Mastering JPA: Tackle Single Table Inheritance Issues
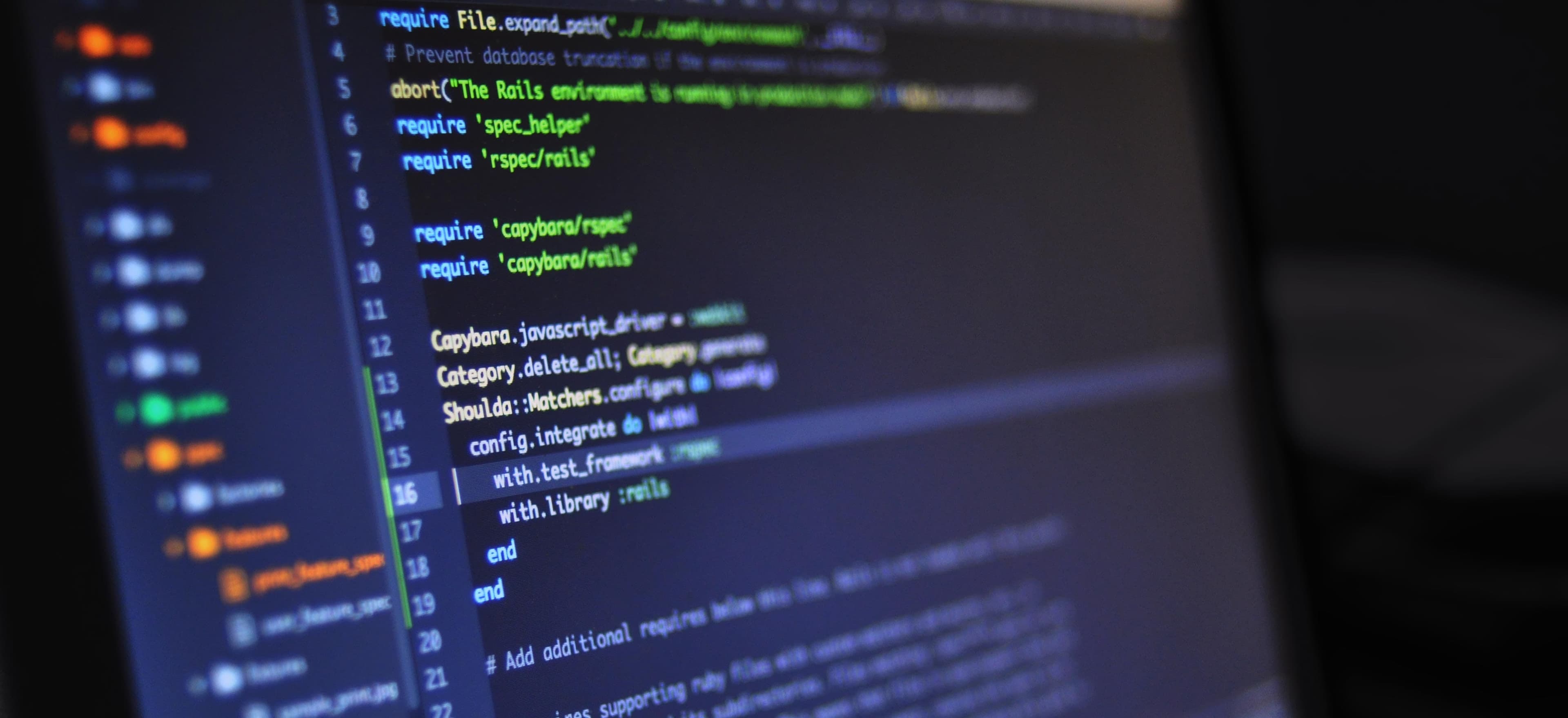
- Published on
Mastering JPA: Tackle Single Table Inheritance Issues
Java Persistence API (JPA) is a powerful tool for managing relational data in Java applications. One of the fascinating features of JPA is its support for inheritance, particularly the Single Table Inheritance strategy. However, while this method can be efficient, it is not without challenges. In this post, we'll explore the inner workings of Single Table Inheritance in JPA, discuss its advantages and pitfalls, and dive into solutions for common issues.
Understanding Single Table Inheritance
What is Single Table Inheritance?
