Understanding the Limitations of Unmodifiable Collections in JDK 10
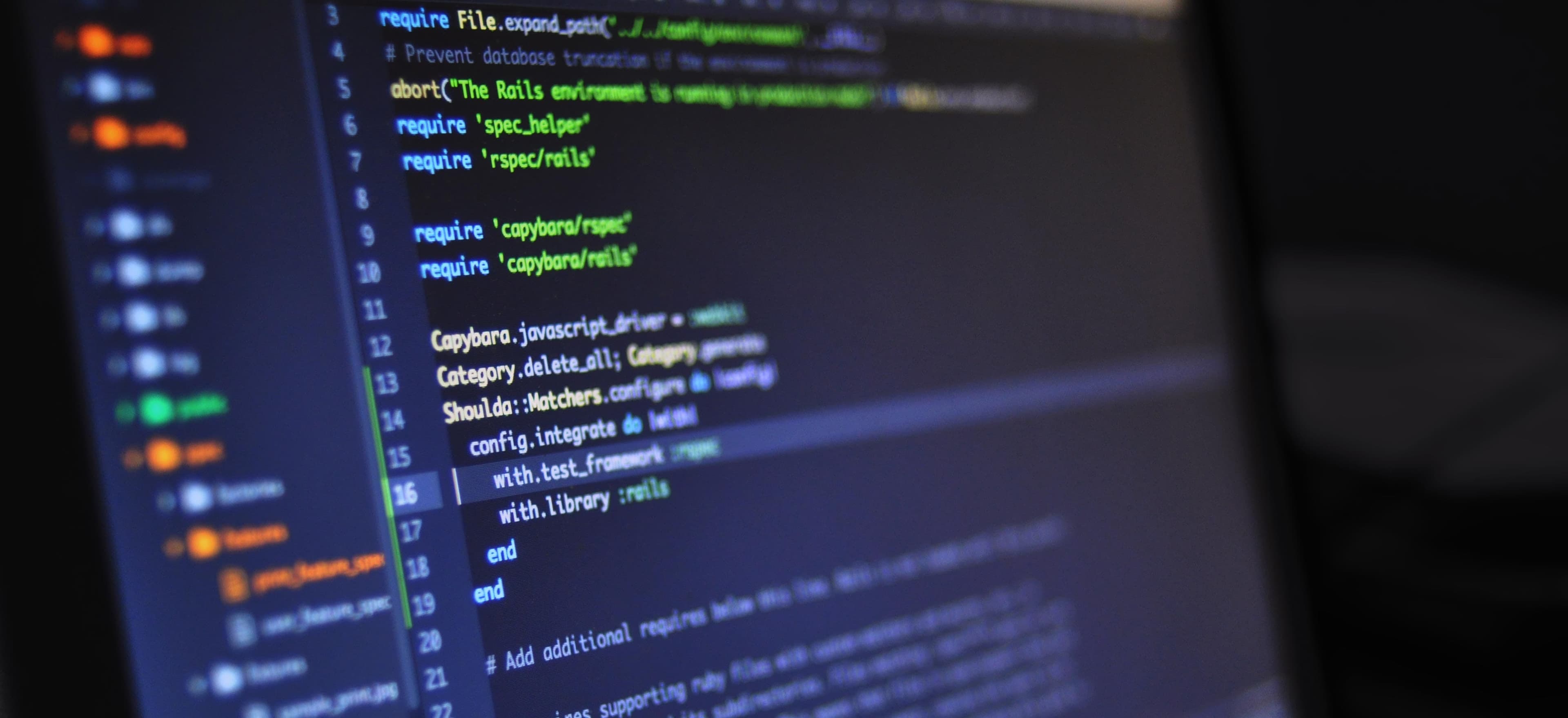
- Published on
Understanding the Limitations of Unmodifiable Collections in JDK 10
Java has come a long way since its inception, offering developers more robust tools for handling data structures efficiently. One significant advancement is the introduction of unmodifiable collections in the Java Development Kit (JDK). Introduced in Java 9, unmodifiable collections prove to be a useful feature for maintaining immutable data structures. However, it’s essential to understand their limitations, especially as programmers continue to adopt JDK 10 and beyond.
In this blog post, we will explore the concept of unmodifiable collections, demonstrate their usage with code snippets, discuss their limitations, and reinforce your understanding of when to utilize them effectively.
What are Unmodifiable Collections?
