Common Spring Framework Mistakes and How to Avoid Them
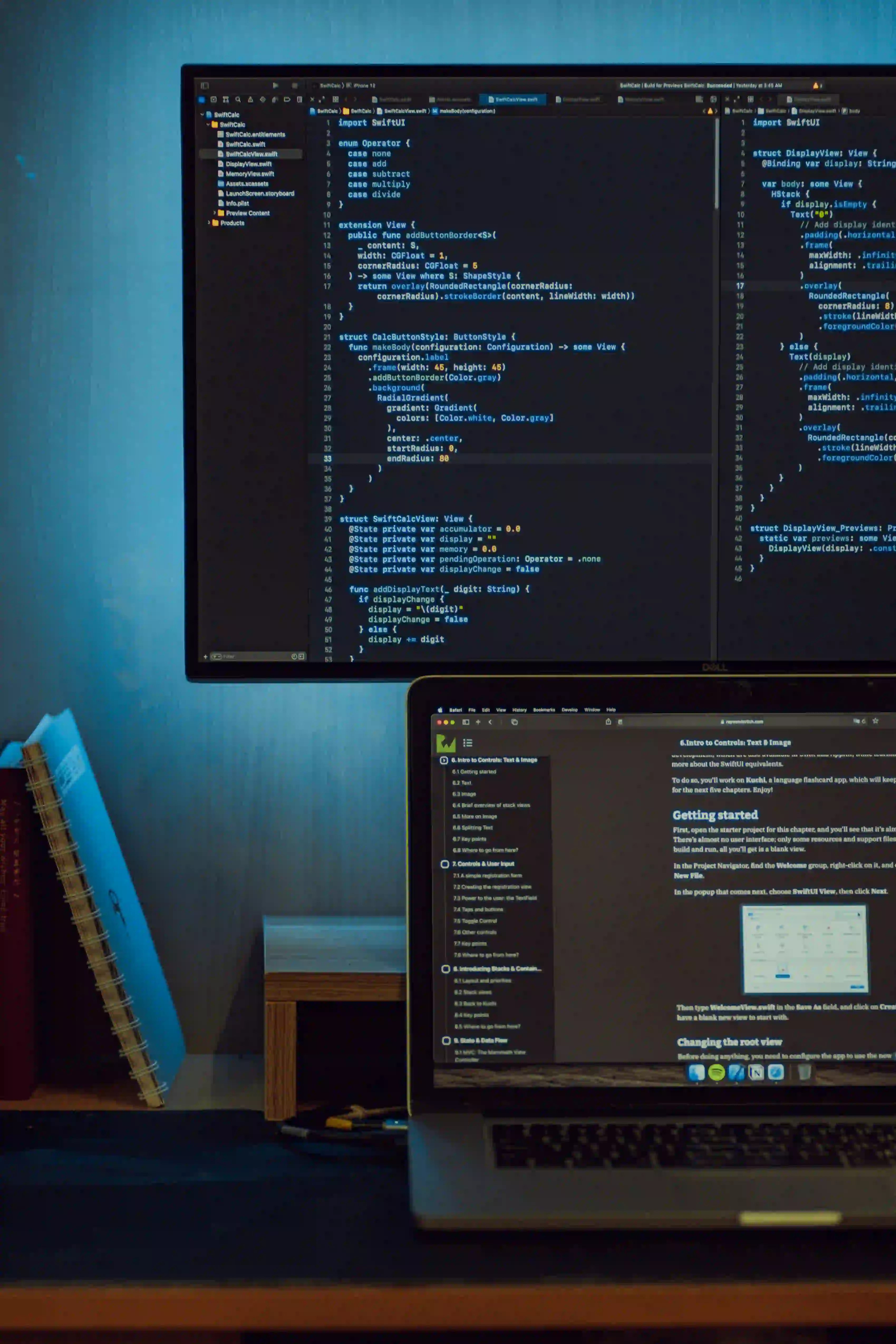
Common Spring Framework Mistakes and How to Avoid Them
The Spring Framework has become the backbone of many Java applications due to its robust features and comprehensive ecosystem. However, even seasoned developers can slip into common pitfalls when working with Spring. This blog post explores some frequent mistakes made by developers utilizing the Spring Framework and provides actionable tips on how to avoid them.
Mistake 1: Ignoring Dependency Injection
What is Dependency Injection?
Dependency Injection (DI) is a design pattern that implements inversion of control and is a key feature of the Spring Framework. It allows developers to create loosely coupled classes by injecting dependencies rather than creating instances directly within the classes.
The Mistake
One of the most common mistakes is manually instantiating dependencies inside a class instead of using Spring's DI capabilities.
Why is it a Mistake?
