Illuminate Your Java Code: Cost-Effective Debugging Tools
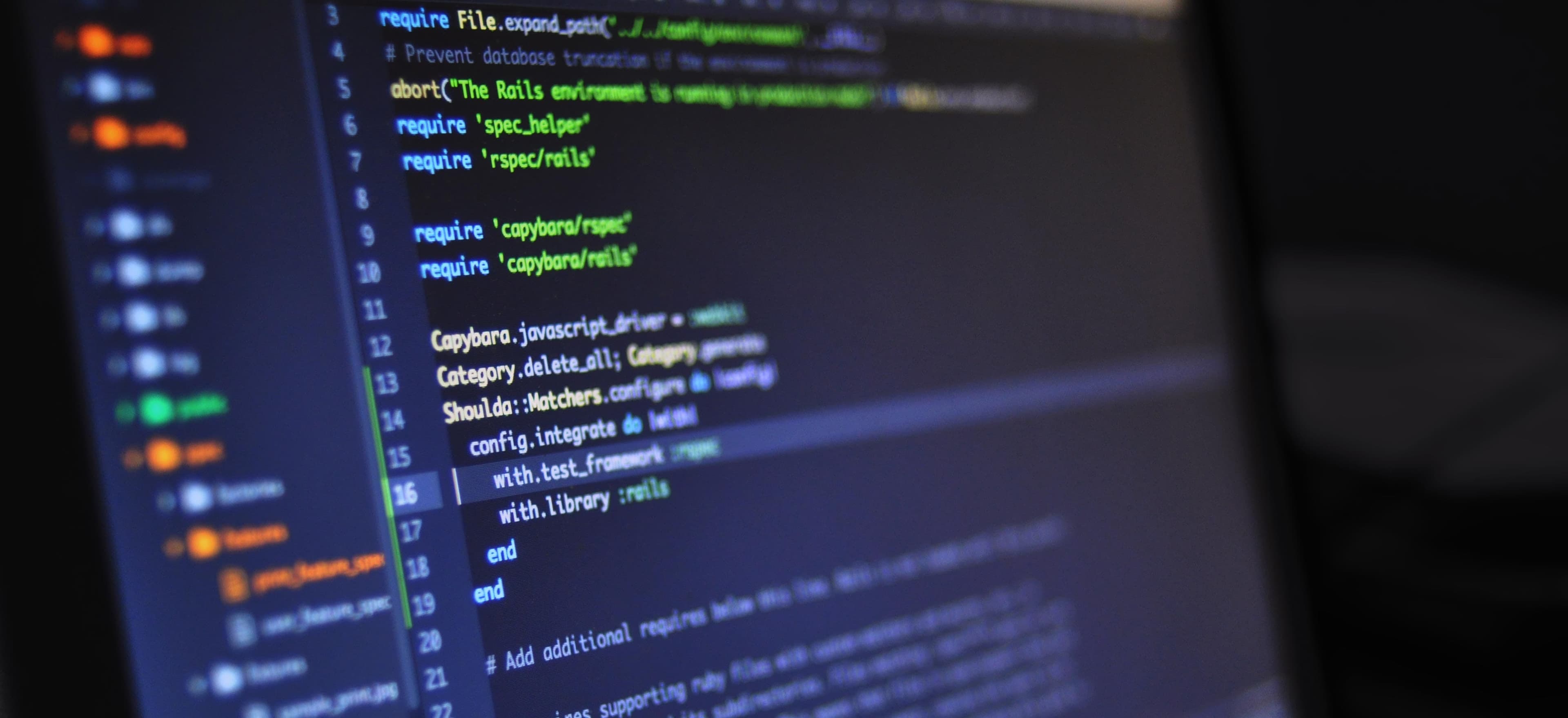
- Published on
Illuminate Your Java Code: Cost-Effective Debugging Tools
Debugging is an essential aspect of software development, particularly for Java developers. It helps identify and fix issues in your code, ensuring smooth operation and performance. However, effective debugging doesn’t always require a hefty budget. In this blog post, we’ll explore cost-effective debugging tools, offering insights and code snippets to improve your debugging process.
