Common Pitfalls in JDK 12 Compact Number Formatting
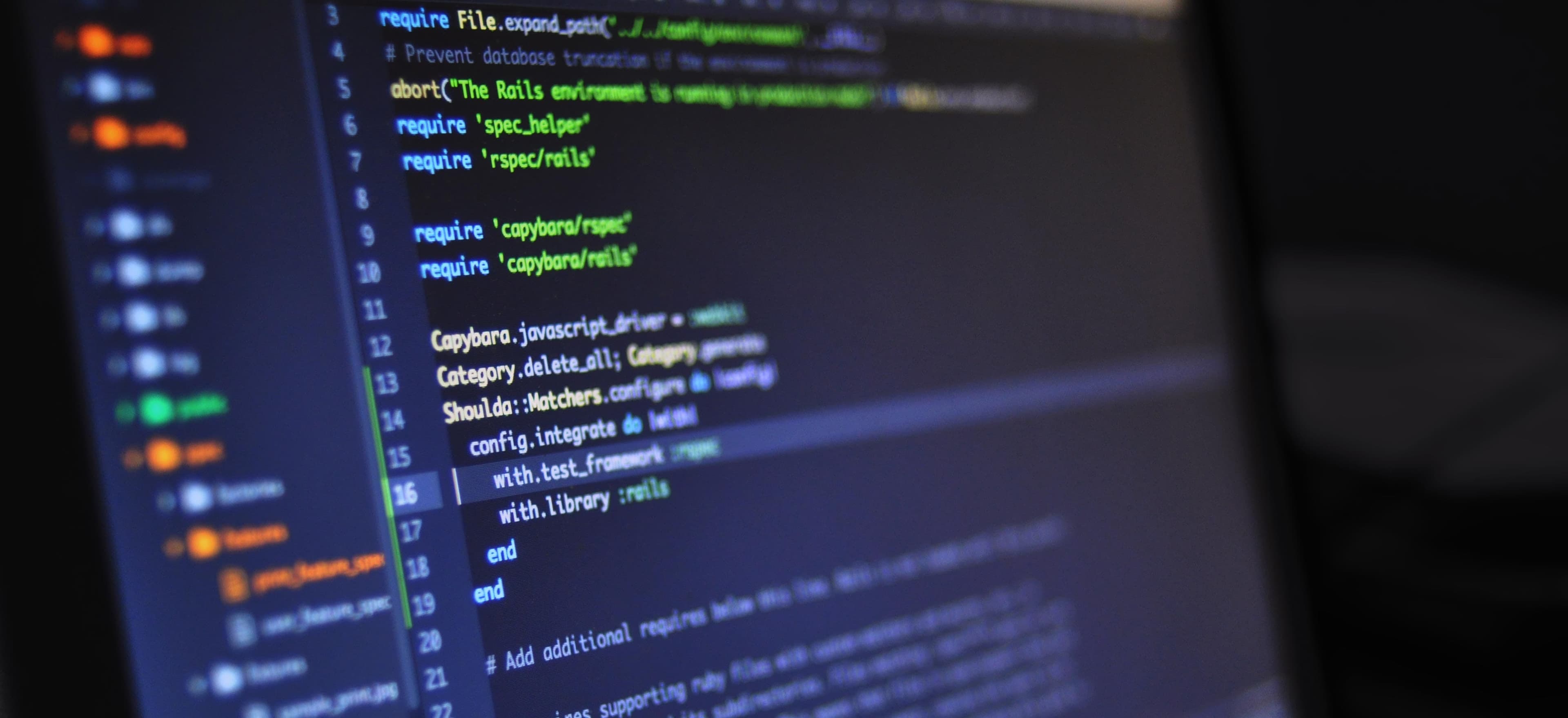
- Published on
Common Pitfalls in JDK 12 Compact Number Formatting
With the introduction of JDK 12, Java developers received a powerful tool for number formatting in the form of the CompactNumberFormat. This feature allows programmers to format large numbers into a more human-readable and compact form, improving the UI and UX. However, while using this feature can significantly enhance your application, it is critical to navigate its common pitfalls. In this blog post, we will discuss these pitfalls in detail, complemented by code snippets to demonstrate the correct usage.
What is Compact Number Formatting?
Before we dive into the pitfalls, let’s quickly review what CompactNumberFormat is. The CompactNumberFormat
class provides the functionality to format numbers into a more compact form. For instance:
- 1000 becomes "1K"
- 1,000,000 turns into "1M"
This is particularly useful in various applications where space is limited, such as mobile apps or dashboards.