When you instantiate dependencies directly, you are tightly coupling your classes, making them less flexible and harder to test. This violates the principle of Separation of Concerns.
How to Avoid
Leverage Spring’s DI features using annotations such as @Autowired
, @Inject
, or @Resource
. Here's an example:
@Service
public class OrderService {
private final PaymentService paymentService;
@Autowired
public OrderService(PaymentService paymentService) {
this.paymentService = paymentService;
}
public void processOrder(Order order) {
paymentService.processPayment(order.getPaymentDetails());
// Other processing logic here
}
}
In this example, the OrderService
class does not create an instance of PaymentService
. Instead, it receives the PaymentService
as a constructor argument, promoting loose coupling and ease of testing.
Mistake 2: Misunderstanding Bean Scopes
What are Bean Scopes?
A bean scope defines the lifecycle of a bean (object) in Spring. The main scopes include Singleton, Prototype, Request, Session, and Global Session.
The Mistake
Developers often default to the Singleton scope without considering the implications, especially for web applications where multiple user sessions may lead to unexpected behavior.
Why is it a Mistake?
Using an inappropriate scope may lead to resource contention or unintentional shared states across users, thereby causing bugs that are hard to trace.
How to Avoid
Consider the context of your application and choose the appropriate scope. For example, if you have a service that should maintain state during a user's session, you could use the Session scope:
@Component
@Scope("session")
public class UserSession {
private String userId;
// Getters and setters
}
In this case, each user will get their own instance of UserSession
, preventing shared state issues.
Mistake 3: Overusing Annotations
The Power of Annotations
Spring is known for its extensive use of annotations like @Component
, @Service
, or @Controller
. They facilitate configuration and wiring of beans.
The Mistake
Some developers overuse annotations, leading to code that is difficult to read or understand, even for those familiar with Spring.
Why is it a Mistake?
While annotations can reduce boilerplate code, overusing them can make the codebase cluttered, difficult to maintain, and challenging for new team members to onboard quickly.
How to Avoid
Limit the use of annotations and consider other configuration approaches such as Java configuration classes or XML configuration if it leads to better readability.
Here is an example using Java configuration:
@Configuration
public class AppConfiguration {
@Bean
public OrderService orderService(PaymentService paymentService) {
return new OrderService(paymentService);
}
@Bean
public PaymentService paymentService() {
return new PaymentService();
}
}
This approach centralizes bean creation, making it clear and manageable.
Mistake 4: Neglecting Exception Handling
Why is Exception Handling Important?
Handling exceptions properly is crucial for maintaining application stability and providing a good user experience.
The Mistake
Developers may neglect structured exception handling within their services, merely logging exceptions, or worse, not managing them at all.
What Will Happen?
This can lead to uncaught exceptions, crashing your application or leaking sensitive information to users.
How to Avoid
Utilize Spring’s @ControllerAdvice
and @ExceptionHandler
to handle exceptions globally in a unified way. Here’s an example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(OrderNotFoundException.class)
public ResponseEntity<String> handleOrderNotFound(OrderNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleGenericException(Exception ex) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("An unexpected error occurred: " + ex.getMessage());
}
}
This structure ensures any unhandled order not found or other exceptions are dealt with appropriately, providing clear feedback to users and keeping the application robust.
Mistake 5: Not Utilizing Spring Profiles
What are Spring Profiles?
Spring Profiles allow developers to segregate parts of their application configuration and make it only available in certain environments (like development, testing, production).
The Mistake
Many developers ignore profiles, hardcoding configurations that lead to environments being less flexible and harder to manage.
Why is it a Mistake?
Ignoring profiles can lead to configurations that work in one environment but break in another, violating the DRY principle and complicating deployments.
How to Avoid
Define profiles in your application properties. As an example:
# application-dev.properties
spring.datasource.url=jdbc:h2:mem:testdb;DB_CLOSE_DELAY=-1;DB_CLOSE_ON_EXIT=FALSE
spring.datasource.driver-class-name=org.h2.Driver
You can activate profiles by specifying them at startup:
java -jar application.jar --spring.profiles.active=dev
This practice keeps your application configurations clean and tailored for specific environment needs.
Key Takeaways
The Spring Framework is a powerful tool for building Java applications, but it comes with its unique set of challenges. By avoiding the common mistakes outlined above—ignoring dependency injection, misunderstanding bean scopes, overusing annotations, neglecting exception handling, and not utilizing profiles—you will improve your code quality, enhance maintainability, and facilitate easier testing.
For further reading on Spring best practices, consider checking out Baeldung and the official Spring documentation. By continuously learning and adapting, you’ll become a more proficient developer in the ever-evolving landscape of Java and Spring development. Happy coding!