Unmodifiable collections are part of the Java Collections Framework. They are designed to prevent modification attempts on the collections they wrap, ensuring data integrity. The primary advantage is promoting a "read-only" approach, thereby reducing the risks associated with unintended modification, which can lead to bugs.
In JDK 10, the approach to creating unmodifiable collections remains similar to the way it was introduced in JDK 9. Let's take a closer look at how to create unmodifiable collections.
Creating Unmodifiable Collections
To create an unmodifiable collection, we can use helper methods from the Collections
class or the new static factory methods introduced in the List
, Set
, and Map
interfaces.
Example: Creating an Unmodifiable List
import java.util.Collections;
import java.util.List;
public class UnmodifiableListExample {
public static void main(String[] args) {
List<String> modifiableList = List.of("Apple", "Banana", "Cherry");
List<String> unmodifiableList = Collections.unmodifiableList(modifiableList);
System.out.println("Unmodifiable List: " + unmodifiableList);
// Attempting to modify the unmodifiableList will throw an exception
try {
unmodifiableList.add("Date");
} catch (UnsupportedOperationException e) {
System.out.println("Modification Attempt: " + e.getMessage());
}
}
}
Commentary:
- Creating an Unmodifiable List: We create a modifiable list and then wrap it with
Collections.unmodifiableList()
. - Attempting a Modification: Trying to add an element to the unmodifiable list will throw an
UnsupportedOperationException
, demonstrating that the collection is truly unmodifiable.
Limitations of Unmodifiable Collections
While unmodifiable collections are a valuable tool, they come with certain limitations that developers must be aware of:
1. Shallow Immutability
Unmodifiable collections are shallowly immutable. This means while the collection's reference cannot change, the objects referenced inside the collection can still be modified, if they are mutable.
import java.util.Collections;
import java.util.List;
public class ShallowImmutabilityExample {
public static void main(String[] args) {
List<StringBuilder> modifiableList = List.of(new StringBuilder("Hello"), new StringBuilder("World"));
List<StringBuilder> unmodifiableList = Collections.unmodifiableList(modifiableList);
// Modifying an element inside the unmodifiable list
unmodifiableList.get(0).append("!");
System.out.println("Modified Element: " + unmodifiableList.get(0)); // Outputs "Hello!"
}
}
Commentary:
- In this example, even though we have wrapped
modifiableList
inCollections.unmodifiableList()
, theStringBuilder
objects it contains can still be modified. Developers must be cautious with the types of objects they are including in unmodifiable collections.
2. Exception Handling
Using unmodifiable collections can lead to runtime exceptions if an attempt is made to modify them. This can be frustrating if not handled correctly. To gracefully manage such scenarios, consider wrapping your code in try-catch blocks, as shown in the examples above.
3. No Bulk Operations
Unmodifiable collections do not support most bulk operations. For example, methods that change the structure of the collection (like addAll
, removeIf
, etc.) are not allowed. If you require swapping data or performing bulk manipulations, you'll need a separate modifiable collection.
4. Performance Overhead
Creating unmodifiable collections introduces some performance overhead. In use cases where thousands of collections are created and disposed of, this could become a bottleneck. It's crucial to assess the size and frequency of these operations in your application.
Best Practices for Using Unmodifiable Collections
To ensure you are leveraging unmodifiable collections effectively, consider the following best practices:
- Use When Necessary: Utilize unmodifiable collections when you want to make sure your data remains unchanged, especially when exposing collections to external entities.
- Wrapper for Mutable Objects: If your unmodifiable collection contains mutable objects, ensure that you encapsulate them properly, possibly by creating a defensive copy mechanism.
- Document Behavior: Provide clear comments and documentation in your code to inform other developers about the immutability of collections, as this can prevent misuse.
- Conduct Thorough Testing: Always test your application extensively to handle any unexpected
UnsupportedOperationException
.
Key Takeaways
Unmodifiable collections are a powerful feature in JDK 10, allowing developers to maintain data integrity effectively. However, as we explored, they are not without their limitations, including shallow immutability and potential performance concerns. By understanding what unmodifiable collections can and cannot do, you can wield them effectively and avoid common pitfalls.
When appropriately employed, unmodifiable collections can significantly enhance the robustness of your applications, making them safer and more predictable. As you continue to work with Java, consider how these tools fit into your overall architecture, and always aim for clarity and maintainability in your code.
For more detailed guidance on collections in Java, you can check out the official Java documentation here and stay up to date with best practices.
Happy coding!
Checkout our other articles