Streamline Month Name Conversion in Java: A Simple Guide
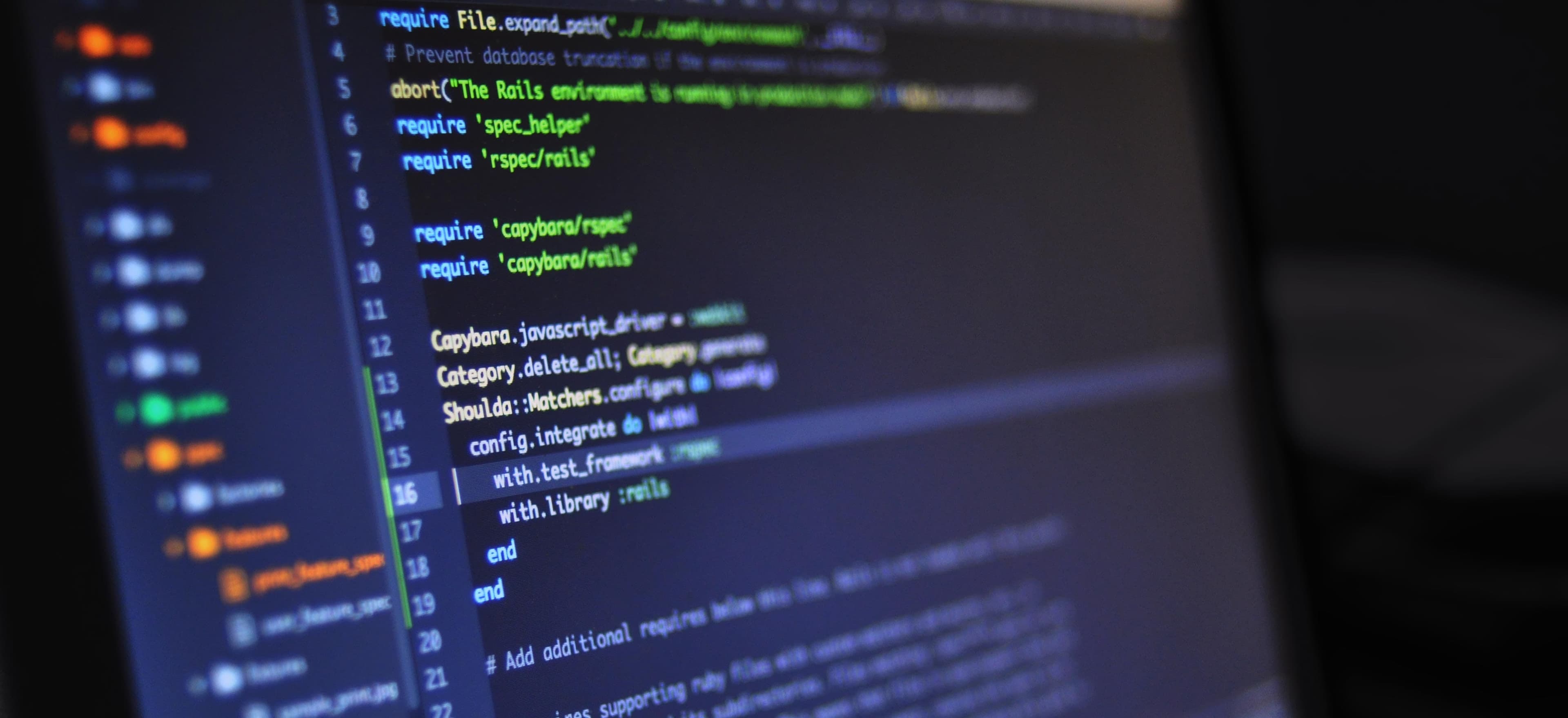
- Published on
Streamline Month Name Conversion in Java: A Simple Guide
In Java programming, handling dates and times efficiently is paramount, especially when we need to convert month numbers to their corresponding names. Whether you are developing a simple application that displays the current month or building a robust enterprise application, having a straightforward way to display month names can greatly enhance user experience.
