Decoding Dreams: Insights for Java Developers' Logic
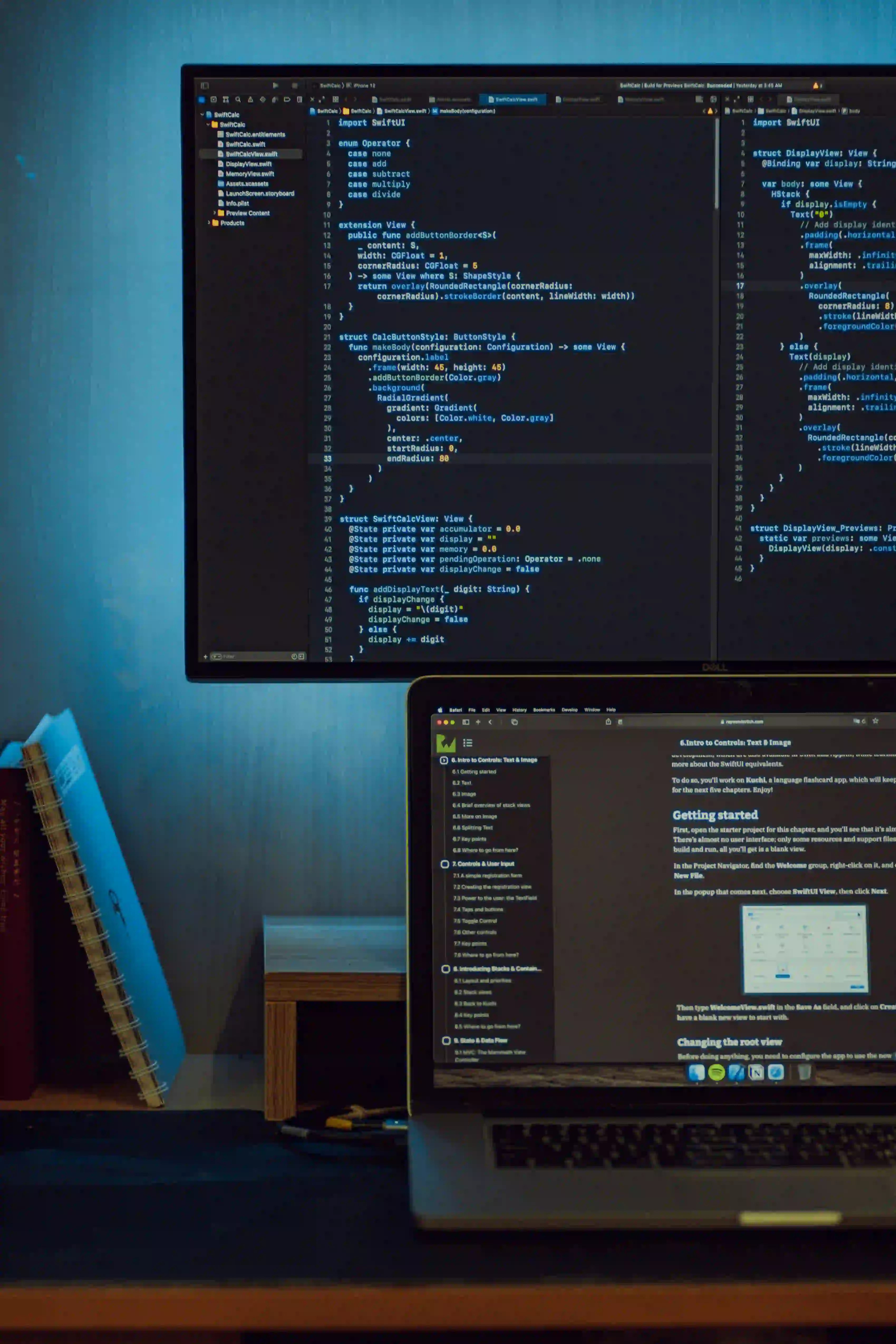
Decoding Dreams: Insights for Java Developers' Logic
The world of dreams fascinates many people, dipping into the unknown layers of our subconscious. This captivating domain reminds us of the complexities we handle as Java developers. Just like interpreting a dream can lead to revelations about ourselves, understanding Java intricacies leads to enlightenment in coding practices. This blog post will explore parallels between dream decoding and Java programming, providing practical insights and examples to boost your coding effectiveness.
When we think about dreams, we reference the understanding of symbols and structures. Similarly, in Java programming, a coherent structure and precise symbolism (data types, methods, etc.) are essential for writing effective code. Your programming logic, much like the logic behind dreams, can be deciphered with diligence and practice.
Understanding Logic and Structure in Java
In Java, structuring your program is similar to creating a framework for understanding dreams. You establish a foundation where components interact seamlessly. Let's uncover a few principles that will enhance your Java coding logic.
1. Classes and Objects: The Building Blocks
At the heart of object-oriented programming (OOP) in Java are classes and objects. Much like how dreams often have various characters and plots, in Java, classes serve as blueprints for creating objects.
