Unlocking Java's Healing Potential: Integrating Health Apps
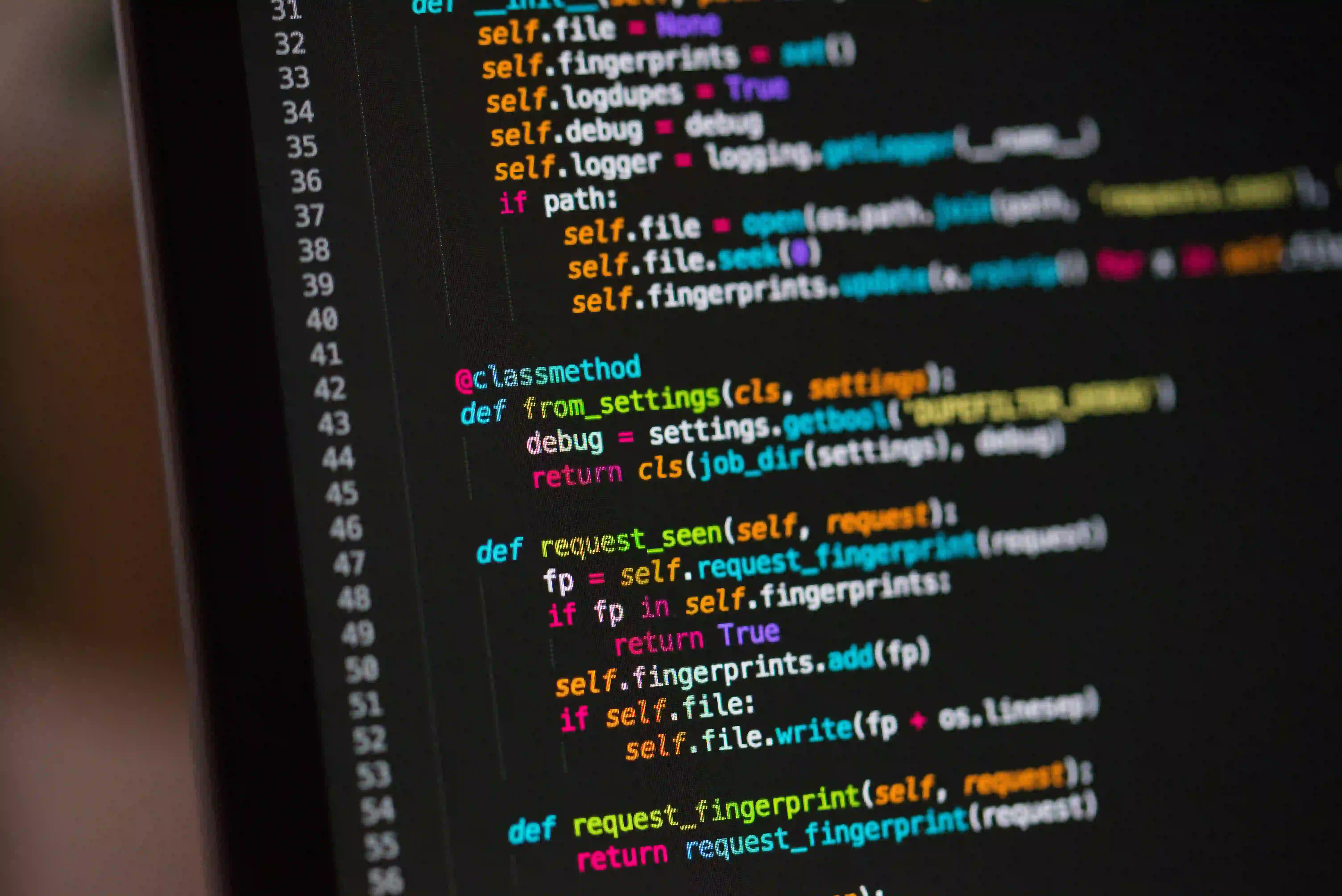
Unlocking Java's Healing Potential: Integrating Health Apps
In the era of health technology, leveraging the capabilities of programming languages to create health-related applications has never been more crucial. Among all the programming languages available, Java stands out due to its versatility, portability, and robust frameworks. Whether you're a seasoned programmer or a newbie venturing into the realm of health tech, this blog post aims to delve into integrating health applications using Java effectively.
Why Java for Health Apps?
Java's Object-Oriented programming paradigm provides a solid foundation for creating modular applications that are easier to maintain and update. Additionally, its extensive ecosystem—with libraries and frameworks like Spring and Hibernate—enables developers to build applications efficiently.
Moreover, Java's platform independence allows applications to run on various devices, from desktop computers to Android smartphones. This versatility is especially beneficial for health apps, which need to function seamlessly across multiple platforms.
Components of a Health Application
Before diving into code, we need to outline what components make up a typical health application. Depending on the nature of your health app, certain features may include:
- User Profiles: Storing user health-related information.
- Data Tracking: Monitoring metrics such as steps, calories burned, or blood pressure levels.
