Decoding Idioms: Boost Your Java Application's Readability
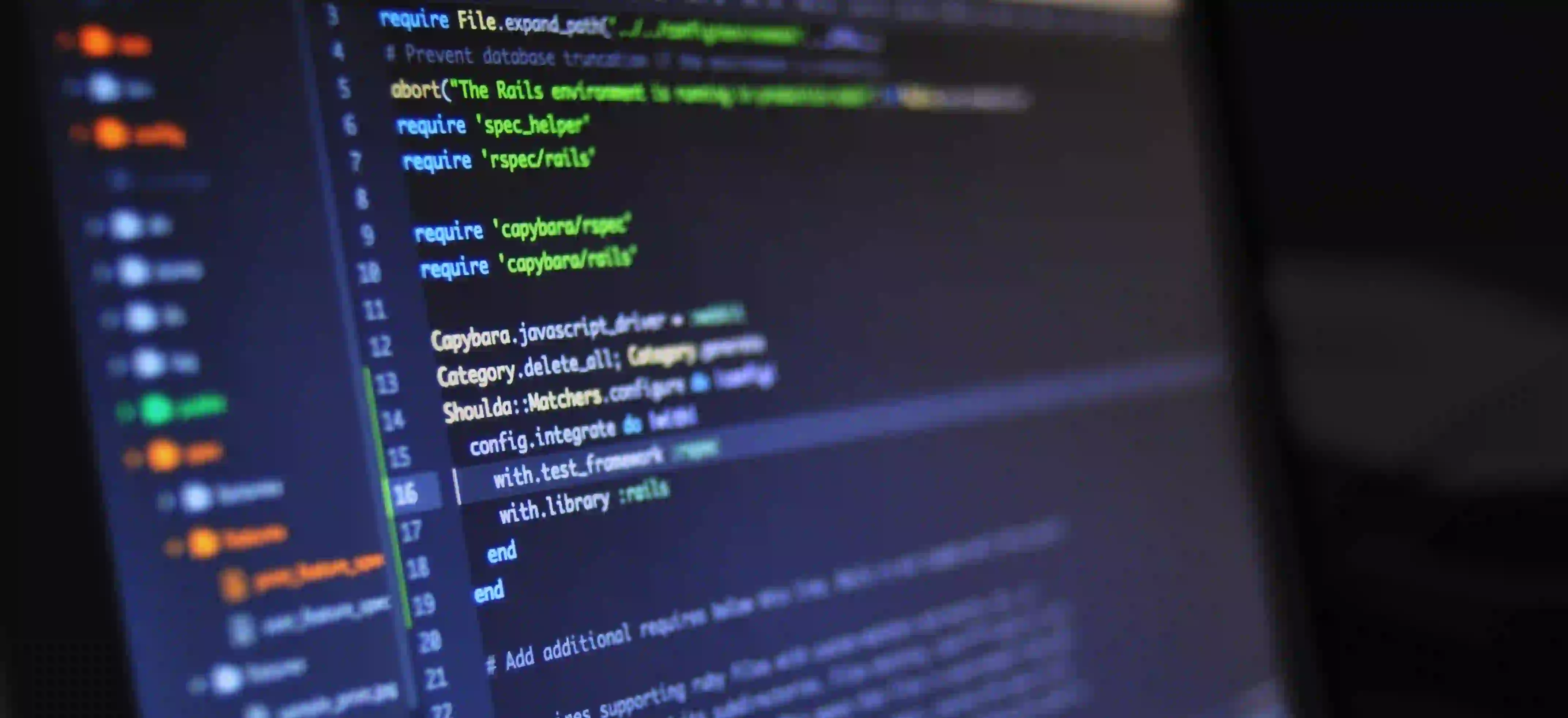
Decoding Idioms: Boost Your Java Application's Readability
In the world of software development, writing readable and maintainable code is as important as the functionality itself. Java, being a widely-used programming language, provides many opportunities to improve code readability, especially through the use of idioms. In this blog post, we will explore the concept of idioms in programming, their significance in Java, and how they can help you produce clearer, more efficient applications.
What Are Programming Idioms?
Programming idioms refer to patterns, techniques, and structures that convey specific meanings within a programming context. Just as idioms in language provide succinct expressions for complex ideas, programming idioms enable developers to communicate more effectively through their code.
For instance, consider the idiom "don't repeat yourself" (DRY). This principle encourages programmers to reduce repetition within their code, leading to easier updates and fewer errors. Another idiom is the use of "behaviors" and "protocols" in object-oriented programming (OOP), which helps to encapsulate functionalities effectively.
