Handling User Input: Efficiently Convert to Java Arrays
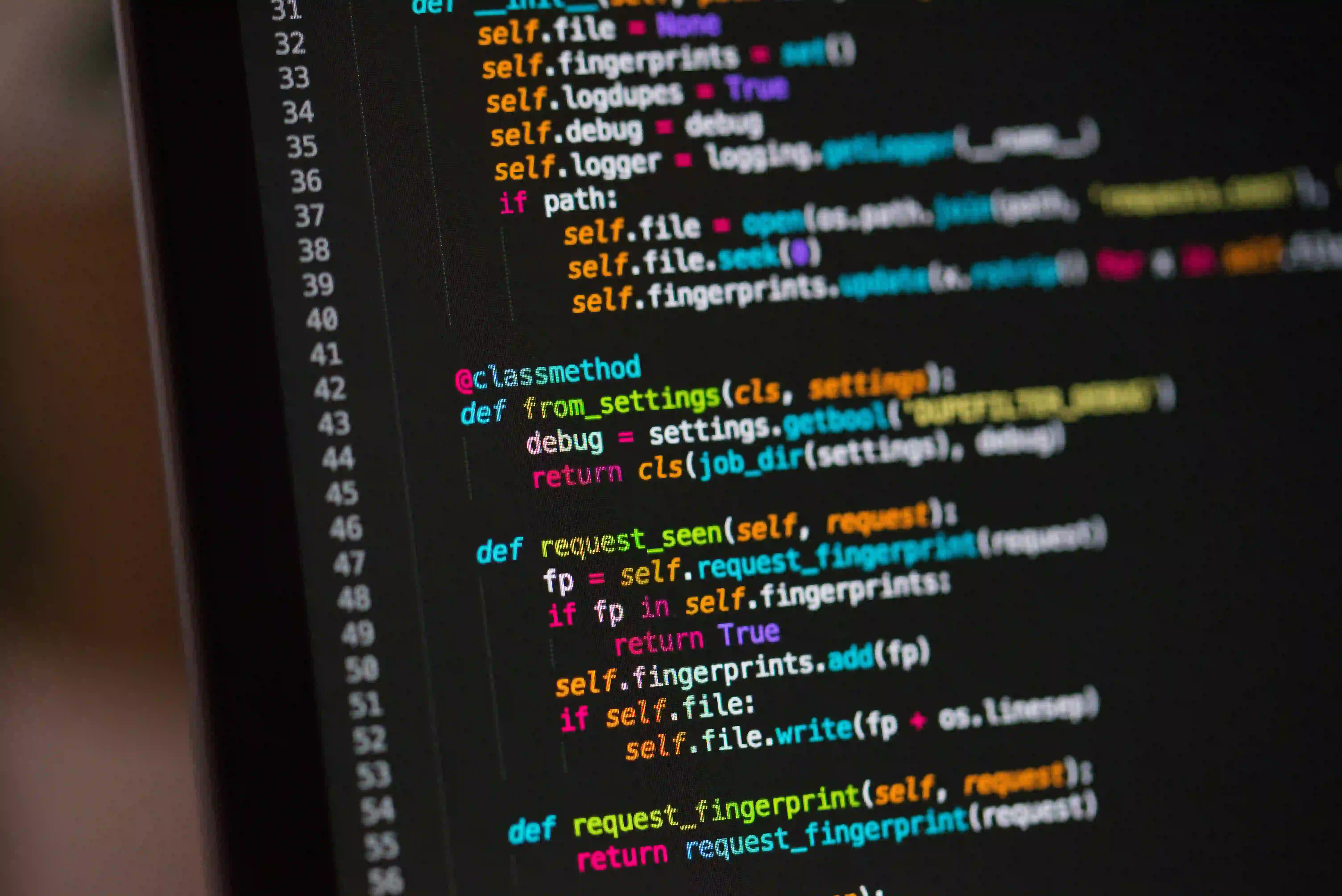
Handling User Input: Efficiently Convert to Java Arrays
In today’s digital landscape, applications increasingly rely on user input to tailor experiences and deliver meaningful content. One fundamental aspect of managing user input is converting it into a format that can be easily processed by our programs. In Java, arrays serve as a foundational data structure for storing data. In this post, we'll explore efficient methods to convert user input into Java arrays, ensuring optimal performance and usability.
To enhance the learning experience, this article will reference the existing resource, Converting User Input to Array: A Step-by-Step Guide. This guide discusses the nuances of converting user input into arrays in Java, providing a solid foundation for what we will cover here.
Understanding Arrays in Java
An array in Java is a collection of items stored at contiguous memory locations. This feature makes accessing elements efficient, as you can retrieve any element with minimal overhead.
Here's a quick rundown of why arrays are crucial in Java:
- Fixed Size: Arrays have a fixed size. When you create an array, you specify how many elements it will hold.
- Efficient Access: Since arrays use contiguous memory, accessing an element by its index is fast.
