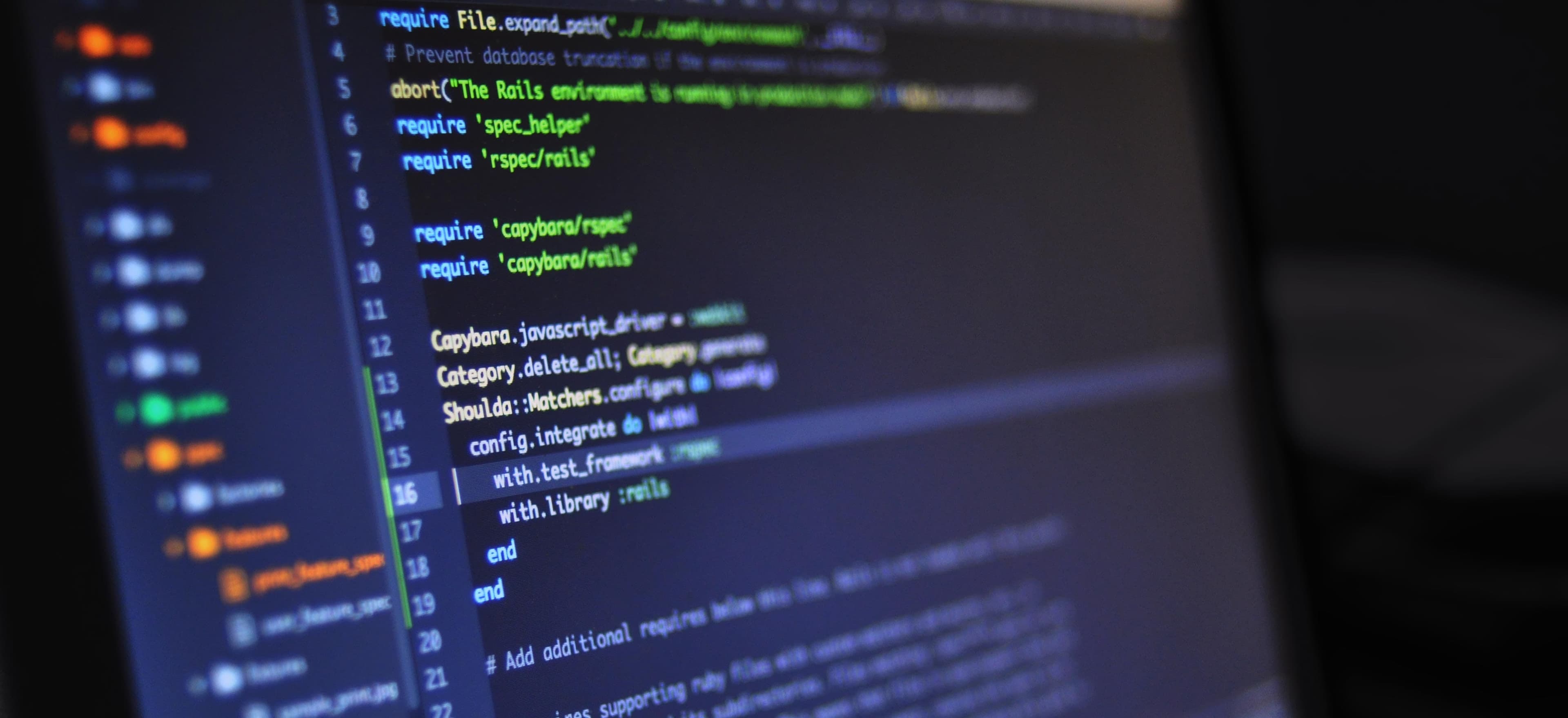
- Published on
How to Avoid Common Pitfalls in TDD Sanity Checks
Test-Driven Development (TDD) has emerged as a cornerstone of modern software development. It encourages writing tests before developing the actual code, fostering an iterative approach that can lead to more reliable and maintainable software. However, despite its advantages, many developers encounter pitfalls when conducting TDD sanity checks. This blog post discusses these pitfalls and provides strategies to avoid them.
Understanding TDD and Sanity Checks
TDD consists of three primary steps:
- Red: Write a failing test case for a new function.
- Green: Implement the function just enough to make the test pass.
- Refactor: Clean up the code while ensuring that all tests still pass.