By utilizing these idioms, programmers can enhance the clarity and purpose of their code, making it easier for others (or themselves in the future) to read, understand, and maintain.
The Importance of Readability in Java
Readability is a vital aspect of programming that contributes to maintainability. When code is readable, it significantly reduces the cognitive load for developers, allowing them to comprehend complex systems with greater ease. The benefits of readability in Java include:
- Easier collaboration: Teams can work together efficiently when the code is clear.
- Simplified debugging: Readable code can help developers quickly pinpoint issues.
- Long-term maintainability: Future code updates become simpler and less error-prone.
This aligns with some of the ideas discussed in an article titled Geheimcode Englisch: Die faszinierende Welt der Idiome, which touches on how idioms embody complex ideas succinctly, much like how programming idioms serve a similar purpose in software development.
Leveraging Java Idioms for Readability
To improve the readability of a Java application, you can adopt several idioms that emphasize clarity and purpose in your code. Below is a collection of several key idioms, along with code snippets and explanations.
1. The Builder Pattern
The Builder Pattern is a creational design pattern that allows for the step-by-step construction of complex objects. This idiom is useful in cases where a class needs to be instantiated with many optional parameters.
Code Example
public class House {
private final int bedrooms;
private final boolean hasPool;
private final int floors;
private House(Builder builder) {
this.bedrooms = builder.bedrooms;
this.hasPool = builder.hasPool;
this.floors = builder.floors;
}
public static class Builder {
private int bedrooms;
private boolean hasPool;
private int floors;
public Builder(int bedrooms) {
this.bedrooms = bedrooms;
}
public Builder pool(boolean hasPool) {
this.hasPool = hasPool;
return this;
}
public Builder floors(int floors) {
this.floors = floors;
return this;
}
public House build() {
return new House(this);
}
}
}
Why This Matters:
Using the Builder Pattern improves code readability by clearly indicating how a House object is constructed. Each builder method conveys a specific intent, making it clear what properties you are setting. This reduces the need for constructors with numerous parameters and lowers the risk of constructing an object with incorrect states.
2. The Single Responsibility Principle
This principle advocates that a class should have one, and only one, reason to change. Adhering to this concept not only enhances readability but also improves maintainability.
Code Example
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUser(int id) {
return userRepository.findById(id);
}
}
public class UserRepository {
// Methods to interact with database
public User findById(int id) {
// Fetch user from the database
}
}
Why This Matters:
In this code snippet, the UserService
is solely focused on business logic related to the user, while UserRepository
handles data access. This clear separation of concerns improves readability. If changes in the data layer arise, you can modify the UserRepository
without affecting service logic.
3. Use of Optional
Java provides the Optional
class as a way to express the possibility of a value being absent, reducing the risk of null pointer exceptions.
Code Example
import java.util.Optional;
public class UserService {
private UserRepository userRepository;
public Optional<User> getUserById(int id) {
return Optional.ofNullable(userRepository.findById(id));
}
}
Why This Matters:
Using Optional
clarifies the possibility of absence in return values. This way, the calling code must explicitly handle the case of no value being present, which promotes better design and readability. No more hidden null checks!
4. Favoring Composition Over Inheritance
When designing systems, prefer composition as a means of achieving code reuse. Composition is easier to manage and enhances code organization.
Code Example
interface Payable {
void pay();
}
class Salary implements Payable {
public void pay() {
System.out.println("Salary has been paid.");
}
}
class Contract implements Payable {
public void pay() {
System.out.println("Contractor payment has been made.");
}
}
class Payroll {
private final List<Payable> payables;
public Payroll(List<Payable> payables) {
this.payables = payables;
}
public void processPayments() {
for (Payable payable : payables) {
payable.pay();
}
}
}
Why This Matters:
By using interfaces to define behavior and planning for composition, we can easily add new payment types without modifying existing code. This design increases modularity and keeps systems scalable while enhancing readability.
Lessons Learned
As we've discussed, idioms are essential tools that Java developers can leverage to improve code readability. Through patterns like the Builder Pattern, applying the Single Responsibility Principle, utilizing Optional
, and favoring composition over inheritance, you can create clear, concise, and maintainable Java applications.
By applying these principles, your Java applications won't just possess functionality; they will stand as exemplars of clean, easily navigable code. Remember, the easier your code is to understand, the easier it will be to maintain and expand in the future.
Let us strive to make our code not just work, but read well—a commitment that brings value not just to ourselves but to our entire development community.
For further insights about idioms in language and how they parallel programming concepts, check out Geheimcode Englisch: Die faszinierende Welt der Idiome. Happy coding!