Java Solutions for Building a Headache-Free User Interface
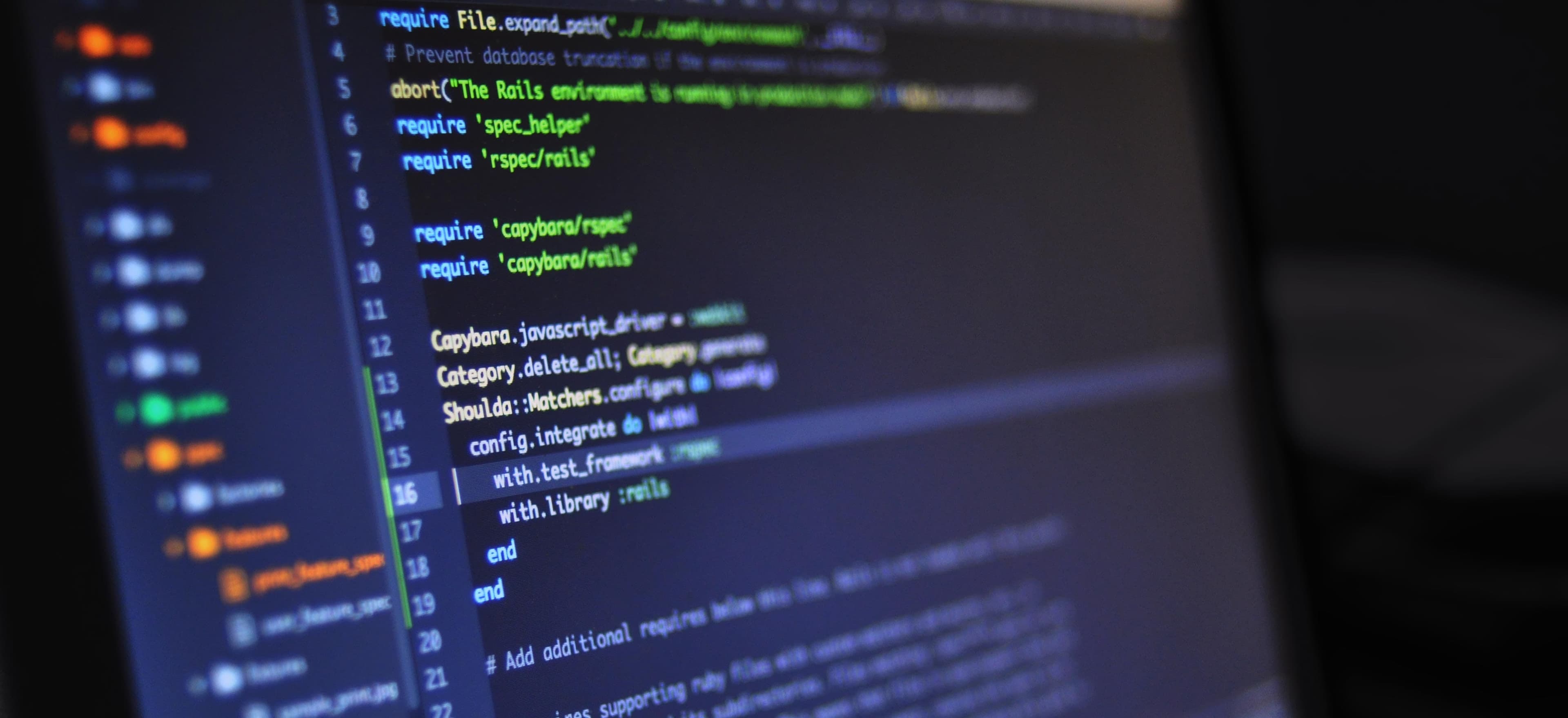
- Published on
Java Solutions for Building a Headache-Free User Interface
Creating a great user interface (UI) is a paramount task when developing any application. The user interface is often the first point of interaction between your users and your application. When it's well-designed, it can lead to higher user satisfaction and a more intuitive experience. However, if it's mismanaged, it can turn enjoyable tasks into frustrating challenges. This article aims to explore various Java solutions and best practices for building an effective, headache-free UI, drawing on principles of usability, design, and simplicity.
