Top 5 Common Mistakes Using Java Libraries
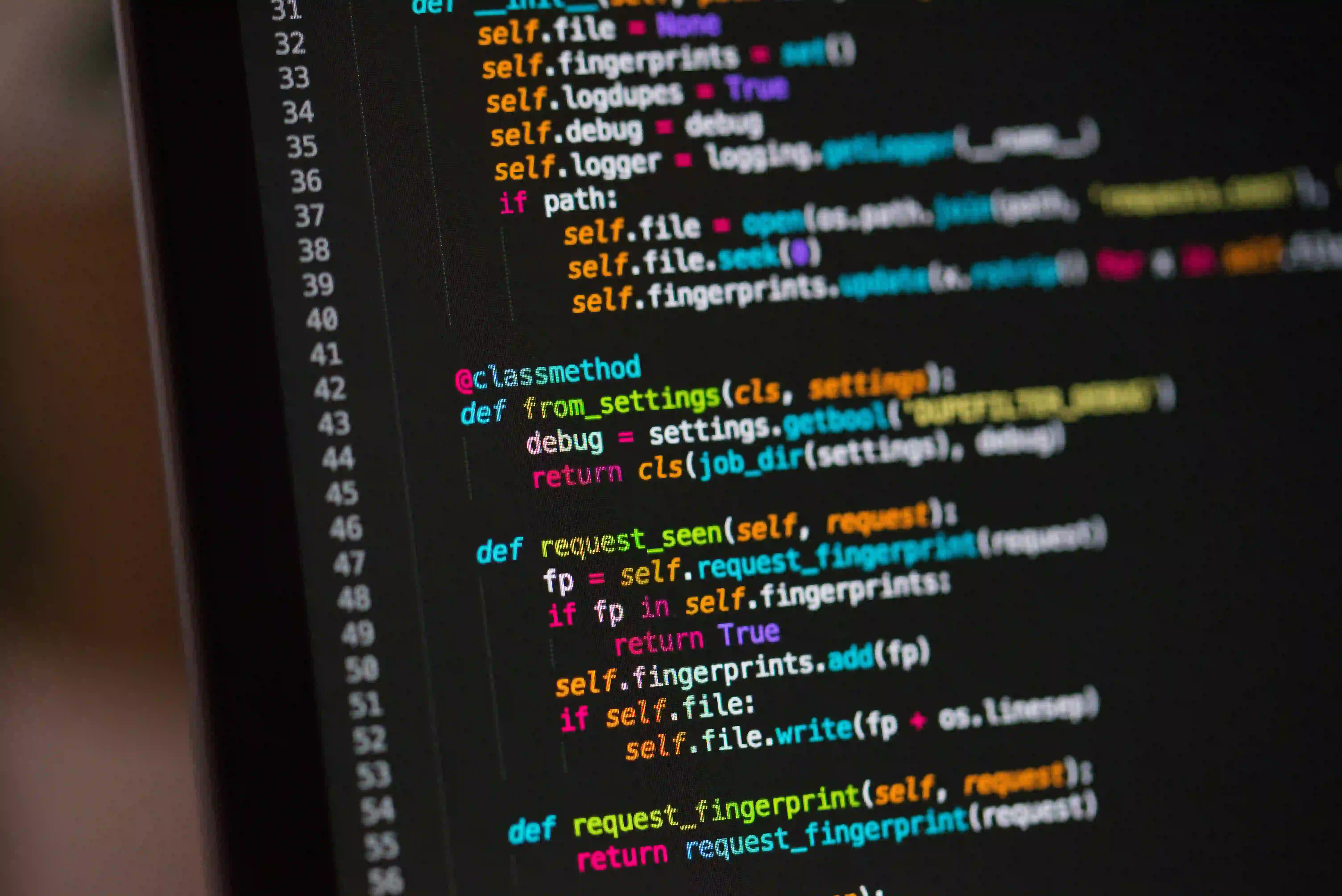
Top 5 Common Mistakes Using Java Libraries
Java is a versatile programming language that benefits from a rich ecosystem of libraries and frameworks. These libraries can significantly reduce development time, increase code quality, and enhance functionality. However, leveraging Java libraries can also lead to common mistakes that developers should be mindful of. In this blog post, we will explore the top five mistakes when using Java libraries, along with tips to avoid them.
1. Ignoring Documentation
Why Documentation Matters
Documentation serves as a guide that explains how a library works, its APIs, and provides examples of use cases. Ignoring it can lead to misusing the library or duplicating efforts unnecessarily.
