Leveraging TypeScript Utility Types in Java Frameworks
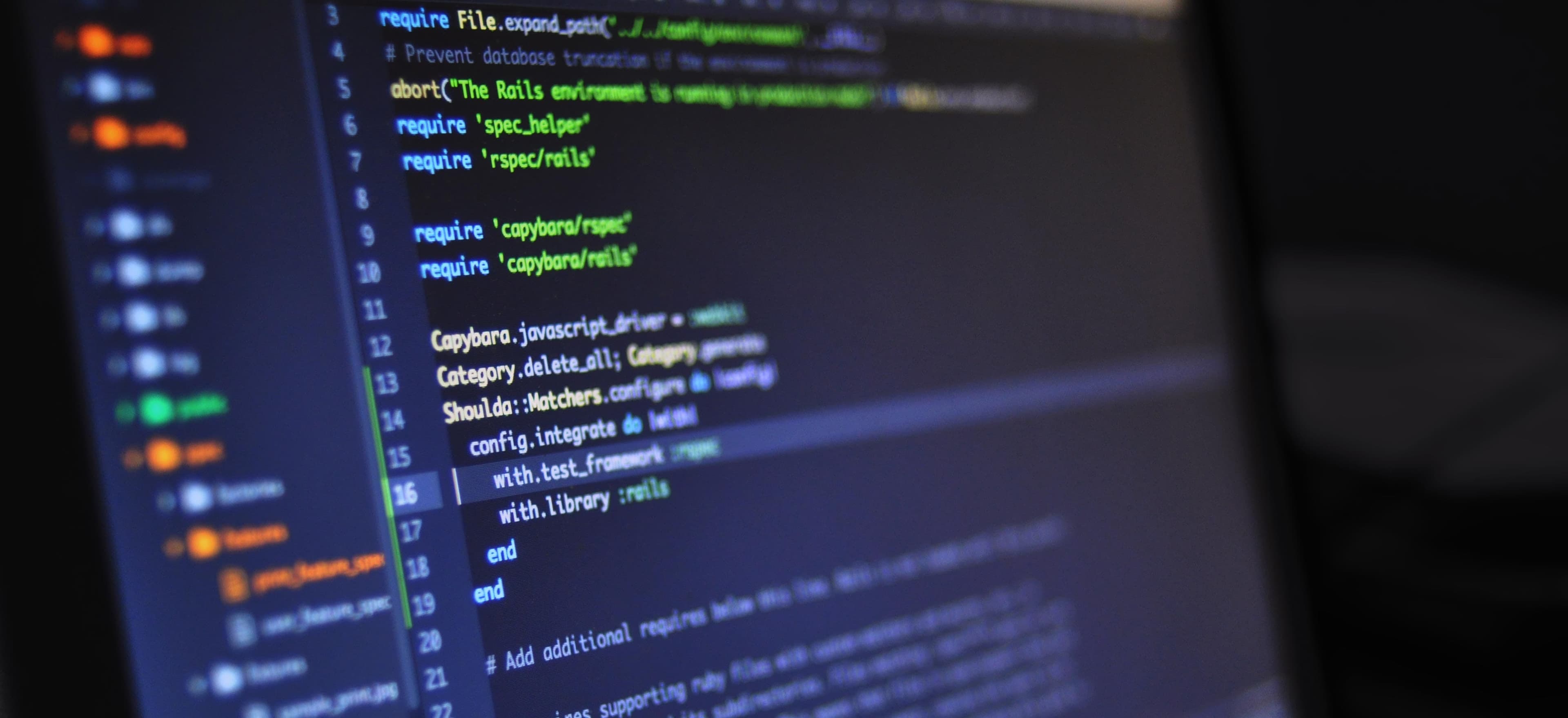
- Published on
Leveraging TypeScript Utility Types in Java Frameworks
In the rapidly evolving world of software development, the ability to write clean, maintainable code is paramount. While TypeScript's utility types provide a powerful way to craft more readable and efficient applications, you may be wondering how these concepts can be utilized within Java frameworks. This blog post will explore the intersections of TypeScript utility types and Java, providing insights on enhancing code quality, readability, and flexibility.
Understanding TypeScript Utility Types
Before delving into their application in Java, let’s take a moment to recap what TypeScript utility types are. According to the article Mastering TypeScript Utility Types for Cleaner Code, utility types are built-in methods to manipulate and construct types in TypeScript. They simplify complex type manipulations and improve code readability.