Understanding Java’s UI Libraries
When it comes to developing user interfaces in Java, you primarily have three libraries to choose from:
- Swing
- JavaFX
- AWT (Abstract Window Toolkit)
1. Swing: The Robust Choice
Swing is a part of Java Foundation Classes (JFC) and is used for building graphical user interfaces (GUIs). It provides a rich set of widgets for building desktop applications.
Why Choose Swing?
- Lightweight: Unlike AWT, Swing doesn't rely heavily on the operating system’s native user interface, making it platform-independent.
- Rich Components: Swing offers a wide range of customizable components, including buttons, text inputs, lists, and tables.
- Look and Feel: You can customize the "look and feel" of your applications, making them more modern and user-friendly.
Example: Swing Button Implementation
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class HelloWorldApp {
public static void main(String[] args) {
JFrame frame = new JFrame("Hello World");
JButton button = new JButton("Click me!");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JOptionPane.showMessageDialog(frame, "Hello, World!");
}
});
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 200);
frame.getContentPane().add(button);
frame.setVisible(true);
}
}
- Why this code works: Here, we create a simple Swing application with a button that, when clicked, displays a dialog box with the message "Hello, World!" This illustrates the ease of interaction and responsiveness that Swing provides to developers.
2. JavaFX: The Modern Approach
JavaFX is the successor to Swing and offers a more modern approach to UI design. It supports web-like interfaces with the use of FXML and CSS.
Why Choose JavaFX?
- Graphical Capabilities: JavaFX supports advanced graphics, making it ideal for applications requiring rich media.
- CSS Styling: You can use CSS to style JavaFX components, allowing for easier and more flexible design.
- Hardware Acceleration: JavaFX takes advantage of hardware acceleration for better performance.
Example: JavaFX with FXML
<!-- sample.fxml -->
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.layout.VBox?>
<VBox xmlns:fx="http://javafx.com/fxml" alignment="CENTER" spacing="10">
<Button text="Click me!" onAction="#handleButtonClick"/>
</VBox>
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class HelloWorldFX extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
Parent root = FXMLLoader.load(getClass().getResource("sample.fxml"));
primaryStage.setTitle("Hello World FX");
primaryStage.setScene(new Scene(root, 300, 200));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
- Why this code works: In this example, we define a UI layout in FXML and link it to a JavaFX application. The separation of design (FXML) and logic (Java) adheres to modern development practices, making it cleaner and more maintainable.
3. AWT: The Legacy Implementation
AWT is the original UI toolkit that comes with Java. Though less frequently used in modern applications, it is crucial to understand its persistence in legacy systems.
Why Use AWT?
- Basic Components: Good for creating simple GUIs.
- Native Look: AWT directly uses native OS components.
AWT is typically not recommended for new applications due to these limitations; however, appreciating its history helps understand Java's UI evolution.
Best Practices for UI Design in Java
1. Simplicity is Key
Ensure that the user interface is clean, intuitive, and devoid of any unnecessary features. Users should not need to struggle to find what they need.
2. Responsive Design
Ensure that your application is responsive and that all elements are user-friendly. This is especially crucial for applications that might be resized or run on various devices.
3. User Feedback
Always provide feedback for user actions, such as button clicks or form submissions. This can be as simple as showing success messages or errors.
4. Consistency
Consistent design across the application enhances user experience. Use uniform colors, fonts, and button styles throughout your application.
5. Accessibility
Make your application accessible to everyone, including users with disabilities. Use proper labels, keyboard shortcuts, and screen-reader compatibility.
A Final Look
Designing a user-friendly interface in Java requires careful consideration of the tools at your disposal. Whether using Swing, JavaFX, or even AWT, each library offers unique strengths that can be leveraged to enhance the user's experience.
To create a UI that avoids causing headaches for your users, adhere to the best practices outlined above. By fostering an environment of simplicity, responsiveness, and user feedback, you can ensure that users enjoy a seamless interaction with your application.
If you find yourself interested in natural remedies, such as teas that may help alleviate headaches, you can read more in the article "Natürliche Hilfe: 5 Teesorten gegen Kopfschmerzen" at auraglyphs.com/post/natuerliche-hilfe-5-teesorten-gegen-kopfschmerzen
.
By focusing on these principles and the Java solutions discussed, you can craft applications that not only perform well but also offer a headache-free user experience. Happy coding!
Checkout our other articles