Brewing Java: How to Optimize Error Handling in Your App
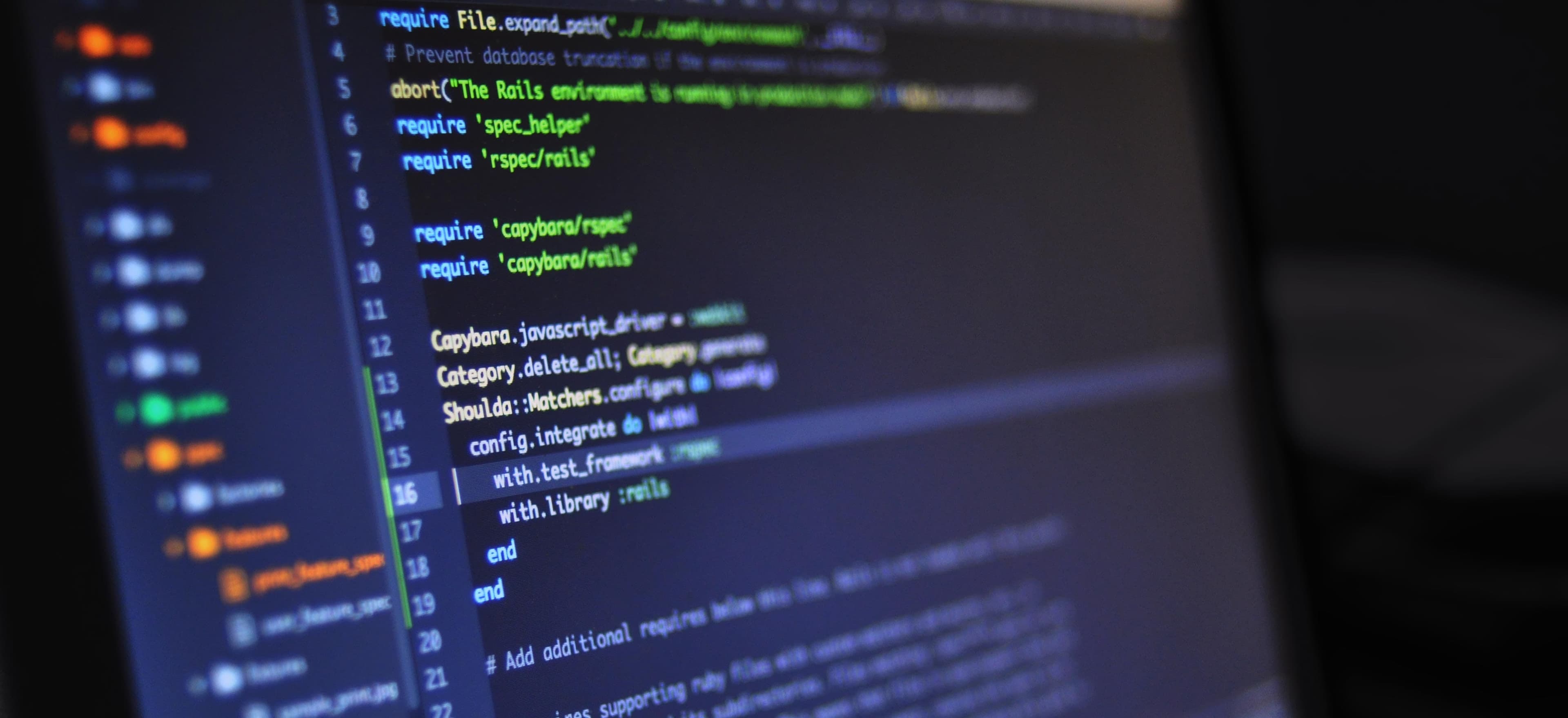
- Published on
Brewing Java: How to Optimize Error Handling in Your App
Error handling is a crucial aspect of software development, especially when working with a robust programming language like Java. Proper error handling can enhance your application's reliability and improve user experience. In this blog post, we'll explore best practices, techniques, and code snippets designed to optimize error handling in Java.
Understanding Java Exceptions
In Java, exceptions are events that disrupt the normal flow of program execution. They are categorized into two main types: checked exceptions and unchecked exceptions.
-
Checked Exceptions: These exceptions are checked at compile-time. They require explicit handling (either caught or declared) in the method signature. Examples include IOException and ClassNotFoundException.
