Common Docker Pitfalls for Java Developers to Avoid
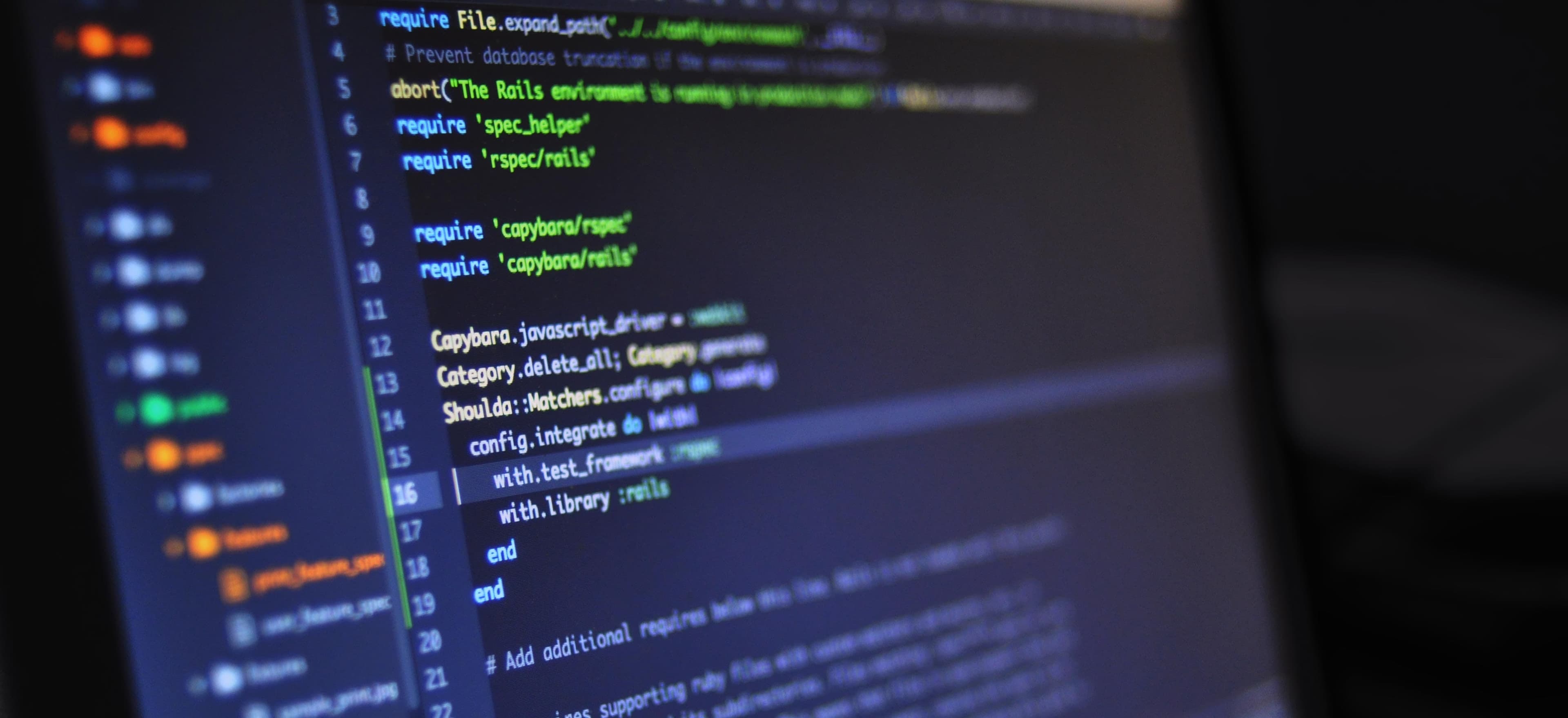
- Published on
Common Docker Pitfalls for Java Developers to Avoid
In today’s software development landscape, containerization has become a key enabling technology, especially for Java developers. Docker, leading the charge, allows developers to package applications and dependencies into containers, ensuring portability and minimizing environment-specific bugs. However, in the quest for efficient containerization, developers can gravitate toward several pitfalls that can diminish their productivity and compromise application performance. This blog post explores these common Docker pitfalls in detail, providing actionable strategies to avoid them.
1. Bloated Images
When building Docker images, one of the most common mistakes Java developers make is leaving unnecessary files and dependencies in their images, leading to bloated images. A larger image results in longer build times and slower deployment speed.
Solution: Multi-Stage Builds
Using Docker's multi-stage builds is a powerful technique to reduce image size. You can create multiple build stages, isolating the build environment from runtime dependencies.
