Debugging JavaScript Blob Errors in Your Java Web Application
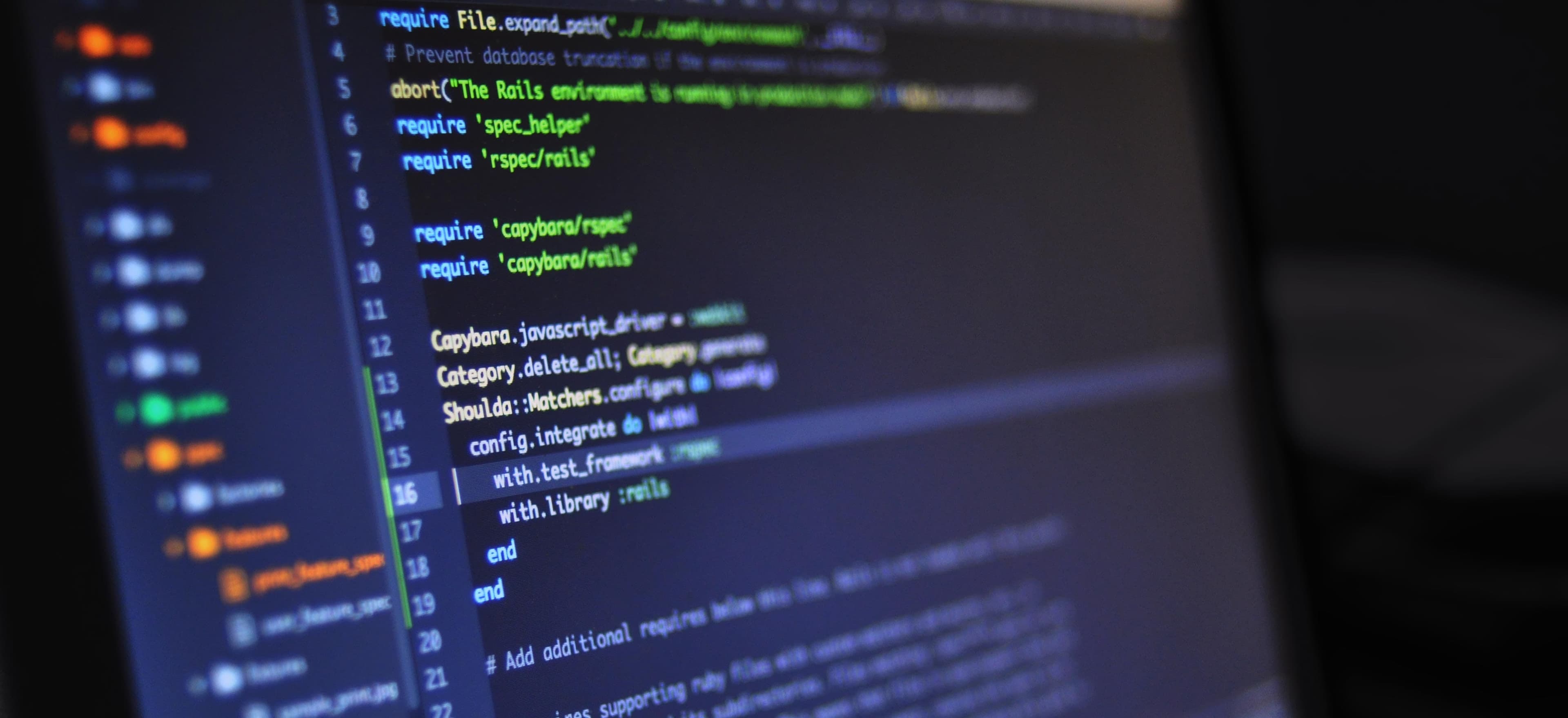
- Published on
Debugging JavaScript Blob Errors in Your Java Web Application
In the landscape of modern web applications, JavaScript plays a pivotal role, especially when handling large datasets or files. One of the features JavaScript offers is the Blob object, which allows for representing binary data. However, many developers encounter issues when implementing this feature within their Java web applications. In this article, we will discuss common Blob-related errors, strategies for debugging them, and how integrating Java with JavaScript can enhance your applications' functionality.
