Avoid Performance Pitfalls: jQuery Loops in Java Code
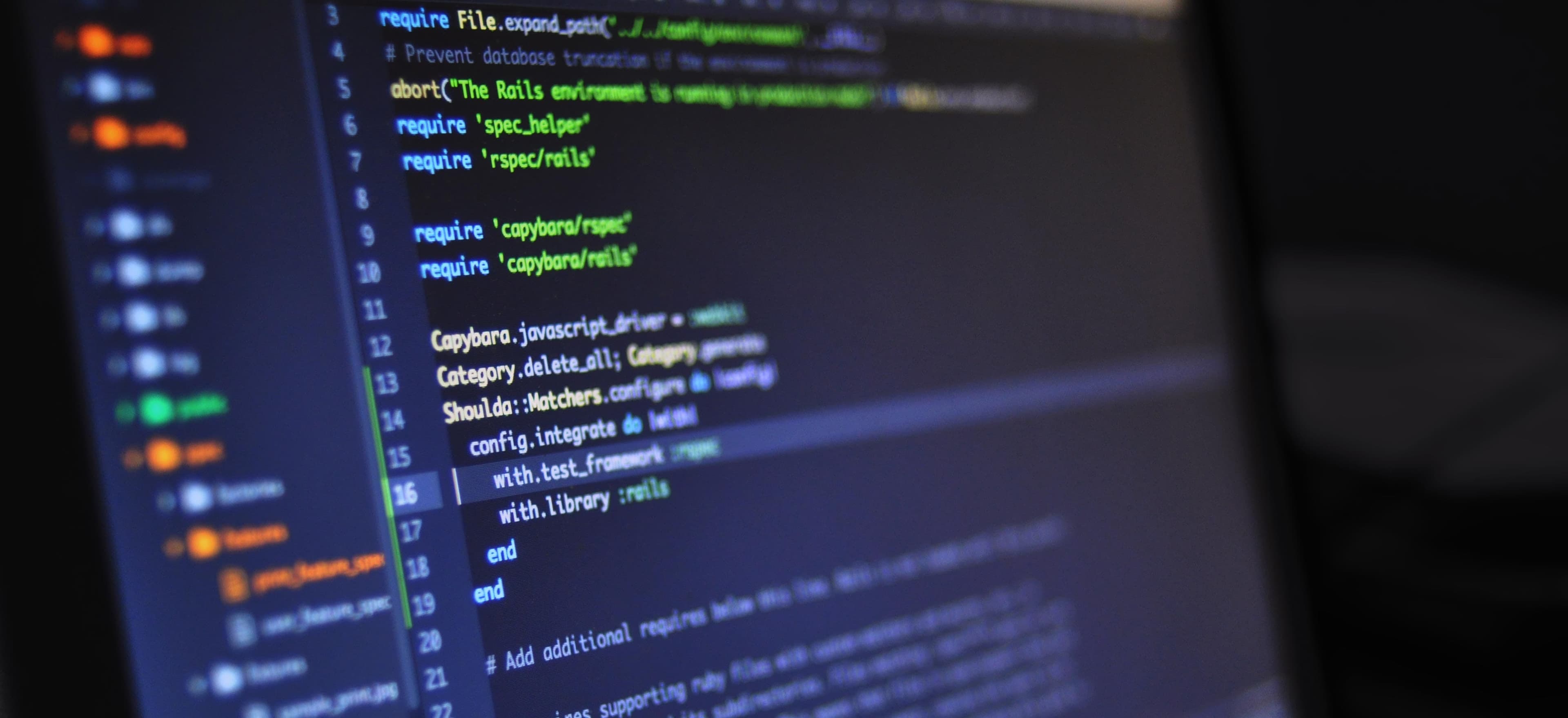
- Published on
Avoid Performance Pitfalls: jQuery Loops in Java Code
When we talk about modern web development, efficiency and performance are crucial. With the rise of Java as a backend technology and jQuery as a popular JavaScript library for front-end development, developers often find themselves integrating both. However, there are common performance pitfalls when looping jQuery functions that can lead to inefficient applications. In this post, we will dive into the intricacies of using jQuery functions in loops within a Java application context and explore ways to enhance performance.
