Boost Your Java App's Performance with AWS Networking Tips
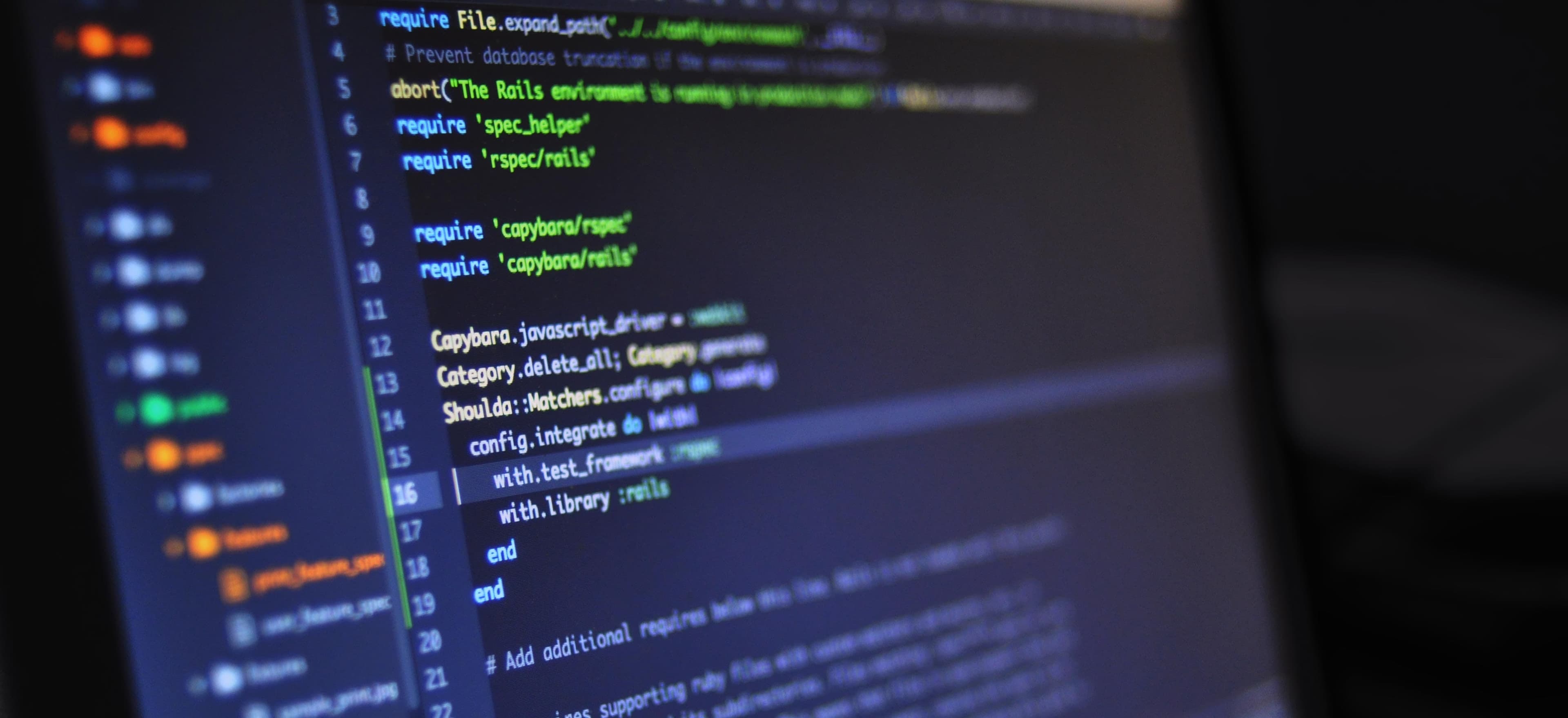
- Published on
Boost Your Java App's Performance with AWS Networking Tips
When developing a Java application, achieving optimal performance is often a chief concern. One of the most critical, yet sometimes overlooked, aspects of application performance is networking, especially when you deploy your Java app on the cloud. Java applications can benefit immensely from understanding and leveraging AWS (Amazon Web Services) networking. This blog post will delve into key AWS networking strategies that specifically enhance Java application performance.
The Importance of Networking in Java Applications
Networking is the backbone of any distributed application. In Java, whether you are working with remote method invocation (RMI), RESTful APIs, or microservices, the way your application communicates can make a significant difference in speed and responsiveness. By adjusting your networking configuration and infrastructure, you can dramatically reduce latency and increase throughput.
Key Considerations for AWS Networking
Before we delve into practical tips, let’s outline some key considerations of AWS networking for Java apps:
- Data Transfer Costs
- Latency
- Scalability
- Security
Armed with these considerations, let’s explore how you can optimize your Java application through AWS networking.
1. Choose the Right VPC Configuration
A Virtual Private Cloud (VPC) is a secure, isolated section of the AWS cloud where you can launch your AWS resources. Every application is unique, so the way you configure your VPC can dramatically affect the performance of your Java application.