Common Pitfalls in Compact Number Formatting
1. Incorrect Locale Usage
Using the default locale can lead to inconsistent formatting, especially for applications that target international users. CompactNumberFormat is locale-sensitive, and different regions may represent numbers differently.
Example Problematic Code:
import java.text.CompactNumberFormat;
// This will use the default locale, which may not match user expectations
CompactNumberFormat cNf = new CompactNumberFormat();
System.out.println(cNf.format(1000));
Solution: Use Specific Locales
Always specify the locale explicitly. For example:
import java.text.CompactNumberFormat;
import java.util.Locale;
CompactNumberFormat cNf = CompactNumberFormat.getCompactNumberInstance(Locale.US);
System.out.println(cNf.format(1000)); // Outputs "1K"
cNf = CompactNumberFormat.getCompactNumberInstance(Locale.GERMANY);
System.out.println(cNf.format(1000)); // Outputs "1 Tsd."
By using Locale.US
, you ensure the output is consistent with your users' expectations.
2. Formatting Issues with Large Numbers
Another common pitfall is failing to account for extremely large numbers. Depending on the implementation, numbers greater than certain thresholds may not format as anticipated.
Example Problematic Code:
import java.text.CompactNumberFormat;
CompactNumberFormat cNf = CompactNumberFormat.getCompactNumberInstance(Locale.US);
System.out.println(cNf.format(1_000_000_000)); // Outputs "1 B"
System.out.println(cNf.format(1_000_000_000_000)); // May output unexpectedly
Solution: Check Maximum Values
It’s essential to be aware of the behavior of your compact formatting tool with larger numbers. To handle this, you can set a limit on the number of digits to make output predictable.
// Custom method to handle large numbers safely
public String formatLargeNumbers(double number) {
if (number >= 1_000_000_000_000L) {
return String.format("%.1f T", number / 1_000_000_000_000.0); // Trillions
} else {
CompactNumberFormat cNf = CompactNumberFormat.getCompactNumberInstance(Locale.US);
return cNf.format(number);
}
}
This method ensures consistent formatting for all ranges of numbers.
3. Not Considering Negative Numbers
CompactNumberFormat does not always handle negative numbers gracefully. For many users, improper formatting of negative numbers can lead to confusion and misinterpretation.
Example Problematic Code:
CompactNumberFormat cNf = CompactNumberFormat.getCompactNumberInstance(Locale.US);
System.out.println(cNf.format(-2000)); // Outputs "-2K"
Solution: Human-Readable Outputs
Customize the formatting for negative numbers where necessary to improve readability and user experience.
public String formatWithNegativeSupport(double number) {
if (number < 0) {
return "-" + formatLargeNumbers(-number);
} else {
return formatLargeNumbers(number);
}
}
// Testing with negative value
System.out.println(formatWithNegativeSupport(-2000)); // Outputs "-2K"
4. Lack of Unit or Context Clarification
When presenting compacted numbers, it’s crucial to ensure your users understand the units being used. Without proper context, users might misinterpret the data being presented.
Example of Lack of Context:
System.out.println(cNf.format(100000)); // Outputs "100K" without context
Solution: Provide Contextual Information
To avoid confusion, always provide accompanying information, perhaps in the form of labels or tooltips.
double value = 100_000;
String formattedValue = cNf.format(value);
System.out.println("Total users: " + formattedValue); // Outputs "Total users: 100K"
5. Not Testing Across Different Devices
Testing your implementation only on desktop might lead you to overlook how numbers display on various mobile devices or screen sizes. Compact numbers can behave differently based on device resolution and available space.
Solution: Responsive Design Testing
Ensure that your UI is adaptive. You can achieve this by utilizing frameworks or libraries that allow dynamic redesign based on available space:
/* Example CSS for responsive design */
@media (max-width: 600px) {
.number-display {
font-size: 12px;
}
}
@media (min-width: 601px) {
.number-display {
font-size: 16px;
}
}
The Bottom Line
CompactNumberFormat in JDK 12 is an amazing tool that can enhance the user experience significantly when properly utilized. However, it is vital to navigate the common pitfalls effectively. Ensure to account for locale variations, large numbers, negative numbers, contextual clarity, and responsive design.
For further exploration of Java's number formatting capabilities, consider checking the official Java documentation for more details and examples.
By keeping these considerations in mind, developers can create a compelling experience for their users while presenting numerical data in a meaningful, compact, and understandable format. Happy coding!
Checkout our other articles