Streamlining Jenkins: Java Solutions for Cloud Config Issues
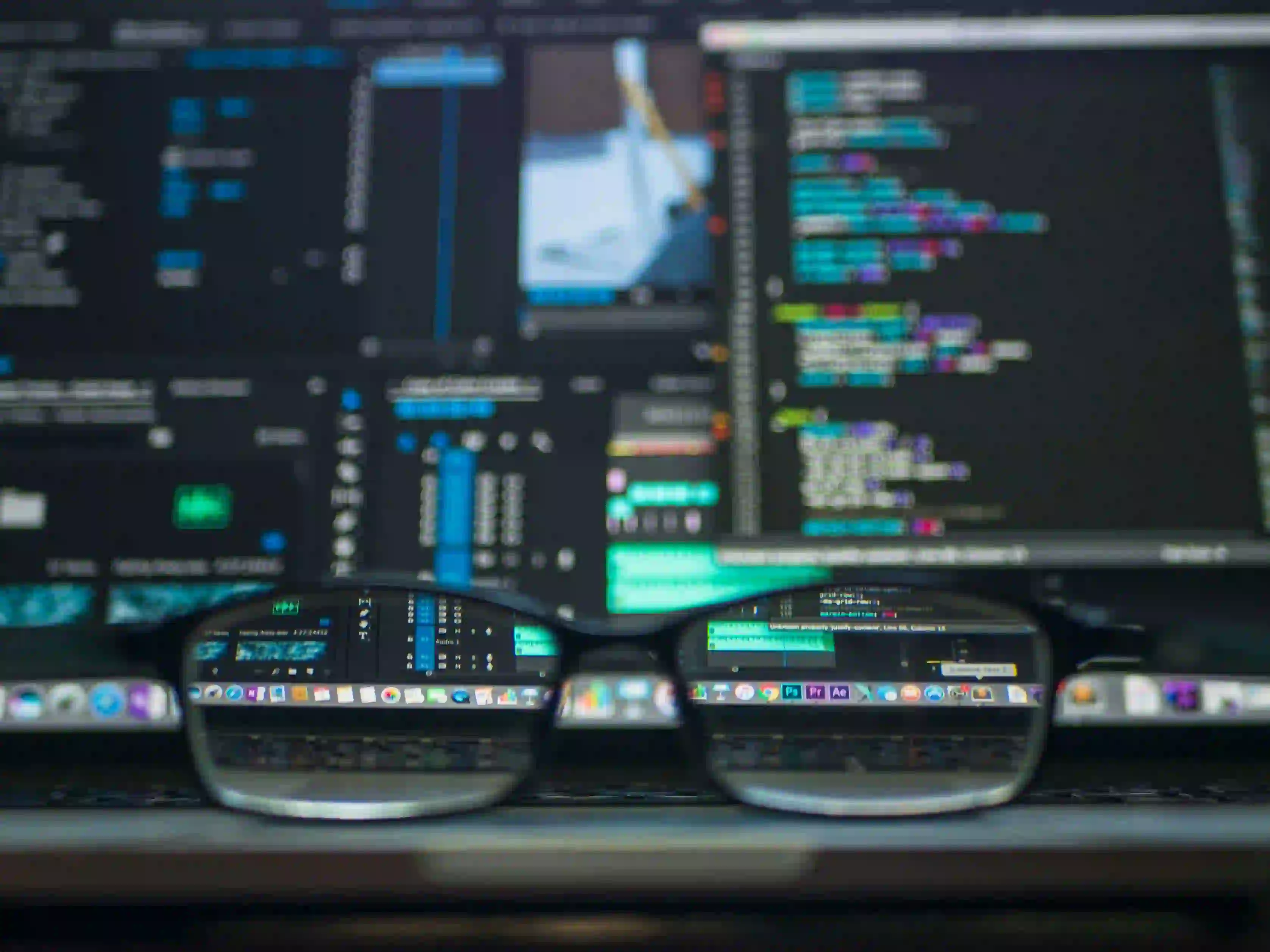
Streamlining Jenkins: Java Solutions for Cloud Config Issues
In today's fast-paced software development environment, Continuous Integration and Continuous Deployment (CI/CD) have become essential practices for teams to adopt. Jenkins, an open-source automation server, is a popular choice for implementing CI/CD pipelines. However, when it comes to deploying Jenkins in the cloud, various configuration challenges can arise, particularly for Java developers. In this blog post, we'll explore ways to streamline Jenkins configurations using Java, and address common cloud configuration challenges. If you're dealing with these issues, you might find insights in the existing article "Overcoming Jenkins Configuration Challenges in the Cloud" available at configzen.com/blog/overcoming-jenkins-cloud-challenges.
