Unlocking the Astral Body's Mysteries with Java Integrations
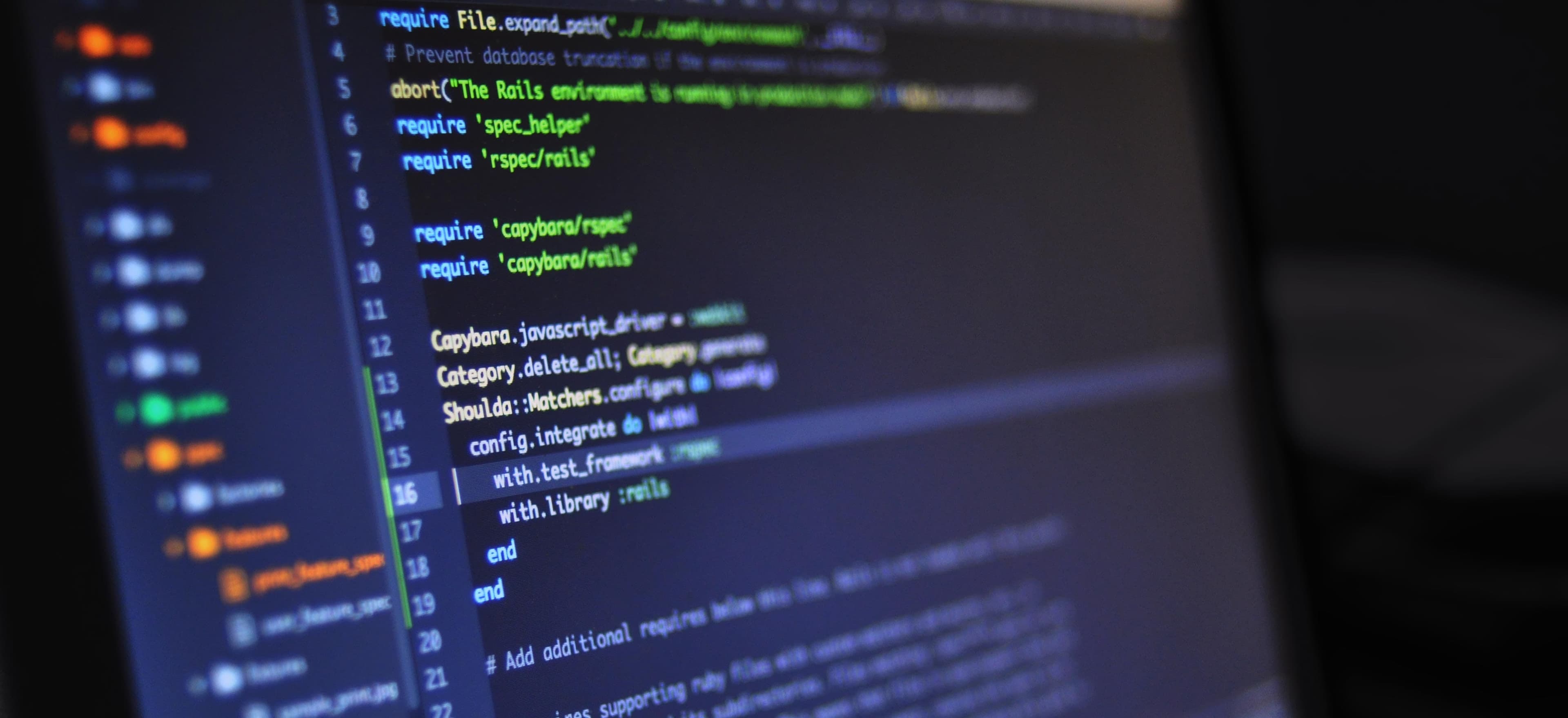
- Published on
Unlocking the Astral Body's Mysteries with Java Integrations
The concept of the astral body has captured human imagination for centuries. Astrologers and mystics have long posited that our astral body plays a crucial role in our spiritual existence and development. In the article Die Geheimnisse des astralen Körpers in der Astrologie, the nuances of this ethereal concept are explored deeply. However, how can we bring this metaphysical pursuit into the realm of technology and programming?
In this blog post, we will delve into how Java, one of the world’s most widely used programming languages, can assist in visualizing and understanding concepts related to the astral body. We will explore the intersections of technology and spirituality by creating applications that allow users to interact with and visualize the astral body concept.
Understanding the Astral Body
Before diving into coding, let’s take a moment to better grasp the concept of the astral body. It is often considered an extension of the physical self, acting as a bridge between the physical and spiritual realms. In astrology, it is believed that the astral body is influential in emotional experiences and spiritual awareness. By gaining a better understanding of it, individuals can aspire towards self-awareness and personal growth.
Java: The Language of Possibilities
Java is renowned for its versatility and portability. It provides frameworks and libraries that can aid in creating visualizations, connecting with databases, or even crafting simulations. In the context of our exploration, Java can enable developers to build applications that simulate interactions with the astral body or visualize emotional and spiritual states through astrological data.
Essential Tools and Libraries
- JavaFX - This library is essential for creating rich desktop applications. Its powerful features make it suitable for developing engaging visualizations.
- Apache Commons Math - This library aids in mathematical computations, crucial for understanding astrological calculations.