# Stage 1: Build
FROM maven:3.6.3-jdk-11 AS build
WORKDIR /app
COPY pom.xml .
COPY src ./src
RUN mvn clean package -DskipTests
# Stage 2: Production
FROM openjdk:11-jre-slim
COPY --from=build /app/target/myapp.jar /app/myapp.jar
ENTRYPOINT ["java", "-jar", "/app/myapp.jar"]
Why This Works: In this example, the first stage uses Maven to build the application, including all dependencies. The final image only contains the JRE with the compiled .jar
file, drastically reducing size and improving performance.
2. Ignoring the Use of .dockerignore
Many developers overlook the .dockerignore
file, which serves a similar purpose to .gitignore
. This omission can lead to unnecessary files being included in your Docker images, further contributing to their bloat.
Solution: Create a Proper .dockerignore
File
target/
*.log
*.class
Dockerfile
.git
Why This Works: By instructing Docker to ignore directories and files that are not needed in the final image, you minimize the context sent to the build server, resulting in faster builds and smaller images.
3. Not Using Environment Variables
Hardcoding sensitive information (like database connection strings) and other configurations directly into your Docker images is a mistake. This practice can expose sensitive data and makes it difficult to change configuration without rebuilding the image.
Solution: Use Environment Variables
You can set environment variables in the Dockerfile or at runtime to pass configuration values.
ENV DB_URL=jdbc:mysql://localhost:3306/mydb
docker run -e DB_URL=jdbc:mysql://db:3306/mydb my-java-app
Why This Works: This approach makes your image more flexible and conducive to different environments, enhancing security by avoiding hardcoded values.
4. Running as Root User
By default, most Docker containers run as the root user. This can be a severe security risk, especially for production applications, as it exposes the container to potential vulnerabilities.
Solution: Run as Non-Root User
In your Dockerfile, you can specify a non-root user:
RUN groupadd -r app && useradd -r -g app app
USER app
Why This Works: By running your applications as a non-root user, you significantly reduce the attack surface, enhancing the security of your containerized applications.
5. Not Leveraging Caching Effectively
Docker uses a layered file system and caches layers during the image build process. Failing to structure your Dockerfile to maximize caching can lead to inefficient builds.
Solution: Order Dockerfile Instructions Wisely
# Put dependency files first
COPY pom.xml .
RUN mvn dependency:go-offline
# Then copy the rest
COPY src ./src
RUN mvn clean package -DskipTests
Why This Works: By separating the dependency installation step from the application code copy step, Docker can cache the dependencies. If you make changes to the application code, Docker will reuse the cached layers for the dependencies, speeding up the build process.
6. Overusing Docker Volumes
One of Docker's powerful features is the ability to mount volumes. However, using this feature excessively can lead to complications, especially when it comes to application portability. External dependencies may not be consistent across multiple environments.
Solution: Use Volumes Judiciously
While it's appropriate to leverage volumes for persistent data storage (like databases), avoid relying on them for application data or configurations unless necessary.
Why This Works: By keeping your application self-contained within the Docker image, you maintain portability across different environments, simplifying deployment and reducing environment-specific issues.
7. Excessive Container Restarting
A common pitfall is not adequately handling application crashes, leading to Docker containers that endlessly restart without resolution.
Solution: Implement Health Checks
In your Dockerfile, you can specify health checks that determine whether your application is functioning correctly.
HEALTHCHECK CMD curl --fail http://localhost:8080/health || exit 1
Why This Works: By integrating health checks, Docker can identify when a container becomes unhealthy and stop it, preventing infinite restarts. This gives you the chance to debug and rectify the underlying issues.
8. Not Monitoring Container Performance
Many developers underestimate the importance of monitoring Docker containers in production. Without your application’s performance metrics, identifying bottlenecks or failures becomes challenging.
Solution: Use Monitoring Tools
Several tools, such as Prometheus and Grafana, can be integrated to track your Docker container's performance.
Why This Works: Monitoring tools provide visibility into your containers, helping you catch issues early and optimize resource usage in real-time.
The Last Word
Docker has undoubtedly revolutionized how Java developers package and deploy their applications. However, steering clear of common pitfalls is vital for reaping the full benefits of Docker’s features. By focusing on best practices, such as using multi-stage builds, managing environment variables carefully, and implementing monitoring, your Docker experience can be more efficient and secure.
For further reading on Docker and Java best practices, check out the official Docker documentation and Java Toolbox. By staying informed and proactive, you can ensure that your Java applications run smoothly in containers, paving the way for faster development cycles and more resilient applications.
Happy Dockerizing!
Checkout our other articles