-
Unchecked Exceptions: These occur at runtime and are not required to be declared or caught, such as NullPointerException or ArrayIndexOutOfBoundsException.
Understanding these distinctions is critical in optimizing error handling.
Example of Checked Exception
Here's how you might handle a checked exception:
import java.io.FileReader;
import java.io.IOException;
public class FileReadExample {
public void readFile(String filePath) {
try (FileReader fileReader = new FileReader(filePath)) {
// Reading from the file
int data = fileReader.read();
while(data != -1){
System.out.print((char) data);
data = fileReader.read();
}
} catch (IOException e) {
System.err.println("File not found: " + e.getMessage());
}
}
}
In this example, the try-with-resources
statement is used. This is crucial as it ensures that the FileReader
is closed automatically despite whether the operation succeeds or fails.
Why Use Custom Exceptions?
Creating custom exceptions can help make your code cleaner and improve error handling. When you create a custom exception, it can define specific error scenarios unique to your application.
Example of Custom Exception
public class UserNotFoundException extends Exception {
public UserNotFoundException(String message) {
super(message);
}
}
You can throw this custom exception in your application as follows:
public class UserService {
public User findUserById(String userId) throws UserNotFoundException {
User user = // ... logic to find user
if(user == null) {
throw new UserNotFoundException("User with ID " + userId + " not found.");
}
return user;
}
}
This allows developers to catch specific exceptions, leading to more accurate error handling in code.
Best Practices for Error Handling in Java
-
Catch Specific Exceptions: Rather than catching generic exceptions, limit yourself to catching specific ones. This avoids hiding unexpected issues.
-
Log Errors: Make use of logging frameworks such as Log4j or SLF4J to log errors in detail. This provides visibility into exceptions and can be vital for debugging.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ExampleService {
private static final Logger logger = LoggerFactory.getLogger(ExampleService.class);
public void process() {
try {
// some processing logic
} catch (Exception e) {
logger.error("An error occurred: ", e);
}
}
}
- Utilize Finally Block: Use the
finally
block to perform cleanup tasks that must occur regardless of whether an exception is thrown.
public void manageResource() {
Resource resource = null;
try {
resource = new Resource();
// use resource
} catch (Exception e) {
logger.error("Error managing resource", e);
} finally {
if (resource != null) {
resource.close(); // Ensure resource is closed
}
}
}
-
Never Silence Exceptions: Avoid empty catch blocks. Silencing exceptions can lead to undiagnosed problems and diminished application quality.
-
Use Assertions: Assertions can validate assumptions in your code during development and testing. However, they should not replace proper exception handling.
public void setAge(int age) {
assert age > 0 : "Age must be positive";
this.age = age;
}
Advanced Error Handling Techniques
1. Exception Wrapping
Sometimes, it makes sense to wrap exceptions into higher-level application-specific exceptions, preserving their stack trace while adding additional context.
public class DatabaseException extends RuntimeException {
public DatabaseException(String message, Throwable cause) {
super(message, cause);
}
}
// Usage
try {
// database operation
} catch (SQLException e) {
throw new DatabaseException("Database operation failed", e);
}
2. Global Exception Handling
Implementing a global exception handler can unify your error response strategy, especially in applications with multiple layers.
For instance, in a Spring Boot application, you can use @ControllerAdvice
:
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
@ResponseStatus(HttpStatus.NOT_FOUND)
public String handleUserNotFound(UserNotFoundException ex) {
return ex.getMessage();
}
}
This method centralizes and simplifies error handling across your application.
Bringing It All Together
Effective error handling in Java is not just about catching exceptions; it’s about understanding when and where to manage them. By following best practices like using custom exceptions, logging, and unifying handling strategies, your application can become more robust and reliable.
As a next step, familiarize yourself with best practices and learn how to integrate lightweight solutions to optimize user experience. Whether you're a seasoned developer or just starting, mastering error handling will considerably elevate your Java development skills.
For those seeking natural remedies to combat everyday challenges like headaches, consider checking out the article "Natürliche Hilfe: 5 Teesorten gegen Kopfschmerzen" here. Handling exceptions is much like brewing the perfect cup of tea; both require patience, precision, and the right blend of elements to achieve a desirable result.
Remember, each exception is not a failure but an opportunity to enhance the quality of your application. Happy coding!
Checkout our other articles