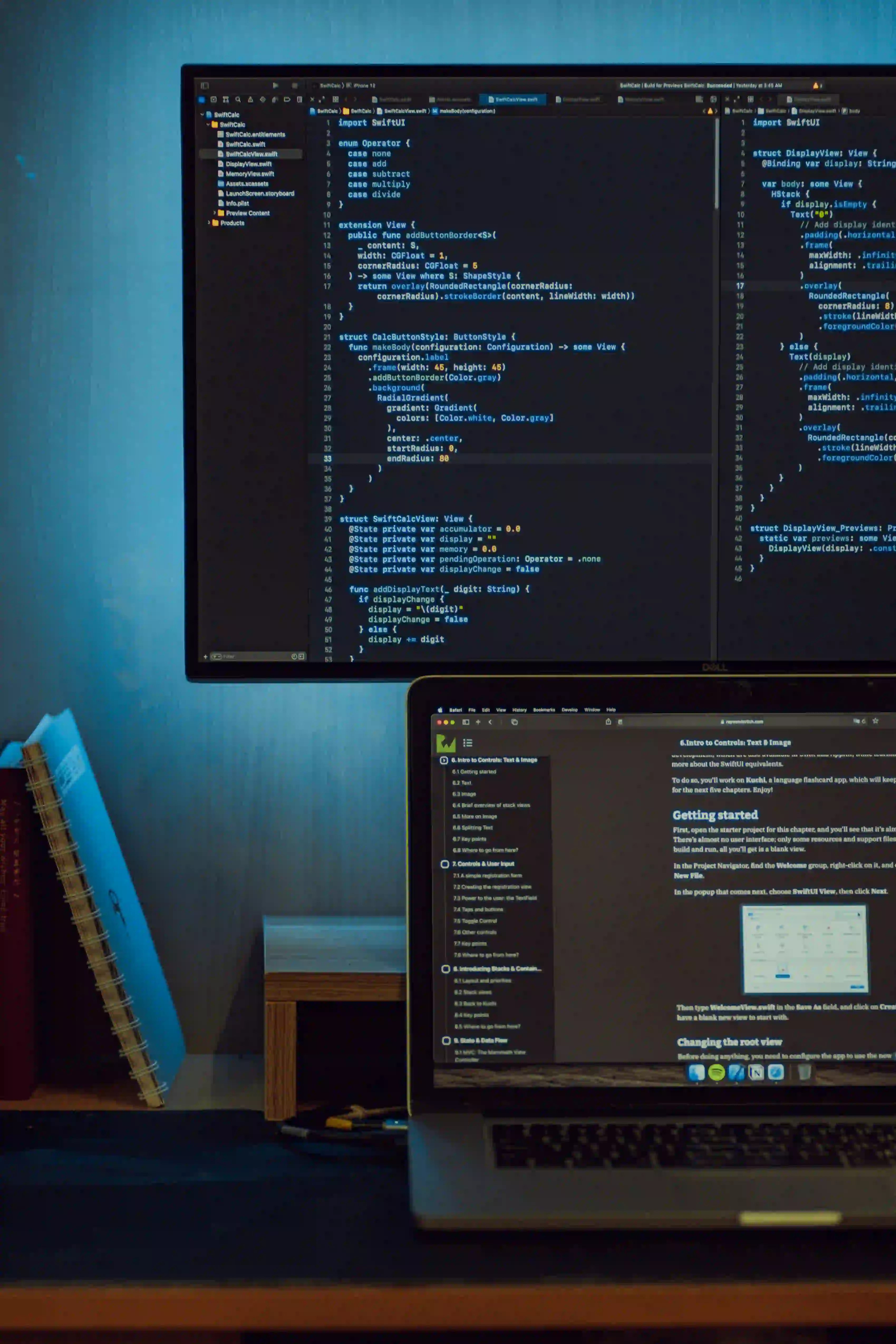
Enhancing Spring Boot Actuator with MVC: A Step-by-Step Guide
Spring Boot Actuator is a powerful feature that provides insight into the running application by exposing operational endpoints. It allows developers to monitor and manage their applications in real-time. While Spring Boot Out-of-the-Box provides many quality endpoints, integrating it with Spring MVC can enhance customization, extendibility, and user experience. In this guide, we'll walk you through how to enhance Spring Boot Actuator with MVC, providing sample code snippets and detailed commentary along the way.