In Single Table Inheritance, JPA stores all class data in a single table. This table contains a column (often called dtype
or type
) which differentiates between the various subclasses. The subclasses share a single structure and can store additional fields relevant to them.
The Benefits
- Performance: Having all data in one table can lead to better read performance. Fewer joins typically mean quicker queries.
- Simplicity: Organizing classes in a single table can simplify the data model and reduce the number of tables in your schema.
- Flexibility: It allows for dynamic creation of new subclasses without altering the database schema.
The Pitfalls
While Single Table Inheritance offers notable benefits, it comes with complications such as:
- Sparse tables: If subclasses have many unique fields, the table can become sparse, leading to wasted space.
- Data integrity: Ensuring valid data across hierarchical fields can be challenging. Nullable fields can lead to confusion and maintenance issues.
- Complex queries: Queries can become convoluted, especially if many subclasses exist.
Implementing Single Table Inheritance in JPA
Basic Setup
To use Single Table Inheritance, you'll need to annotate your base class with @Inheritance
. Here’s a simple example:
import javax.persistence.*;
@Entity
@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
@Table(name = "vehicles")
public abstract class Vehicle {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String make;
private String model;
// Getters and Setters
}
Subclass Implementation
Next, you can create subclasses representing specific types of vehicles:
@Entity
@DiscriminatorValue("Car")
public class Car extends Vehicle {
private int numberOfDoors;
// Getters and Setters
}
@Entity
@DiscriminatorValue("Truck")
public class Truck extends Vehicle {
private double payloadCapacity;
// Getters and Setters
}
Comment on Design
Here’s why this design makes sense: We’re using an abstract class (Vehicle) as a base for our subclasses (Car and Truck). This structure allows for shared fields while letting subclasses introduce their own specific attributes.
Common Issues with Single Table Inheritance
Despite its benefits, developers often run into issues with Single Table Inheritance. Below are some common problems and how to tackle them.
1. Managing NULL values
When different subclasses have distinctive fields, it can lead to many NULL values, which complicates data integrity.
Solution: Use Value Objects
Consider using Value Objects for optional fields:
@Entity
@DiscriminatorValue("Car")
public class Car extends Vehicle {
private int numberOfDoors;
// Optional Value Object
@Embedded
private NavigationSystem navigationSystem;
// Getters and Setters
}
This removes the clutter from your primary entity while maintaining clarity.
2. Query Complexity
Queries can become convoluted if you're not careful, especially with many subclasses involved.
Solution: Define Named Queries
JPA allows you to define named queries, which can simplify the complexity of your query logic.
@NamedQueries({
@NamedQuery(name="findAllCars", query="SELECT c FROM Car c"),
@NamedQuery(name="findAllTrucks", query="SELECT t FROM Truck t")
})
Named queries provide better readability, making your intentions clearer.
3. Performance Impact
When you have a lot of subclasses, retrieving entities may become slower due to the sheer size of the table.
Solution: Use Projections
Instead of fetching complete entities, use JPA projections to retrieve only the data you need.
@Query("SELECT c.make, c.model FROM Car c")
List<CarProjection> findAllCarMakesAndModels();
In this code snippet, CarProjection
would be an interface that defines how to represent the necessary data structure.
Bulk Operations and Single Table Inheritance
One overlooked aspect of Single Table Inheritance is performing bulk operations.
Example: Bulk Update
A naive update operation can lead to performance penalties if not handled correctly. Instead of updating each entity individually, use the following method:
@Modifying
@Query("UPDATE Vehicle v SET v.make = ?1 WHERE v.type = ?2")
int updateVehicleMakeByType(String newMake, String type);
This allows significant boosts in speed, especially when dealing with large datasets.
The Closing Argument
In conclusion, while Single Table Inheritance in JPA can significantly simplify your object-relational mapping, it isn't without its challenges. By understanding the underlying mechanics and anticipating common issues, you can build a robust application that leverages the strengths of JPA effectively.
For further reading on JPA's inheritance strategies, check out the official JPA documentation and Spring Data JPA Guides. By mastering these concepts, you'll be better prepared for upcoming projects and more equipped to tackle any potential issues that arise.
In summary, whether you are facing sparse tables, complex queries, or performance impacts, applying the solutions discussed will help you refine your use of JPA and Single Table Inheritance. Happy coding!
Checkout our other articles