Overcoming MongoDB Performance Issues in Scaling Applications
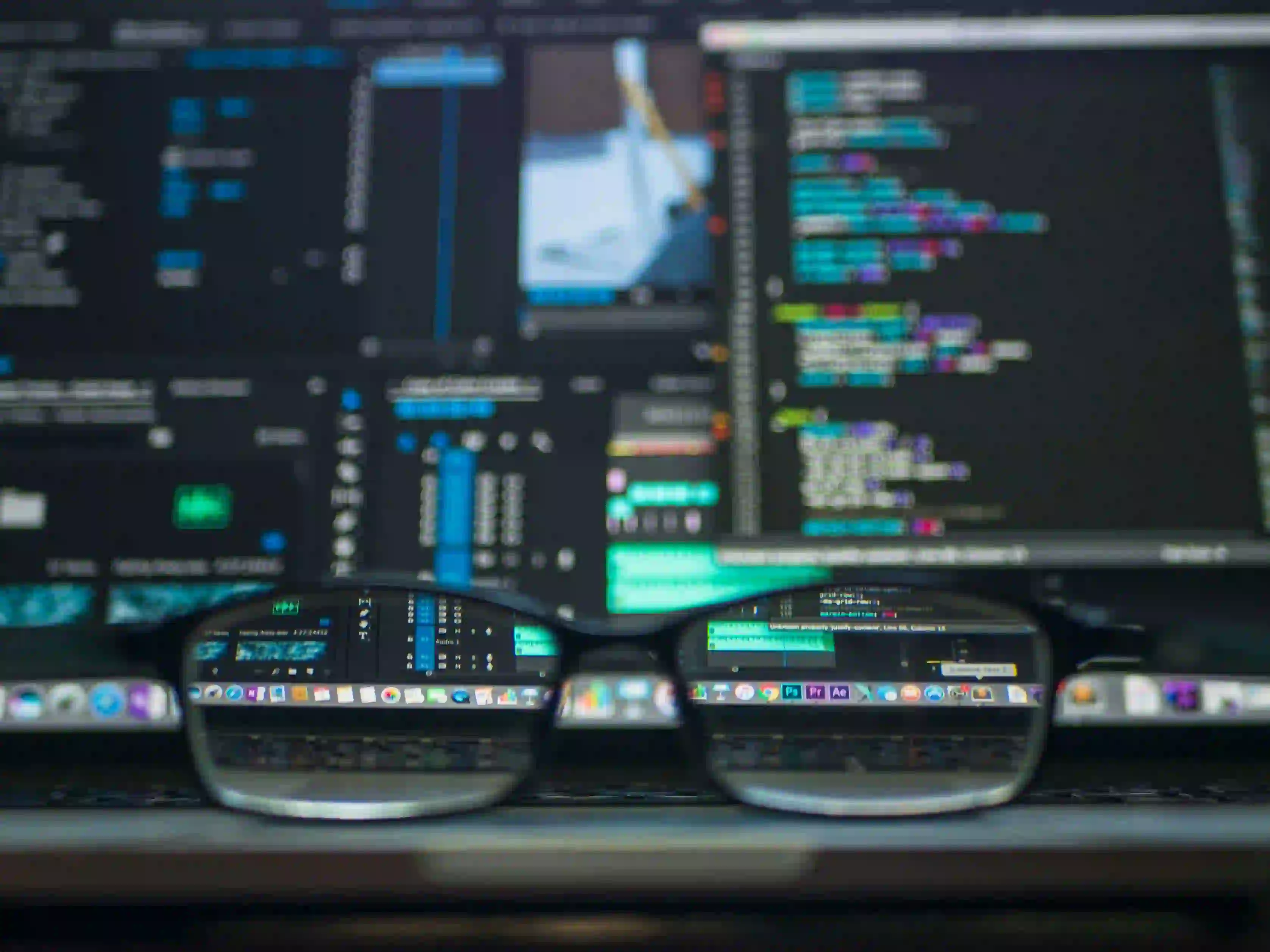
Overcoming MongoDB Performance Issues in Scaling Applications
MongoDB, as a NoSQL document database, has gained immense popularity for its flexibility, scalability, and ease of use, particularly in modern web applications. However, with this powerful tool comes performance challenges that can hinder scaling applications effectively. In this blog post, we'll explore common performance issues in MongoDB and provide actionable strategies to overcome them.
Understanding MongoDB Performance Bottlenecks
Before diving into solutions, it's essential to identify the common performance issues that developers encounter:
- Slow Queries: Queries that take longer than expected can significantly slow down your application.
