Avoiding Common Mistakes in Java Automation with Ansible
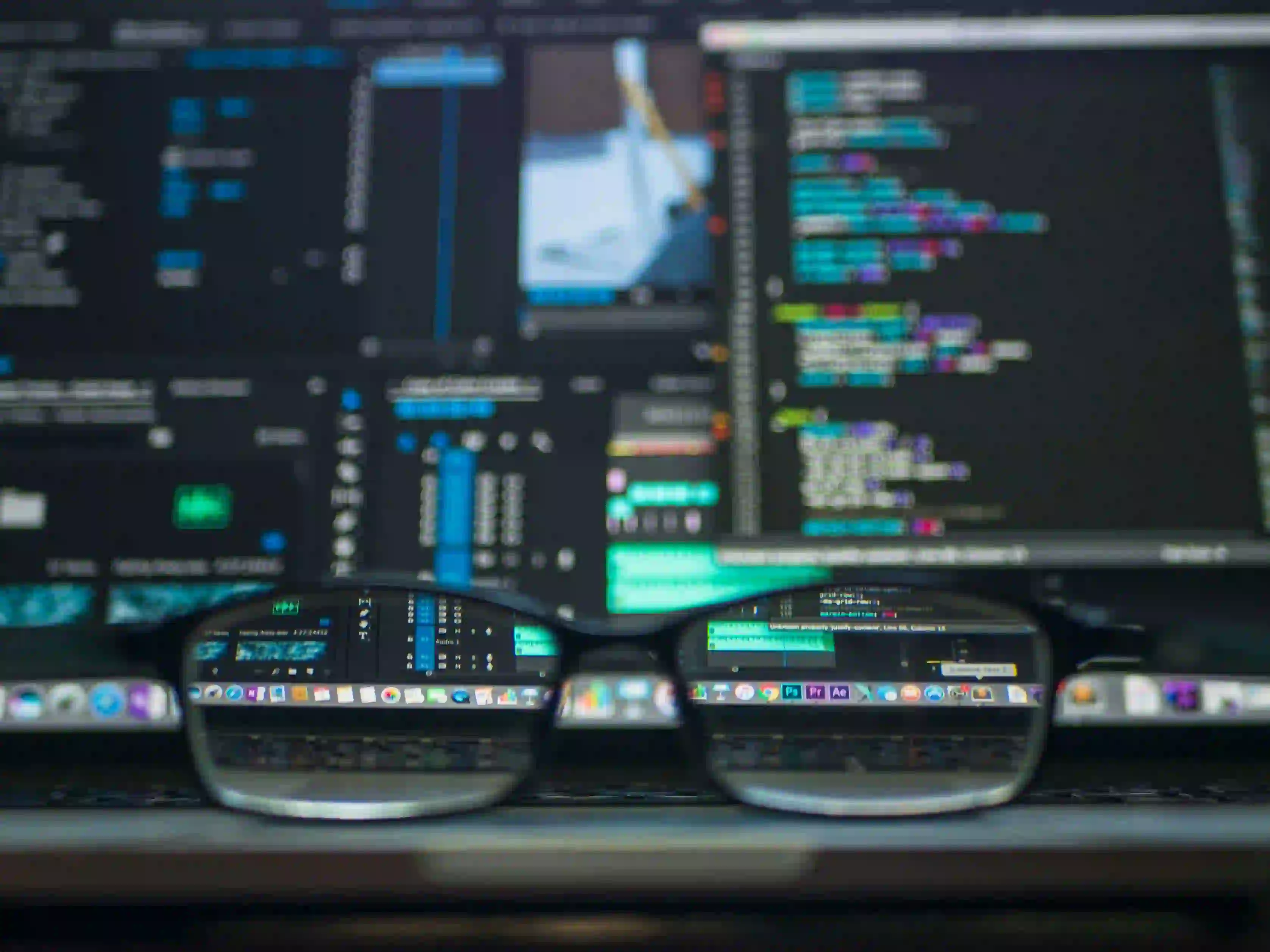
Avoiding Common Mistakes in Java Automation with Ansible
Automating Java applications with Ansible can significantly streamline deployments, improve consistency, and reduce errors in production environments. However, many developers run into pitfalls as they navigate the intricacies of both Java and Ansible. In this blog post, we will explore common mistakes when automating Java applications with Ansible, providing actionable insights and showcasing best practices.
Why Choose Ansible for Java Automation?
