How Netflix's Preppers Use Java to Handle Unpredictability
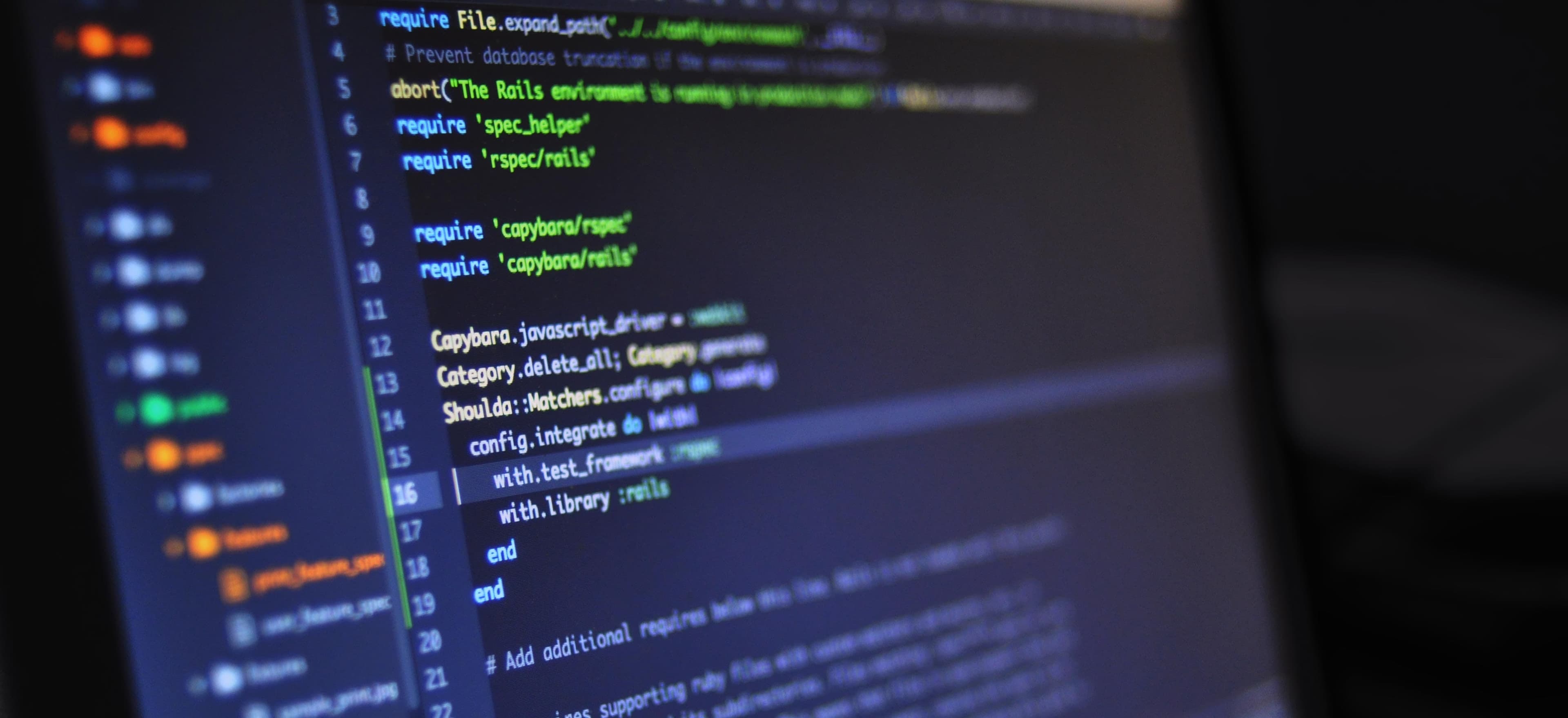
- Published on
How Netflix's Preppers Use Java to Handle Unpredictability
In the ever-evolving tech landscape, unpredictability can be daunting. It requires a behavior adjustment that allows for rapid adaptation. A striking example of this adaptability comes from Netflix, a company that has redefined how we consume media. Their series, "Preppers," demonstrates key lessons in handling chaos and unpredictability—lessons that resonate beyond reality television into the realm of software development, particularly Java programming.
For developers, understanding how to navigate uncertainty is paramount. In this article, we will explore how principles from Netflix's Preppers can inform Java practices for developing resilient, adaptive applications. So let’s dive in!
Understanding Unpredictability
Unpredictability is inherent in both life and technology. As we learned from the insights in the article "Surviving Chaos: Key Lessons from Netflix's Preppers," effective preparation can help tackle unpredictable scenarios effectively. Read the article here.
