Mockito Core vs Inline: Which to Choose for Testing?
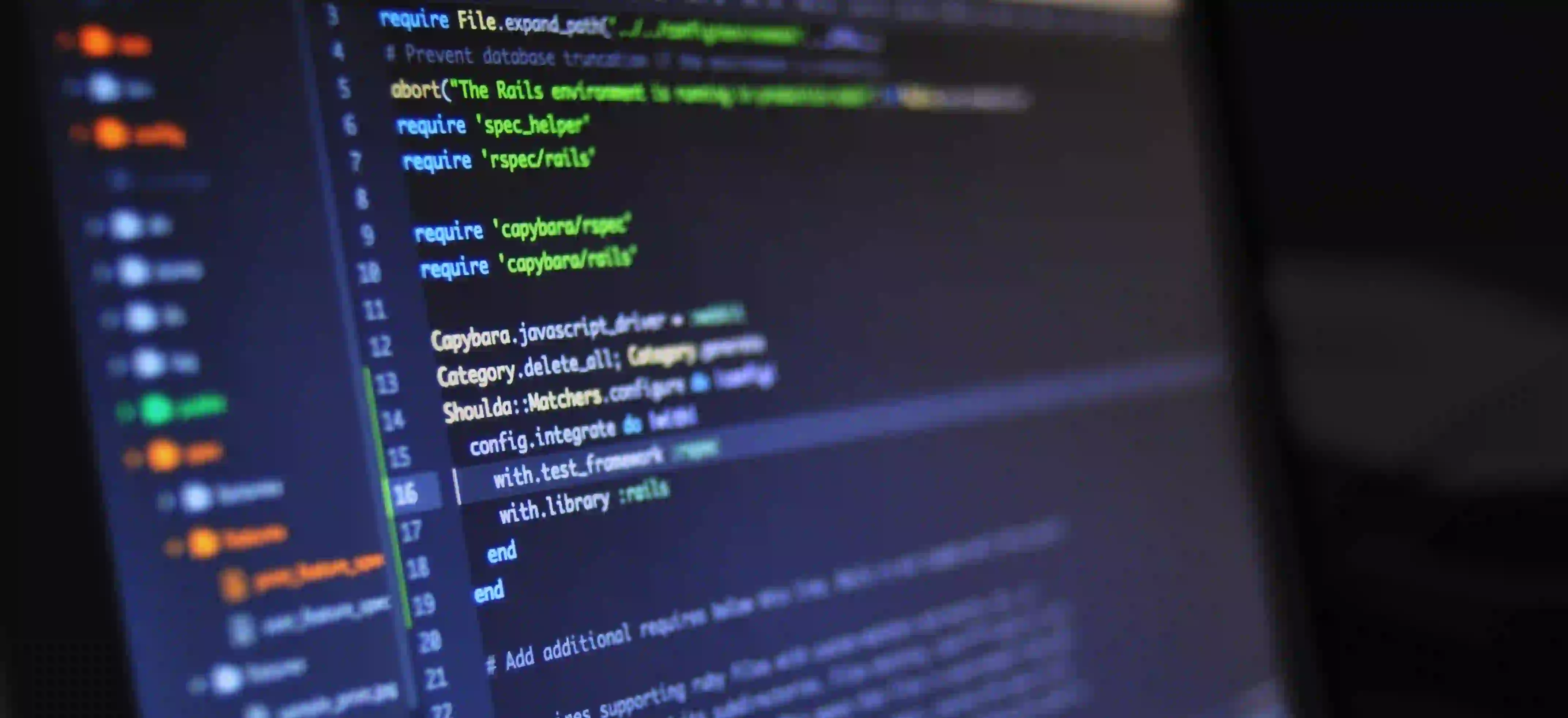
Mockito Core vs Inline: Which to Choose for Testing?
When it comes to unit testing in Java, Mockito stands out as one of the most popular frameworks. Its powerful capabilities allow developers to create mock objects, facilitating isolated testing of components. However, Mockito offers two different flavors: Mockito Core and Mockito Inline. In this post, we will explore the differences between these two variations, when to use each, and best practices to get the most out of your testing workflows.
