Unlocking Java: Mastering Idiomatic Expressions in Code
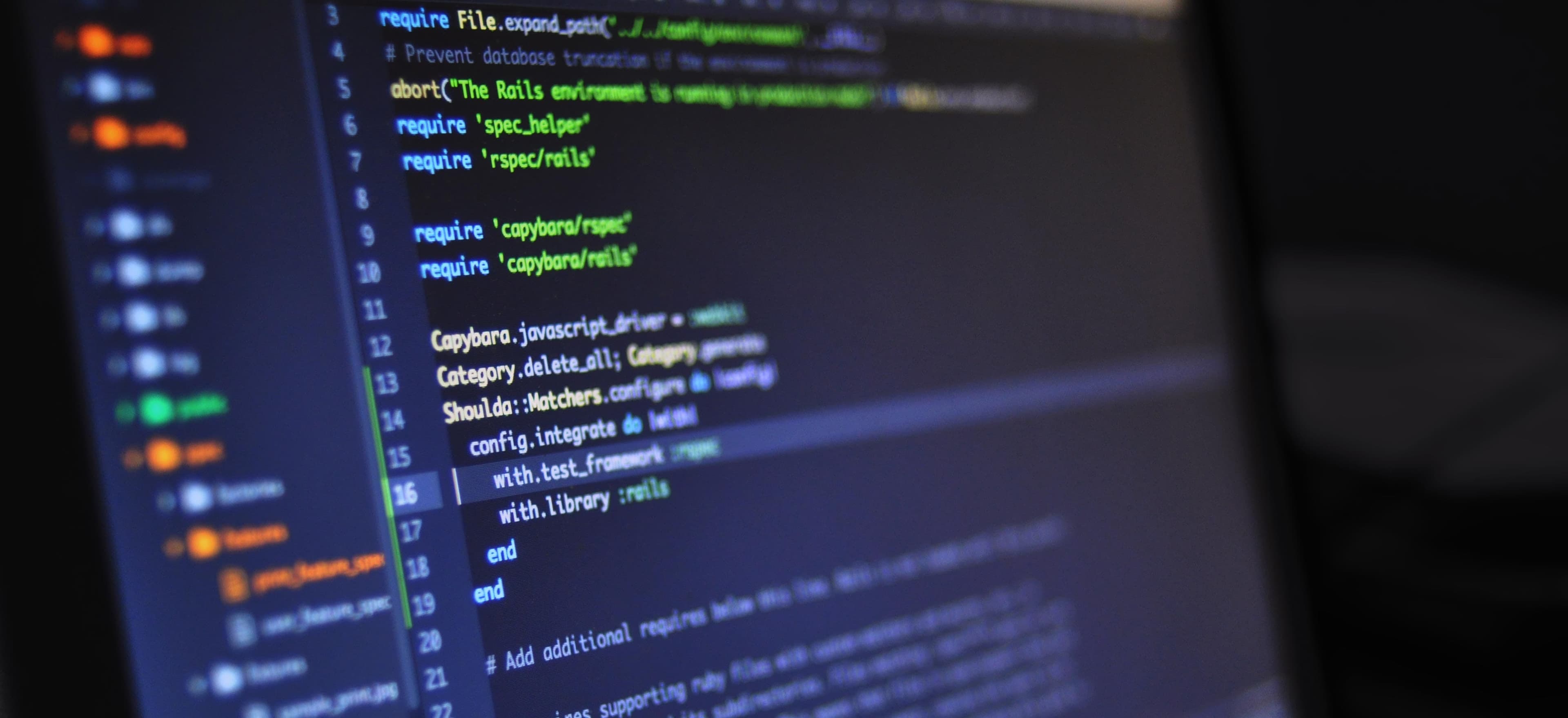
- Published on
Unlocking Java: Mastering Idiomatic Expressions in Code
As any seasoned Java developer will tell you, understanding the idioms of the language can take your coding skills from average to extraordinary. Just as idioms in spoken language convey meaning beyond the literal, Java's idiomatic expressions enhance code clarity, maintainability, and performance. This blog post aims to unravel some of the most crucial Java idioms and express how they can significantly improve your coding practices.
What Are Idiomatic Expressions in Java?
