Optimizing Java Applications with AWS Networking Strategies
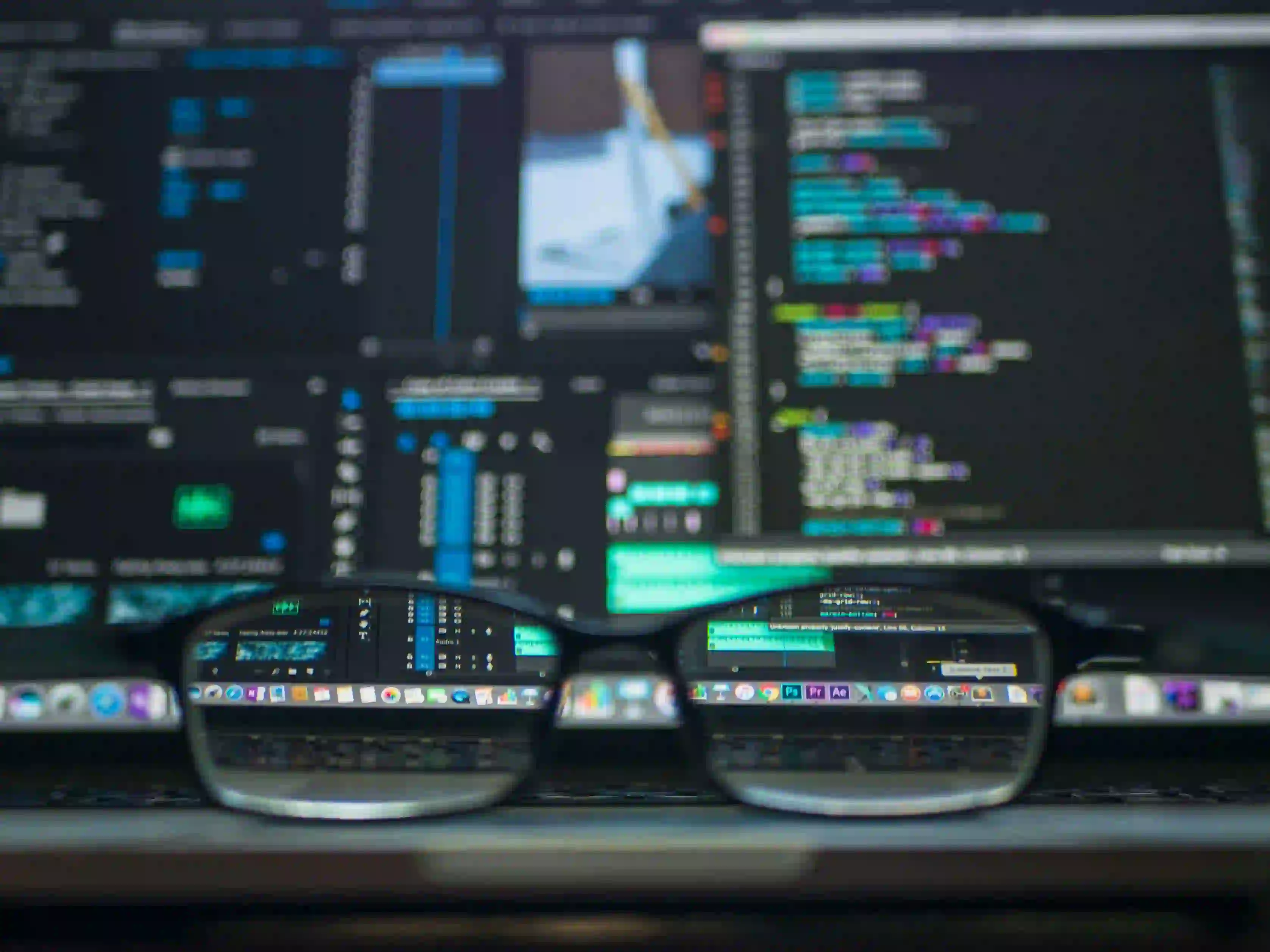
Optimizing Java Applications with AWS Networking Strategies
In today's cloud-driven world, the efficiency of Java applications heavily relies on how you manage networking on platforms like Amazon Web Services (AWS). The interplay between your Java application and AWS networking capabilities can significantly impact performance, latency, and cost-effectiveness. This post explores practical strategies to optimize your Java applications using AWS networking.
Understanding AWS Networking Fundamentals
Before diving into optimization techniques, it's crucial to understand the basic components of AWS networking. Key elements include:
- Virtual Private Cloud (VPC): A dedicated virtual network for your AWS resources.
