Managing Blob Data in Java: Overcoming Bulk Import Challenges
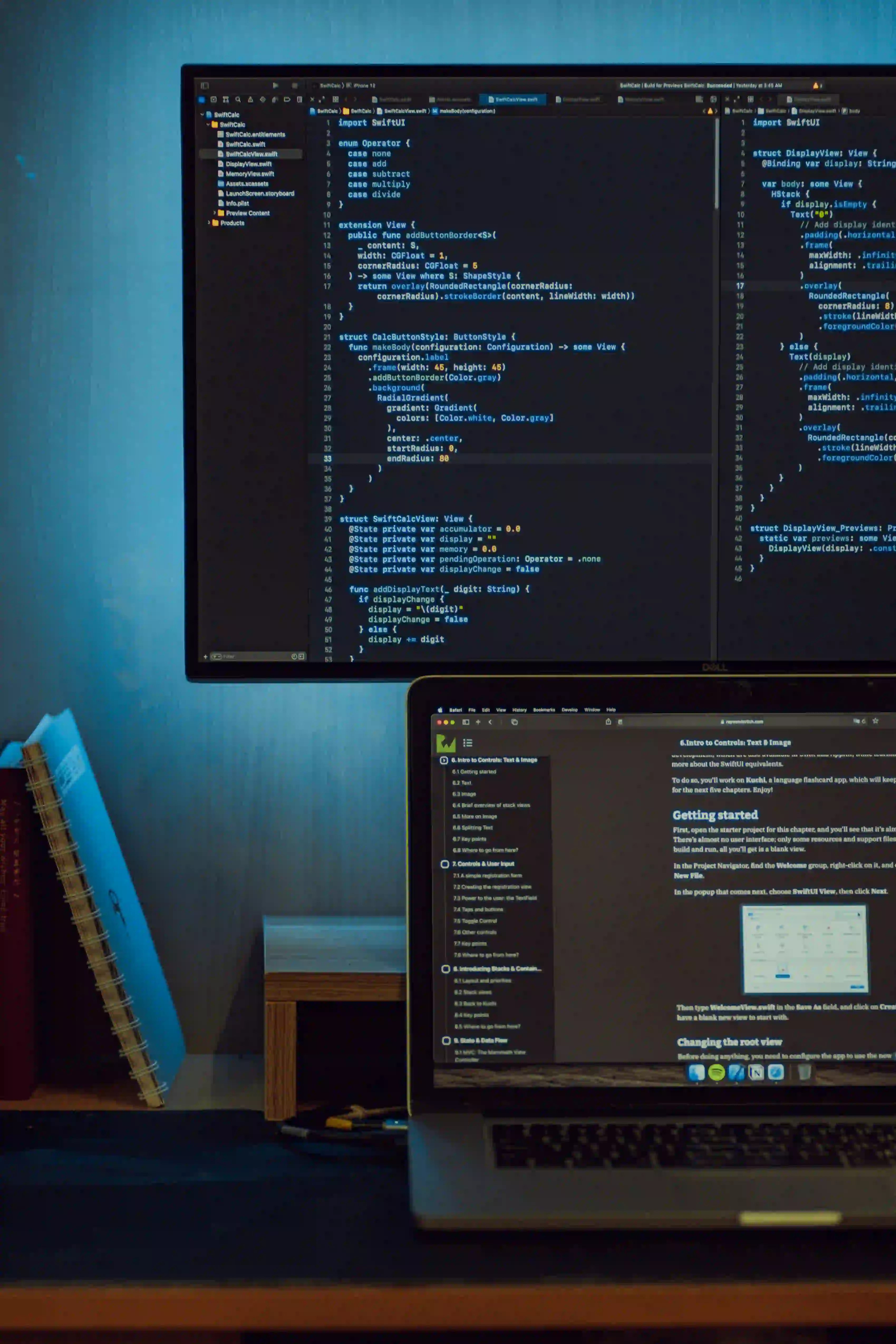
Managing Blob Data in Java: Overcoming Bulk Import Challenges
When it comes to handling large amounts of binary data in Java, specifically with the use of Binary Large Objects (BLOBs), developers often face a myriad of challenges. Whether you are dealing with images, multimedia files, or other large datasets, optimized management of BLOBs can make a significant difference in application performance and user experience. In this blog post, we will explore common issues encountered during bulk imports of BLOB data, offer solutions, and highlight best practices that can help you manage BLOBs efficiently.