Understanding Mockito
Mockito is a mocking framework for Java that allows you to create mock objects to simulate the behavior of real objects in your tests. This enables you to focus on testing only the component you are working on without dealing with its dependencies.
Mockito Core
Mockito Core is the original version of Mockito, designed to work with Java bytecode at runtime. It is ideal for most testing scenarios, particularly when your code adheres to standard Java programming practices. If you are using Mockito Core, you often don’t need special configuration or adapter setups.
Mockito Inline
Mockito Inline, on the other hand, is a more recent addition designed to extend Mockito’s capabilities. Specifically, it allows you to mock final classes and methods, static methods, and even private methods. This versatility makes it suitable for complex applications that involve legacy codebases or where you cannot modify class structures.
When to Use Mockito Core
Simplicity and Performance
If your unit test scenarios involve only mocking regular classes or interfaces, Mockito Core is your best bet.
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testGetUser() {
UserRepository userRepository = mock(UserRepository.class);
UserService userService = new UserService(userRepository);
User mockUser = new User("John Doe");
when(userRepository.findById(1)).thenReturn(mockUser);
User user = userService.getUser(1);
assertEquals("John Doe", user.getName());
}
}
In this example, we create a mock UserRepository and specify its behavior using the when
and thenReturn
methods. The simplicity and performance of this approach are among its main advantages.
Robust Community and Documentation
With a larger user base, Mockito Core has extensive documentation and community support. For anyone starting with testing in Java, using a well-supported framework minimizes potential issues.
When to Use Mockito Inline
Advanced Testing Scenarios
Mockito Inline is your best choice when you need to mock classes that are either final or have static methods. For example:
import static org.mockito.Mockito.*;
public class UtilityTest {
@Test
public void testStaticMethod() {
mockStatic(Utility.class);
when(Utility.performCalculation(anyInt())).thenReturn(42);
int result = Utility.performCalculation(5);
assertEquals(42, result);
}
}
In this case, Utility.performCalculation
is a static method that we want to mock. This functionality is exclusive to Mockito Inline, making it essential for scenarios like this.
Legacy Code Compatibility
If you are working with legacy code that uses final classes or methods, switching to Mockito Inline can save considerable time. Trying to refactor such code for compatibility with Mockito Core could lead to new bugs and regressions.
Performance Considerations
While Mockito Inline offers advanced features, it comes with performance penalties due to bytecode manipulation. If performance is a concern, sticking with Mockito Core is advisable. On the other hand, if you require the functionalities of Mockito Inline, keep performance implications in mind and test them thoroughly.
Best Practices
Regardless of whether you choose Mockito Core or Inline, adhering to certain best practices ensures effective unit tests:
-
Keep Tests Isolated: Your unit tests should focus on a single concern. Avoid dependencies on the state of other tests.
-
Use Descriptive Naming: Name your test methods clearly to reflect what they are testing.
-
Use
@Mock
Annotations: This makes your tests cleaner and easier to read. -
Verify Behavior: Always verify that interactions happen as expected.
☕snippet.javaverify(userRepository).findById(1);
-
Prefer Constructor Injection: This makes it easier to mock dependencies.
A Final Look
Choosing between Mockito Core and Inline depends on your specific test cases and code structure. If your application uses standard classes and interfaces, Mockito Core should suffice. However, for scenarios involving final classes or static methods, Mockito Inline becomes indispensable.
In conclusion, always consider your application needs, performance implications, and the complexity of your testing scenarios before making a decision. For deeper insights into unit testing with Mockito, you can explore the official Mockito documentation and JUnit 5 user guide.
With the right approach and understanding of both frameworks, you can enhance your testing strategies, leading to more reliable and maintainable code. Whether you're just dipping your toes into testing or you're a seasoned developer, mastering these tools can significantly boost your development efficiency. Happy Testing!