Mastering SOLID Principles to Enhance Java Frameworks
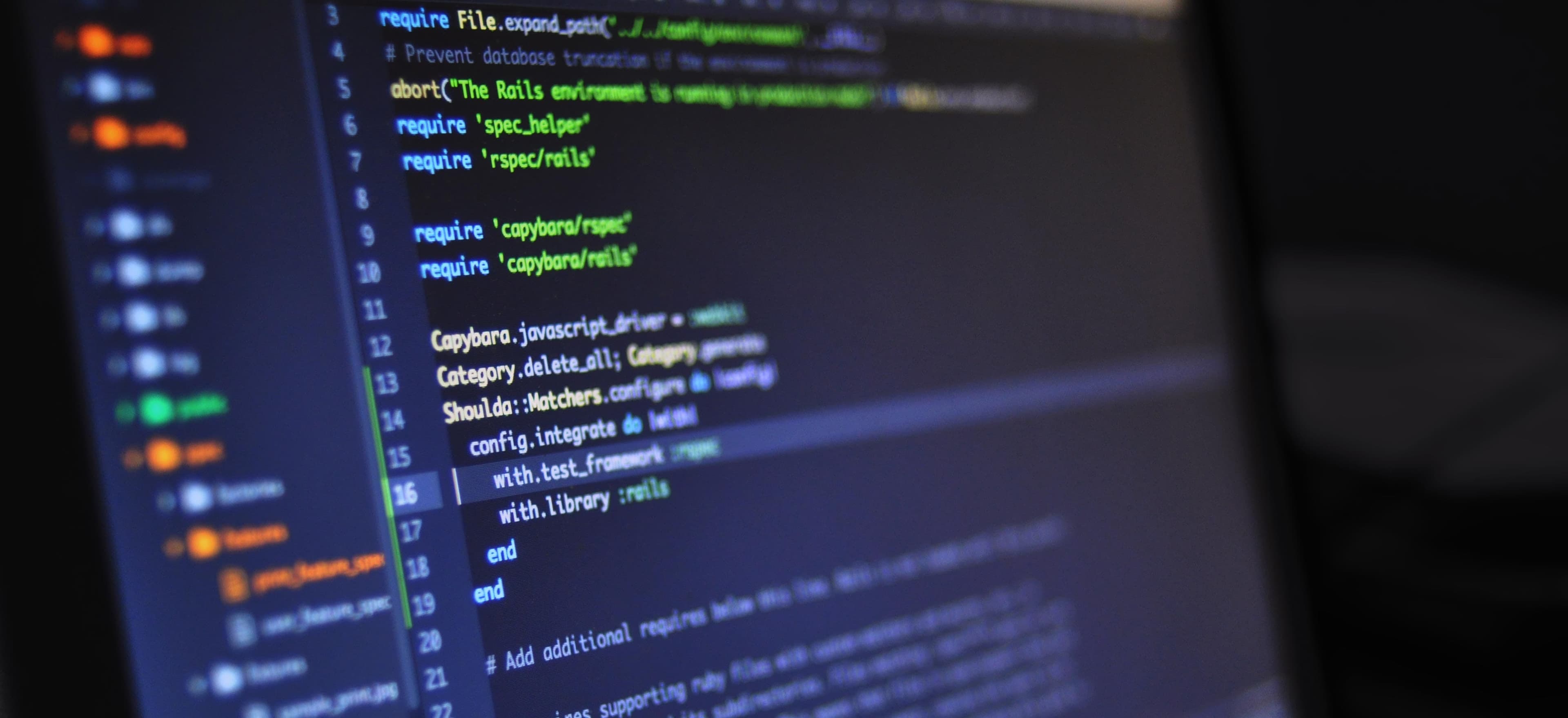
- Published on
Mastering SOLID Principles to Enhance Java Frameworks
When developing software, particularly in Java, following certain foundational principles can make the difference between a robust application and a codebase that is difficult to maintain and scale. One sets of principles that stands out are the SOLID principles. These are five design principles that can help developers create more understandable, flexible, and maintainable code.
In this blog post, we will explore each of the SOLID principles in detail, with examples applicable to Java frameworks, showcasing how they can enhance your software development process. We will also draw insights from the existing article titled "Why Ignoring the SOLID Principles Could Break Your Code".
Overview of SOLID Principles
The SOLID acronym represents five important design principles:
- S - Single Responsibility Principle (SRP)
- O - Open/Closed Principle (OCP)
- L - Liskov Substitution Principle (LSP)
- I - Interface Segregation Principle (ISP)
- D - Dependency Inversion Principle (DIP)
By adhering to these principles, software developers can create systems that are easier to understand and modify, ultimately saving time and reducing bugs.
1. Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. This means that each class should focus on a single task or functionality. When a class has multiple responsibilities, changes to one responsibility can inadvertently affect another, leading to unpredictability.