Sanity checks are a form of quick assessments that help determine if your code is functioning correctly at a high level. They are often simple tests run to check basic functionalities without delving into exhaustive scenarios.
Why Sanity Checks Matter
Sanity checks are crucial because they:
- Provide immediate feedback on changes in the codebase.
- Help catch simple mistakes early in the development process.
- Serve as a lightweight method to ensure that fundamental features work as intended.
However, when not executed correctly, they can lead to a false sense of security about the quality of your code. Let's explore common pitfalls in TDD sanity checks and how to sidestep them.
Common Pitfalls in TDD Sanity Checks
1. Relying Solely on Sanity Checks
A common mistake developers make is to rely exclusively on sanity checks and neglect rigorous testing. While sanity checks can catch obvious issues, they cannot substitute for a comprehensive suite of automated tests.
Solution
Always complement your sanity checks with unit tests and integration tests. For instance, if you have a function that processes user input, your sanity check might assess if the function runs without error. However, it would be more effective to write a unit test that checks for correct outputs with various inputs.
public class InputProcessor {
public String processInput(String input) {
// Logic to process input
return input.trim();
}
}
public class InputProcessorTest {
@Test
public void testProcessInput() {
InputProcessor processor = new InputProcessor();
String result = processor.processInput(" Hello World ");
assertEquals("Hello World", result); // Checks if spaces are trimmed
}
}
This unit test confirms expected behavior beyond mere execution.
2. Ignoring Edge Cases
Sanity checks should cover common scenarios, but overlooking edge cases can lead to significant issues down the line. Developers often assume that if the basic functionality works, everything else will function properly.
Solution
Be mindful of edge cases and integrate them into your sanity checks. For instance, if your function adds two numbers, ensure that your tests also evaluate extreme values like zero and negative numbers.
public class MathOperations {
public int add(int a, int b) {
return a + b;
}
}
public class MathOperationsTest {
@Test
public void testAdd() {
MathOperations operations = new MathOperations();
assertEquals(5, operations.add(2, 3)); // Common case
assertEquals(0, operations.add(0, 0)); // Edge case 1
assertEquals(-1, operations.add(2, -3)); // Edge case 2
}
}
Incorporate edge cases into your test coverage, ensuring your code is robust across a wider range of scenarios.
3. Not Running Tests Frequently
Another frequent error is neglecting to run sanity checks and other tests often. The longer code remains untested, the more likely it is to deviate from its expected behavior.
Solution
Incorporate continuous integration (CI) tools into your development workflow. Tools like Jenkins or CircleCI automatically run tests every time changes are pushed to the repository, catching issues early.
4. Failing to Maintain Tests
As your codebase evolves, neglecting to update or refactor your tests can lead to outdated sanity checks that no longer reflect current functionality. This can result in false positives or negatives.
Solution
Make it a practice to review and adjust your sanity checks alongside your code changes. Regularly revisiting tests during the refactoring phase is vital for maintaining their relevance.
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User createUser(String name) {
User user = new User(name);
userRepository.save(user);
return user;
}
}
// Keep your tests in sync with changes in your application
public class UserServiceTest {
@Test
public void testCreateUser() {
UserRepository mockUserRepository = Mockito.mock(UserRepository.class);
UserService userService = new UserService(mockUserRepository);
User user = userService.createUser("Alice");
verify(mockUserRepository).save(user); // Updated to verify that a user is persisted
}
}
5. Overcomplicating Sanity Checks
Sanity checks should be straightforward and quick to run. A common error is creating overly complex tests that dilute their purpose.
Solution
Keep sanity checks simple. Limit them to high-level functionality and keep them easy to read and maintain. If a specific feature requires complicated testing, consider writing dedicated unit or integration tests instead.
Final Thoughts
TDD is an excellent approach to software development, and sanity checks can be a valuable tool. However, avoiding common pitfalls is essential for reaping the most benefits from this testing strategy. By ensuring thorough test coverage, being mindful of edge cases, leveraging CI, keeping tests maintained, and simplifying sanity checks, developers can significantly improve software quality.
For more in-depth coverage of TDD best practices, consider checking out Martin Fowler's article on TDD. Embracing these principles will set you on a path to developing robust, maintainable, and high-quality software.
By being conscious of these pitfalls, you will not only write better tests but also cultivate a more efficient development lifecycle, allowing you to focus more on innovation and less on firefighting bugs post-deployment. Happy coding!
Checkout our other articles