Mastering Cache Management in Java with Spring Framework
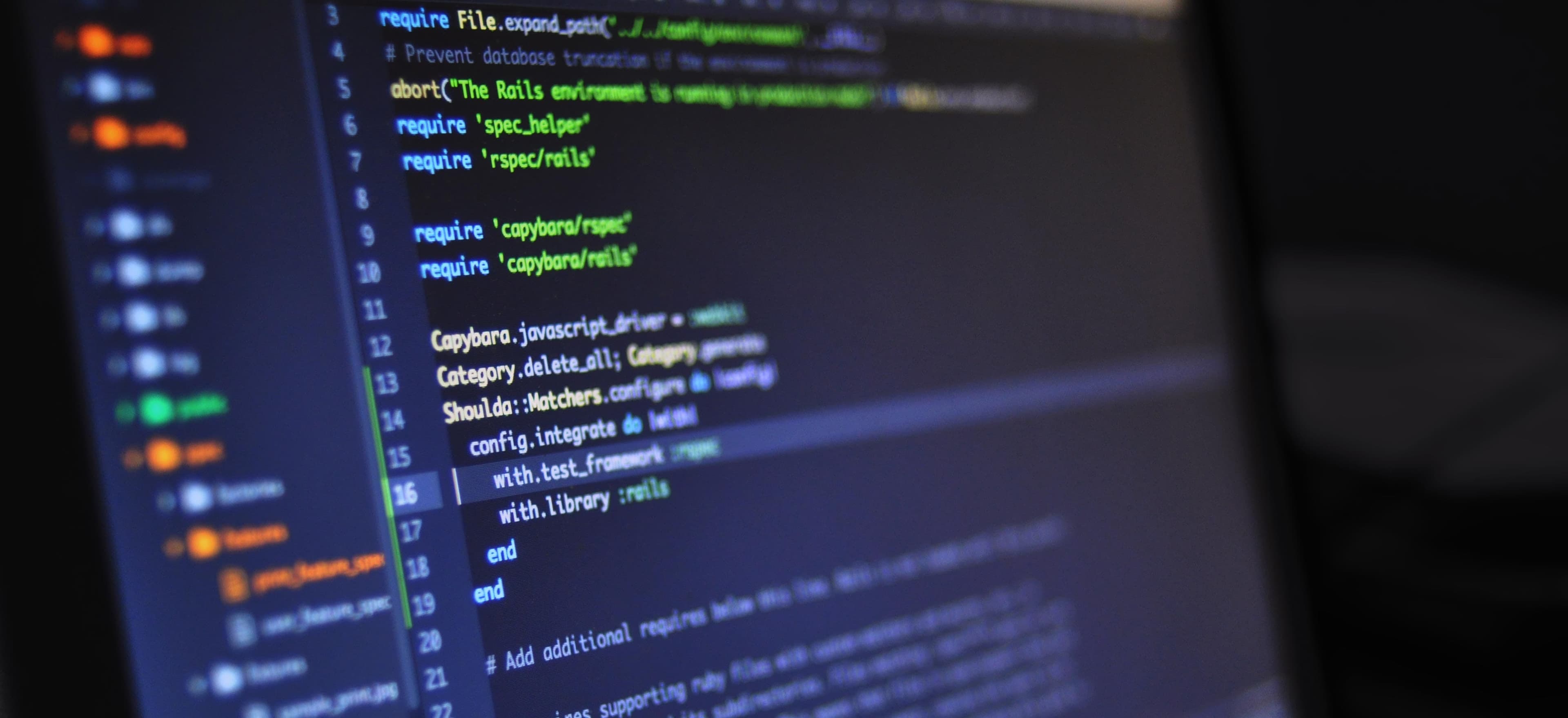
- Published on
Mastering Cache Management in Java with Spring Framework
Caching is a crucial aspect of modern application development, especially when striving for high performance and reduced latency. In the realm of Java, the Spring Framework provides powerful caching abstractions that simplify the implementation of caching strategies.
In this article, we will explore how to manage cache effectively using Spring, highlight its various configurations, and provide practical code examples. By the end, you'll have a solid understanding of how to integrate caching into your Java applications seamlessly.
What is Cache Management?
Cache management involves storing data in a temporary storage layer (cache) to reduce the time it takes to access frequently used data. This approach can dramatically improve application performance by minimizing data retrieval times from more resource-intensive sources, such as databases.
In simple terms, the cache acts as a middleman that retains frequently accessed data, enabling faster retrieval during successive requests. However, efficient cache management is essential to avoid stale data, ensure data consistency, and balance memory usage.