Understanding Jenkins in the Cloud
Before we dive into solutions, let's clarify why Jenkins configurations can become complex when deployed in a cloud environment. The cloud provides scalability and flexibility but also introduces new variables. Network latency, diverse cloud services, and container orchestration can complicate configurations.
Configuration File Management
One common area of configuration challenges is managing the Jenkins configurations themselves. Traditionally, Jenkins relies on XML files for its configuration. When deployed in the cloud, these files can quickly become unwieldy. Java can help manage these configurations more effectively than direct XML manipulation.
Using Java to read, modify, and maintain Jenkins configuration files allows us to implement stronger data validation and error handling.
Here's an example code snippet to read a Jenkins configuration file and parse it:
import java.io.File;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
public class JenkinsConfigReader {
public Document readConfig(String configFilePath) {
try {
File configFile = new File(configFilePath);
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(configFile);
doc.getDocumentElement().normalize();
return doc;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
Why Use Java for Configuration Management?
Using Java for reading configuration files allows for more robust error handling, abstraction, and potential integration with other Java-based tools. When you catch exceptions, you can provide meaningful error messages, allowing developers to diagnose issues faster.
Automating Jenkins with Java
Another area where Java can benefit Jenkins cloud deployments is automation. Jenkins has numerous APIs that allow for automation, which can be leveraged using Java. Let's look at how you can automate the job creation process in Jenkins.
Job Creation with Java
To create a new Jenkins job programmatically, you can use the Jenkins REST API. Below is an example using the HttpURLConnection
class in Java:
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class JenkinsJobCreator {
public void createJob(String jobName, String jobConfigXml, String jenkinsUrl, String apiToken) {
try {
String url = jenkinsUrl + "/createItem?name=" + jobName;
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Authorization", "Bearer " + apiToken);
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/xml");
OutputStream os = con.getOutputStream();
os.write(jobConfigXml.getBytes());
os.flush();
os.close();
int responseCode = con.getResponseCode();
System.out.println("Response Code: " + responseCode);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Matters
Automating job creation ensures consistent deployments across environments. It reduces the risk of human error and allows your team to rapidly scale its operations.
Custom Jenkins Plugins
When facing specific challenges that cannot be resolved with existing solutions, you might consider creating custom Jenkins plugins using Java. Jenkins supports plugin development, allowing you to extend its capabilities tailored to your needs.
Getting Started with a Plugin
Creating a Jenkins plugin typically involves using the Jenkins Plugin Parent POM, which provides all the necessary dependencies. Here’s a basic structure of a Java-based Jenkins plugin:
import hudson.Extension;
import hudson.model.Descriptor;
import hudson.model.Job;
import hudson.model.Run;
import hudson.tasks.Builder;
@Extension
public class MyJenkinsPlugin extends Builder {
@Override
public boolean perform(Job job, Run run, hudson.model.TaskListener listener) {
listener.getLogger().println("Executing My Jenkins Plugin...");
return true;
}
@Override
public Descriptor<Builder> getDescriptor() {
return super.getDescriptor();
}
}
Why Build a Plugin?
Creating a custom plugin allows developers the flexibility to integrate proprietary APIs, enforce certain configurations, or even log information in a specific way that aligns with organizational standards. It ultimately provides a way to tailor Jenkins to fit more closely with development workflows.
Handling Configuration Drift
In distributed systems, configuration drift refers to the phenomenon of discrepancies between the expected and actual configurations across environments. To mitigate this, you can implement versioning for your configurations.
Using Java for Version Control
You can store Jenkins configurations in a Git repository, and use Java to handle CRUD operations on your config files. For instance, a simple method to commit changes to a repository can look like this:
import org.eclipse.jgit.api.Git;
public class GitConfigVersioner {
public void commitConfigChange(String repoPath, String configFilePath, String commitMessage) {
try (Git git = Git.open(new File(repoPath))) {
git.add().addFilepattern(configFilePath).call();
git.commit().setMessage(commitMessage).call();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why Version Control Is Important
Versioning provides the ability to revert to previous configurations when something goes wrong. It also allows teams to track changes and understand what modifications affect deployment behavior.
Final Thoughts
Navigating Jenkins configurations in a cloud environment can indeed be challenging. However, with Java’s robust capabilities, you can streamline the process and resolve common configuration issues effectively. From handling configuration files to automating job generation and creating custom plugins, tools exist for developers to enhance their Jenkins experience.
For those grappling with Jenkins optimizations in cloud environments, further insights can be found in the article "Overcoming Jenkins Configuration Challenges in the Cloud" available at configzen.com/blog/overcoming-jenkins-cloud-challenges.
With these strategies in mind, your team will not only overcome existing challenges but thrive in a competitive development landscape. Happy coding!