- Indexing Issues: Lack of proper indexes can lead to full scans and degraded performance.
- Data Model Inefficiencies: Poorly structured data can lead to complicated queries and increased load times.
- Hardware Limitations: Scaling up resources can help, but it’s not always the most efficient solution.
- Concurrency: High write-read contention can lead to slow database operations.
Key Concepts
Understanding these performance issues can significantly enhance your application’s performance. Let’s explore strategies to tackle these challenges.
1. Optimize Your Queries
Slow queries can bog down your application, making it critical to focus on optimizing them. MongoDB provides tools like the Query Profiler and explain()
method to analyze query performance.
Example: Analyzing a Query
// Use explain() to analyze a query
db.collection.find({ field: "value" }).explain("executionStats")
This command gives you insights into how MongoDB processes the query, including execution time and index usage. By studying the output, you can understand whether you're using indexes effectively or if full collection scans are occurring.
Query Optimization Strategies
- Use Indexes: Ensure indexes are in place for frequently queried fields.
- Limit Returned Documents: Always use projections to return only the necessary fields.
- Filter Early: Apply conditions to limit the size of documents returned.
2. Implement Efficient Indexing
Indexing is crucial for performance in MongoDB. Without proper indexes, data retrieval may become slow, especially in large datasets.
Example: Creating an Index
// Create an index on the "username" field
db.users.createIndex({ username: 1 })
This command creates an ascending index on the username
field of the users
collection. Properly indexed collections can accelerate query execution.
Indexing Best Practices
- Use Compound Indexes: Combine multiple fields into a single index when applicable.
- Avoid Over-Indexing: Each index incurs overhead; only index fields that will be queried.
- Monitor Index Performance: Use tools like MongoDB Compass to monitor index efficiency.
3. Optimize Your Data Model
MongoDB allows for flexible data modeling, but this flexibility can lead to inefficiencies if not managed correctly.
Example: Data Structuring
Instead of normalizing your data as you would in a relational database, consider denormalization where it makes sense. For example:
{
postId: "123",
title: "Scaling with MongoDB",
author: {
username: "devguru",
email: "devguru@example.com"
},
comments: [
{
userId: "456",
content: "Great post!",
timestamp: "2023-01-01"
}
]
}
This schema allows you to store related information together, reducing the number of queries needed to retrieve related data.
Data Modeling Strategies
- Use Embedded Documents: For data that is closely related and often retrieved together, embed documents instead of referencing.
- Limit Document Size: Be wary of exceeding the maximum BSON document size of 16MB.
- Shard Your Data: Use sharding for very large datasets to distribute load effectively.
4. Improve Hardware Configuration
While optimizing queries and data models is essential, sometimes hardware limitations can be a bottleneck.
Storage Considerations
- Use SSDs over HDDs: Solid State Drives provide significantly better I/O performance.
- Increase RAM: More memory allows for a larger dataset to fit in memory, which improves performance.
Example: Configuring a Replica Set
Configuring a replica set not only helps with data redundancy but also improves read performance through read scaling.
// Initialize a replica set
rs.initiate({
_id: "rs0",
members: [
{ _id: 0, host: "mongo1:27017" },
{ _id: 1, host: "mongo2:27017" },
{ _id: 2, host: "mongo3:27017" }
]
})
This command initializes a basic replica set, allowing for high availability and read scaling by adding secondary nodes.
5. Manage Concurrency Effectively
With high write-read contention, operations can slow down significantly. To tackle this issue:
Example: Using Write Concern
Utilizing write concern can ensure the operations succeed before acknowledging a write, but choose wisely based on your requirements.
// Set write concern to ensure data is written to two nodes
db.collection.insertOne(
{ field: "value" },
{ writeConcern: { w: 2 } }
)
This configuration ensures that the write operation is acknowledged by at least two members of the replica set.
Concurrency Strategies
- Limit Locking: Implement sharding to minimize lock contention.
- Optimize Bulk Operations: Use bulk writes for multiple operations to reduce the number of round trips to the database.
- Monitor Performance: Utilize the
db.currentOp()
command to track currently running operations and identify potential blockages.
Key Takeaways
Scaling applications with MongoDB requires careful consideration of various performance aspects. By optimizing queries, implementing efficient indexing, refining your data model, enhancing hardware configurations, and effectively managing concurrency, you can greatly improve the overall performance of your application.
For further reading, you might find the following resources useful:
- MongoDB Performance Best Practices
- Understanding MongoDB Indexes
- MongoDB Data Modeling
By employing these strategies, you’ll be well on your way to overcoming the performance challenges associated with scaling your applications in MongoDB. Happy coding!