Debugging Common Issues When Creating External DSLs with ANTLR
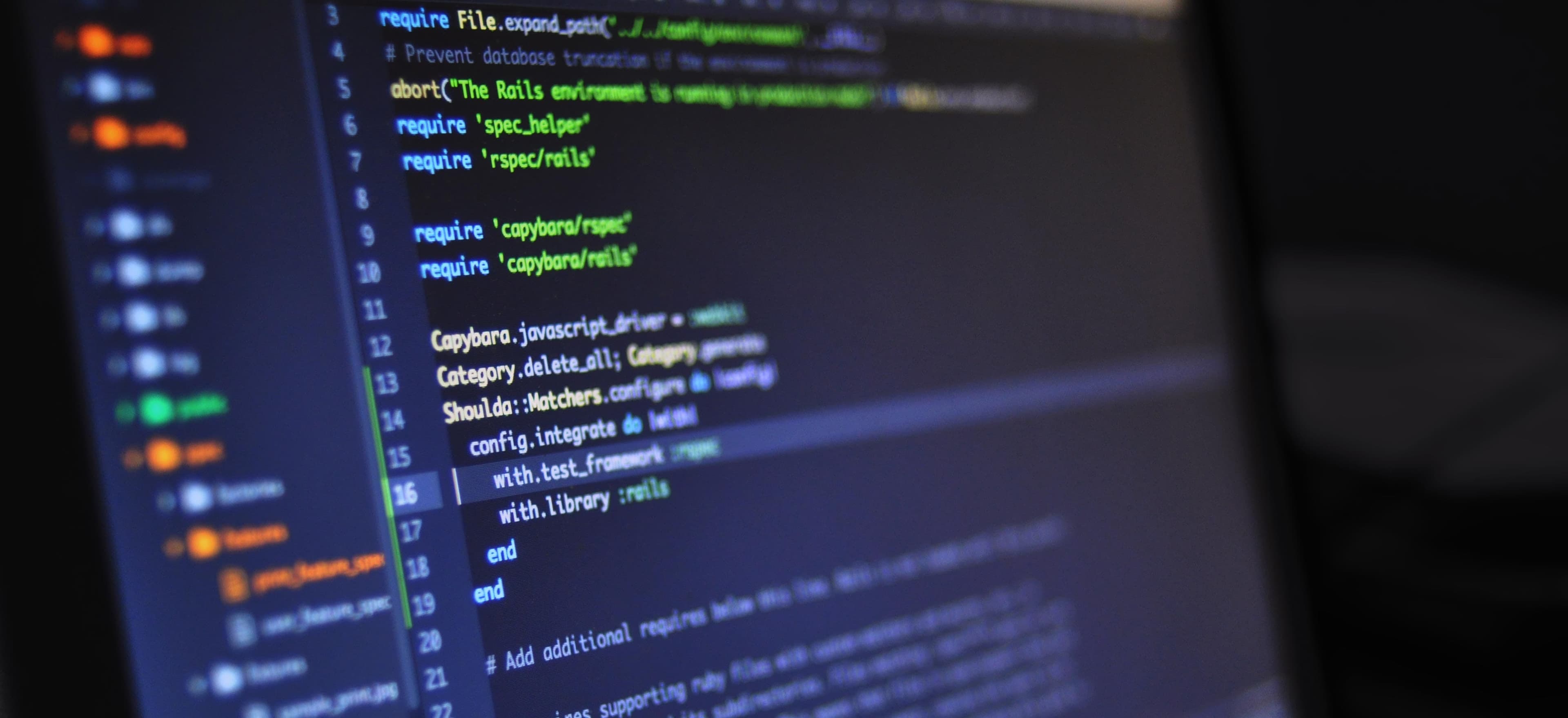
- Published on
Debugging Common Issues When Creating External DSLs with ANTLR
Creating an external Domain-Specific Language (DSL) can be a fulfilling yet sometimes overwhelming task. When using ANTLR (ANother Tool for Language Recognition), it is essential to be aware of potential issues that can arise during the design and implementation process. This blog post will take you through common pitfalls and debugging techniques when building external DSLs with ANTLR, offering Java code snippets and commentary to illuminate the reasoning behind various solutions.
Understanding ANTLR and External DSLs
Before diving into debugging methods, it's vital to understand what ANTLR is and the purpose of external DSLs. ANTLR is a powerful tool for generating parsers for processing structured text. It excels in parsing languages, making it an apt choice for creating DSLs.
An external DSL is a language that is tailored for specific applications but exists outside of the primary programming language. For instance, SQL is an external DSL specifically designed for database queries.
Common Issues Encountered When Creating DSLs
Creating a DSL involves several stages, including defining syntax, creating parser rules, and implementing actions. Here are some common issues:
1. Incorrect Grammar Rules
Issue: One of the most frequent issues lies in the grammar rules defined in your ANTLR files not matching the intended syntax of your DSL.
