Overcoming Common Challenges in Data-Driven Refactoring
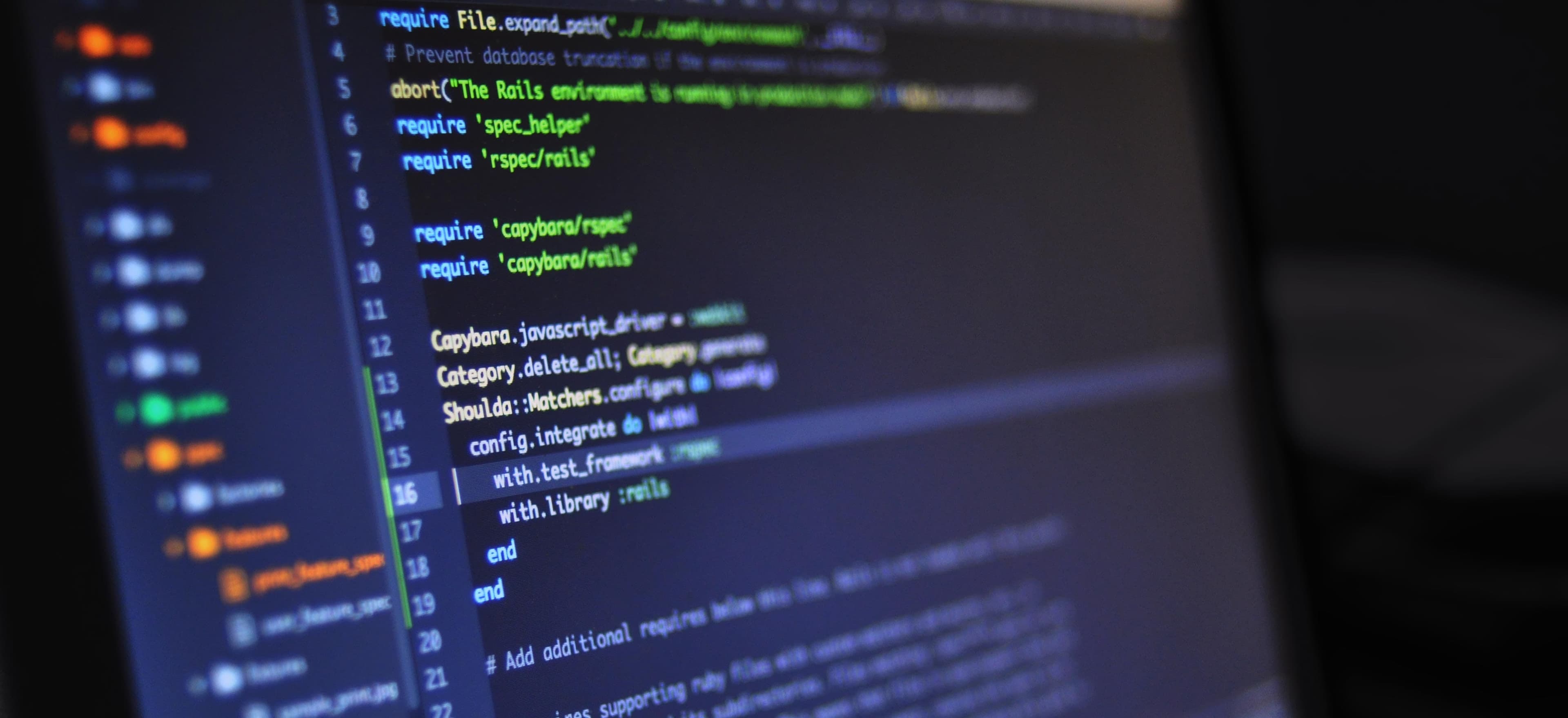
- Published on
Overcoming Common Challenges in Data-Driven Refactoring
In the dynamic world of software development, maintaining a clean codebase is paramount. As applications evolve, refactoring emerges as a key strategy to improve code maintainability, performance, and readability. However, data-driven refactoring presents its own set of challenges. In this blog post, we'll delve into common challenges faced during data-driven refactoring and offer strategies for overcoming these hurdles effectively.
What is Data-Driven Refactoring?
Data-driven refactoring is an approach that utilizes data metrics to guide the refactoring process. It focuses on improving specific aspects of the code—such as code complexity, duplication, and performance—based on measurable data points. The goal is to enhance code structure and functionality while minimizing disruptions to the existing system.