Example of What Happens When Documentation is Ignored
When using a library like Apache Commons Lang, developers might overlook the utility functions provided for String manipulation. Instead of using StringUtils
, they could end up writing complex code for functionality that is already available.
import org.apache.commons.lang3.StringUtils;
public class StringExample {
public static void main(String[] args) {
String input = " Hello, World! ";
// Correctly using StringUtils to trim and check for empty
String trimmed = StringUtils.trim(input);
if (StringUtils.isNotEmpty(trimmed)) {
System.out.println(trimmed);
}
}
}
Commentary: This code demonstrates how to leverage the functionality of a library for string manipulation. By using StringUtils
, developers save time and reduce potential bugs in their own implementations.
Tip: Always read the documentation for libraries you integrate. It will clarify functionalities and help you implement features more efficiently.
For more information on the importance of documentation, check out this link: Importance of Documentation.
2. Overusing or Underusing Libraries
Balancing Your Library Usage
While Java libraries can speed up development, overusing them by implementing numerous libraries for small tasks can bloat your project, making it hard to maintain. Conversely, underusing libraries might lead to unnecessary reinvention of the wheel.
Example of Overusing Libraries
Suppose a developer requires basic HTTP operations. Instead of using a simple HttpURLConnection
, they import a heavy library like Apache HttpClient for a single request.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class SimpleHttpGet {
public static void main(String[] args) throws Exception {
String url = "https://api.example.com/data";
HttpURLConnection conn = (HttpURLConnection) new URL(url).openConnection();
conn.setRequestMethod("GET");
try (BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()))) {
String inputLine;
StringBuilder content = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
content.append(inputLine);
}
System.out.println(content.toString());
}
}
}
Commentary: This code demonstrates how to perform an HTTP GET request without needing an additional library, thereby keeping the project more lightweight.
Tip: Assess the requirements of your project carefully. Use libraries when they genuinely add value, but don’t hesitate to implement simple tasks directly when feasible.
3. Not Considering Dependency Management
The Importance of Dependency Management
With libraries comes dependencies, which can lead to conflicts. Failing to manage these can create runtime issues or even application crashes.
Example of Dependency Conflict
Using different versions of the same library in your project can lead to runtime failures. Tools like Maven and Gradle help manage dependencies effectively.
<!-- Example of a Maven dependency declaration -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version> <!-- Ensure this is consistent across your project -->
</dependency>
Commentary: Declaring dependencies in Maven ensures you're using specific versions and helps avoid conflicts with transitive dependencies.
Tip: Use tools like Maven or Gradle to manage your project's libraries and keep track of versioning.
Learn more about dependency management best practices in this article: Maven Dependency Management.
4. Not Handling Exceptions Properly
Why Exception Handling is Crucial
Java libraries are often designed to throw exceptions for error conditions. Failing to handle these exceptions can ultimately lead to ungraceful application failures.
Example of Proper Exception Handling
When using a library for file I/O, it’s critical to handle IOException
effectively. Here’s an example:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class ReadFileExample {
public static void main(String[] args) {
Path filePath = Paths.get("example.txt");
try {
String content = Files.readString(filePath);
System.out.println("File Content: " + content);
} catch (IOException e) {
System.out.println("Error reading the file: " + e.getMessage());
}
}
}
Commentary: This example shows how to read a file while properly handling exceptions. Without handling IOException
, the program might crash if the file is missing.
Tip: Always use try-catch blocks to manage exceptions thrown by library methods, enabling robust error handling.
5. Overlooking Performance Implications
Balancing Functionality and Performance
Some Java libraries come with performance trade-offs. Failing to consider the performance impact before integrating a new library can negatively affect your application's responsiveness.
Example of Performance Considerations
Using streams for data processing can simplify code but may lead to suboptimal performance if not handled correctly.
import java.util.Arrays;
import java.util.List;
public class StreamPerformance {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using streams
numbers.stream()
.filter(n -> n % 2 == 0)
.forEach(System.out::println);
}
}
Commentary: While streams improve readability, they may not be the fastest option for large data sets. Always benchmark and test performance based on your project's requirements.
Tip: Profile your application regularly, especially after adding new libraries, and evaluate whether they meet your performance criteria.
Bringing It All Together
Java libraries are powerful tools that can accelerate development, but savvy developers must navigate pitfalls associated with their use. By avoiding these common mistakes, you'll enhance your project’s longevity and maintainability while achieving optimal performance.
To summarize:
- Always check documentation to maximize library usage.
- Balance your library usage to avoid bloating your project.
- Manage dependencies meticulously to prevent conflicts.
- Handle exceptions properly for a more graceful experience.
- Monitor performance and benchmark new libraries’ impact.
With these tips in mind, you can leverage Java libraries effectively, ensuring your projects are optimized and maintainable over time. Happy coding!