In this guide, we will explore how you can easily convert month numbers into their respective names in Java. We will employ various methods, including using arrays, the Java DateTimeFormatter
, and even Java 8's LocalDate
class. Let's dive right in!
Why Convert Month Numbers to Names?
Converting month numbers (like 1 for January, 2 for February, etc.) into their full names allows for better user communication and enhances readability in reports, logs, and UI displays. Here are some scenarios where this conversion might be necessary:
- Generating month-wise reports.
- Displaying user-friendly messages.
- Formatting dates for logs or user interfaces.
Method 1: Using an Array
One of the simplest ways to map month numbers to their names in Java is through an array.
public class MonthConverter {
// Array holding month names
private static final String[] MONTHS = {
"", "January", "February", "March",
"April", "May", "June",
"July", "August", "September",
"October", "November", "December"
};
// Method to get month name
public static String getMonthName(int month) {
if (month < 1 || month > 12) {
throw new IllegalArgumentException("Month must be between 1 and 12");
}
return MONTHS[month];
}
public static void main(String[] args) {
System.out.println(getMonthName(5)); // Output: May
}
}
Why Use an Array?
Using an array is an efficient way to directly map month numbers to their names. This approach is straightforward, and once the array is initialized, lookups can happen in constant time O(1). The method also checks for valid input, ensuring that users cannot pass invalid month values.
Method 2: Using java.time.Month
Java 8 introduced a new date and time API that can simplify many date-related tasks. The Month
enum is a powerful utility that makes converting month numbers to names seamless.
import java.time.Month;
public class MonthConverter {
public static String getMonthName(int month) {
if (month < 1 || month > 12) {
throw new IllegalArgumentException("Month must be between 1 and 12");
}
return Month.of(month).name(); // Returns the name in uppercase
}
public static void main(String[] args) {
System.out.println(getMonthName(8)); // Output: AUGUST
}
}
Why Use Month
Enum?
The Month
enum provides an elegant solution to work with months. The of()
method allows for passing in a month number and retrieves the associated month. One thing to note is that the name returned is in uppercase, but it's a small trade-off for using a more modern, robust API.
Method 3: Using a Formatter with LocalDate
Another effective way to convert month numbers to names is by using the LocalDate
class along with DateTimeFormatter
. This method is particularly useful when you are working with full dates, as it allows for versatile formatting.
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class MonthConverter {
public static String getMonthName(int month) {
if (month < 1 || month > 12) {
throw new IllegalArgumentException("Month must be between 1 and 12");
}
LocalDate date = LocalDate.of(2023, month, 1); // Year is arbitrary
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("MMMM");
return date.format(formatter); // Output: full month name
}
public static void main(String[] args) {
System.out.println(getMonthName(11)); // Output: November
}
}
Why Use LocalDate
and DateTimeFormatter
?
By utilizing LocalDate
, you allow for greater flexibility in dealing with complete dates. This method is particularly useful if you need to format entire date strings neatly. The DateTimeFormatter
class enables developers to specify how they want the date formatted, offering an extensive range of formatting options.
Comparing the Methods
| Method | Pros | Cons | |-----------------------|--------------------------------------------|--------------------------------------| | Array | Fast and simple | Hard-coded values, less dynamic. | | Month Enum | Uses Java 8 API, type-safe, consistent | Returns names in uppercase by default.| | LocalDate & Formatter | Highly flexible; supports complete dates | Slightly more complex implementation.|
Bringing It All Together
Effective month name conversion in Java can be implemented in various ways depending on your project's needs. The array method is the simplest, while the Month
enum offers type safety and better integration with Java's modern date-time API. The approach using LocalDate
and DateTimeFormatter
is ideal for applications that require complete date manipulation and formatting.
Depending on your application's context, you can choose the method that best aligns with your needs. For further resources on Java date and time handling, consider reading Java Date and Time Tutorial.
Key Takeaway
Always validate month inputs. Handling erroneous input gracefully can save a lot of headaches later on. With these methods at your disposal, you can streamline your approach to month name conversions and improve your Java application's usability.
Feel free to explore each method in your projects and see how they can enhance your date handling capabilities in Java. Happy coding!
Checkout our other articles