Overcoming Data Overload: Simplifying Decision-Making
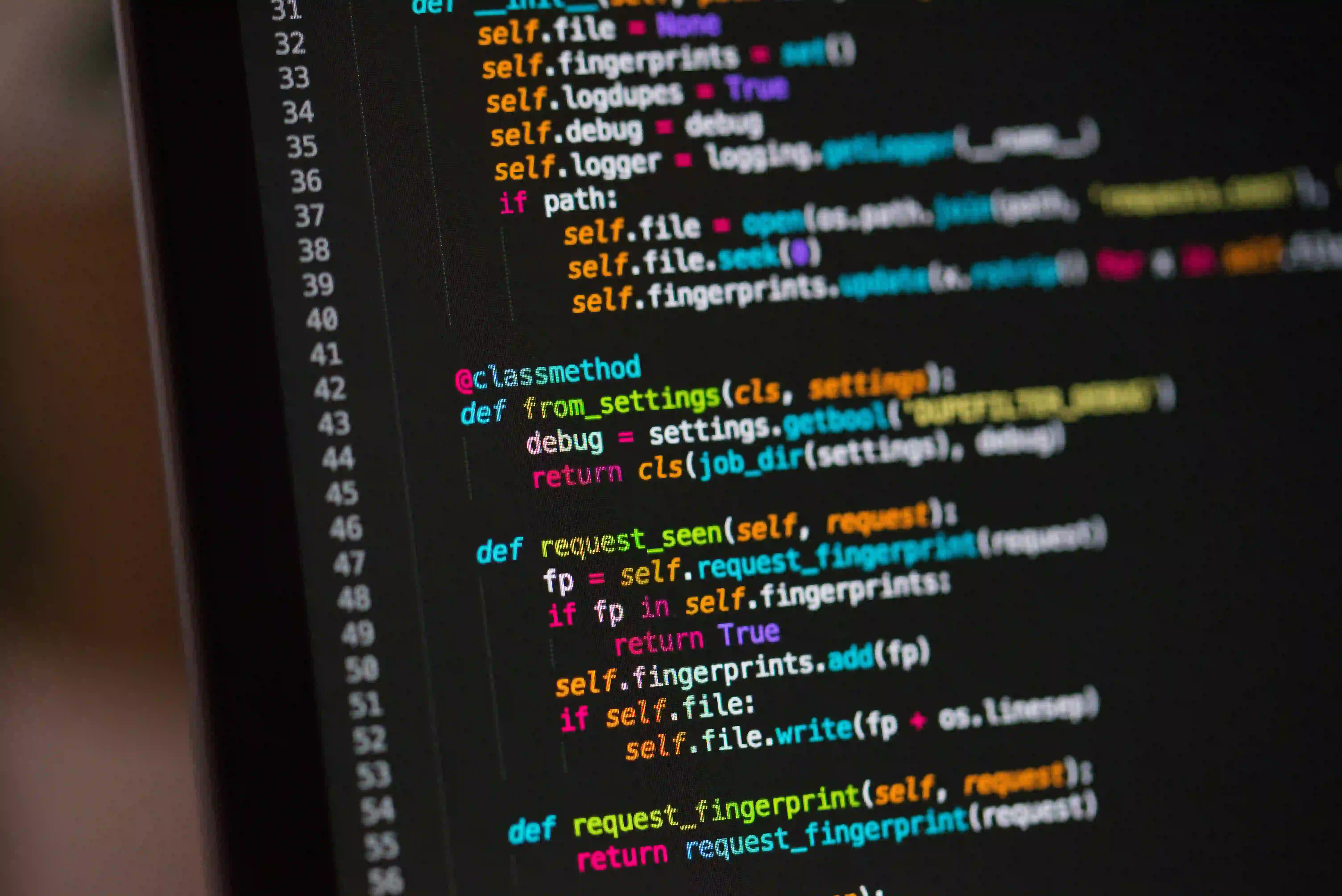
Overcoming Data Overload: Simplifying Decision-Making in Java
In a world where data is abundant, organizations often find themselves grappling with the challenge of data overload. With an overwhelming amount of information at our disposal, making informed decisions can feel like searching for a needle in a haystack. For Java developers, effectively managing data is key to ensuring that their applications are not only efficient but also user-friendly.
This post explores strategies to simplify decision-making in Java applications while overcoming data overload. We'll look at practical coding examples and provide context to ensure your Java applications can manage data effectively.
Understanding Data Overload
Data overload occurs when the volume of data exceeds the processing capacity of human decision-makers, leading to confusion and analysis paralysis. In consumer applications, users are bombarded with choices and information, which can result in dropped engagement, frustration, or even abandonment.
Key Contributors to Data Overload
- Excessive Options: Too many choices can overwhelm users.
- Repetitive Information: Redundant data can clutter the interface and confuse users.
- Poorly Structured Data: Unorganized data presents challenges in understanding and interpretation.
The Importance of Simplified Decision-Making
Simplifying decision-making helps users focus on what truly matters. When applications prioritize relevant data, they enhance user experience and promote effective decision-making.
Strategies to Overcome Data Overload
- Data Filtering: Present only the most relevant data.
- User Customization: Allow users to personalize their experience.
- Effective Visualization: Use graphs and charts for intuitive decision-making.