Why Use Caching?
-
Improved Performance: Caching reduces the number of requests sent to your database or API by storing responses closer to your application.
-
Reduced Latency: With faster access times, the user experience improves significantly.
-
Lower Backend Load: Reduced demands on the backend allow you to scale more efficiently and allocate resources where they are most needed.
-
Cost Efficiency: Utilizing cache can diminish operational costs by optimizing resource use.
Using Caching with Spring Framework
The Spring Framework provides a comprehensive caching abstraction that simplifies cache management. With Spring, you can easily integrate caches in your applications using annotations, XML configuration, or Java configuration.
Let's delve into the basic steps for implementing Spring's cache management capabilities.
Step 1: Add Dependencies
Before using caching in your Spring application, you need to include the necessary dependencies in your pom.xml
if you are using Maven. Ensure you have the following:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
</dependency>
The spring-boot-starter-cache
dependency sets up the cache support, while ehcache
is a popular caching library for Java.
Step 2: Enable Caching
Enable caching in your Spring Boot application by using the @EnableCaching
annotation in your main application class.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cache.annotation.EnableCaching;
@SpringBootApplication
@EnableCaching
public class CacheApplication {
public static void main(String[] args) {
SpringApplication.run(CacheApplication.class, args);
}
}
By adding @EnableCaching
, you inform Spring to look for caching annotations in your application.
Step 3: Configure Cache
You can configure your cache management in multiple ways, including Java configuration, annotations, or XML. Let's focus on Java configuration for this example.
import org.springframework.cache.CacheManager;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.cache.concurrent.ConcurrentMapCacheManager;
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("items");
}
}
In this example, the ConcurrentMapCacheManager
is used, which stores the cache in memory. Note the name "items," which represents our cache name.
Using Caching Annotations
Spring provides a set of annotations to denote caching behavior easily.
Cacheable
The @Cacheable
annotation indicates a method's return value can be cached. Here's how to use it:
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class ItemService {
@Cacheable("items")
public Item getItem(Long itemId) {
simulateSlowService(); // Simulate delay
return findItem(itemId);
}
private void simulateSlowService() {
try {
Thread.sleep(3000); // Simulate a time-consuming operation
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
private Item findItem(Long itemId) {
// Simulated method to retrieve the item from a database
return new Item(itemId, "Sample Item");
}
}
Why use @Cacheable
?: This annotation caches the result of getItem
method calls. If the method is called with the same itemId
, the cached result is returned instead of executing the method again, drastically reducing response times.
Cache Evict
When data changes, it’s crucial to clear the cache. The @CacheEvict
annotation is used for this purpose.
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.stereotype.Service;
@Service
public class ItemService {
// Other methods
@CacheEvict(value = "items", key = "#itemId")
public void updateItem(Long itemId, Item item) {
// Logic to update the item
// After updating, the cache for that itemId will be cleared
}
}
Why use @CacheEvict
?: This annotation ensures that stale data doesn't linger in the cache after changes, maintaining data validity.
Caching All Entries
Sometimes it's necessary to clear the cache entirely. You can achieve this using the allEntries
attribute.
@CacheEvict(value = "items", allEntries = true)
public void clearCache() {
// Logic to clear cache
}
This method will clear all entries from the items
cache, useful in scenarios where you need to refresh the entire dataset.
Advanced Cache Management
In real applications, you may need to consider different caching strategies like time-based expiration or cache size limits. This can be configured in your chosen caching provider.
For example, with EhCache, you can configure an XML file to set these properties:
<ehcache>
<defaultCache
maxEntriesLocalHeap="1000"
eternal="false"
timeToLiveSeconds="120"/>
</ehcache>
This configuration specifies that the cache can hold up to 1000 entries, with a lifespan of 120 seconds.
Real-World Use Case
Imagine an application where users frequently request product details. By implementing caching for product data, the application can return details instantly after the first request, preventing needless database queries.
If you find yourself struggling with cache management in conjunction with other frameworks, such as React, consider reading the article titled "Struggling with Cache Management in React Query?" at https://tech-snags.com/articles/struggling-cache-management-react-query.
The Closing Argument
Effective cache management in Java applications using the Spring Framework can significantly enhance performance and user experience. With easy-to-use annotations like @Cacheable
and @CacheEvict
, integrating caching into your application framework becomes seamless.
Whether you're building a simple CRUD application or a complex microservice, harnessing the power of caching will lead to performance improvements and resource savings. So, start incorporating caching strategies to make your Java applications more efficient today!
Checkout our other articles