Understanding jQuery Functions
Before we go deep into loops and performance, it's essential to understand what jQuery functions are. jQuery is a fast, small, and feature-rich JavaScript library that simplifies things like HTML document traversal and manipulation, event handling, and animation. This allows developers to write less code while achieving more.
While jQuery is powerful, its performance can suffer when used inefficiently, especially inside loops. Let's examine this further.
Common Performance Pitfalls
-
Repeated DOM Access: One of the most significant issues encountered is repeated access to the DOM within loops. Every time you call a jQuery selector, it has to search through the DOM, which can be time-consuming.
-
Inefficient Event Binding: Binding the same event handler multiple times within a loop can lead to performance degradation. Each event binding creates a new instance of the handler, eating into resources.
-
Callback Hell: Nesting multiple callbacks inside loops can create hard-to-read code. This makes debugging and maintaining your application more complicated.
To get a clearer picture of performance issues, it's worth exploring the insights provided in the article titled Common Mistakes When Using jQuery Functions in Loops.
Example Scenario: DOM Manipulation
Let’s illustrate the problem with a real-world example: Suppose you want to update multiple elements on the page based on certain criteria. Here’s an example of how to do this inefficiently:
// Inefficient jQuery loop
$('.items').each(function() {
if ($(this).text() === "Target") {
$(this).addClass('highlight');
$(this).css('color', 'red');
}
});
Why This is Inefficient
- Multiple DOM Access: The code accesses the DOM elements multiple times within the loop, which is expensive.
- Repeated jQuery Calls: Each call to $(this) results in a search through the DOM.
Better Approach
We can optimize this by caching the jQuery selectors and making fewer DOM manipulations:
// Efficient jQuery loop
var $items = $('.items'); // Cache the jQuery selector
$items.each(function() {
var $this = $(this); // Cache this instance of jQuery object
if ($this.text() === "Target") {
$this.addClass('highlight')
.css('color', 'red'); // Chain methods for cleaner code
}
});
Explanation
In the optimized version, we:
- Cached the Selector: By caching
$('.items')
, we avoid searching the DOM multiple times. - Minimized jQuery Calls by Using a Variable: The variable
$this
points to the current item in the loop, allowing us to minimize additional DOM access.
Using Delegate Events
Instead of binding events inside a loop, consider event delegation. Here’s an example:
Problematic Event Binding
$('.items').each(function() {
$(this).on('click', function() {
alert('Item clicked');
});
});
Optimized Event Delegation
$('.items').on('click', function() {
alert('Item clicked');
});
Why is This Better?
Using event delegation minimizes the number of event handlers that are created. Instead of having one handler for each .item
, you only have one handler for the entire set. This is particularly beneficial for dynamic lists where items may be added or removed.
Performance Implications
By optimizing how we use jQuery functions in loops, we improve performance in several ways:
- Reduced Latency: Fewer DOM manipulations lead to faster rendering.
- Lower Memory Usage: Less memory is used for event handlers.
- Cleaner Code: It leads to more maintainable and readable code.
Profiling Performance Bottlenecks
Tools like Chrome DevTools can help you profile performance issues in your JavaScript code, including jQuery usage. Follow these steps:
- Open Chrome DevTools.
- Navigate to the "Performance" tab.
- Start a recording, then perform the actions you want to test.
- Stop recording and analyze the results to find bottlenecks related to jQuery loops.
To Wrap Things Up
Efficiently using jQuery functions in your Java code is essential for delivering high-performance web applications. Caching selectors, minimizing DOM access, and using event delegation are just a few strategies to avoid common pitfalls. Remember, the aim is to write clean, efficient code that maintains high performance while enhancing user experience.
By changing how we approach jQuery loops, we can create robust applications without sacrificing performance. For more insights on jQuery loops and pitfalls, check out the article on Common Mistakes When Using jQuery Functions in Loops.
Explore the vast world of optimization and make your applications shine! Happy coding!
Checkout our other articles