Solution: Validate your grammar rules. ANTLR provides error messages when there's a mismatch in your grammar. Below is a simple example to illustrate how grammar is defined:
grammar SimpleMath;
expr: term (('+' | '-') term)*;
term: factor (('*' | '/') factor)*;
factor: INT | '(' expr ')';
INT: [0-9]+;
WS: [ \t\n\r]+ -> skip;
Commentary: This example outlines a simple mathematical expression interpreter. The expr
, term
, and factor
are mutually recursive definitions reflecting mathematical precedence. If you experience parsing issues, ensure that each grammar component accurately represents the expected syntax.
2. Ambiguities in Grammar
Issue: Ambiguities can lead to unpredictable behavior during parsing. ANTLR requires a clear hierarchy within grammar rules.
Solution: Refine your grammar rules. Consider the following refined version of the initial DSL:
grammar PreciseMath;
expr: term (('+' | '-') term)*;
term: factor (('*' | '/') factor)*;
factor: INT | '(' expr ')';
INT: [0-9]+;
WS: [ \t\r\n]+ -> skip;
Commentary: Here, we've ensured that mathematical operations respect the correct order of operations: addition and subtraction operate on terms, while multiplication and division operate on factors. Keeping rules clear and well-structured mitigates ambiguity.
3. Error Handling Mechanisms
Issue: Users of your DSL will inevitably make mistakes, and not handling errors gracefully can lead to a poor user experience.
Solution: Implement robust error reporting. You can leverage ANTLR's built-in error handling facilities. Here's how to customize error messages:
@Override
public void recover(Parser recognizer, RecognitionException e) {
System.err.println("Error parsing the input: " + e.getMessage());
recognizer.consume(); // Move past the error
}
Commentary: This snippet defines custom behavior to recover from parsing errors, providing feedback to users. It captures important information about the error and ensures that the parser continues processing.
4. Dependency on External Libraries
Issue: Creating complex DSLs often involves dependencies on external libraries. Mismatched versions can lead to unforeseen errors.
Solution: Maintain consistent library versions. Use build tools like Maven or Gradle to manage dependencies. A sample Maven dependency configuration looks like this:
<dependencies>
<dependency>
<groupId>org.antlr</groupId>
<artifactId>antlr4-runtime</artifactId>
<version>4.9.2</version>
</dependency>
<!-- Include any other dependencies you need -->
</dependencies>
Commentary: This ensures that you're using a specific version of ANTLR, which is necessary to avoid compatibility issues. Version mismatches can lead to various debugging challenges that can hinder your development process.
5. Performance Bottlenecks
Issue: As your DSL grows in complexity, you may encounter performance issues, particularly when parsing large files.
Solution: Profile and optimize your grammar. Utilizing ANTLR's built-in listener and visitor patterns can help structure your DSL evaluation logic efficiently.
Here's an example of utilizing a visitor:
public class MathVisitor extends SimpleMathBaseVisitor<Integer> {
@Override
public Integer visitExpr(SimpleMathParser.ExprContext ctx) {
int result = visit(ctx.term(0));
for (int i = 1; i < ctx.term().size(); i++) {
if (ctx.getChild(i * 2 - 1).getText().equals("+")) {
result += visit(ctx.term(i));
} else {
result -= visit(ctx.term(i));
}
}
return result;
}
}
Commentary: This visitor pattern approach allows efficient traversal and evaluation of the expression tree generated by ANTLR. Each method corresponds to a grammar rule and recursively evaluates expressions, which can significantly improve performance and maintainability.
6. Lack of Documentation
Issue: Without proper documentation, both the developers and end users can become confused about the correct usage of your DSL.
Solution: Invest time in creating clear documentation. Consider tools that might help automate API documentation based on comments, such as JavaDoc. In addition, an example DSL usage guide promotes better understanding:
# SimpleMath DSL Usage
## Examples
```plaintext
3 + 5
10 * (2 - 4)
This will evaluate to...
**Commentary**: Comprehensive documentation aids not just users but also future developers who may work on your DSL.
## In Conclusion, Here is What Matters
Designing a DSL using ANTLR presents several challenges that can lead to debugging complexities. By addressing common issues such as grammar inaccuracies, performance bottlenecks, and error management, you can develop a robust and user-friendly DSL.
Always remember: writing good documentation is just as critical as writing good code. For further reading on ANTLR and debugging external DSLs, you can visit the [ANTLR documentation](https://www.antlr.org/) and explore the [ANTLR GitHub repository](https://github.com/antlr/antlr4). These resources provide valuable insights and examples that will enhance your DSL creation journey.
By embracing these strategies and techniques, you will be well on your way to mastering the intricacies of building effective and efficient external DSLs with ANTLR. Happy coding!
Checkout our other articles