Understanding JavaScript Blobs
A Blob (Binary Large Object) is essentially a file-like object of immutable, raw data. Blobs are especially useful when working with file uploads or downloads, as they enable developers to handle binary data streams more efficiently. In modern web applications, it is common to use Blobs in scenarios such as:
- Uploading images/files from the user’s system
- Processing binary data for API calls
- Creating downloadable resources on the fly
Nevertheless, when faced with errors during bulk import operations, it becomes crucial to have a clear understanding of how to debug these issues effectively.
Common JavaScript Blob Errors
-
Error 400: Bad Request
- This typically indicates malformed data being sent to your server. Ensure that the Blob is correctly defined and that the server is equipped to handle the incoming data format.
-
File Not Found
- When attempting to access a file that does not exist, the Blob constructor will return an error. Verify that the filepath is accurate.
-
Network Errors During uploads
- Temporary network issues may affect file uploads, leading to incomplete or interrupted data. Implementing retry logic can help alleviate this problem.
-
Cross-Origin Restrictions
- Accessing Blob data from a different domain can sometimes trigger CORS issues. Always ensure that your server is set up to allow cross-origin requests if necessary.
-
Memory Limits Exceeded
- With large Blobs, you may hit memory limits imposed by the browser. Consider chunking large files into smaller pieces.
Setting Up A Java Web Application
Integrating Java with JavaScript for handling Blobs requires a well-structured setup. Below is a simple example of how to create an endpoint in a Java Spring Boot application that allows clients to upload a Blob.
Maven Dependency
Make sure you have the necessary dependencies in your pom.xml
.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Spring Boot Controller for Handling File Upload
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
@RestController
@RequestMapping("/api/files")
public class FileUploadController {
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST)
.body("Please choose a file to upload.");
}
// Process the incoming file
// You could save the file to storage or process it
return ResponseEntity.ok("File uploaded successfully: " + file.getOriginalFilename());
}
}
Why Use This Approach?
The above method efficiently handles uploaded files while ensuring you provide feedback to the user. The use of MultipartFile
simplifies file handling in Spring applications, encapsulating the complexities associated with raw data streams.
JavaScript Code for Uploading a Blob
Once your backend is ready, it is essential to handle file uploads on the client-side using JavaScript. Below is a sample script that creates a Blob and then uploads it.
function uploadFile() {
const inputElement = document.getElementById('fileInput');
const file = inputElement.files[0];
if (!file) {
alert('Please select a file to upload.');
return;
}
const blob = new Blob([file], { type: file.type });
const formData = new FormData();
formData.append('file', blob, file.name);
fetch('/api/files/upload', {
method: 'POST',
body: formData,
})
.then(response => {
if (!response.ok) {
throw new Error('Upload failed');
}
return response.text();
})
.then(data => alert(data))
.catch(error => {
console.error('Error uploading file:', error);
alert('Error uploading file: ' + error.message);
});
}
Why It Works?
This approach ensures the file is treated correctly by creating a Blob from the user-uploaded file. The FormData object is key in sending the Blob to the server, making the process seamless.
Debugging Blob Errors
When you run into Blob-related errors, follow these debugging tips:
-
Check Network Traffic
- Use your browser's DevTools to inspect the network calls made during file uploads. Confirm the request's status and payload.
-
Console Logging
- Log relevant data before sending it to the server. This includes checking the Blob's properties and ensuring the content is as expected.
-
Error Handling
- Implement global error handlers to catch errors occurring during the Blob manipulation or file transfer.
-
Use Try-Catch
- In JavaScript, encapsulate your Blob operations in a try-catch block to catch runtime errors promptly.
-
Refer to Existing Solutions
- Articles like Taming JavaScript Blobs: Fixing Bulk Import Errors provide invaluable insights into managing Blob issues in various scenarios.
A Final Look
Debugging JavaScript Blob Errors in your Java web application can seem daunting at first. However, by understanding common pitfalls, setting up a robust server-side application, and meticulously checking for issues through debugging techniques, you can significantly improve your application's reliability. As developers, keeping up with related articles and practices enhances our knowledge base and allows for better problem-solving.
With this knowledge, you can confidently handle Blobs in your Java applications and reduce errors effectively. Always remember to keep your JavaScript synchronized with your Java backend, ensuring smooth data handling across multiple systems.
Checkout our other articles