Integrating Product Names in Java Image Processing
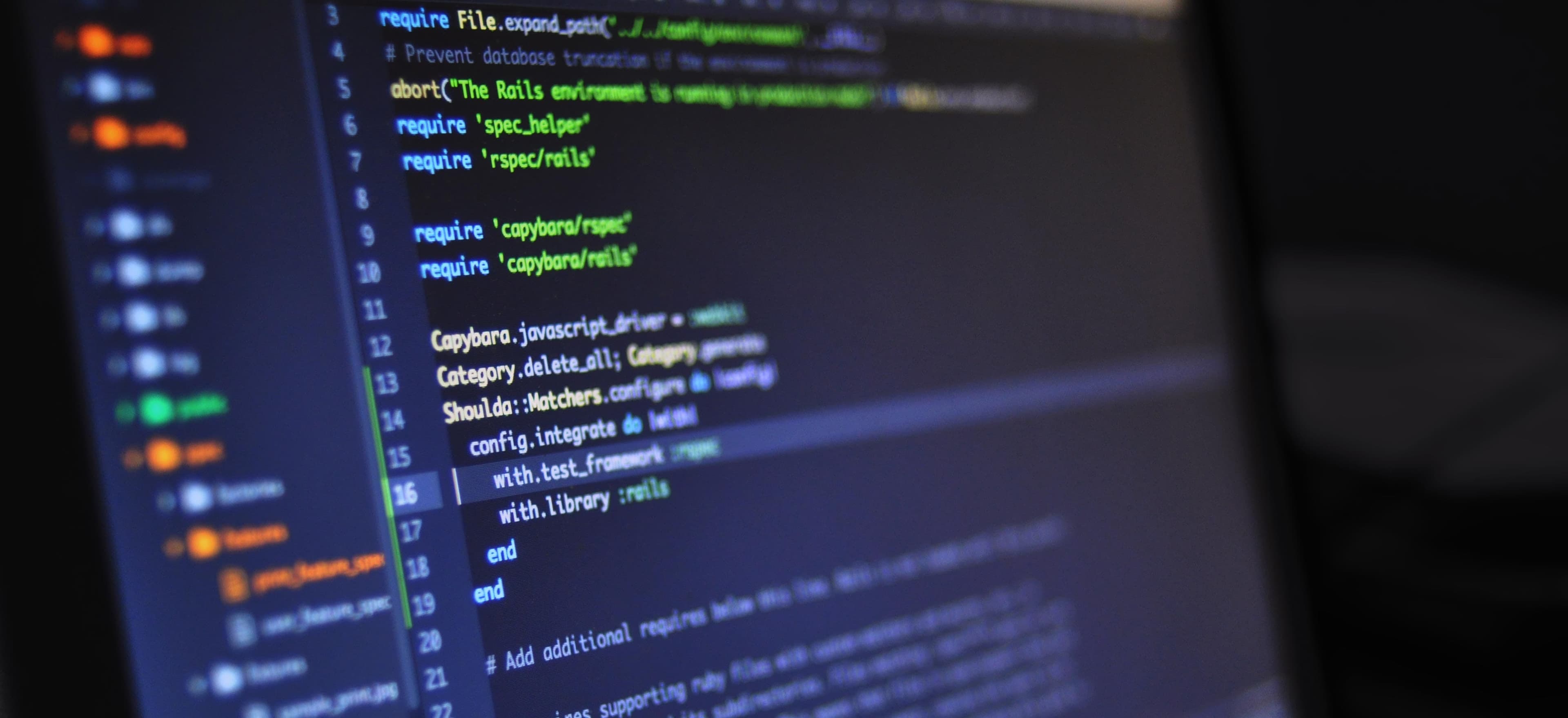
- Published on
Integrating Product Names in Java Image Processing
In today’s fast-paced online retail environment, the user experience hinges significantly on visual elements. One effective method to enhance this experience is by incorporating product names directly into images. By doing so, not only do you provide clarity and context for potential buyers, but you also improve SEO tactics related to image content.
In this blog post, we will explore how to use Java for image processing to add product names to images. We will touch on the necessary libraries, walk through some essential code snippets, and discuss why proper integration is crucial for your eCommerce strategy.
Why Add Product Names to Images?
Adding product names directly to images brings several key benefits:
- Visual Clarity: Customers can quickly identify products without having to read long descriptions.
- SEO Benefits: Search engines index text within images, improving discoverability.
- Brand Recognition: Consistent branding with product names can enhance memorability and loyalty.
For a comprehensive guide on how to effectively add product names to images, check out this existing article.
Java Image Processing Libraries
To proceed with our task, we will use Java Image Processing and Graphics libraries. A popular choice for this is the Java 2D API, which provides robust capabilities for image manipulation. We will also utilize the java.awt
package, which helps in rendering graphics.
Prerequisites
Before diving into coding, ensure you have:
- Java Development Kit (JDK) installed.
- An IDE setup (Eclipse, IntelliJ, etc.).
- The required libraries if using alternatives like Apache Commons Imaging.
Basic Workflow to Add Product Names to Images
Here’s an overview of the steps involved in this process:
- Load the image.
- Create a graphics context to draw on the image.
- Set the font and color for the text.
- Draw the product name on the image.
- Save or display the modified image.
Code Example
Let’s dive into some example code demonstrating how to add a product name to an image in Java:
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class ImageProcessor {
public static void main(String[] args) {
String sourceImagePath = "path/to/original/image.jpg";
String destinationImagePath = "path/to/modified/image.jpg";
String productName = "Product XYZ";
try {
// Load the image
BufferedImage image = ImageIO.read(new File(sourceImagePath));
// Add product name to the image
addProductName(image, productName);
// Save the modified image
ImageIO.write(image, "jpg", new File(destinationImagePath));
System.out.println("Product name added to image successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
private static void addProductName(BufferedImage image, String productName) {
Graphics2D g2d = image.createGraphics();
// Set font and color
Font font = new Font("Arial", Font.BOLD, 40);
g2d.setFont(font);
g2d.setColor(Color.WHITE);
// Set anti-aliasing for smoother text
g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
// Draw the string at the specified coordinates
int x = 50; // x coordinate
int y = 50; // y coordinate
g2d.drawString(productName, x, y);
// Dispose of the graphics context
g2d.dispose();
}
}
Breakdown of the Code
- Loading the Image: The
ImageIO.read
method loads the image from the specified path. - Creating a Graphics Context: The
createGraphics
method allows us to draw on the image. - Setting Font and Color: The choice of
Arial
in bold and white color ensures readability. This is critical, especially if the background is complex. - Anti-Aliasing: Improved text rendering will make your product name visually appealing.
- Coordinate Placement: Adjust
x
andy
values to position the product name as per your design preferences. - Saving the Image: The modified image is saved back to a specified location using
ImageIO.write
.
Additional Considerations
Image Format
While saving images, the choice of format matters. Each format has its pros and cons. JPEGs are generally for photographs, while PNGs support transparency, which is useful for overlaying text.
Testing with Various Images
Diverse images can have different colors and complexities. Always test with multiple product images to ensure that the text remains readable across various backgrounds. You can automate this testing process using unit tests in Java.
A Final Look
By integrating product names directly into images, you can significantly enhance user experience and improve your SEO efforts. The approach is straightforward with Java’s robust capabilities for image processing.
However, remember to consider visual design principles, readability, and branding consistency. For a deeper dive into how to effectively add product names to images, refer to this article.
By mastering these techniques, you'll position your eCommerce business for success in the competitive digital marketplace. Happy coding!
Checkout our other articles