Illuminate Your Code: Essential Tips for Java Debugging Tools
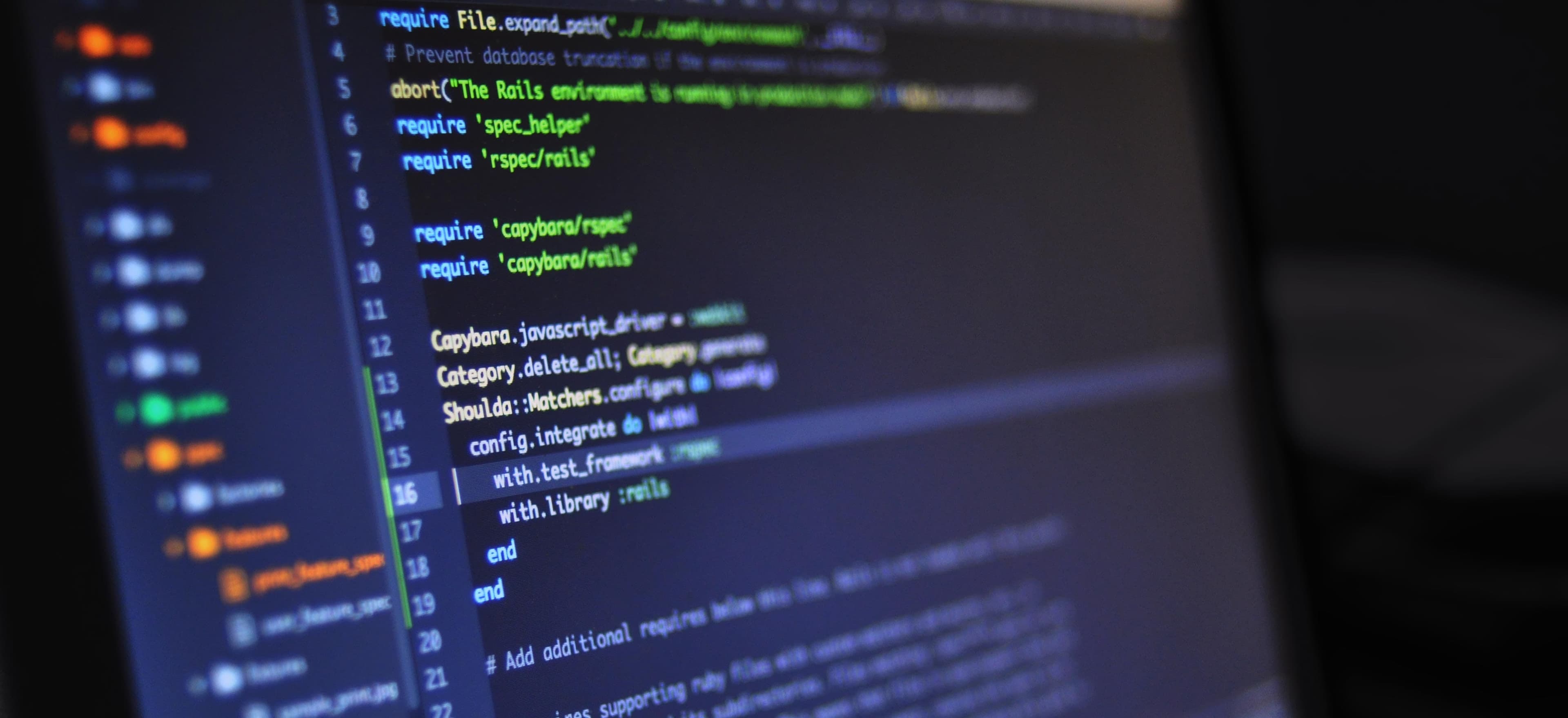
- Published on
Illuminate Your Code: Essential Tips for Java Debugging Tools
Java programming is both an art and a science. As developers, we constantly strive for flawless code, but the reality is that bugs and errors are a natural part of the development process. In fact, debugging is often more than just fixing problems; it’s a critical skill that can elevate both your coding and overall software quality. In this blog post, we will discuss some essential tips for using Java debugging tools and techniques effectively, all while ensuring your Java applications run smoothly.
The Importance of Debugging
Before diving into the tools of the trade, it's crucial to understand why debugging is such a significant part of software development. It helps identify and resolve issues, improves code quality, and ultimately leads to a better user experience. Debugging can feel daunting, but the right tools and mindset can make this process manageable.
