Streamline Java Application Networking in AWS for DevOps
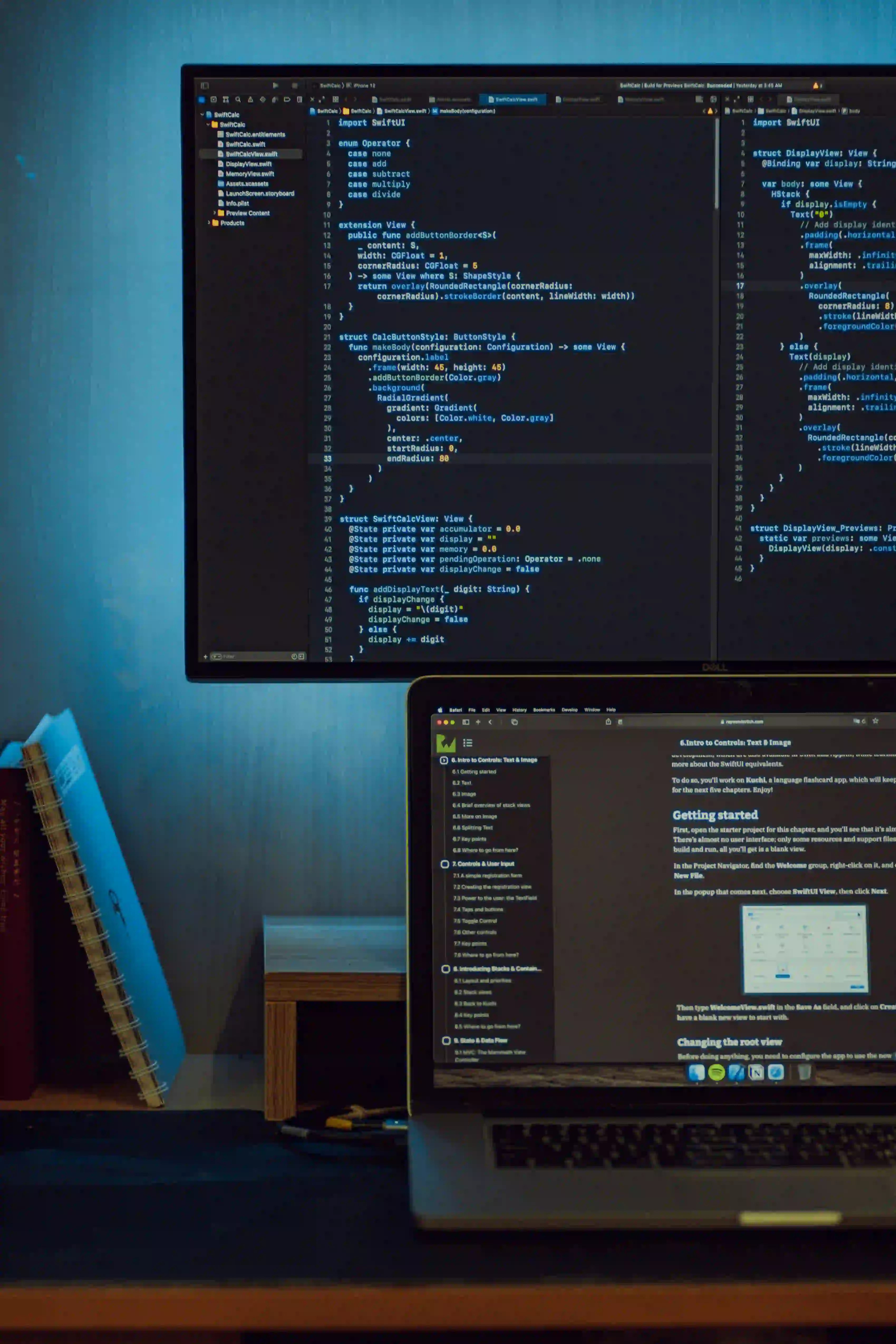
Streamline Java Application Networking in AWS for DevOps
In the realm of modern development and operations (DevOps), efficiency and performance come to the forefront. As Java developers, integrating AWS into our networking strategies can vastly improve our applications. This blog post explores how to streamline Java application networking within AWS, simplifying processes and enhancing functionality.
Understanding the Fundamentals
Before we dive into specific networking strategies, let's clarify what we mean by "networking" in the context of Java applications on AWS. Networking relates to how different components of your application communicate over the internet. This can include APIs, databases, and services hosted on various AWS resources.
The core networking services in AWS that are crucial for Java applications include:
- Amazon VPC (Virtual Private Cloud): Isolated virtual network for your AWS resources.
- AWS Route 53: A scalable DNS web service for domain name management.
- Elastic Load Balancing (ELB): Distributes incoming application traffic across multiple targets.
- AWS Direct Connect: A dedicated network connection between your premises and AWS.
Understanding these services will help you leverage them effectively in your Java applications.