In software development, unpredictability often manifests in server overloads, sudden spikes in user traffic, or outright system failures. Java, with its flexibility and extensive ecosystem, provides developers with a toolkit for building applications that can withstand these uncertainties.
Java and Resilience
Java is a multi-paradigm language that supports object-oriented, functional, and imperative programming styles. One of its standout features is its robustness, which plays a crucial role in building resilient applications.
1. Exception Handling
Effective error and exception handling is paramount. In unpredictable environments, things will go wrong. Here's a basic example:
public void readFile(String filePath) {
try {
BufferedReader reader = new BufferedReader(new FileReader(filePath));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
} catch (FileNotFoundException e) {
// Log exception
System.err.println("File not found: " + filePath);
} catch (IOException e) {
// Log exception
System.err.println("I/O error while reading the file: " + e.getMessage());
}
}
Why this matters: Using try-catch blocks helps your application gracefully handle errors instead of crashing. Logging errors also allows developers to track issues, which is vital for diagnosing problems in unpredictable scenarios.
2. Multi-Threading
In a chaotic environment, relying on a single thread can be limiting. Using Java’s concurrency framework allows you to efficiently manage multiple tasks simultaneously. Consider the following code snippet that demonstrates multi-threading:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class MultiThreadingExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(3);
for (int i = 0; i < 10; i++) {
final int taskId = i;
executor.submit(() -> handleTask(taskId));
}
executor.shutdown();
}
private static void handleTask(int taskId) {
System.out.println("Handling task " + taskId + " in thread " + Thread.currentThread().getName());
}
}
Why this matters: With multi-threading, you can handle multiple tasks simultaneously, leading to more efficient resource usage. In unpredictable situations, this robustness can lead to quicker responses and improved application performance.
3. Load Balancing
Similar to how Netflix manages viewership spikes, developers must build applications that can efficiently handle varying loads. Load balancing can distribute incoming requests across multiple servers, ensuring no single server becomes overwhelmed.
Here's a pseudo-code example illustrating a load balancer:
import java.util.List;
import java.util.Random;
public class LoadBalancer {
private List<Server> servers;
private Random random = new Random();
public LoadBalancer(List<Server> servers) {
this.servers = servers;
}
public Server chooseServer() {
int index = random.nextInt(servers.size());
return servers.get(index); // Randomly select a server to distribute load
}
}
Why this matters: By implementing load balancing techniques, your application can continue to function optimally, even during traffic spikes, effectively preparing for unpredictable user behavior much like Netflix in its peak streaming hours.
Adapting to Change: The Agile Approach
The Agile methodology aligns well with the chaotic nature of software development. It emphasizes iterative progress, flexibility, and responsiveness. This aligns with the adaptability exhibited by Netflix's Preppers who constantly reassess their environments and adjust strategies.
Leveraging Java Frameworks
Java frameworks such as Spring allow for a more agile development process. Allowing for rapid iteration through dependency injection and aspect-oriented programming, these frameworks let teams pivot quickly:
@Configuration
public class AppConfig {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
}
Why this matters: Using frameworks enhances the robustness of Java applications and facilitates a quick response to changes—a key lesson from Netflix's adaptability.
Monitoring and Feedback Loops
To survive chaos, continuous monitoring and feedback loops are essential. Application Performance Monitoring (APM) tools like New Relic or dynamic logging can alert developers to impending issues before they escalate.
In Java, leveraging observers can help keep track of events:
import java.util.ArrayList;
import java.util.List;
public class EventSource {
private List<EventListener> listeners = new ArrayList<>();
public void addListener(EventListener listener) {
listeners.add(listener);
}
public void notifyListeners(Event event) {
for (EventListener listener : listeners) {
listener.update(event);
}
}
}
Why this matters: Implementing a system of observation allows you to react to system events in real-time, much like Netflix preppers who must constantly adapt to new information in their environment.
Closing Remarks
Java offers numerous tools and methodologies for tackling unpredictability in application development. By understanding concepts such as exception handling, multi-threading, load balancing, and Agile methodologies, developers can create applications that not only withstand challenges but thrive amidst chaos.
By embracing the lessons from Netflix's "Preppers," you too can develop software that is not only robust but also responsive to the constantly changing environment in which it operates. The principles of adaptation and continuous improvement are essential for any successful Java developer navigating the unpredictable technological landscape.
Take these principles to heart, and remember, with every unpredictable twist you encounter, there is an opportunity to innovate and enhance your coders’ toolkit.
Checkout our other articles