Troubleshooting MongoDB Installation on Java-Driven Projects
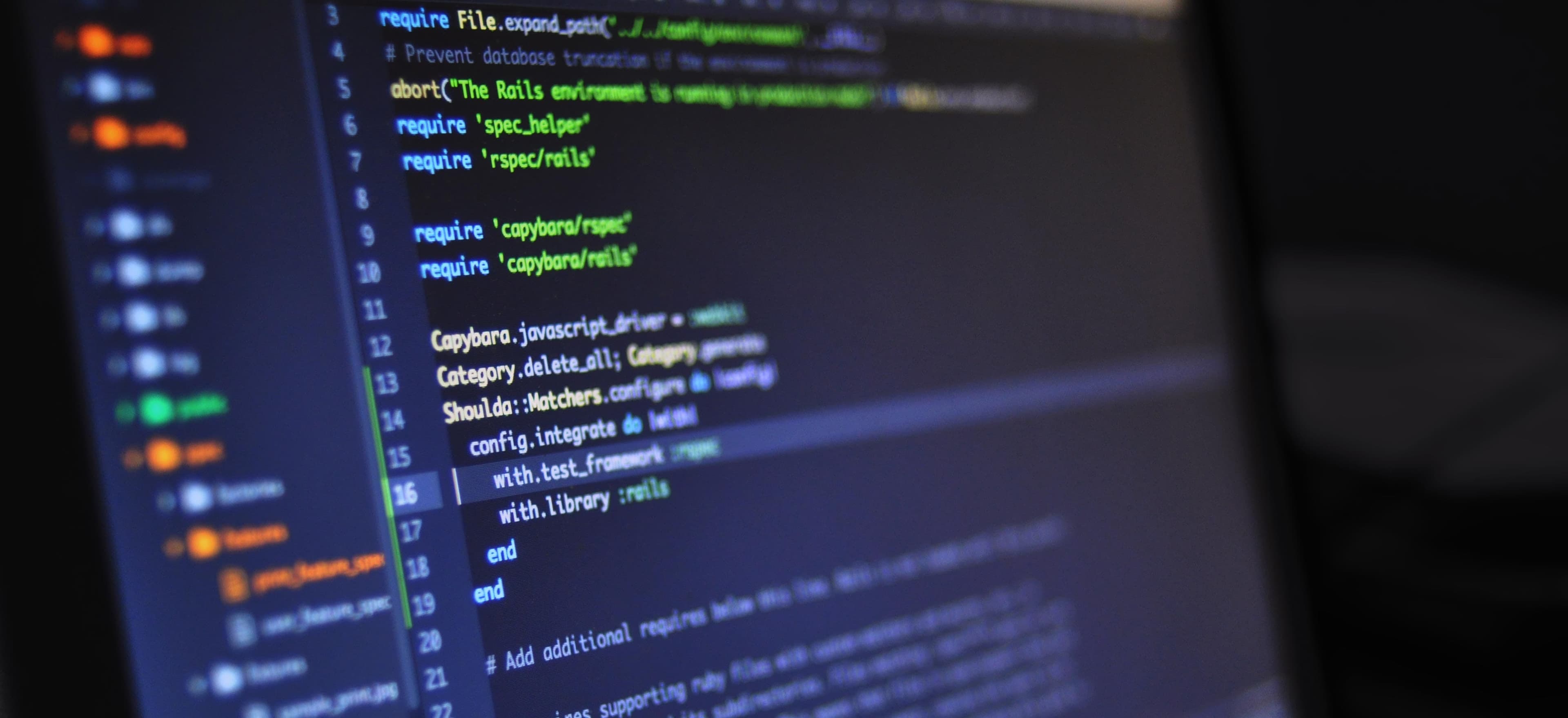
- Published on
Troubleshooting MongoDB Installation on Java-Driven Projects
As developers increasingly integrate NoSQL databases like MongoDB into Java applications, understanding the common installation issues can save time and frustration. Challenges often arise during the installation phase, which can lead to so-called "dependency hell" or misconfigurations. In this blog post, we will dive into the common problems developers face when installing MongoDB on Oracle Linux for their Java projects, providing guidance, code snippets, and best practices to address these issues effectively.
Understanding MongoDB and Java Integration
MongoDB is a popular NoSQL database that provides flexibility and scalability. Combining MongoDB with Java offers a diverse range of functionalities, thanks to various libraries and frameworks like Spring Data MongoDB and the MongoDB Java Driver.
Why MongoDB?
- Schema Flexibility: Changes to the schema can be made without downtime.
- Scalability: MongoDB scales horizontally; adding more servers can increase capacity.
- Rich Query Language: It supports diverse data types and extensive querying capabilities.
Before diving into troubleshooting, ensure you have some prerequisites in place for successful integration and installation.
