Mastering User Input: Transforming Data into Java Arrays
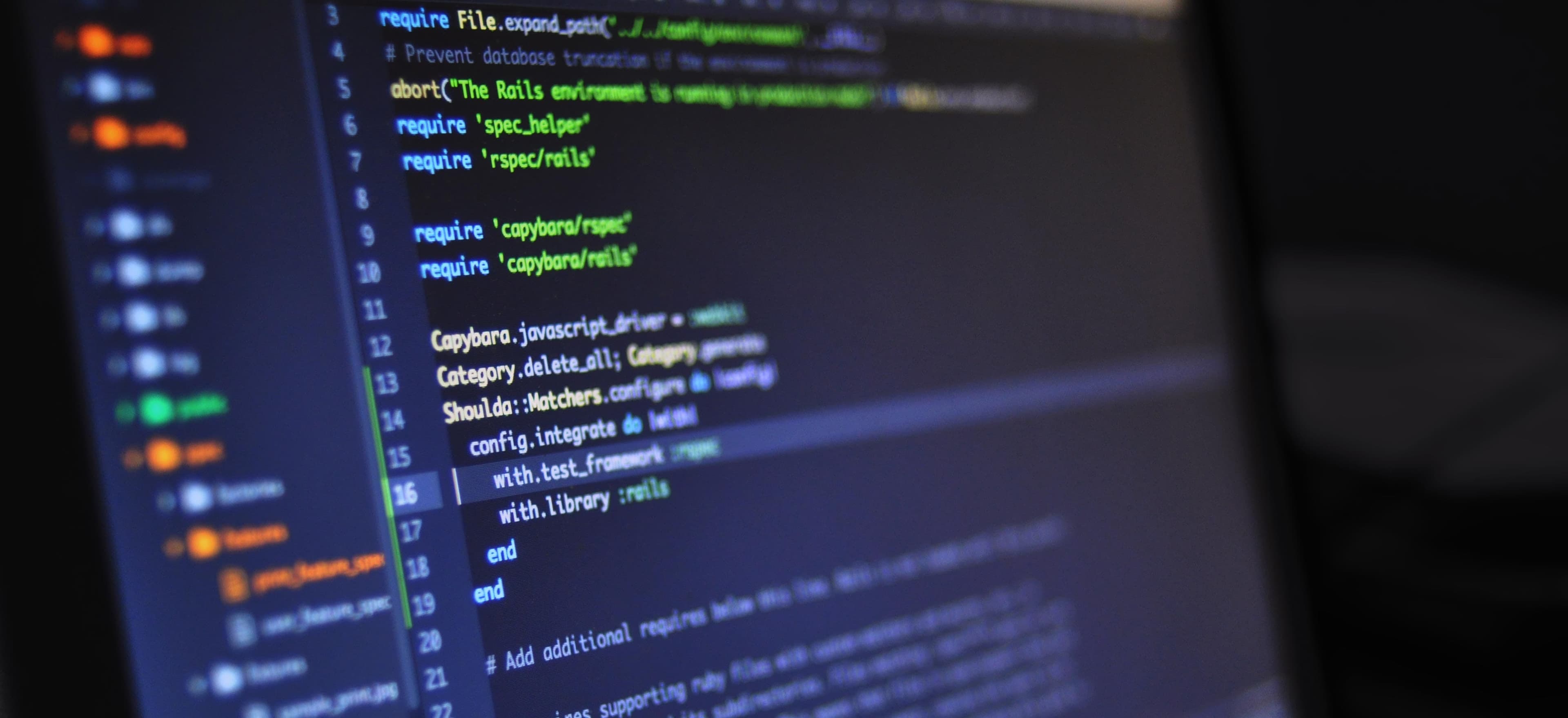
- Published on
Mastering User Input: Transforming Data into Java Arrays
In the world of programming, user input serves as the gateway for interaction between humans and machines. In Java, efficiently managing user input and transforming it into useful data structures is foundational for developing robust applications. One of the most common and versatile data structures in Java is the array. In this article, we will delve into the techniques and best practices for converting user input into arrays in Java, building upon the concepts outlined in Converting User Input to Array: A Step-by-Step Guide.