The Importance of Data
Data serves as the foundation of this refactoring strategy. It offers insights into code quality and allows developers to make informed decisions. By leveraging metrics like cyclomatic complexity or code churn, teams can prioritize refactoring efforts effectively.
Common Challenges in Data-Driven Refactoring
1. Identifying Relevant Data Metrics
Challenge: Not all data is equally useful in guiding refactoring efforts. With a plethora of available metrics, determining which ones to focus on can be overwhelming.
Solution: Narrow down metrics to a handful that align with your project goals. Commonly used metrics include:
-
Cyclomatic Complexity: Measures the number of linearly independent paths through a program's source code. High values suggest complex code that may need simplification.
-
Code Duplication: Identifies repeated code fragments, which can lead to maintenance headaches. A lower duplication score indicates better maintainability.
Here’s a simple example of how to calculate cyclomatic complexity in Java:
public int calculateCyclomaticComplexity(Method method) {
int complexity = 1; // start with 1 for the method
for (String line : method.getLines()) {
if (isControlFlowStatement(line)) {
complexity++; // Increment for each control flow statement
}
}
return complexity;
}
// Helper method to check for control flow statements
private boolean isControlFlowStatement(String line) {
return line.contains("if") || line.contains("for") || line.contains("while");
}
Why: This code counts the number of control flow statements in a method, allowing developers to assess its complexity. By identifying methods with high cyclomatic complexity, teams can prioritize them for refactoring.
2. Resistance from Development Teams
Challenge: Developers often resist refactoring efforts due to the fear of introducing bugs or the belief that existing code works fine.
Solution: Foster a culture that prioritizes code quality. Regular code reviews and collaborative refactoring sessions can encourage team participation.
-
Education: Provide resources on the benefits of refactoring and share success stories.
-
Security in Testing: Implement automated testing beforehand to reassure team members that functionality can be preserved during refactoring.
3. Lack of Comprehensive Testing Frameworks
Challenge: Insufficient tests can make refactoring risky, as it's hard to ensure that changes won't break the existing functionality.
Solution: Invest in a robust testing framework that supports both unit tests and integration tests.
import org.junit.jupiter.api.*;
public class SampleClassTest {
@Test
public void testSampleMethod() {
SampleClass sample = new SampleClass();
int result = sample.sampleMethod();
Assertions.assertEquals(expectedValue, result);
}
}
Why: This JUnit example showcases a simple way to verify the correctness of your methods. By having a strong suite of tests, developers can refactor more confidently.
For in-depth testing strategies, check out Martin Fowler's Refactoring book.
4. Managing Dependencies
Challenge: In large codebases, refactoring can lead to unexpected cascading changes due to interdependencies among various modules and libraries.
Solution: Use tools that visualize code dependencies. This can help assess the impact of changes before undertaking refactoring.
-
Code Visualization Tools: Tools like SonarQube or JDepend can provide a visual representation of dependencies in your project.
-
Decoupling: Aim to design your application using principles of separation of concerns and modularization. This makes refactoring less daunting.
5. Balancing Refactoring with Feature Development
Challenge: Often, teams are pressured to prioritize new feature development over refactoring efforts. This can lead to technical debt.
Solution: Integrate refactoring into the regular development cycle. Dedicate a certain percentage of project time or resources to handle refactoring in tandem with feature development.
- Sprint Planning: Weight your sprints with a mix of new features and necessary refactoring tasks.
6. Measuring Success and Continuous Improvement
Challenge: After refactoring, measuring the success of your efforts can be tricky. Without clear metrics, it’s hard to assess improvements.
Solution: Define what success looks like before initiating refactoring. This could be lower cyclomatic complexity, reduced bug counts, or improved performance metrics.
Leverage Continuous Integration/Continuous Deployment (CI/CD) pipelines to automate testing and ensure that metrics are gathered consistently over time.
The Last Word
Data-driven refactoring can significantly improve the quality of a codebase, but teams must be aware of and strategically address the common challenges involved. By identifying relevant data metrics, fostering a culture supportive of refactoring, establishing robust testing frameworks, and balancing feature development with code maintenance, teams can navigate these obstacles more effectively.
Remember, the goal of data-driven refactoring isn't just to improve code—it’s to create a more sustainable, efficient, and productive development environment. For more insights on this topic, feel free to explore Refactoring Guru for techniques and patterns that can aid in your refactoring journey.
As you embark on your refactoring endeavors, keep in mind that consistent reflection and adaptation will drive continuous improvement—both in your code and your development processes. Happy refactoring!