Leveraging TypeScript with Java for Type Safety Solutions
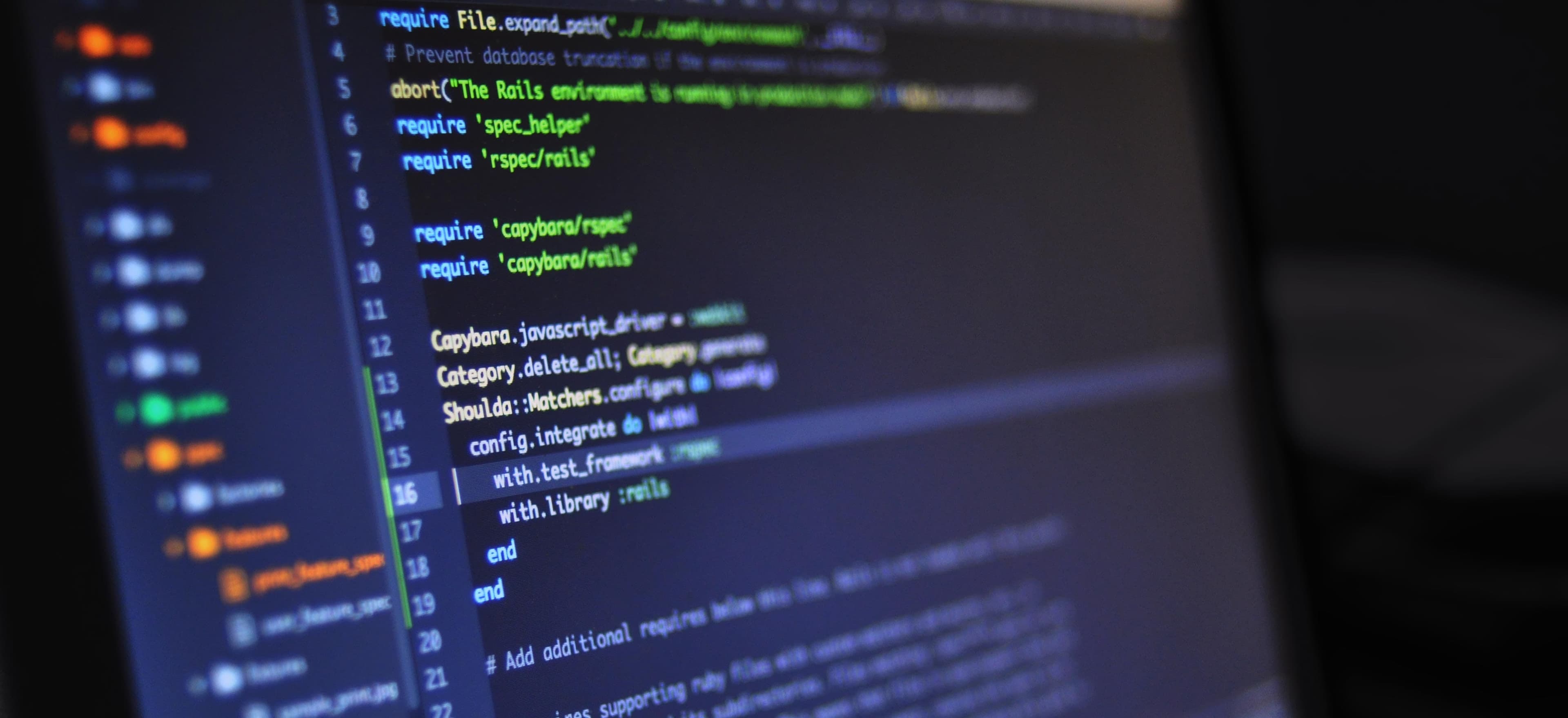
- Published on
Leveraging TypeScript with Java for Type Safety Solutions
In today's development landscape, achieving type safety is a crucial aspect of building robust applications. While Java has always been a strongly typed language, the growing prominence of TypeScript in web development has sparked interest in how these two powerful technologies can complement each other. In this blog post, we'll explore how to leverage TypeScript alongside Java to create safer and cleaner solutions.