Here's a simple example to illustrate this:
class Dream {
private String description;
private String emotion;
public Dream(String description, String emotion) {
this.description = description;
this.emotion = emotion;
}
public void interpret() {
System.out.println("Interpreting dream: " + description + " with emotion: " + emotion);
}
}
public class DreamInterpreter {
public static void main(String[] args) {
Dream myDream = new Dream("Flying over mountains", "Elation");
myDream.interpret();
}
}
Commentary:
- The Dream class encapsulates the dream's properties (description and emotion).
- The interpret() method evaluates these properties. This is your utility function to clarify dream symbolism.
- The process of instantiating the myDream object illustrates how structured logic forms a cohesive program.
2. Inheritance: Building Relationships
Much like recognizing how different dreams influence one another, Java's inheritance feature allows us to create new classes based on existing ones. This establishes relationships and enhances code reuse.
class Nightmares extends Dream {
private String fear;
public Nightmares(String description, String emotion, String fear) {
super(description, emotion);
this.fear = fear;
}
public void warn() {
System.out.println("Nightmare alert! Fear: " + fear);
}
@Override
public void interpret() {
super.interpret();
warn();
}
}
public class NightmareTester {
public static void main(String[] args) {
Nightmares myNightmare = new Nightmares("Falling into a void", "Fear", "Loss of control");
myNightmare.interpret();
}
}
Commentary:
- Here, Nightmares extends the Dream class, inheriting its features but also adding unique characteristics such as fear.
- The overridden interpret() method showcases polymorphism—adapting the existing function enhances your application's logic.
- This mirrors how dreams evolve from familiar feelings or experiences into more convoluted narratives.
3. Interfaces: The Intersection of Ideas
Interfaces in Java often represent common behaviors, somewhat like common themes found in dreams. They allows us to develop disparate classes to share methods without sharing a strict class structure.
interface Interpretable {
void interpret();
}
class AbstractDream implements Interpretable {
private String description;
public AbstractDream(String description) {
this.description = description;
}
@Override
public void interpret() {
System.out.println("Interpreting abstract dream: " + description);
}
}
public class DreamAnalyzer {
public static void main(String[] args) {
Interpretable myDream = new AbstractDream("Being chased");
myDream.interpret();
}
}
Commentary:
- Interpretable defines a contract influencing how dreams are processed.
- AbstractDream implements this interface, emphasizing flexibility—this mirrors how dreams can connect disparate themes or thoughts.
- Utilizing interfaces elevates coding efficiency and promotes collaboration among different program elements.
Decoding Dreams: Bridging Psychology and Java Logic
As highlighted in the article, "Träume entziffern: Tor zum geheimnisvollen Innenleben", the interpretation of dreams provides insight into our underlying fears, desires, and motivations. Every element within a dream embodies a particular intention, much akin to how every line of code serves a specific purpose in programming.
Taken together, the principles we explored form the bedrock of effective coding logic.
4. Exception Handling: Managing Conflicts
In dreams, unexpected events often lead to unsettling feelings. Likewise, managing exceptions in Java prevents disruptions in your application flow. Proper handling of exceptions can elevate your coding practice by creating robustness within your applications.
public class DreamException extends Exception {
public DreamException(String message) {
super(message);
}
}
public class DreamValidator {
public void validateDream(String dream) throws DreamException {
if (dream == null || dream.isEmpty()) {
throw new DreamException("Dream cannot be null or empty!");
}
System.out.println("Dream is valid: " + dream);
}
public static void main(String[] args) {
DreamValidator validator = new DreamValidator();
try {
validator.validateDream("");
} catch (DreamException e) {
System.out.println(e.getMessage());
}
}
}
Commentary:
- DreamException helps represent specific error scenarios.
- Validation prevents undesirable states in your program, much as reflecting on dreams helps avoid repeating undesirable experiences.
- Utilizing try-catch blocks promotes clean error handling, enhancing the overall program stability.
5. Unit Testing: Validating Our Interpretations
Finally, just as every interpretation of a dream is subjective, developers must validate their code to ensure it performs as expected. This is where JUnit comes into play for unit testing in Java.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class DreamTest {
@Test
public void testDreamInterpretation() {
Dream myDream = new Dream("Flying", "Joy");
String expectedOutput = "Interpreting dream: Flying with emotion: Joy";
assertEquals(expectedOutput, myDream.interpret());
}
}
Commentary:
- Unit tests substantiate and validate your interpretations (code) by ensuring they yield consistent results.
- The test evaluates the interpret() method to confirm that it produces the correct output, mirroring how revisiting dreams can validate our understanding or change our perception.
The Last Word
Decoding dreams and coding in Java share profound levels of complexity and structure. By employing the discussed principles—classes, inheritance, interfaces, exception handling, and unit testing—you can refine your coding approach, much like honing your dream interpretation skills. Ultimately, the practice of dissecting both layers can lead to self-improvement and mastery.
Next time you drift into the realm of dreams, reflect on how you can similarly decode complexity in your Java applications. Embrace these programming philosophies, and watch as your proficiency expands, unlocking new dimensions in coding.