Common Pitfalls When Deploying Java AWS Lambda Functions
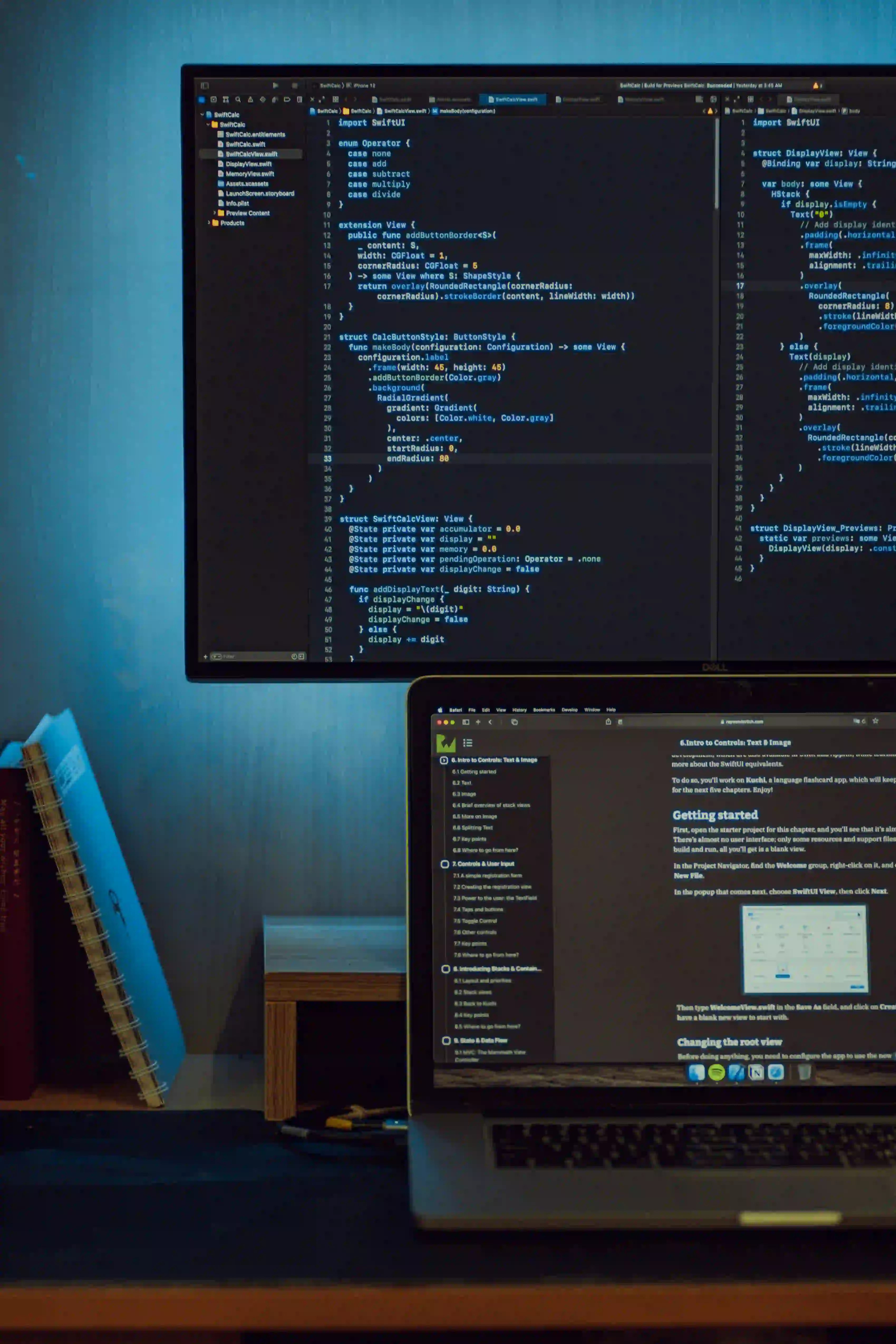
Common Pitfalls When Deploying Java AWS Lambda Functions
Deploying Java applications on AWS Lambda can significantly improve scalability and reduce operational overhead. However, it comes with its own set of challenges. In this post, we will discuss the common pitfalls developers encounter when using Java in AWS Lambda and how to avoid them.
Table of Contents
- Understanding AWS Lambda
- Pitfall 1: Cold Starts
- Pitfall 2: Package Size
- Pitfall 3: Memory Configuration
- Pitfall 4: Poor Dependency Management
- Pitfall 5: Exception Handling
- Conclusion
Understanding AWS Lambda
AWS Lambda is a serverless computing service that enables you to run code in response to events without provisioning or managing servers. Java, being a versatile and powerful programming language, is often used to build Lambda functions. However, developers often overlook certain aspects that can lead to suboptimal performance or functionality.
