Troubleshooting MongoDB Integration in Java Applications
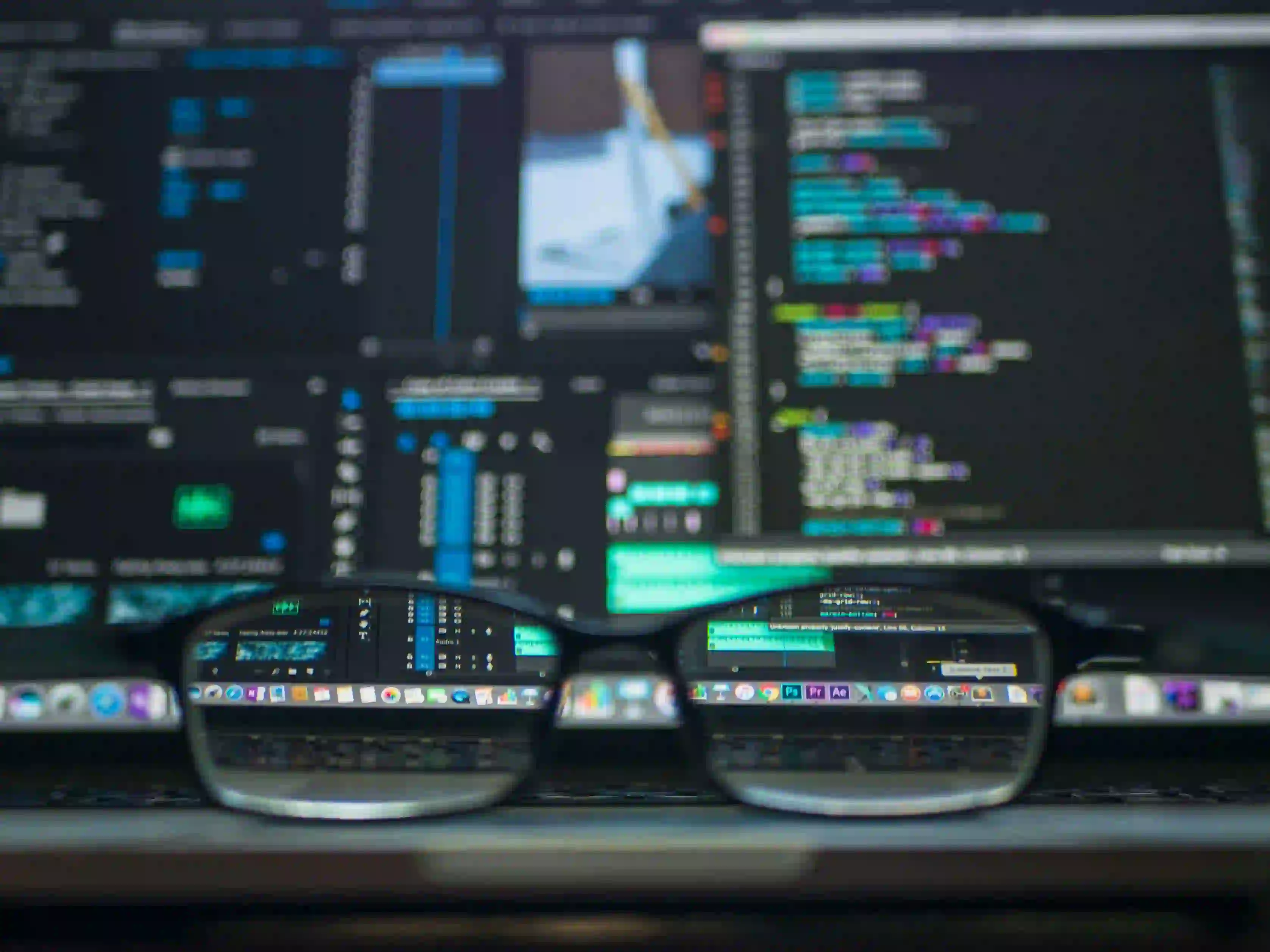
Troubleshooting MongoDB Integration in Java Applications
Integrating MongoDB with Java applications can significantly enhance your ability to work with data in a flexible and scalable manner. However, as with any technology integration, you may encounter certain issues that hinder your progress. In this blog post, we'll explore common troubleshooting techniques specifically related to integrating MongoDB within Java applications.
Understanding MongoDB and Java Interactions
MongoDB is a NoSQL database designed for storing large volumes of data in a flexible, schema-less structure. Java, a versatile and widely-used programming language, offers excellent support for database connectivity. Together, they form a powerful toolset for developers working on modern applications.