Understanding User Input in Java
When working with Java, the process of gathering user input typically revolves around the Scanner
class, which is part of the java.util package. This class provides methods to read various types of input, such as strings, integers, and more.
The Scanner Class: An Overview
The Scanner
class is a powerful tool that simplifies input handling. Below is a simple example of how to instantiate a Scanner
object and read user input:
import java.util.Scanner;
public class InputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your name: ");
String userName = scanner.nextLine();
System.out.println("Hello, " + userName + "!");
scanner.close();
}
}
Why Use Scanner
?
The main advantage of using the Scanner
class is its simplicity. It allows for easy parsing of different data types. This promotes clean, readable code while handling user input efficiently.
Converting Input into Arrays
The crux of our discussion today is converting the user input into arrays. This is essential for applications that require data processing, storage, or manipulation.
Step 1: Reading Input
Let's first capture user input in a simple, comma-separated format. For demonstration purposes, we will ask the user to enter several numbers as strings.
import java.util.Scanner;
public class ArrayInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter numbers separated by commas: ");
String input = scanner.nextLine();
scanner.close();
}
}
Why Capture Input Like This?
This format is user-friendly and allows for easy parsing later. Users are accustomed to typing lists in such a manner, making it an intuitive choice.
Step 2: Splitting Input into an Array
Once we have the input string, we can split it into an array using the split()
method.
import java.util.Scanner;
public class ArrayInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter numbers separated by commas: ");
String input = scanner.nextLine();
// Split the input string into an array
String[] inputArray = input.split(",");
scanner.close();
// Print the array to verify the conversion
System.out.println("You entered: ");
for (String number : inputArray) {
System.out.println(number.trim()); // trim() removes excess whitespace
}
}
}
Why Use split()
?
The split()
method is ideal for breaking down strings based on a delimiter, which, in this case, is a comma. This creates an array containing individual string elements that can be processed further.
Step 3: Converting String Array to Integer Array
In some cases, we may need to convert these string values into integers for computational purposes. Here's how to achieve that:
import java.util.Scanner;
public class ArrayInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter numbers separated by commas: ");
String input = scanner.nextLine();
// Split into String array
String[] inputArray = input.split(",");
scanner.close();
// Convert to integer array
int[] numberArray = new int[inputArray.length];
for (int i = 0; i < inputArray.length; i++) {
numberArray[i] = Integer.parseInt(inputArray[i].trim()); // trim white spaces and convert
}
// Display the integer array
System.out.println("You entered the numbers: ");
for (int number : numberArray) {
System.out.println(number);
}
}
}
Why Convert to Integer?
This conversion is essential for performing arithmetic operations or any computations, as the string data type does not support numeric calculations directly.
Best Practices for Handling User Input
When working with user input in Java, following best practices can help in building cleaner and more efficient code. Here are some recommendations:
-
Validate Input: Always validate user inputs before processing them. This prevents exceptions and ensures a smooth user experience.
-
Use Exception Handling: Incorporate error handling to manage unexpected inputs gracefully.
try {
int number = Integer.parseInt(input.trim());
} catch (NumberFormatException e) {
System.out.println("Invalid input! Please enter numbers only.");
}
-
Provide Clear Instructions: Guide your users on how to format their input. This reduces confusion and enhances usability.
-
Close Resources: Always close the
Scanner
or any other I/O resources to prevent memory leaks.
Lessons Learned
Mastering the conversion of user input into Java arrays is a vital skill that enhances your programming capabilities. By effectively utilizing the Scanner class, splitting strings, and converting data types, you can streamline user interactions in your applications.
For a more detailed look at converting user input into arrays, refer to the comprehensive article, Converting User Input to Array: A Step-by-Step Guide.
By implementing these techniques with clarity and consistency, you'll have a fundamental understanding that can be applied across various Java applications, paving the way to more complex data structures such as lists and maps. Happy coding!
Checkout our other articles