- Subnets: Segments of your VPC where you can define resources.
- Internet Gateway: Allows communication between your VPC and the internet.
- Route Tables: Control the routing of traffic within your VPC.
This foundation enables you to structure your resources optimally, ensuring they can perform at their best.
Leveraging the Power of VPC Configuration
By configuring your VPC appropriately, you can create a more efficient network environment for your Java applications. Here are some steps to help you set it up effectively.
Create Public and Private Subnets
Dividing your environment into public and private subnets helps isolate critical database instances from the public internet, minimizing risk.
// Example of AWS CLI command to create a VPC
aws ec2 create-vpc --cidr-block 10.0.0.0/16
Why? This separation improves security and can help decrease network latency by ensuring that backend services are only accessible to internal resources.
Utilize AWS Direct Connect
For Java applications needing to interact with on-premises resources, AWS Direct Connect can offer more reliable and consistent networking performance.
// Example of setting up AWS Direct Connect through AWS Management Console
Why? Direct Connect establishes a dedicated network connection. This reduces latency and provides a more stable bandwidth compared to standard internet connections.
Optimizing API Calls for Latency Reduction
Java applications often rely on API calls, both internal and external. You can optimize these calls for a better user experience:
Implement Amazon API Gateway
Using Amazon API Gateway, you can create, publish, and maintain REST APIs securely and reliably.
// Pseudocode for configuring an API Gateway
apiGateway.createApi("MyJavaApplicationAPI", new ApiConfig());
Why? This service can manage traffic spikes efficiently and handle authentication, so your Java application can focus on what it does best: serving business logic.
Use AWS Lambda for Event-Driven Architecture
To optimize processing, consider using AWS Lambda, which allows you to run functions in response to certain events, such as incoming API calls.
public class MyLambdaFunction {
public String handleRequest(InputType input, Context context) {
// Handle request logic
return "Response";
}
}
Why? By decoupling your application components and letting AWS Lambda manage scaling, you can enhance your application's responsiveness and reduce operational costs.
Data Management and Caching
Efficient data management and caching strategies can significantly enhance the performance of your Java application.
Employ Amazon RDS with Read Replicas
Utilizing Amazon RDS (Relational Database Service) with read replicas helps to distribute database load effectively.
-- SQL syntax for creating a read replica
CREATE DATABASE my_replica
WITH REPLICA OF my_db;
Why? This approach reduces latency by allowing read operations to be performed on replicas, thereby freeing up resources for more critical write operations.
Implement Amazon ElastiCache
Caches stored in-memory can drastically improve data retrieval speeds. By using Amazon ElastiCache, you can store frequently accessed data closer to your application.
// Pseudocode for using ElastiCache with a Java application
Jedis jedis = new Jedis("my-elasticache-cluster");
jedis.set("key", "value");
Why? This reduces the need for repeated database calls, leading to faster response times for users.
Monitoring and Troubleshooting
Monitoring your application in real-time is an essential part of keeping it optimized.
Utilize Amazon CloudWatch
Integrating Amazon CloudWatch into your Java application lets you track performance metrics effortlessly.
import com.amazonaws.services.cloudwatch.AmazonCloudWatch;
import com.amazonaws.services.cloudwatch.AmazonCloudWatchClientBuilder;
AmazonCloudWatch cloudWatch = AmazonCloudWatchClientBuilder.defaultClient();
cloudWatch.putMetricData("MyMetricNamespace", "MyMetricName", value);
Why? CloudWatch provides vital insights into application performance, helping developers detect potential issues proactively.
Leverage VPC Flow Logs
These logs capture information about the IP traffic going to and from network interfaces in your VPC.
# VPC Flow Logs command
aws ec2 create-flow-logs --resource-type VPC --resource-id vpc-12345678 --traffic-type ALL
Why? Analyzing these logs allows you to pinpoint bottlenecks and optimize your networking strategy further.
My Closing Thoughts on the Matter
Optimizing Java applications through effective AWS networking strategies can lead to substantial improvements in performance, reliability, and security. Whether you’re leveraging VPCs, using API Gateway, or implementing caching solutions, each element contributes to a robust application architecture.
By applying these strategies, you not only streamline your operations but also position your application for future growth. As systems become more complex and demands increase, having a well-structured network architecture is paramount.
For a deeper understanding of AWS networking, consider checking out the article "Master AWS Networking for DevOps in 5 Easy Steps" at configzen.com/blog/master-aws-networking-devops-5-easy-steps.
By adopting the techniques discussed in this article and staying up-to-date with best practices, you can ensure your Java applications are both resilient and efficient in the cloud landscape.