Getting Started with Java Debugging Tools
Java offers a variety of debugging tools that can streamline the process of finding and fixing bugs. Here are some popular options:
- Java Debugger (JDB): The command-line debugger provided with the Java Development Kit (JDK). It's lightweight and great for quick debugging tasks.
- Integrated Development Environment (IDE) Debuggers: Modern IDEs like IntelliJ IDEA, Eclipse, and NetBeans come with built-in debugging capabilities, providing a more user-friendly experience.
- Logging Frameworks: Utilizing logging libraries like Log4j, SLF4J, and JUL (Java Util Logging) helps track down issues by providing log statements throughout your code.
Let's take a closer look at how to effectively use some of these tools, starting with the Java Debugger (JDB).
Using the Java Debugger (JDB)
JDB is a simple yet powerful tool. To start debugging with JDB, follow these steps:
-
Compile Your Java Program: First, ensure that your Java file is compiled with the
-g
option to include debugging information.javac -g MyProgram.java
-
Start JDB: Begin the debugger by running the following command:
jdb MyProgram
-
Set Breakpoints: Using the JDB command
stop at
, you can set breakpoints at specific lines to pause execution.stop at MyProgram:10
-
Run the Program: Execute the program within JDB and pause at the defined breakpoints.
run
-
Step Through the Code: Use commands such as
next
,step
, orprint
to inspect variables and control flow.
These simple commands can greatly enhance your debugging experience. The ability to pause execution at key points and inspect variable states is crucial for diagnosing problems.
IDE Debugging
While JDB is effective, many developers prefer IDE debuggers due to their user interface and advanced features. Here's how to effectively use an IDE debugger:
-
Run in Debug Mode: Most IDEs allow you to run your program in debug mode. Look for a 'Debug' button in the IDE's toolbar.
-
Set Breakpoints Graphically: Simply click on the margin next to the line numbers to set breakpoints.
-
Variable Inspection: During execution, hover over variables to view their values. This instant feedback is invaluable.
-
Watch Expressions: Use the 'Watch' feature to keep tabs on specific variables even when they go out of scope.
-
Evaluating Expressions: Evaluate expressions on the fly to test behavior without modifying the code. This can be done in the debug console.
An Example of Effective Debugging
Let’s look at an example that illustrates debugging in action:
public class Example {
public static void main(String[] args) {
int result = divide(10, 0);
System.out.println("Result: " + result);
}
public static int divide(int a, int b) {
return a / b;
}
}
In the above code, trying to divide by zero will lead to an ArithmeticException
. To debug this, you can:
- Set a Breakpoint: On the
return a / b;
line. - Run the Code: When the breakpoint is hit, inspect the values of
a
andb
. - Evaluate Different Scenarios: Before running the program, check potential inputs that could cause issues.
Optimizing Your Debugging Strategy
No two bugs are alike, and the strategies you employ should be tailored to your circumstances. Here are a few tips to optimize your debugging process:
- Master the Tools: Invest time in understanding your debugger and its features. The advanced capabilities can save you considerable time.
- Incremental Development: Build your programs incrementally. This approach allows you to test smaller chunks of code, making it easier to spot and fix bugs.
- Stay Organized: Utilize comments and clean coding practices to make your code easier to read. This simplification helps isolate issues.
Logging as a Debugging Strategy
Logging frameworks play a vital role in debugging by recording application behavior over time. Here’s how to effectively use logging:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class MyApp {
private static final Logger logger = LogManager.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("Application starting...");
try {
int result = divide(10, 0);
logger.info("Result: " + result);
} catch (ArithmeticException e) {
logger.error("Divide by zero error: " + e.getMessage());
}
logger.info("Application finished.");
}
public static int divide(int a, int b) {
return a / b;
}
}
In this example, logging provides valuable insights into the application's behavior and helps identify where exceptions occur. By hierarchically logging information (info, error, etc.), you can effectively track the flow and catch issues before they escalate.
For deeper insights into budgeting your development tools, check out the article, "Choosing the Right EDC Flashlight on a Budget" at youvswild.com/blog/choosing-edc-flashlight-budget.
Wrapping Up
Debugging is more than just a necessary evil; it’s an opportunity to improve your coding skills, enhance your software quality, and improve the overall user experience. By mastering tools like JDB and IDE debuggers, and by effectively leveraging logging frameworks, you can transform the debugging process from a frustrating chore into an enlightening experience.
In conclusion, embrace debugging as an essential part of your development journey, and make the most of the tools at your disposal. After all, every bug is just a stepping stone to becoming a better Java developer.
Happy coding!
Checkout our other articles