Avoiding Loop Pitfalls: Java Alternatives to jQuery Mistakes
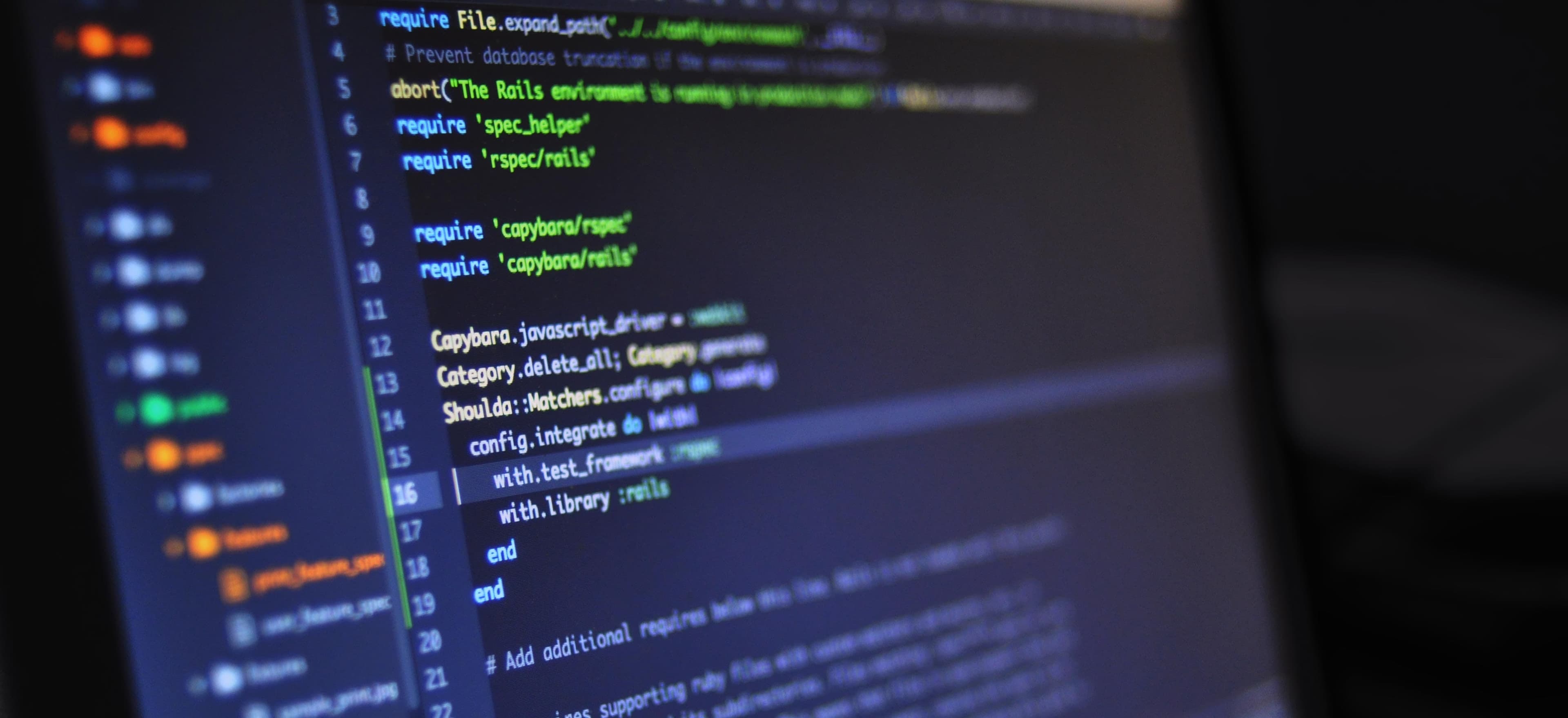
- Published on
Avoiding Loop Pitfalls: Java Alternatives to jQuery Mistakes
When it comes to web development, JavaScript, particularly libraries and frameworks like jQuery, play a crucial role. However, as you advance in your programming journey, you may encounter various pitfalls associated with the misuse of jQuery functions in loops. If you are transitioning to Java or integrating Java with your web applications, understanding these pitfalls can enhance your coding practices and optimize performance.