- Type-Safety: Java is statically typed, which means the type of an array is determined at compile-time, improving safety.
Given these advantages, you need to be able to efficiently convert user input into arrays.
Getting User Input
The first step in our task is gathering user input. In Java, the Scanner class is typically used for this purpose due to its flexibility and ease of use. Here's a basic implementation:
import java.util.Scanner;
public class UserInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter numbers separated by spaces:");
String input = scanner.nextLine();
// Process input
String[] inputArray = input.split(" ");
// Output the result
for (String number : inputArray) {
System.out.println(number);
}
scanner.close();
}
}
Commentary on the Code
- Scanner Class: This built-in class allows you to read input from various sources, including console input. Using
scanner.nextLine()
, we can read an entire line of user input. - String Splitting: The
input.split(" ")
method splits the input string wherever there's a space, returning an array of string elements. This simple yet powerful method empowers us to manipulate user input effectively.
Converting String Input to an Integer Array
Once we've captured the input, data conversion often becomes necessary—typically from strings to integers. This is essential when we want to perform numerical operations on the input. Here’s an example of converting a string array to an integer array:
public class UserInputIntegerArray {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter numbers separated by spaces:");
String input = scanner.nextLine();
// Process input
String[] inputArray = input.split(" ");
int[] numbers = new int[inputArray.length];
for (int i = 0; i < inputArray.length; i++) {
numbers[i] = Integer.parseInt(inputArray[i]); // Convert each String to Integer
}
// Output the result
for (int number : numbers) {
System.out.println(number);
}
scanner.close();
}
}
Commentary on the Conversion
-
Integer Conversion: The line
numbers[i] = Integer.parseInt(inputArray[i])
is pivotal. It converts each String in the input array to an integer, leveraging Java’s built-inInteger
class. -
Error Handling: In a real-world scenario, you should include error handling here. If the user inputs non-numeric data, the program will throw a
NumberFormatException
. We can address this using atry-catch
block, enhancing robustness.
Enhancing User Experience with Error Handling
To make our input handling more resilient, let’s incorporate error handling:
public class UserInputIntegerArrayWithErrorHandling {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter numbers separated by spaces:");
String input = scanner.nextLine();
// Process input
String[] inputArray = input.split(" ");
int[] numbers = new int[inputArray.length];
for (int i = 0; i < inputArray.length; i++) {
try {
numbers[i] = Integer.parseInt(inputArray[i]);
} catch (NumberFormatException e) {
System.out.println("Invalid input: " + inputArray[i]);
numbers[i] = 0; // Assign a default value or use another form of error handling
}
}
// Output the result
for (int number : numbers) {
System.out.println(number);
}
scanner.close();
}
}
Commentary on Error Handling
-
Try-Catch Block: Encapsulating the
Integer.parseInt()
method in a try-catch block allows us to gracefully handle exceptions. Instead of crashing the program, we inform the user of the invalid input and continue processing. -
Default Value: Here, we assigned a default value (0) for erroneous inputs. Depending on the application requirements, you could alter this behavior—perhaps prompting for input again or skipping the value entirely.
Dynamic Arrays: Using ArrayLists
While traditional arrays have their advantages, they come with limitations—most notably, their fixed size. To address this, Java provides the ArrayList
class, which allows for dynamic sizing and better handling of user input.
Here’s how you can implement user input with ArrayList
:
import java.util.ArrayList;
import java.util.Scanner;
public class UserInputWithArrayList {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
ArrayList<Integer> numbersList = new ArrayList<>();
System.out.println("Enter numbers separated by spaces (type 'done' to finish):");
// Continuously gather input
while (true) {
String input = scanner.nextLine();
if (input.equalsIgnoreCase("done")) {
break;
}
String[] inputArray = input.split(" ");
for (String value : inputArray) {
try {
numbersList.add(Integer.parseInt(value));
} catch (NumberFormatException e) {
System.out.println("Invalid input: " + value);
}
}
}
// Output the result
for (int number : numbersList) {
System.out.println(number);
}
scanner.close();
}
}
Commentary on Using ArrayLists
-
Dynamic Sizing: The
ArrayList
can grow as needed. This feature is particularly useful for collecting an unknown number of inputs from the user. -
Loop for Continuous Input: The while-loop allows the user to keep entering numbers until they type "done". This pattern effectively gathers all data while managing input flexibility.
Bringing It All Together
Converting user input to arrays in Java is an essential skill for developers. By using the basic Scanner
for input, handling conversions, and implementing error handling, we can create robust applications capable of processing user data efficiently. If you want to deepen your understanding of this process further, I highly recommend checking out the Converting User Input to Array: A Step-by-Step Guide.
Through effective use of Java’s features, we can ensure an engaging user experience, marrying usability with efficiency. As your applications evolve, understand that proper input handling can significantly affect performance and user satisfaction. So go ahead and implement these practices into your Java programming endeavors!