Prerequisites
Before diving into the implementation, ensure you have the following:
- Basic knowledge of Spring Boot and Spring MVC
- Java Development Kit (JDK) installed on your machine (Java 8 or higher)
- Maven or Gradle as a build tool
- An Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse
If you're new to Spring Boot, you can start by checking Spring Boot's official guides for more context.
What is Spring Boot Actuator?
Spring Boot Actuator provides a set of production-ready features to help monitor and manage your application. It includes various endpoints like:
/actuator/health
- shows application health/actuator/info
- provides application information- And many more...
These endpoints provide vital metrics necessary for administrators and DevOps.
Creating a Spring Boot Application
To begin, let's create a simple Spring Boot application. You can use Spring Initializr to generate a project with the necessary dependencies.
- Go to Spring Initializr.
- Select Maven Project.
- Choose Java and the Spring Boot version.
- Add dependencies: Spring Web, Spring Boot Actuator.
- Click on "Generate" to download the project zip file.
Unpack the zip and open it in your IDE. The pom.xml
should include dependencies for Spring Boot and Actuator like so:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
Explanation
spring-boot-starter-web
: This is necessary for creating web applications, including RESTful applications using Spring MVC.spring-boot-starter-actuator
: This starter is what enables all Actuator functionality.spring-boot-starter-thymeleaf
: If we plan on rendering views, Thymeleaf is a great templating engine for that purpose.
Step 1: Enable Actuator Endpoints
In your application.properties
, enable the necessary actuator endpoints you plan to use. For instance:
management.endpoints.web.exposure.include=health,info
management.endpoint.health.show-details=always
Explanation
- The first line determines which endpoints are accessible over HTTP.
- The second line allows us to see detailed health information, which can be helpful during the development phase.
Step 2: Adding MVC Support
To enhance the Actuator with MVC views, we will create a simple Thymeleaf HTML file to visualize information. Create a new directory under src/main/resources/templates
called actuator
and add a file named health.html
:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Health Status</title>
</head>
<body>
<h1>Application Health</h1>
<div th:if="${health}">
<pre th:text="${health}"></pre>
</div>
</body>
</html>
Explanation
In this HTML structure:
- We use Thymeleaf's
th
attributes to bind data from our Spring application. - The
${health}
variable will be populated with the application's health status, providing a clearer view of the app's state.
Step 3: Creating a Controller
Now, let's create a controller that retrieves the actuator's health information and populates the Thymeleaf view. Here’s a simple controller:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.actuate.health.Health;
import org.springframework.boot.actuate.health.HealthEndpoint;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HealthController {
@Autowired
private HealthEndpoint healthEndpoint;
@GetMapping("/actuator/health")
public String health(Model model) {
Health health = healthEndpoint.health();
model.addAttribute("health", health.getStatus().toString());
return "actuator/health";
}
}
Explanation
- This controller uses Spring's
@Controller
annotation to define a controller. - It injects
HealthEndpoint
, which allows access to the health status. - The
health
method retrieves health status information and adds it to the model for the view.
Step 4: Running the Application
Now that we have set up the application, we can run it. Use the following Maven command from your terminal:
./mvnw spring-boot:run
Once the application is up and running, navigate to http://localhost:8080/actuator/health
. You should see the health status displayed by the Thymeleaf page.
Step 5: Securing Endpoints (Optional)
To enhance security, consider adding basic authentication to protect your actuator endpoints. Add Spring Security to your project via Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Then configure the security settings in your application.properties
:
spring.security.user.name=user
spring.security.user.password=password
Explanation
- Adding Spring Security allows access control to your endpoints, securing them with username and password.
Closing the Chapter
Enhancing Spring Boot Actuator with MVC provides a robust solution for monitoring application health and other metrics. By creating a simple Thymeleaf view, we transformed standard actuator endpoints into user-friendly interfaces.
For further reading on Spring Boot and Actuator, consider exploring the following resources:
By integrating and customizing these features, you are well on your way to leveraging the full power of Spring Boot for your application needs. Happy coding!