Setting Up Your AWS Environment for Java Networking
The first step in streamlining Java application networking is ensuring your AWS environment is set up correctly to support your application architecture.
Step 1: Setting Up Amazon VPC
A well-designed VPC is crucial. AWS VPC allows you to create a network topology that mimics your on-premises environment.
Example Code Snippet:
import software.amazon.awssdk.services.ec2.Ec2Client;
import software.amazon.awssdk.services.ec2.model.CreateVpcRequest;
import software.amazon.awssdk.services.ec2.model.CreateVpcResponse;
public class VPCManager {
public static void main(String[] args) {
Ec2Client ec2 = Ec2Client.create();
CreateVpcRequest request = CreateVpcRequest.builder()
.cidrBlock("10.0.0.0/16")
.instanceTenancy("default")
.build();
CreateVpcResponse response = ec2.createVpc(request);
System.out.println("Created VPC: " + response.vpc().vpcId());
}
}
Why Use VPC?
Using VPC ensures that your applications can run within a defined network boundary, contributing to security and performance. It enables fine-grained control of networking, such as the ability to define subnets, route tables, and more.
Step 2: Configure Subnets
Within your VPC, create public and private subnets. Use public subnets for resources that must be exposed to the internet, while private subnets are for databases or application servers.
Example Code Snippet:
// Example to create a subnet in the VPC
import software.amazon.awssdk.services.ec2.model.CreateSubnetRequest;
CreateSubnetRequest createSubnetRequest = CreateSubnetRequest.builder()
.vpcId("your-vpc-id")
.cidrBlock("10.0.1.0/24")
.availabilityZone("us-west-2a")
.build();
ec2.createSubnet(createSubnetRequest);
Why Opt for Subnets?
Subnets allow for optimal organization of resources. Public subnets can host web servers while private subnets can house sensitive data or backend services. This separation maximizes security and improves application performance.
Utilizing Elastic Load Balancing
Once your subnets are set up, consider using Elastic Load Balancing to manage traffic across your application instances. This provides fault tolerance and improves application availability.
Example Configuration:
- Create a target group.
- Register your Java application instances.
- Create an application load balancer.
Java Code to Register Instances:
import software.amazon.awssdk.services.elbv2.Elbv2Client;
public class LoadBalancerManager {
public void registerInstances(String targetGroupArn) {
Elbv2Client elbv2Client = Elbv2Client.create();
RegisterTargetsRequest registerRequest = RegisterTargetsRequest.builder()
.targetGroupArn(targetGroupArn)
.targets(TargetDescription.builder()
.id("instance-id")
.build())
.build();
elbv2Client.registerTargets(registerRequest);
}
}
Why Load Balancing?
Load balancing helps distribute incoming traffic efficiently, thus preventing overload on any single instance and ensuring your application remains performant under load.
Optimal DNS Management with Route 53
DNS management is a crucial aspect of networking. Route 53 ensures that your domain names are correctly resolved to the appropriate AWS resource.
Implementing Route 53:
- Create a hosted zone.
- Define record sets for your resources.
Java Snippet to Create a Hosted Zone:
import software.amazon.awssdk.services.route53.Route53Client;
import software.amazon.awssdk.services.route53.model.CreateHostedZoneRequest;
Route53Client route53Client = Route53Client.create();
CreateHostedZoneRequest hostedZoneRequest = CreateHostedZoneRequest.builder()
.name("example.com")
.callerReference("unique-identifier")
.hostedZoneConfig(HostedZoneConfig.builder().comment("Hosted Zone for example.com").build())
.build();
route53Client.createHostedZone(hostedZoneRequest);
Why Use Route 53?
Using Route 53 ensures that users can find your application easily. It improves user experience by efficiently directing traffic and managing failover scenarios.
Consider Direct Connect for Low Latency
For enterprises requiring low latency, AWS Direct Connect can be a valuable resource. This dedicated network connection can optimize performance for Java applications, particularly in hybrid environments.
Why Use Direct Connect?
By providing a private connection to AWS, Direct Connect minimizes network costs and increases bandwidth throughput while providing a more consistent network experience than traditional internet connections.
Monitoring and Optimization
After setting up your networking components, monitoring is key to maintaining performance and availability. AWS CloudWatch provides tools to monitor your application health and performance metrics proactively.
Closing the Chapter
Integrating AWS networking into your Java application allows for a more efficient, secure, and performant infrastructure. By leveraging services like VPC, ELB, Route 53, and Direct Connect, DevOps professionals optimize their applications for an ever-increasing user base.
For more insights on enhancing networking strategies specifically tailored for DevOps, check out our article on "Master AWS Networking for DevOps in 5 Easy Steps" at configzen.com/blog/master-aws-networking-devops-5-easy-steps.
With a solid understanding and implementation of these concepts, Java developers can not only improve their application networking but also play a pivotal role in the success of their organization’s cloud journey.
For further discussions on optimizing your Java applications on AWS, feel free to comment below or reach out on platforms like LinkedIn.