Why Type Safety Matters
Type safety is the degree to which a programming language discourages or prevents type errors. This is essential because type errors can lead to hard-to-diagnose bugs and runtime failures. By ensuring that types are well-defined and enforced, developers can significantly reduce errors and improve the maintainability of their codebases.
In Java, we inherently benefit from static type checking. TypeScript brings similar benefits to JavaScript, helping developers catch errors during development instead of at runtime. This blog post will delve into leveraging TypeScript's advanced type system—especially its utility types—and discuss how they intersect with Java's type system.
For a deep dive into TypeScript utility types, check out the article on Mastering TypeScript Utility Types for Cleaner Code.
Integrating TypeScript and Java
Before we dive into examples, let's clarify how TypeScript and Java can work together. Here are a few common use cases:
-
Frontend and Backend Communication: TypeScript can be employed to provide a type-safe interface for REST APIs developed in Java.
-
Shared Models: When both your frontend and backend share data models, TypeScript can ensure those models match your Java counterparts.
-
Hardware Abstraction: For applications involving microservices, TypeScript can define the expected types for service responses and requests, maintaining integrity between services.
Example Project: Type-Safe API Communication
Let's create a simple project that demonstrates how TypeScript can be used to ensure a type-safe interaction with a Java backend.
Java Backend: Spring Boot Setup
Here's a Java controller we might define in a Spring Boot application.
@RestController
@RequestMapping("/api/users")
public class UserController {
@GetMapping("/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
User user = userService.findById(id);
return ResponseEntity.ok(user);
}
}
In the code above:
- We define a REST endpoint to retrieve a user's information.
- The response is typed as
ResponseEntity<User>
, which guarantees that the response will align with theUser
model.
User Model Example
public class User {
private Long id;
private String username;
private String email;
// Getters and Setters
}
This model serves not just as a data structure but also provides type information essential for strong type-checking.
TypeScript Frontend
Now, let's see how we can interact with the Java backend using TypeScript and ensure we maintain type safety.
TypeScript Interface Definition
interface User {
id: number;
username: string;
email: string;
}
By defining a TypeScript interface that mirrors the Java model, we can ensure that the data structures are compatible. This is crucial for maintaining consistent data across the application.
Fetching User Data with Type Assertion
Here’s how we might fetch user data from the API:
async function fetchUser(userId: number): Promise<User> {
const response = await fetch(`/api/users/${userId}`);
// Ensure the response is successful
if (!response.ok) {
throw new Error('Network response was not ok');
}
const user: User = await response.json();
return user;
}
In the code above:
- We make an asynchronous call to the Java backend.
- Upon receiving the response, we assert that it conforms to our
User
interface, allowing us to catch discrepancies at compile-time rather than run-time.
Advanced TypeScript Utility Types
TypeScript has several built-in utility types that can be invaluable when working with complex data structures. For instance, Partial<T>
, Pick<T, K>
, and Record<K, T>
can assist in shaping our API responses or managing user inputs more effectively.
Example of Using Partial
Consider a scenario where we might want to update user details but do not require all properties. Here’s how Partial
can help:
async function updateUser(userId: number, userData: Partial<User>): Promise<User> {
const response = await fetch(`/api/users/${userId}`, {
method: 'PUT',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(userData),
});
if (!response.ok) {
throw new Error('Failed to update user');
}
return response.json();
}
By using Partial<User>
, we can pass any subset of User
properties, ensuring our API is flexible and easy to use while remaining type-safe.
For further exploration of these utility types, check out the article on Mastering TypeScript Utility Types for Cleaner Code.
Final Considerations
By combining Java's type safety with TypeScript's expressive type system, we can build applications that are both robust and maintainable. The interplay between these two languages not only ensures clear contracts between client and server but also leverages type-checking to catch errors early in the development cycle.
As development continues to evolve, understanding and utilizing both technologies can lead to cleaner, safer, and more effective applications. Embrace TypeScript alongside Java, and you'll experience the full power of type safety in your projects.
Key Takeaways
- Type Safety: Reduces potential errors and improves maintainability.
- Integration: Leveraging TypeScript with Java can enhance API communication.
- Utility Types: Built-in features in TypeScript can help create cleaner and more dynamic code structures.
By employing TypeScript in your Java applications, you can harmonize the strengths of both languages, leading to a smoother development experience.
Checkout our other articles