Pitfall 1: Cold Starts
What is a Cold Start?
Cold starts occur when a Lambda function is invoked but there's no pre-existing instance of that function running. This forces AWS Lambda to allocate resources and initialize the function, resulting in a delay. For production-level applications, this time lag can severely impact performance.
Solutions:
-
Minimize Dependencies: Keep your deployment package lightweight by including only the essential dependencies.
☕snippet.java// Add only necessary libraries dependencies { implementation 'com.amazonaws:aws-java-sdk-s3:1.11.1000' // Avoid unnecessary libraries }
-
Provisioned Concurrency: Use Provisioned Concurrency to minimize cold start impacts. This feature allows you to pre-warm instances of your Lambda function.
Pitfall 2: Package Size
Why is Package Size Important?
AWS Lambda enforces limits on package size: 50 MB (compressed) for direct uploads and 250 MB when stored in S3. An oversized package may lead to increased cold start times or resource limitations.
Solutions:
-
Use the Lambda Layer: Share common libraries among multiple Lambda functions using Lambda Layers.
🔧snippet.shaws lambda publish-layer-version --layer-name my-layer --zip-file fileb://my-layer.zip
-
Remove Unused Files: Scan your project for unnecessary resources (e.g., test files, documentation, etc.) that aren’t needed in production.
Pitfall 3: Memory Configuration
Understanding Memory Settings
AWS Lambda allows you to set the memory allocation for each function between 128 MB to 10,240 MB. It's crucial to find the right balance between memory and performance; too little can lead to increased execution time, while too much can inflate costs.
How to Configure Properly:
- Benchmarking: Use AWS Lambda Power Tuning to identify the optimal memory settings based on execution time and cost.
aws lambda invoke --function-name my-function output.txt
- Environment Variables: Store memory and timeout settings in environment variables for easier modifications later.
String memory = System.getenv("MEMORY_SIZE");
Pitfall 4: Poor Dependency Management
Why Dependency Management Matters
Java applications can have multiple dependencies, making it crucial to manage them effectively. Poorly managed dependencies can lead to conflicts and increased build sizes.
Best Practices:
-
Use a Dependency Manager: Utilize tools like Maven or Gradle to manage and version dependencies efficiently.
📄snippet.txt<!-- Example Maven Dependency --> <dependency> <groupId>com.amazonaws</groupId> <artifactId>aws-java-sdk-lambda</artifactId> <version>1.11.1000</version> </dependency>
-
Shade Your Jars: Avoid conflicts by using the Maven Shade Plugin or Gradle Shadow Plugin.
📄snippet.txt<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-shade-plugin</artifactId> <version>3.2.4</version> <executions> <execution> <phase>package</phase> <goals> <goal>shade</goal> </goals> </execution> </executions> </plugin>
Pitfall 5: Exception Handling
Understanding Exception Handling
Not properly managing exceptions can lead to incomplete transactions and unhandled errors, impacting user experience and application integrity.
Best Practices:
-
Use Try-Catch: Always wrap critical code segments in try-catch blocks to handle exceptions gracefully.
☕snippet.javatry { // critical code } catch (Exception e) { System.err.println("Error: " + e.getMessage()); throw new RuntimeException(e); }
-
Custom Exception Classes: Define custom exceptions to throw specific errors, making it easier to debug.
public class CustomLambdaException extends RuntimeException {
public CustomLambdaException(String message) {
super(message);
}
}
-
CloudWatch Logs: Utilize AWS CloudWatch to monitor logs and set alerts for critical errors.
☕snippet.java// Log error message AWSLogs logger = AWSLogsClient.builder().build(); logger.putLogEvents(/* log event details */);
Lessons Learned
Deploying Java AWS Lambda functions is not without its challenges. By understanding the common pitfalls, such as cold starts, package size, memory configuration, dependency management, and exception handling, you can significantly improve your Lambda function's reliability and performance.
Keep these best practices in mind, and you will be better prepared to harness the full power of AWS Lambda in your Java applications. As you explore further, consider integrating other AWS services such as DynamoDB and S3 to develop more robust serverless architectures.
For further reading, refer to the following resources:
- AWS Lambda Documentation
- Java on AWS Articles
- Serverless Framework Documentation
By proactively addressing these common pitfalls, you can ensure a smoother deployment process, enhanced application performance, and ultimately a more successful project.