Example: Create a VPC with Public and Private Subnets
import com.amazonaws.services.ec2.AmazonEC2;
import com.amazonaws.services.ec2.AmazonEC2ClientBuilder;
import com.amazonaws.services.ec2.model.CreateVpcRequest;
import com.amazonaws.services.ec2.model.CreateVpcResult;
public class CreateVPC {
public static void main(String[] args) {
AmazonEC2 ec2 = AmazonEC2ClientBuilder.defaultClient();
CreateVpcRequest request = new CreateVpcRequest()
.withCidrBlock("10.0.0.0/16");
CreateVpcResult response = ec2.createVpc(request);
System.out.printf("VPC created with ID: %s", response.getVpc().getVpcId());
}
}
Why This Matters: Using separate subnets for various resources (like databases on private subnets) can lower your exposure to external threats. Additionally, it reduces unnecessary data traffic across your network, enabling smoother communication within your application.
2. Optimize Network ACLs and Security Groups
AWS provides Network Access Control Lists (ACLs) and Security Groups that function as virtual firewalls to control incoming and outgoing traffic. Ensuring seamless and efficient traffic can enhance your Java app's responsiveness.
Example: Configure Security Groups
import com.amazonaws.services.ec2.AmazonEC2;
import com.amazonaws.services.ec2.AmazonEC2ClientBuilder;
import com.amazonaws.services.ec2.model.CreateSecurityGroupRequest;
import com.amazonaws.services.ec2.model.CreateSecurityGroupResult;
public class CreateSecurityGroup {
public static void main(String[] args) {
AmazonEC2 ec2 = AmazonEC2ClientBuilder.defaultClient();
CreateSecurityGroupRequest request = new CreateSecurityGroupRequest()
.withGroupName("JavaAppSecurityGroup")
.withDescription("Security group for Java application");
CreateSecurityGroupResult response = ec2.createSecurityGroup(request);
System.out.printf("Security Group created with ID: %s", response.getGroupId());
}
}
Why This Matters: By tightly controlling access to your resources, you can reduce the chances of unauthorized access, which in turn can influence application performance. Also, consider utilizing VPC peering to minimize latency when communicating between different VPCs in your setup.
3. Leverage AWS Direct Connect for Better Latency
When it comes to latency, AWS Direct Connect establishes a dedicated network connection from your premises to AWS. This can drastically reduce latency over standard internet connections, allowing for smoother execution of your Java applications.
How to Use Direct Connect: You can access Direct Connect via the AWS Management Console. Create a new connection and follow the setup instructions provided.
Why This Matters: A dedicated line means reliable bandwidth that doesn't fluctuate based on internet traffic, making it ideal for high-performance applications.
4. Leverage Load Balancers
AWS offers several types of load balancers (Application Load Balancers, Network Load Balancers) that can help in distributing incoming traffic evenly across multiple instances of your Java application. This not only keeps your application responsive but also ensures high availability.
Example: Create an Application Load Balancer
import com.amazonaws.services.elasticloadbalancing.AmazonElasticLoadBalancing;
import com.amazonaws.services.elasticloadbalancing.AmazonElasticLoadBalancingClientBuilder;
import com.amazonaws.services.elasticloadbalancing.model.CreateLoadBalancerRequest;
import com.amazonaws.services.elasticloadbalancing.model.CreateLoadBalancerResult;
public class CreateLoadBalancer {
public static void main(String[] args) {
AmazonElasticLoadBalancing elb = AmazonElasticLoadBalancingClientBuilder.defaultClient();
CreateLoadBalancerRequest request = new CreateLoadBalancerRequest()
.withLoadBalancerName("JavaAppLoadBalancer")
.withSubnets("subnet-12345", "subnet-67890")
.withSecurityGroups("sg-123456");
CreateLoadBalancerResult response = elb.createLoadBalancer(request);
System.out.printf("Load Balancer created with DNS: %s", response.getDNSName());
}
}
Why This Matters: By employing load balancers, you can ease the pressure on specific server instances, ensuring that your app remains responsive under heavy traffic loads.
5. Use Amazon CloudFront for Content Delivery
For high-speed content delivery around the globe, consider using Amazon CloudFront, a web service that accelerates the delivery of your Java app's static and dynamic content.
Why This Matters: CloudFront caches your content at edge locations, reducing the distance data travels and thereby lowering latency. This is particularly important if your users are geographically distributed.
The Bottom Line
Optimizing your Java application's performance using AWS networking strategies can greatly improve user experience and operational efficiency. Therefore, take the time to assess your current setup and consider revisiting the aspects highlighted in this article.
Remember, networking isn't merely an infrastructural component. It directly impacts the performance of your Java applications. For more insights on enhancing your networking capabilities, I recommend checking out the article Master AWS Networking for DevOps in 5 Easy Steps.
By implementing the strategies outlined, you can transform your Java applications into high-performance powerhouses. Happy coding!
Checkout our other articles