Understanding BLOBs and Their Importance
BLOBs are a data type in databases for storing large binary objects. They are essential for applications that require high-volume storage of multimedia data. Unlike regular data types, BLOBs handle binary data such as:
- Images
- Audio files
- Video files
- Documents
Using BLOBs has advantages, such as allowing developers to store large binary data directly in the database and ensuring data integrity. However, when importing large sets of data, particularly in bulk, several issues may arise.
Common Challenges with Bulk Importing BLOBs
-
Performance Bottlenecks: Bulk imports can lead to performance issues, especially when inserting large data volumes into the database. Operations can become slow, causing timeouts or failures.
-
Memory Management: Loading large BLOBs into memory can lead to
OutOfMemoryError
exceptions if not managed correctly. -
Transaction Management: Ensuring data consistency during bulk inserts can be tricky. If one operation fails, it can lead to a partially completed operation, leaving the dataset in an inconsistent state.
By understanding these challenges, developers can create effective strategies to successfully manage BLOB data in Java.
Best Practices for Managing BLOBs
1. Stream BLOB Data to Minimize Memory Usage
When importing large files, avoid loading the entire file into memory. Instead, stream the file data into the database. This method allows the process to manage memory more effectively:
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class BlobImportExample {
// Method to insert BLOB
public void insertBlob(Connection conn, String filePath) throws SQLException {
File file = new File(filePath);
try (InputStream inputStream = new FileInputStream(file)) {
String sql = "INSERT INTO my_table (file_data) VALUES (?)";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setBlob(1, inputStream); // Stream the input instead of reading the whole file
pstmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why stream? Streaming helps to mitigate memory overload during large imports by only processing chunks of data instead of loading everything upfront.
2. Use Batch Processing for Efficient Inserts
Using batch processing commands can significantly improve performance during bulk imports. Instead of executing a single insert repeatedly, group them into batches:
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class BlobBatchInsert {
private static final int BATCH_SIZE = 1000;
public void insertBatchBlobs(Connection conn, List<File> files) throws SQLException {
String sql = "INSERT INTO my_table (file_data) VALUES (?)";
try (PreparedStatement pstmt = conn.prepareStatement(sql)) {
int count = 0;
for (File file : files) {
try (InputStream inputStream = new FileInputStream(file)) {
pstmt.setBlob(1, inputStream);
pstmt.addBatch();
count++;
if (count % BATCH_SIZE == 0) {
pstmt.executeBatch(); // Execute in batch
}
}
}
pstmt.executeBatch(); // Execute remaining items
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why batch processing? Batch processing reduces the number of database roundtrips, improving overall performance during bulk imports.
3. Database Transaction Management
Managing transactions correctly ensures that your data remains consistent. If an error occurs during a batch operation, the database should roll back the transaction:
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class TransactionManagement {
public void insertWithTransaction(Connection conn, List<File> files) throws SQLException {
conn.setAutoCommit(false); // Start transactions
try (PreparedStatement pstmt = conn.prepareStatement("INSERT INTO my_table (file_data) VALUES (?)")) {
for (File file : files) {
try (InputStream inputStream = new FileInputStream(file)) {
pstmt.setBlob(1, inputStream);
pstmt.addBatch();
}
}
pstmt.executeBatch();
conn.commit(); // Commit if all successful
} catch (SQLException e) {
conn.rollback(); // Rollback on error
e.printStackTrace();
} finally {
conn.setAutoCommit(true); // Re-enable auto-commit
}
}
}
Why manage transactions? Transaction management enables safe and atomic operations to ensure data integrity. If something goes wrong, you can roll back any changes.
4. Linkage to Frontend Management
Imagine importing BLOBs through a web frontend that captures the files for the server to process. As addressed in the article "Taming JavaScript Blobs: Fixing Bulk Import Errors" (infinitejs.com/posts/taming-js-blobs-fixing-import-errors), consider how front-end issues can lead to hefty upload errors. Handling client-side validations or chunking files can ease pressure on backend processing.
In Conclusion, Here is What Matters
Efficiently managing BLOBs in a Java application requires an understanding of both the data's nature and the challenges that arise with bulk imports. By employing strategies such as streaming data, batch processing, transaction management, and ensuring frontend reliability, developers can overcome these challenges effectively.
Always prioritize proactive measures to safeguard your application's performance and data integrity. Remember, the way you handle BLOBs not only impacts your application but also shapes the user experience.
For those interested in diving deeper into managing BLOBs and handling related challenges, the referenced article "Taming JavaScript Blobs: Fixing Bulk Import Errors" provides valuable insights that complement this discussion. Feel free to refer to the article for further context and practical advice!