- Progressive Disclosure: Show information incrementally rather than all at once.
- Automated Insights: Use algorithms to provide insights rather than raw data.
Implementing Data Filtering in Java
Let's start with data filtering. When users are confronted with vast datasets, showing them only the information they need can significantly enhance their experience.
Example of Data Filtering
Here's a simple Java program that filters a list of products based on user-defined criteria:
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
class Product {
private String name;
private double price;
public Product(String name, double price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
@Override
public String toString() {
return String.format("Product{name='%s', price=%.2f}", name, price);
}
}
public class ProductFilter {
public static List<Product> filterProducts(List<Product> products, double maxPrice) {
return products.stream()
.filter(product -> product.getPrice() <= maxPrice)
.collect(Collectors.toList());
}
public static void main(String[] args) {
List<Product> products = new ArrayList<>();
products.add(new Product("Smartphone", 699.99));
products.add(new Product("Laptop", 999.99));
products.add(new Product("Tablet", 399.99));
double userMaxPrice = 500.00; // Example user-defined max price
List<Product> filteredProducts = filterProducts(products, userMaxPrice);
System.out.println("Filtered Products: " + filteredProducts);
}
}
Commentary on Code
In the code above, we define a simple Product
class with a name and price. The filterProducts
method uses Java Streams to filter products based on a user-defined maximum price.
- Why Use Streams?: The Stream API makes the code more readable and reduces boilerplate code. It allows for functional-style operations and enhances performance through lazy evaluation.
- User-Centric: This user-driven filter simplifies decision-making by allowing users to see only the products they can afford, addressing the "excessive options" challenge effectively.
User Customization: Personalizing the Experience
Allowing users to tailor their data presentation can significantly reduce feelings of being overwhelmed. Implementing a simple customization feature can enhance usability.
Example of User Customization
Here's a basic implementation that lets users set their preferences for viewing products based on categories:
import java.util.HashMap;
import java.util.Map;
class Preference {
private String category;
public Preference(String category) {
this.category = category;
}
public String getCategory() {
return category;
}
}
public class UserPreference {
private Map<String, Preference> userPreferences = new HashMap<>();
public void setPreference(String user, String category) {
userPreferences.put(user, new Preference(category));
}
public void displayProducts(List<Product> products, String user) {
Preference preference = userPreferences.get(user);
if (preference != null) {
products.stream()
.filter(product -> product.getName().toLowerCase().contains(preference.getCategory().toLowerCase()))
.forEach(System.out::println);
} else {
// Default display all products
products.forEach(System.out::println);
}
}
public static void main(String[] args) {
UserPreference userPref = new UserPreference();
userPref.setPreference("Alice", "smartphone");
List<Product> products = new ArrayList<>();
products.add(new Product("Smartphone", 699.99));
products.add(new Product("Laptop", 999.99));
products.add(new Product("Tablet", 399.99));
System.out.println("Alice's Product Preferences:");
userPref.displayProducts(products, "Alice");
}
}
Commentary on Code
- UserPreference Class: The
UserPreference
class stores the user preferences in a simple map. It allows customization of displayed products according to user-defined criteria. - Importance of Personalization: This code snippet demonstrates how to enhance user engagement by giving control over the information they consume.
For further reading on user customization and the impact it can have, check out Nielsen Norman Group’s article on user experience.
Effective Visualization Techniques
Data visualization greatly aids in making sense of large datasets. Graphs, charts, and dashboards help distill complex information into comprehensible formats. For Java developers, libraries like JFreeChart or JavaFX provide excellent options for implementing visualization in applications.
Progressive Disclosure Implementation
Progressive disclosure involves showing only critical information upfront while hiding complex options under expandable sections or links. This strategy minimizes initial information load but allows users to access more detailed data if desired.
Example of Progressive Disclosure
Here’s a simple conceptual representation in Java:
public class ProductDetails {
public static void displayProductSummary(Product product) {
System.out.println("Product: " + product.getName());
System.out.println("Price: $" + product.getPrice());
System.out.println("Click for more details...");
}
public static void displayProductDetails(Product product) {
// Imagine these details are fetched from a database or an external source
System.out.println("Advanced details for " + product.getName());
System.out.println("Specifications: High resolution display...");
}
public static void main(String[] args) {
Product smartphone = new Product("Smartphone", 699.99);
displayProductSummary(smartphone);
// Simulate Click
displayProductDetails(smartphone);
}
}
Commentary on Code
This implementation uses a simple summary function that displays essential product info and a detailed view function that provides additional details. It demonstrates the progressive disclosure model, where users can engage further by clicking or interacting.
Leveraging Automated Insights
Automated insights through algorithms can streamline the decision-making process. Instead of presenting users with tables of data, providing actionable insights helps them make quicker decisions.
Final Thoughts
Overcoming data overload is crucial for improving decision-making in Java applications. Through strategies such as data filtering, user customization, effective visualization, and progressive disclosure, developers can create a more manageable and enjoyable user experience.
By implementing these practices, you will not only enhance user satisfaction but also drive engagement and improve overall application performance.
For a deeper understanding of data overload and how to mitigate its effects, refer to these resources:
- Harvard Business Review on the Truth about Data Overload
- Gartner Research on Data Overload
By applying these insights and strategies, you can develop Java applications that streamline user experiences and simplify decision-making processes.