Before diving into integrations and troubleshooting techniques, it's essential to set up a reliable environment. For a comprehensive guide on Common Installation Issues with MongoDB on Oracle Linux, you can refer to the article on ConfigZen.
Basic Setup Steps
To start integrating MongoDB into your Java application, you need to follow these steps:
Step 1: Install MongoDB and Java Driver
Ensure that you have MongoDB installed on your system. You can download the latest version from the official MongoDB website.
Next, add the MongoDB Java Driver to your project. If you are using Maven, add the following dependency in your pom.xml
:
<dependency>
<groupId>org.mongodb</groupId>
<artifactId>mongodb-driver-sync</artifactId>
<version>4.7.0</version> <!-- Check for latest version -->
</dependency>
Step 2: Connecting to MongoDB
Creating a connection to MongoDB is the first step after ensuring that the driver is installed. Here’s how you can do it:
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoClient;
public class MongoConnection {
public static void main(String[] args) {
// Create a new client and connect to the server running on localhost
try (MongoClient mongoClient = MongoClients.create("mongodb://localhost:27017")) {
System.out.println("Connection to database successful!");
} catch (Exception e) {
System.err.println("Connection failed: " + e.getMessage());
}
}
}
Why this Code? The MongoClients.create()
method establishes a connection to the MongoDB server. It also handles exceptions smoothly and ensures that resources are cleaned up when no longer needed.
Step 3: Perform CRUD Operations
With the connection established, you can start performing CRUD (Create, Read, Update, Delete) operations. Here's how you can perform a simple insert operation:
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.MongoClients;
import org.bson.Document;
public class InsertExample {
public static void main(String[] args) {
try (var mongoClient = MongoClients.create("mongodb://localhost:27017")) {
MongoDatabase database = mongoClient.getDatabase("testdb");
MongoCollection<Document> collection = database.getCollection("testCollection");
Document document = new Document("name", "John Doe")
.append("age", 30)
.append("city", "New York");
collection.insertOne(document);
System.out.println("Document inserted successfully");
} catch (Exception e) {
System.err.println("Insert failed: " + e.getMessage());
}
}
}
Why this Code? We create a Document
instance, which is analogous to a row in SQL databases. We then insert it into the designated collection using insertOne()
. Handling exceptions allows you to understand what went wrong, if anything.
Troubleshooting Common Issues
While performing operations, you might face several common issues. Here is a breakdown of them along with possible solutions.
1. Connection Refused Errors
If you receive connection refused errors, ensure that:
- MongoDB is running on the specified port.
- You are using the correct connection string (
mongodb://localhost:27017
). - Your firewall allows traffic on the MongoDB port (default is 27017).
2. Authentication Problems
If you have enabled authentication but receive authentication errors:
- Verify that your credentials are correct.
- Ensure that the user has the necessary permissions to access the database.
Here’s an example of how to use credentials in your connection string:
MongoClients.create("mongodb://username:password@localhost:27017");
3. Driver Compatibility Issues
Make sure you are using the compatible version of the MongoDB Java Driver with the version of MongoDB you have installed. Review the official compatibility chart for more information.
4. Document Structure Issues
When working with document structures, ensure they match what your application expects. Missing fields can lead to null
values or run-time exceptions, as shown below:
try {
Document doc = collection.find(eq("name", "Jane Doe")).first();
if (doc == null) {
throw new Exception("Document not found!");
} else {
// Process document
}
} catch (Exception e) {
System.err.println("Query failed: " + e.getMessage());
}
Why this Code? By checking whether the document is null
, you can handle cases where no record matches the query, ensuring your application does not throw unexpected null-pointer exceptions.
5. Connection Pooling Performance Issues
In high-load applications, connection pooling is critical. Inadequate connections can lead to slow performance or timeouts. To configure connection pooling, you can set options when creating the MongoClient:
import com.mongodb.MongoClientOptions;
import com.mongodb.MongoClientURI;
MongoClientURI uri = new MongoClientURI("mongodb://localhost:27017/?maxPoolSize=100");
try (MongoClient mongoClient = MongoClients.create(uri)) {
// Use mongoClient...
}
Why this Code? Here, we configure maxPoolSize
to 100, allowing for more simultaneous connections, thus improving performance.
A Final Look
Successfully troubleshooting MongoDB integrations in Java applications involves understanding the setup, performing proper CRUD operations, and being ready to tackle common issues as they arise. Implementing sound coding practices and test-driven development can significantly minimize problems.
By actively referencing resources like the helpful guide on Common Installation Issues with MongoDB on Oracle Linux, you can better equip yourself to face challenges head-on.
Feel free to ask questions or share your experiences with MongoDB and Java integrations in the comments section below!