Here are some commonly used utility types in TypeScript:
- Partial: Makes all properties of a type optional.
- Required: Makes all properties of a type required.
- Readonly: Makes all properties of a type readonly.
- Record: Constructs an object type with specific properties.
Understanding how to replicate this functionality in Java can greatly enhance your productivity and the quality of your codebase.
Bridging TypeScript and Java Concepts
Though Java is statically typed and lacks some of the functional features found in TypeScript, it's still possible to work with similar concepts. Let's explore how Java can leverage ideas from TypeScript utility types by designing our classes and interfaces with these principles in mind.
Example 1: Partial Type Implementation
In TypeScript, the Partial utility type allows developers to work with objects wherein only some properties are required. In Java, we can achieve similar flexibility using builders or optional values.
Here’s a comparison:
TypeScript Example
interface User {
id: number;
name: string;
email?: string; // optional
}
function updateUser(userId: number, userUpdates: Partial<User>) {
// Update user logic here
}
Equivalent Java Example
In Java, you can use a builder pattern:
public class User {
private final int id;
private final String name;
private final String email;
private User(UserBuilder builder) {
this.id = builder.id;
this.name = builder.name;
this.email = builder.email;
}
public static class UserBuilder {
private final int id;
private final String name;
private String email; // optional
public UserBuilder(int id, String name) {
this.id = id;
this.name = name;
}
public UserBuilder setEmail(String email) {
this.email = email;
return this;
}
public User build() {
return new User(this);
}
}
}
// Usage:
User user = new User.UserBuilder(1, "John Doe").setEmail("john.doe@example.com").build();
Commentary
In the Java example, the builder pattern mimics the behavior of the Partial utility type by allowing object creation with optional fields. This pattern increases the flexibility of our class, permitting partial updates while maintaining type safety.
Example 2: Readonly Implementation
The Readonly utility type in TypeScript creates immutable types. Java has a concept of immutability, generally promoted through the use of final variables or immutable collections.
TypeScript Example
interface Config {
readonly apiUrl: string;
}
const config: Config = {
apiUrl: "https://api.example.com"
};
// config.apiUrl = "https://api.another-example.com"; // Error
Equivalent Java Example
In Java, immutability can be achieved as follows:
public class Config {
private final String apiUrl;
public Config(String apiUrl) {
this.apiUrl = apiUrl;
}
public String getApiUrl() {
return apiUrl;
}
}
// Usage:
Config config = new Config("https://api.example.com");
// config.apiUrl = "https://api.another-example.com"; // Not possible
Commentary
In this Java implementation, the Config
class encapsulates immutable fields using the final keyword. Such designs not only prevent accidental changes to critical fields but also conform to best practices in software design, ensuring that objects remain consistent throughout their lifespan.
Example 3: Required Type Implementation
In TypeScript, the Required utility type ensures all properties are specified. In Java, we can enforce this through constructor requirements or validation techniques.
TypeScript Example
interface User {
id: number;
name: string;
email: string; // required
}
function createUser(user: Required<User>) {
// User creation logic here
}
Equivalent Java Example
In Java, constructor parameters can be made mandatory:
public class User {
private final int id;
private final String name;
private final String email;
public User(int id, String name, String email) {
if (email == null || email.isEmpty()) {
throw new IllegalArgumentException("Email cannot be null or empty");
}
this.id = id;
this.name = name;
this.email = email;
}
}
// Usage:
User user = new User(1, "John Doe", "john.doe@example.com");
Commentary
In the Java example, the constructor enforces that all properties, including the email, must be provided. This validation ensures that the object is created in a valid state, similar to how the Required utility type operates in TypeScript.
Lessons Learned
In this blog post, we explored how the principles underlying TypeScript's utility types can be effectively utilized in Java frameworks. By employing patterns such as builders, immutability, and constructor validation, developers can replicate the flexibility and readability offered by TypeScript utility types.
The discussions in Mastering TypeScript Utility Types for Cleaner Code can significantly inspire Java developers looking to write cleaner, more maintainable code.
Together, these practices encourage a shift towards more thoughtful design when crafting classes and interfaces, ultimately leading to a more robust software development experience. Whether you are in a TypeScript or Java environment, the underlying principles of good design are universal.
Feel free to implement these principles in your Java projects to harness the true potential of your coding practices!
Checkout our other articles