- Reminders and Notifications: Keeping users engaged and informed about their health regimen.
- Data Visualization: Offering visual insights into tracked health metrics.
- Integration with APIs: For accessing broader health data, like nutritional databases.
Example: User Profile Management
Let’s start by creating a simple user profile model in Java. We will use this as the foundation for managing user data.
public class UserProfile {
private String name;
private int age;
private double height; // in meters
private double weight; // in kilograms
public UserProfile(String name, int age, double height, double weight) {
this.name = name;
this.age = age;
this.height = height;
this.weight = weight;
}
// Getter and Setter methods
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
// Method to calculate BMI
public double calculateBMI() {
return weight / (height * height);
}
}
Why This Code is Important
The UserProfile
class encapsulates the primary user information required for health calculations. The calculateBMI
method offers instant insight into a user’s health, thus enhancing user engagement.
Data Tracking
Next, let's integrate basic data tracking features. This can monitor user activities, such as daily step count.
import java.util.ArrayList;
import java.util.List;
public class ActivityTracker {
private List<Integer> dailySteps;
public ActivityTracker() {
this.dailySteps = new ArrayList<>();
}
public void addDailySteps(int steps) {
dailySteps.add(steps);
}
public int getTotalSteps() {
return dailySteps.stream().mapToInt(Integer::intValue).sum();
}
public double getAverageSteps() {
return dailySteps.stream().mapToInt(Integer::intValue).average().orElse(0);
}
}
The Utility of the ActivityTracker
This class helps track daily steps and provides methods to calculate total and average steps. Such straightforward data tracking promotes long-term user motivation and health improvement.
Notifications and Reminders
To keep users engaged, integrating a reminder system can be a game changer. Below is a rudimentary implementation using Java's built-in Timer
class.
import java.util.Timer;
import java.util.TimerTask;
public class Reminder {
private Timer timer;
public Reminder() {
timer = new Timer();
}
public void setReminder(long delayMillis) {
timer.schedule(new TimerTask() {
@Override
public void run() {
System.out.println("Reminder: Time to take your health metrics!");
}
}, delayMillis);
}
}
Engaging Users with Reminders
The Reminder
class allows users to set reminders in the form of alerts. This feature is invaluable for health apps, as it prompts users to log their metrics consistently.
Integrating Third-Party APIs
To enhance functionality, integrate your app with third-party APIs. When it comes to nutrition-based health applications, consider integrating with the FoodData Central API. Such APIs provide extensive databases for food items and their nutritional information.
Here's a simple example of how you might structure an API client in Java:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class NutritionAPIClient {
private static final String API_KEY = "YOUR_API_KEY_HERE";
private static final String API_URL = "https://api.nal.usda.gov/fdc/v1/foods";
public String getFoodData(String foodId) throws Exception {
URL url = new URL(API_URL + "/" + foodId + "?api_key=" + API_KEY);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
try (BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()))) {
StringBuilder response = new StringBuilder();
String inputLine;
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
return response.toString();
}
}
}
Why APIs Matter
Integrating with an API enriches the app functionality and provides users with immediate access to broader food data, empowering them to make better dietary choices.
Data Visualization
Finally, a health app's effectiveness can hinge on how well users understand their health data. Utilizing libraries such as JavaFX or Swing can help create visual representations of data.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.chart.BarChart;
import javafx.scene.chart.NumberAxis;
import javafx.scene.chart.XYChart;
import javafx.stage.Stage;
public class HealthDataChart extends Application {
@Override
public void start(Stage stage) {
stage.setTitle("Health Data Tracking");
final NumberAxis xAxis = new NumberAxis();
final NumberAxis yAxis = new NumberAxis();
final BarChart<Number, Number> bc = new BarChart<>(xAxis, yAxis);
xAxis.setLabel("Days");
yAxis.setLabel("Steps");
XYChart.Series series = new XYChart.Series();
series.setName("Tracked Steps");
series.getData().add(new XYChart.Data(1, 5000));
series.getData().add(new XYChart.Data(2, 7000));
series.getData().add(new XYChart.Data(3, 8000));
Scene scene = new Scene(bc, 800, 600);
bc.getData().addAll(series);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Enriching User Experience through Visualization
Visual data representation encourages users to stay informed about their health. The HealthDataChart
class utilizes JavaFX to create a simple bar graph, thus helping users visualize their daily step counts.
Bringing It All Together
Building a health application in Java opens up vast possibilities. From user profile management to reminders and data visualization, Java provides developers with a powerful toolkit for creating user-centered applications.
Moreover, as health apps increasingly focus on engaging users, the methods discussed enhance user motivation and provide essential insights into fitness journeys. As you delve into integrating health technology further, don't overlook articles that discuss similar topics, such as the comprehensive insights in "Kamillentee: Ein Wundermittel für Gesundheit & Wohlbefinden".
By integrating thoughtful features and leveraging Java's robust capabilities, we can no doubt create applications that are not only functional but also transformative to users’ health and well-being. Happy coding!