Ansible is a robust tool for configuration management and automation, leveraging a simple YAML-based language to define processes. Here are some compelling reasons to use Ansible for Java automation:
- Agentless Architecture: Ansible requires no installation of agents on managed hosts, simplifying management across multiple servers.
- Idempotency: Ensure that your Ansible playbooks can be run multiple times with the same outcome, avoiding unintended changes.
- Rich Ecosystem: Ansible has an extensive collection of modules and integrations for Java applications.
Common Mistakes to Avoid
1. Neglecting Dependency Management
One frequent oversight is not handling Java dependency management effectively. Java projects often rely on external libraries (e.g., Maven libraries). Not including these dependencies in your Ansible playbook can lead to a failed deployment.
Best Practice: Use Maven with Ansible
To ensure dependencies are downloaded and managed appropriately, integrate Maven into your Ansible playbooks.
- name: Ensure Maven is installed
apt:
name: maven
state: present
- name: Build Java project with Maven
command: mvn clean install
args:
chdir: /path/to/java/project
Why? The command
module executes the Maven build directly, which facilitates dependency resolution. Always ensure that the chdir
is correctly set to the location of your pom.xml
file.
2. Not Using Version Control for Playbooks
Ignoring version control for your Ansible playbooks can lead to confusion, especially in collaborative environments. Without a VCS, reverting to a previous configuration becomes arduous.
Best Practice: Use Git for Version Control
Start a Git repository for your Ansible playbooks.
git init ansible-playbooks
cd ansible-playbooks
touch maven-build.yml
Why? Version control ensures that any changes can be tracked and reverted if necessary, providing a historical context that prevents loss of important configurations.
3. Overcomplicating Playbooks
Simplicity should be the guiding principle when crafting your playbooks. Overcomplicated playbooks can lead to errors and confusion.
Best Practice: Split Playbooks into Roles
By using Ansible roles, you can create modular playbooks.
ansible-galaxy init java_project
In the java_project
role, separate tasks can be organized into distinct files, such as installing dependencies, building the project, and deploying it.
Why? Modular roles enhance reusability and understandability, making troubleshooting easier.
4. Skipping Error Handling
Ignoring error handling may lead to silent failures during the deployment process. Ansible has built-in mechanisms to handle errors.
Best Practice: Use ignore_errors
You can specify where to ignore errors while also logging them for further analysis. This can be especially useful in multi-task plays.
- name: Build Java project with Maven
command: mvn clean install
args:
chdir: /path/to/java/project
ignore_errors: yes
Why? This approach allows other tasks to execute even in the face of failures, giving you room to gather additional context without halting the entire playbook execution.
5. Forgetting About Configuration Files
Hardcoding configuration parameters in your playbooks can create issues when deploying to different environments.
Best Practice: Use Variables
Using Ansible's variables allows you to define environment-specific configurations.
- name: Set up application configuration
template:
src: app_config.j2
dest: /etc/myapp/config.yml
vars:
db_host: "{{ lookup('env', 'DB_HOST') }}"
Why? Utilizing templates and variables enables flexible configurations that adapt to various environments without modifying the core playbook logic.
6. Lack of Documentation
Failing to document your playbooks can hinder collaboration and make it difficult for team members to understand the automation processes you've set up.
Best Practice: Comment Your Code
Adding comments and maintaining a README file within your repository can clarify the purpose of each playbook and set of roles.
# This playbook installs and configures our Java application
- hosts: all
tasks:
# Ensure Maven is installed
...
Why? Comments and documentation serve as a road map for others—facilitating onboarding and troubleshooting.
7. Ignoring Testing and Validation
Finally, skipping tests in your automation routine can lead to issues in production.
Best Practice: Use Test-Driven Development (TDD)
Integrate TDD with your Ansible playbooks.
ansible-playbook -i inventory test.yml
This allows for testing Ansible roles and playbooks.
Why? Validating configurations before production deployment minimizes the risk of errors impacting your applications.
Lessons Learned
When automating Java applications with Ansible, consider avoiding these common mistakes. By embracing best practices related to dependency management, version control, simplicity, error handling, configuration flexibility, documentation, and testing, you will set yourself up for a successful automation experience.
For those interested in further improving your automation skills, check out "Common Pitfalls When Writing Your First Ansible Playbook" at configzen.com/blog/common-pitfalls-first-ansible-playbook.
With the right strategies, Ansible can simplify and enhance your deployment processes, making every release smoother and more efficient. Happy automating!