Table of Contents
- Understanding the Importance of Debugging
- Essential Features of Debugging Tools
- Cost-Effective Debugging Tools for Java
- Eclipse IDE
- IntelliJ IDEA Community Edition
- Visual Studio Code
- Best Practices in Debugging
- Conclusion
1. Understanding the Importance of Debugging
Debugging involves locating and resolving errors in your code. It is not merely about fixing syntax errors but also addressing logical issues that can lead to unexpected behaviors. Understanding the nuances of debugging can drastically reduce the amount of time spent troubleshooting.
The Cost of Poor Debugging
Poor debugging can lead to software failures, which can be costly in terms of time, resources, and reputation. Investing time in mastering debugging tools equips developers to resolve issues quickly and effectively, enabling faster development cycles and reduced downtime.
2. Essential Features of Debugging Tools
When considering debugging tools, certain features can significantly enhance your debugging experience. These include:
- Breakpoints: Allow developers to pause execution at a specific line to examine the state of the application.
- Step-through Debugging: Enabling developers to execute code line by line, providing an opportunity to observe variable changes.
- Variable Watchlists: Tracking specific variables to monitor changes in real-time.
- Call Stack Examination: Understanding the sequence of method calls leading up to an error.
These features are vital for effective debugging, allowing for a thorough investigation of issues.
3. Cost-Effective Debugging Tools for Java
There are several debugging tools available for Java developers that won’t break the bank. We’ll explore some of the most popular options below.
Eclipse IDE
Eclipse is a robust Integrated Development Environment (IDE) offering a free version. It has a powerful built-in debugger that supports:
- Setting breakpoints
- Monitoring variable changes
- Inspecting the call stack
Example: Using Eclipse to Debug
To set a breakpoint in Eclipse, follow these steps:
- Open your Java file in the editor.
- Double-click in the left margin next to the line where you want to set the breakpoint.
public class Example {
public static void main(String[] args) {
int result = add(5, 10); // Set breakpoint here
System.out.println(result);
}
public static int add(int a, int b) {
return a + b;
}
}
With the breakpoint set, when you run the program in debug mode, execution will pause at the line, allowing you to inspect values and execution flow.
IntelliJ IDEA Community Edition
IntelliJ IDEA's Community Edition is another great option for Java developers. Its debugging features are intuitive and user-friendly. You can set breakpoints, watch expressions, and evaluate code snippets during runtime.
Example: Debugging in IntelliJ IDEA
To debug your Java application:
- In your code, right-click on the left gutter next to the line to set a breakpoint.
- Run your project in debug mode.
public class DebugExample {
public static void main(String[] args) {
User user = new User("John", 25); // Set breakpoint here
System.out.println(user);
}
}
class User {
String name;
int age;
User(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "User{name='" + name + "', age=" + age + '}';
}
}
With IntelliJ, you can inspect the user
object’s properties seamlessly at runtime by hovering over the variable in the editor.
Visual Studio Code
Visual Studio Code (VS Code) may not be a traditional option for Java development but offers extensions that support Java programming, including debugging features. Its lightweight nature makes it a suitable choice for many developers.
Example: Debugging in Visual Studio Code
To debug a Java application in VS Code:
- Install Java Extension Pack.
- Click on the left panel to navigate to the Debug tab and configure your launch settings.
public class VscodeDebugExample {
public static void main(String[] args) {
int total = calculateTotal(10, 20); // Set breakpoint here
System.out.println("Total: " + total);
}
static int calculateTotal(int a, int b) {
return a + b;
}
}
Adding a breakpoint allows you to monitor the execution and validate your calculations closely.
4. Best Practices in Debugging
While tools are important, understanding some best practices can tremendously enhance your debugging efficiency:
- Reproduce the Issue: Ensure you can reliably reproduce the problem to study it in-depth.
- Simplify the Problem: Strip down your code to the minimal version that still reproduces the issue.
- Read Error Messages: Often, messages can guide you directly to the source of the problem.
- Consult Documentation: Familiarize yourself with documentation related to libraries and APIs being used.
5. Conclusion
In conclusion, debugging is a vital aspect of Java development, and using cost-effective debugging tools can greatly enhance your productivity without emptying your wallet. Tools like Eclipse, IntelliJ IDEA Community Edition, and Visual Studio Code provide robust debugging features that can significantly streamline troubleshooting.
For more insights on related topics, you can check out our article on Choosing the Right EDC Flashlight on a Budget at youvswild.com/blog/choosing-edc-flashlight-budget.
Mastering these tools and techniques will not only improve your coding experience but also result in cleaner, more efficient code. Happy debugging!
Checkout our other articles