Example:
public class User {
private String name;
private String email;
public User(String name, String email) {
this.name = name;
this.email = email;
}
public void sendEmail(String message) {
// Code to send email
}
// Other user-related methods...
}
In this example, the User
class has two responsibilities: holding user data and sending email. According to SRP, we should refactor this.
Refactored Example:
public class User {
private String name;
private String email;
public User(String name, String email) {
this.name = name;
this.email = email;
}
// User-related methods...
}
public class EmailService {
public void sendEmail(User user, String message) {
// Code to send email
}
}
Now, the User
class solely focuses on user data, while the EmailService
manages the email sending responsibilities. This clear separation makes the code easier to maintain and understand.
2. Open/Closed Principle (OCP)
The Open/Closed Principle states that classes should be open for extension but closed for modification. This encourages developers to extend existing class behavior without altering their source code, thereby reducing the risk of introducing new bugs.
Example:
public class Shape {
public double area() {
// Default implementation
return 0;
}
}
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
public class Square extends Shape {
private double side;
public Square(double side) {
this.side = side;
}
@Override
public double area() {
return side * side;
}
}
In this example, we can add more shapes without modifying existing classes. This makes the system easily extensible—a key element of maintaining a clean architecture.
3. Liskov Substitution Principle (LSP)
The Liskov Substitution Principle asserts that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. Violating LSP leads to unexpected behavior and bugs.
Example:
public class Bird {
public void fly() {
System.out.println("Flying");
}
}
public class Ostrich extends Bird {
@Override
public void fly() {
throw new UnsupportedOperationException("Ostrich can't fly");
}
}
The Ostrich
class violates LSP because it cannot be substituted for Bird
without throwing an exception.
Ideal Solution:
You can use an interface to separate flying birds from non-flying ones:
public interface Flyable {
void fly();
}
public class Sparrow implements Flyable {
@Override
public void fly() {
System.out.println("Sparrow flying");
}
}
public class Ostrich {
// Ostrich specific behavior.
}
By segregating the flying capability, we maintain polymorphism correctly, complying with LSP.
4. Interface Segregation Principle (ISP)
The Interface Segregation Principle states that no client should be forced to depend on methods it doesn’t use. In simpler terms, it’s better to have many smaller, client-specific interfaces than a large, all-encompassing one.
Example:
public interface Worker {
void work();
void eat();
}
public class WorkerImpl implements Worker {
@Override
public void work() {
// Working implementation ...
}
@Override
public void eat() {
// Eating implementation ...
}
}
public class Robot implements Worker {
@Override
public void work() {
// Working implementation ...
}
@Override
public void eat() {
// Robots don't eat, so this violates ISP.
}
}
Refactored Example:
By separating functionalities, we can create interfaces specific to client needs.
public interface Workable {
void work();
}
public interface Eatable {
void eat();
}
public class WorkerImpl implements Workable, Eatable {
@Override
public void work() {
// Working implementation ...
}
@Override
public void eat() {
// Eating implementation ...
}
}
public class Robot implements Workable {
@Override
public void work() {
// Working implementation ...
}
}
Now, Robot
does not need to implement eat
, adhering to the ISP.
5. Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules but rather both should depend on abstractions. This promotes loose coupling, making systems easier to manage.
Example:
public class LightBulb {
public void turnOn() {
System.out.println("LightBulb turned on");
}
public void turnOff() {
System.out.println("LightBulb turned off");
}
}
public class Switch {
private LightBulb lightBulb;
public Switch(LightBulb lightBulb) {
this.lightBulb = lightBulb;
}
public void operate() {
lightBulb.turnOn(); // tight coupling
}
}
Here, the Switch
class directly depends on LightBulb
. If we needed to use another light implementation, it would require changing the Switch
code.
Refactored Example:
public interface Light {
void turnOn();
void turnOff();
}
public class LightBulb implements Light {
@Override
public void turnOn() {
System.out.println("LightBulb turned on");
}
@Override
public void turnOff() {
System.out.println("LightBulb turned off");
}
}
public class Switch {
private Light light;
public Switch(Light light) {
this.light = light; // oearly dependency injection
}
public void operate() {
light.turnOn();
}
}
By using an interface, the Switch
is now decoupled from specific light implementations, following the DIP, which enhances flexibility.
Key Takeaways
Mastering the SOLID principles is essential for every Java developer. By incorporating these principles into your projects, you can greatly improve code readability, maintainability, and scalability. Each principle has its own merits and should be considered carefully during the design phase of any application.
For further exploration on the implications of neglecting these principles, take a look at the article titled "Why Ignoring the SOLID Principles Could Break Your Code".
In today’s fast-paced development world, having a solid architectural foundation is not just beneficial; it’s imperative. Start implementing these principles today to enhance your Java frameworks, and witness the transformative power of clean, maintainable code.