When Microservices Fail: Common Pitfalls to Avoid
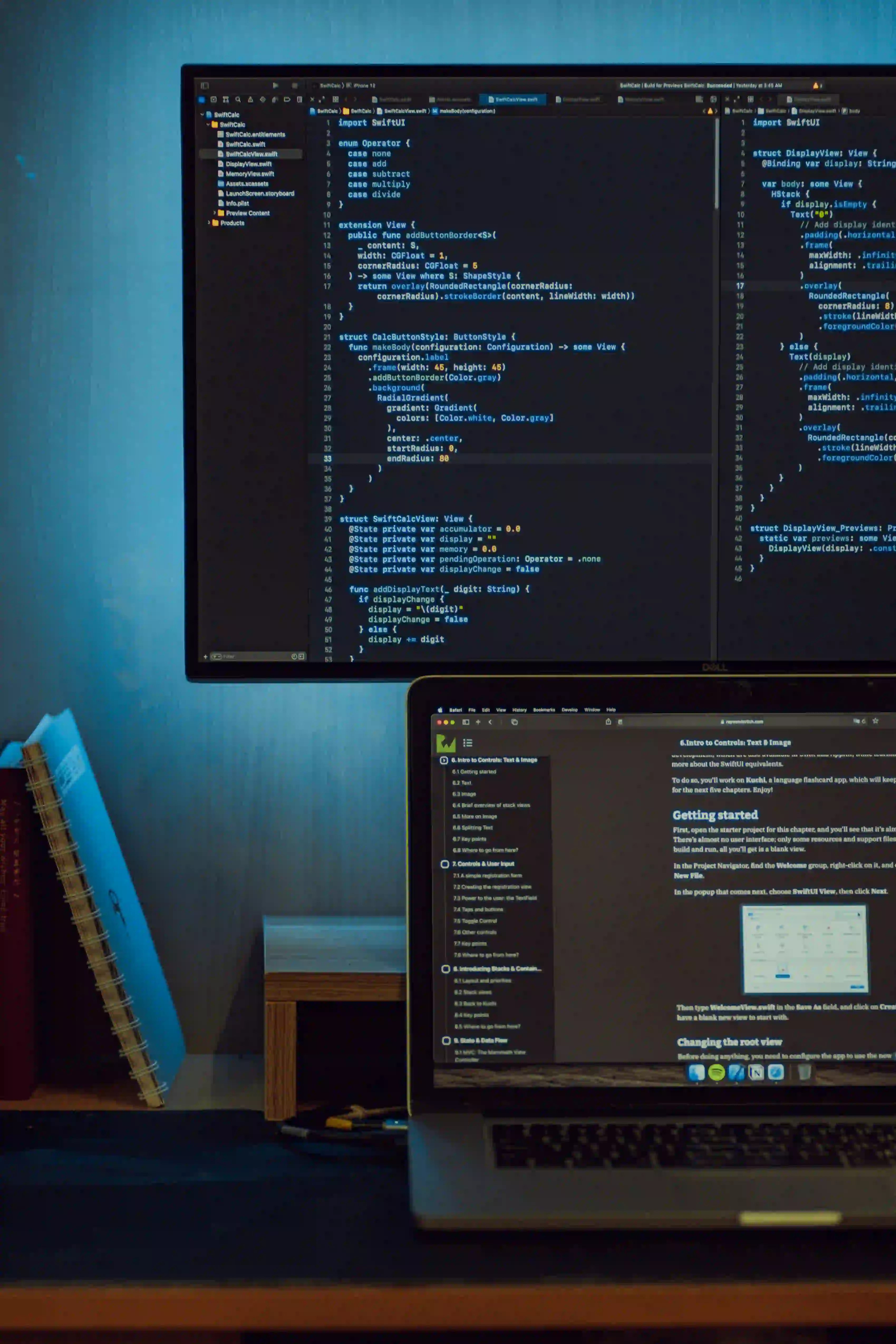
When Microservices Fail: Common Pitfalls to Avoid
The microservices architecture has rapidly gained popularity among organizations looking to build scalable, flexible, and resilient applications. However, the shift from a monolithic to a microservices architecture is not without its challenges. In this blog post, we will explore some common pitfalls that developers and organizations encounter when adopting microservices, as well as strategies to avoid these traps.
Understanding Microservices
Before diving into the pitfalls, it's essential to clarify what microservices are. Microservices architecture involves developing applications as a collection of loosely coupled services. Each microservice can be deployed, scaled, and updated independently. This offers several advantages, including flexibility and rapid deployment, but it also introduces complexity.
Common Pitfalls and How to Avoid Them
-
Lack of Proper Service Design
One of the most critical aspects of a successful microservices architecture is proper service design. When services are too granular or too large, it can lead to communication overhead, bottlenecks, and difficulties in management.
Solution: Aim for a well-balanced approach. Each microservice should have a single responsibility and manage a specific business capability. Applying the Single Responsibility Principle (SRP) in your design helps in maintaining clear boundaries.