Simply put, idioms in Java are established patterns or styles of coding that are widely accepted by the Java community. They enable developers to write code that is not only functional but also clean and efficient. These idioms are usually born out of common practices and are the result of many developers collaborating and sharing knowledge over the years.
Let’s explore some essential idiomatic expressions in Java, along with practical examples to illustrate their importance.
1. The Builder Pattern
The builder pattern is a well-known architectural design pattern used in Java. It simplifies object creation and is particularly useful for constructing complex objects step by step.
Why Use the Builder Pattern?
- Readability: It makes the code easier to read.
- Flexibility: New parameters can be added without changing existing code.
- Immutability: The constructed object can often be made immutable.
Example
public class User {
private final String username;
private final String email;
private final int age;
private User(UserBuilder builder) {
this.username = builder.username;
this.email = builder.email;
this.age = builder.age;
}
public static class UserBuilder {
private String username;
private String email;
private int age;
public UserBuilder(String username) {
this.username = username;
}
public UserBuilder withEmail(String email) {
this.email = email;
return this;
}
public UserBuilder withAge(int age) {
this.age = age;
return this;
}
public User build() {
return new User(this);
}
}
@Override
public String toString() {
return "User{" + "username='" + username + '\'' + ", email='" + email + '\'' + ", age=" + age + '}';
}
}
In this example, we’ve created a User
class with a nested UserBuilder
. The builder pattern provides a flexible and readable way to construct user objects, allowing you to manage complex object creation without the need for numerous constructors.
2. The Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. This is particularly useful for managing resources such as configuration settings or connection pools.
Why Use the Singleton Pattern?
- Controlled Access to Resources: It helps in resource management.
- Global State: It provides a way to hold the state consistently throughout the application.
Example
public class ConfigManager {
private static ConfigManager instance;
private String configValue;
private ConfigManager() {
// Private constructor to prevent instantiation
configValue = "Default Config";
}
public static ConfigManager getInstance() {
if (instance == null) {
instance = new ConfigManager();
}
return instance;
}
public String getConfigValue() {
return configValue;
}
public void setConfigValue(String configValue) {
this.configValue = configValue;
}
}
By implementing the Singleton pattern as shown above, we ensure that there is only one instance of ConfigManager
throughout the application. This makes configuration management centralized and less error-prone.
3. Using Streams for Data Processing
Java Streams provide a powerful way to work with sequences of data. They allow for functional-style operations and can significantly reduce the amount of boilerplate code required, making your code more concise and expressive.
Why Use Streams?
- Efficiency: Streams support lazy evaluation.
- Improved Readability: They can make data processing code much clearer.
- Composable: Stream operations can be easily chained.
Example
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
long count = names.stream()
.filter(name -> name.startsWith("C"))
.count(); // Counts names starting with 'C'
System.out.println("Count of names starting with 'C': " + count);
}
}
In this example, we count how many names start with the letter "C" using a stream. The benefit here is clarity; the intent is immediately clear, compared to traditional loops.
4. Enhanced For-Loop
The enhanced for-loop, introduced in Java 5, allows developers to iterate through collections without explicitly using an iterator. This can simplify the code, making it cleaner and easier to read.
Why Use the Enhanced For-Loop?
- Simplifies iteration: Reduces boilerplate code.
- Increased clarity: Makes the code more understandable.
Example
import java.util.Arrays;
import java.util.List;
public class EnhancedForLoopExample {
public static void main(String[] args) {
List<String> fruits = Arrays.asList("Apple", "Banana", "Cherry");
for (String fruit : fruits) {
System.out.println(fruit);
}
}
}
Using the enhanced for-loop here allows us to iterate through the list of fruits effortlessly, improving readability without sacrificing functionality.
The Closing Argument
Mastering idiomatic expressions in Java is essential for writing clean, efficient, and maintainable code. Patterns like the Builder, Singleton, using Streams, and the Enhanced For-Loop are just a few among many. Understanding and applying these idioms will undoubtedly contribute to your growth as a developer.
For more insights into the language of programming, the idioms of spoken language, and how they shape communication, you might find it interesting to read the article titled Geheimcode Englisch: Die faszinierende Welt der Idiome. This exploration could further enhance your appreciation for languages, both natural and programming.
Happy coding!
Checkout our other articles