Prerequisites for MongoDB Installation on Oracle Linux
Before installing MongoDB, confirm you have the following:
- Java Development Kit (JDK): Ensure you have JDK version 8 or above installed.
- Oracle Linux Distribution: Ensure it's configured correctly with up-to-date packages.
- Network Access: Allow access to MongoDB ports (default 27017).
Installing Java
Here’s a succinct way to install JDK:
sudo yum install -y java-11-openjdk-devel
This command installs JDK 11 on your Oracle Linux system. You can check the installation by running:
java -version
Installing MongoDB on Oracle Linux
Referencing the article "Common Installation Issues with MongoDB on Oracle Linux" available at configzen.com/blog/common-mongodb-installation-issues-oracle-linux will help you understand common pitfalls during installation.
Step 1: Create a MongoDB Repo File
To install MongoDB, first create a repository file:
echo "[mongodb-org-5.0]
name=MongoDB Repository
baseurl=https://repo.mongodb.org/yum/redhat/7/mongodb-org/5.0/x86_64/
gpgcheck=1
enabled=1
gpgkey=https://www.mongodb.org/static/pgp/server-5.0.asc" | sudo tee /etc/yum.repos.d/mongodb-org-5.0.repo
This block sets up the MongoDB repository. The "baseurl" points to the MongoDB packages, while the "gpgkey" makes sure the packages are trustworthy.
Step 2: Install MongoDB
Next, install MongoDB with the following command:
sudo yum install -y mongodb-org
The command installs the latest stable version of MongoDB. If you encounter issues here, verify if your YUM repository is configured correctly.
Step 3: Starting MongoDB Service
After installation, you can start the MongoDB service:
sudo systemctl start mongod
To check the status of MongoDB:
sudo systemctl status mongod
Common Installation Issues
1. Dependency Issues
When installing MongoDB, dependency issues can arise, particularly with older or conflicting packages. If YUM complains about unmet dependencies, update your existing packages:
sudo yum update
2. Configuration File Issues
MongoDB relies on a configuration file typically located at /etc/mongod.conf
. If there are syntax errors or incorrect settings, MongoDB won't start. Ensure that the format looks like this:
# mongod.conf
storage:
dbPath: /var/lib/mongo
systemLog:
destination: file
path: /var/log/mongodb/mongod.log
logAppend: true
net:
bindIp: 127.0.0.1
port: 27017
Ensure the dbPath
directory exists and permissions are appropriately set.
3. Firewall Issues
The firewall on Oracle Linux may block MongoDB’s default port (27017). Allow access by running:
sudo firewall-cmd --zone=public --add-port=27017/tcp --permanent
sudo firewall-cmd --reload
4. SELinux Configuration
If SELinux is enforced, it may prevent MongoDB from accessing the required directories. Temporarily disable SELinux for testing:
sudo setenforce 0
For a permanent solution, modify the SELinux configuration. Refer to official documentation for detailed steps.
5. Checking Logs for Errors
If MongoDB fails to start, viewing the logs can provide guidance. You can check logs using:
cat /var/log/mongodb/mongod.log
Logs will detail any issues with the startup or runtime configuration.
Integrating MongoDB with Java Applications
To use MongoDB from your Java application, include the MongoDB Java driver in your project dependencies. If you're using Maven, add the following to your pom.xml
:
<dependency>
<groupId>org.mongodb</groupId>
<artifactId>mongodb-driver-sync</artifactId>
<version>4.9.0</version>
</dependency>
Sample Java Code to Connect to MongoDB
import com.mongodb.MongoClient;
import com.mongodb.client.MongoDatabase;
public class MongoDBConnection {
public static void main(String[] args) {
// Establish connection
MongoClient mongoClient = new MongoClient("localhost", 27017);
// Access database
MongoDatabase database = mongoClient.getDatabase("myDatabase");
// Print the name of the database
System.out.println("Connected to database: " + database.getName());
// Closing the connection
mongoClient.close();
}
}
Code Commentary
- MongoClient: This is the main entry point for connecting to MongoDB. In this instance, we connect to the localhost on the default port.
- MongoDatabase: Represents our target database. Change "myDatabase" to the desired database name.
- Close the Connection: Always ensure to close the connection after operations to free up resources.
Final Thoughts
Proactively troubleshooting MongoDB installation issues on Oracle Linux can significantly enhance your experience while working on Java-driven projects. Always refer back to foundational guides, such as the article on common installation issues, to troubleshoot effectively.
Use the best practices shared in this post to ensure smooth integration and operation. With MongoDB's robust features and Java's versatility, you can build sophisticated applications with ease.
For further detailed insights and troubleshooting tips related to MongoDB installation on Oracle Linux, check out Common Installation Issues with MongoDB on Oracle Linux.
Let's build great applications!
Checkout our other articles