☕snippet.javapublic class OrderService { public void createOrder(Order order) { // Logic for creating an order } }
By having focused services, you will minimize the interdependencies that often complicate microservices architectures.
-
Ignoring Data Management Challenges
In a monolithic architecture, a single database can suffice. However, microservices usually require multiple database instances, and this can lead to issues regarding data consistency and management.
Solution: Implement a saga pattern to handle distributed transactions. This pattern breaks a transaction into smaller, manageable parts, allowing for eventual consistency. Here’s an example of how you could implement this in Java:
☕snippet.javapublic class PaymentService { public void processPayment(Order order) { // Process payment logic // If payment fails, trigger rollback for previous transactions if (!paymentSuccessful) { rollBackOrder(order); } } }
This helps ensure that if one microservice fails, the entire system remains consistent, leading to less data fragility.
-
Overlooked Monitoring and Logging
With multiple services running independently, monitoring becomes significantly more complex. Without adequate monitoring, diagnosing issues can become a nightmare.
Solution: Incorporate centralized logging and monitoring tools like ELK Stack or Prometheus. Use structured logging in your applications to capture essential metrics.
☕snippet.javaimport org.slf4j.Logger; import org.slf4j.LoggerFactory; public class UserService { private static final Logger logger = LoggerFactory.getLogger(UserService.class); public void registerUser(User user) { logger.info("Registering user: {}", user.getEmail()); // Registration logic... } }
Having structured and organized logs will significantly enhance your ability to trace and monitor system behavior, leading to quicker resolutions.
-
Neglecting API Design
In a microservices architecture, APIs act as the primary communication channel between services. Poorly designed APIs can lead to unnecessary complexity and tight coupling between microservices.
Solution: Follow RESTful principles or consider using GraphQL for flexible queries. Consider using API gateways like Spring Cloud Gateway that provide a single entry point for external clients to interact with your microservices.
☕snippet.java@RestController public class UserController { @Autowired private UserService userService; @PostMapping("/users") public ResponseEntity<User> createUser(@RequestBody User user) { User createdUser = userService.registerUser(user); return new ResponseEntity<>(createdUser, HttpStatus.CREATED); } }
Properly designed APIs not only facilitate better communication but also promote separation of concerns among services.
-
Failing to Manage Inter-Service Communication
Microservices heavily rely on network communications, which can introduce latency and potential points of failure. Developers may unwittingly design services that are overly dependent on each other.
Solution: Use asynchronous communication methods and message brokers, such as RabbitMQ or Kafka. This reduces the interdependencies between services and abstracts the communication layer.
☕snippet.javapublic class NotificationService { private final MessageBroker messageBroker; public NotificationService(MessageBroker messageBroker) { this.messageBroker = messageBroker; } public void sendEmailNotification(String email) { // Send notification asynchronously messageBroker.publish(new EmailMessage(email)); } }
By using asynchronous messaging, services can function independently and gracefully handle network issues.
-
Underestimating Operational Complexity
Managing microservices can lead to operational challenges, including deployment, scaling, and orchestration. Developers may underestimate the importance of DevOps practices.
Solution: Adopt containerization (e.g., using Docker) and orchestration tools (such as Kubernetes). These tools ease deployment and scaling tasks, allowing microservices to adapt more readily to changing demands.
⚙️snippet.ymlapiVersion: apps/v1 kind: Deployment metadata: name: order-service spec: replicas: 3 selector: matchLabels: app: order-service template: metadata: labels: app: order-service spec: containers: - name: order-service image: order-service:latest ports: - containerPort: 8080
Deploying your microservices with Kubernetes will help you manage your applications on a global scale without losing sight of individual service performance.
-
Not Prioritizing Security
The complexity of microservices often leads to security oversight. Each additional service creates a new surface area for potential vulnerabilities.
Solution: Implement security best practices, such as OAuth2 for authentication and HTTPS for secure communication. Ensure that all microservices validate inputs and authenticate requests.
☕snippet.java@RestController @RequestMapping("/api/v1/orders") public class OrderController { @PreAuthorize("hasRole('USER')") @GetMapping("/{orderId}") public Order getOrder(@PathVariable String orderId) { // Fetch the order details } }
By embedding security measures like role-based access control into your services, you safeguard your application against unauthorized access.
My Closing Thoughts on the Matter
Transitioning to microservices offers remarkable benefits but also presents multiple challenges. By understanding and addressing the common pitfalls outlined in this blog post, organizations can implement microservices in a way that maximizes their advantages while minimizing risk.
For a deeper dive into microservices, consider checking out resources like Martin Fowler’s Microservices and Microsoft's Microservices Architecture. With careful planning, design, and execution, moving toward a microservices architecture not only becomes possible but also manageable and rewarding.
By understanding these critical pitfalls, you can pave the way to a successful microservices implementation, setting your project up for long-term success! If you have encountered other challenges, please share your experiences in the comments below.