- Spring Boot - Although primarily a back-end framework, Spring Boot can help manage data flow smoothly if the application interacts with databases.
With these tools, we can start creating our metaphysical application.
Setting Up Your Java Environment
Ensure you have the following setup on your machine:
- Java Development Kit (JDK): Download the latest version from the Oracle website.
- Integrated Development Environment (IDE): Tools like IntelliJ IDEA or Eclipse can ease your development process.
Sample Structure of a Java Application
Let's lay out the structure of our Java project. This project will have a simplistic UI that simulates the relationship between astrological signs and emotional states.
astral-body-viewer/
│
├── src/
│ ├── main/
│ │ ├── java/
│ │ │ └── com/
│ │ │ └── astralbodyviewer/
│ │ │ ├── Main.java
│ │ │ ├── AstrologicalDataModel.java
│ │ │ └── UIController.java
│ │ └── resources/
│ │ └── styles.css
│ └── test/
│ └── java/
└── pom.xml
Starting with the Main Class
The Main.java
class serves as the entry point for the Java application. Here’s a basic implementation:
package com.astralbodyviewer;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
UIController uiController = new UIController();
Scene scene = new Scene(uiController.createContent());
primaryStage.setTitle("Astral Body Viewer");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Commentary: The Main
class extends Application
from the JavaFX library. This enables us to create our application’s window and manage its lifecycle. The UIController
is responsible for constructing the user interface, allowing for modular code that adheres to good programming practices.
Creating the User Interface
Next, let’s build a simple interface where users can select their astrological sign and visualize correlating emotional states.
package com.astralbodyviewer;
import javafx.scene.layout.VBox;
import javafx.scene.control.ComboBox;
import javafx.scene.text.Text;
public class UIController {
public VBox createContent() {
VBox layout = new VBox(15);
ComboBox<String> zodiacSelector = new ComboBox<>();
// Add zodiac signs for selection
zodiacSelector.getItems().addAll("Aries", "Taurus", "Gemini", "Cancer", "Leo", "Virgo", "Libra", "Scorpio", "Sagittarius", "Capricorn", "Aquarius", "Pisces");
Text emotionalState = new Text("Select your Zodiac Sign to see its Emotional state.");
// Update emotional state when a sign is selected
zodiacSelector.setOnAction(e -> {
String selectedSign = zodiacSelector.getValue();
emotionalState.setText(getEmotionalState(selectedSign));
});
layout.getChildren().addAll(zodiacSelector, emotionalState);
return layout;
}
public String getEmotionalState(String sign) {
switch (sign) {
case "Aries": return "Energetic and full of life.";
case "Taurus": return "Grounded and peaceful.";
// Add more cases for other signs...
default: return "Unknown emotional state.";
}
}
}
Commentary: This snippet introduces a simple UI component using JavaFX's VBox
layout. The user selects their zodiac sign, and the application responds with an associated emotional state. By defining this functionality, we enhance the user experience, merging technology with astrological insights.
Data Model: Astrological Insights
Let’s create a basic data model to store emotional states based on zodiac signs. This involves creating a separate class, AstrologicalDataModel
.
package com.astralbodyviewer;
import java.util.HashMap;
public class AstrologicalDataModel {
private HashMap<String, String> zodiacEmotions = new HashMap<>();
public AstrologicalDataModel() {
zodiacEmotions.put("Aries", "Energetic and full of life.");
zodiacEmotions.put("Taurus", "Grounded and peaceful.");
// Populate emotional states for all zodiac signs...
}
public String getEmotionalState(String sign) {
return zodiacEmotions.getOrDefault(sign, "Unknown emotional state.");
}
}
Commentary: Using a HashMap
allows for efficient lookups of emotional states tied to zodiac signs. This design decision highlights the importance of organizing data for quick access and maintainability.
Integrating with External APIs
To truly bring depth to our application, consider integrating with an astrology API to pull live data related to emotional states based on astrological events.
A popular choice is the Astrology API. By making HTTP requests, you can fetch real-time astrological insights and integrate them into your Java application seamlessly.
Making HTTP Requests in Java
Here’s a simple code snippet for making an HTTP GET request using Java's built-in libraries.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class AstrologyAPI {
private static final String API_URL = "https://api.example.com/astrology";
public String getAstrologicalData(String zodiacSign) {
try {
URL url = new URL(API_URL + "?sign=" + zodiacSign);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
StringBuilder response = new StringBuilder();
String inputLine;
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
} catch (Exception e) {
e.printStackTrace();
return "Error encountered while fetching data.";
}
}
}
Commentary: This method uses Java's HttpURLConnection
to send a GET request to the API. By incorporating real-time data, we enhance our application’s interactivity, adding another layer to our understanding of the astral body.
The Bottom Line
The integration of Java with astrological concepts provides fascinating avenues for exploration. By building applications such as the Astral Body Viewer, you can engage users in understanding their emotional and spiritual states better.
The balance of programming and spirituality can lead to profound insights about oneself and one's surroundings. By leveraging the tools available within Java, developers can make the intangible aspects of spirituality more accessible and engaging.
If you're intrigued by that metaphysical journey of discovering the astral body’s mysteries, consider diving deeper into related topics in astrology through resources like the article Die Geheimnisse des astralen Körpers in der Astrologie.
Happy coding and spiritual exploration!
Checkout our other articles