In this article, we'll delve into common mistakes when using jQuery functions in loops, comparing them to efficient alternatives in Java. We aim to enhance your understanding of both languages while avoiding costly errors in your development workflow.
Overview of Common Pitfalls in jQuery Loops
A comprehensive discussion on the deficiencies in using jQuery functions within loops can be found in the article Common Mistakes When Using jQuery Functions in Loops. The article highlights that crucial oversights such as redundant DOM queries, poor performance with large datasets, and memory usage issues can lead to significant slowing down of your web applications.
Key Pitfalls to Avoid
- Redundant DOM Manipulations: Accessing items in the DOM repeatedly inside a loop.
- Neglecting Event Delegation: Binding events on every iteration instead of using event delegation.
- Overusing jQuery Functions: The performance overhead associated with methods like
.css()
and.html()
inside loops.
Why Java?
Java, being a statically typed language, provides a different approach to loops and operations. Its structured nature encourages developers to write code that is not only clean but also efficient. Let’s explore some Java alternatives to combat the pitfalls encountered in jQuery.
Java Loop Structures
Java offers several loop constructs that can be utilized for data processing without falling into the same traps that can ensnare a jQuery developer. Common structures include:
for
loopwhile
loop- Enhanced
for
loop (for-each)
1. The Standard For Loop
Java’s traditional for
loop allows you to enumerate through a collection, offering a straightforward mechanism to avoid costly repeated operations.
List<String> items = Arrays.asList("Item1", "Item2", "Item3", "Item4");
for (int i = 0; i < items.size(); i++) {
String currentItem = items.get(i);
// Process each item - here we could perform an operation like logging the item.
System.out.println(currentItem);
}
Why This Works: This code snippet demonstrates how to iterate through a list effectively. The use of get(i)
accesses elements without repeated queries, in contrast to DOM manipulations in jQuery.
2. While Loop Usage
While loops can also be employed effectively, especially when you do not know the number of iterations in advance.
int counter = 0;
while (counter < items.size()) {
System.out.println(items.get(counter));
counter++;
}
Efficiency Note: Similar to the for
loop, this maintains low overhead by avoiding complex operations inside the loop.
3. Enhanced For Loop
The enhanced for
loop offers a simplified approach, automatically iterating through all items.
for (String item : items) {
System.out.println(item);
}
Simplicity in Action: The enhanced for
loop is particularly useful for readability while maintaining performance.
Event Handling: Java's Approach
In Java, event handling takes on a different structure. While jQuery allows binding events in a repetitive manner, Java requires you to attach listeners once, similar to event delegation in jQuery.
Event Delegation Example in Java
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
public class MyFrame {
public static void main(String[] args) {
JFrame frame = new JFrame("Event Delegation");
JButton button = new JButton("Click Me");
// Event listener is added only once
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button Clicked!");
}
});
frame.add(button);
frame.setSize(300, 200);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
Why It Matters: This code snippet illustrates how to manage events efficiently without the pitfalls of excessive event binding.
Performance Considerations
When comparing performance, it's vital to recognize that Java compiles to bytecode, which is executed by the Java Virtual Machine (JVM), offering benefits in terms of optimization. In contrast, repeated DOM manipulations in jQuery can lead to slower performance, especially with large datasets.
Memory Management
Java has built-in garbage collection, which can help manage memory effectively compared to the frequent memory allocations that occur when repeating DOM operations using jQuery.
To Wrap Things Up
Transitioning from jQuery to Java, or utilizing both, requires an understanding of the best practices that mitigate common pitfalls. As showcased, using Java’s structured loop constructs and event handling paradigms can lead to more efficient and maintainable code.
By avoiding redundant operations and unnecessary complexities, you not only enhance the performance of your Java applications but also harness the underlying advantages of both languages—ensuring your web applications are responsive and efficient.
For an extensive understanding of the pitfalls in jQuery when using loops, feel free to visit the article Common Mistakes When Using jQuery Functions in Loops.
In your journey as a developer, consider these strategies, and embrace the power of Java to avoid loop pitfalls effectively. Happy coding!
Checkout our other articles