Common Pitfalls When Sorting Objects in Java 8 Collections
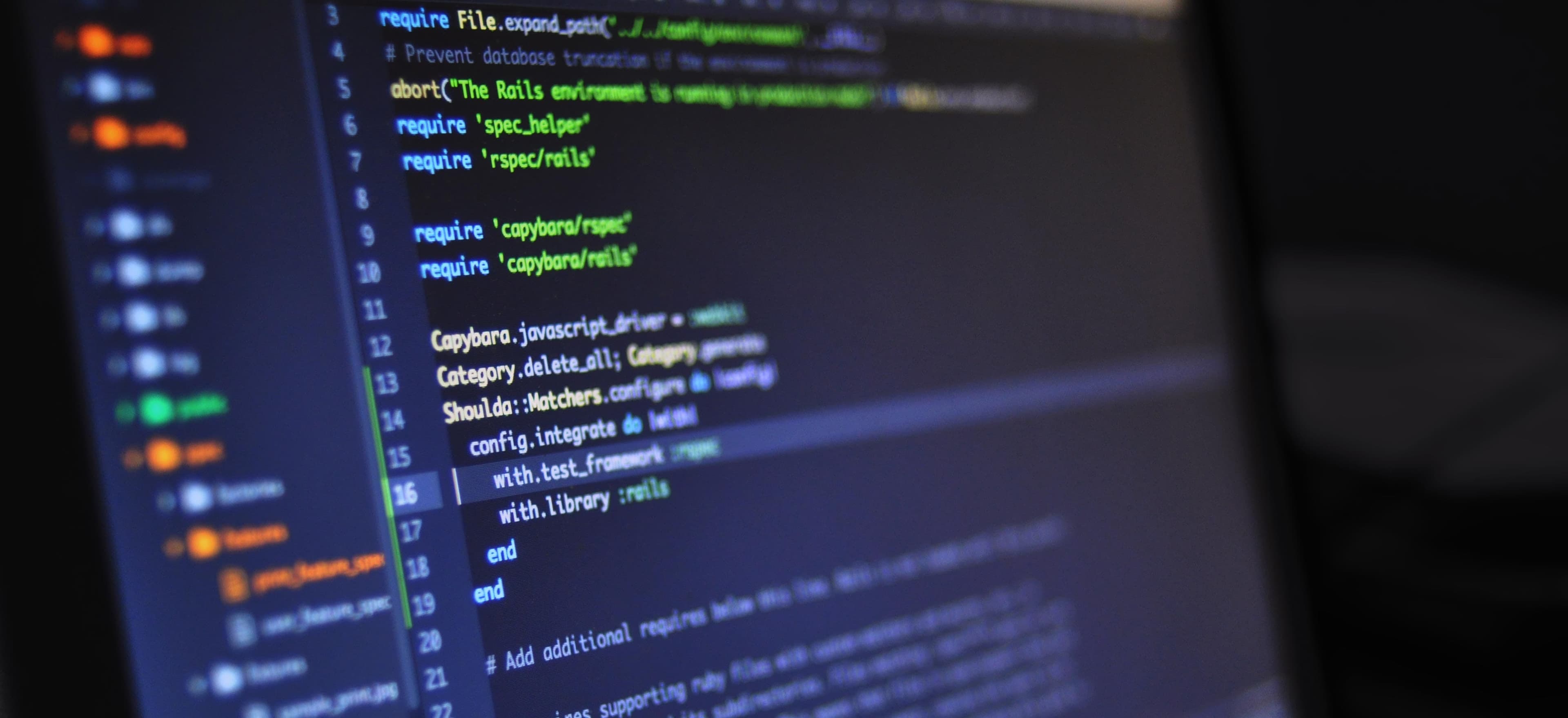
- Published on
Common Pitfalls When Sorting Objects in Java 8 Collections
Sorting collections in Java is a fundamental operation that developers need to perform frequently. With the introduction of Java 8, the process became significantly more streamlined due to the power of Streams and lambda expressions. However, the enhanced capabilities come with their quirks and nuances. This article discusses common pitfalls developers may encounter when sorting objects in Java 8 Collections and how to avoid them.
Understanding Sorting in Java Collections
Before diving into the pitfalls, it is essential to understand how sorting works in Java collections. The Collections
class provides a method called sort()
, which can sort lists of objects, while the Stream
API allows for more functional-style sorting.
Here's a quick example of sorting a list of strings:
List<String> names = Arrays.asList("Charlie", "Alice", "Bob");
Collections.sort(names);
System.out.println(names); // Output: [Alice, Bob, Charlie]
We can achieve the same using the Stream API:
List<String> sortedNames = names.stream()
.sorted()
.collect(Collectors.toList());
System.out.println(sortedNames); // Output: [Alice, Bob, Charlie]
While sorting might seem straightforward, several pitfalls can arise depending on the complexity of the objects being sorted.
Pitfall 1: Inconsistent compareTo
and equals
Methods
One of the most critical mistakes lies in incorrect implementation of the Comparable
interface. When an object implements Comparable
, it must ensure that the compareTo
method is consistent with the equals
method. If two objects are considered equal by equals()
, they should also have a return value of zero when passed to compareTo()
.
Here's an example of what not to do:
public class Person implements Comparable<Person> {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public int compareTo(Person other) {
return this.age - other.age; // Sorting by age
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (!(obj instanceof Person)) return false;
Person other = (Person) obj;
return this.name.equals(other.name); // Equals only by name
}
}
In this code, two Person
objects can be considered equal (by name) but differ in their comparison logic, leading to inconsistent results, particularly when sorting.
Fixing the Issue
To avoid this pitfall ensure that both compareTo
and equals
are consistent:
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (!(obj instanceof Person)) return false;
Person other = (Person) obj;
return this.name.equals(other.name) && this.age == other.age; // Equals by both fields
}
Pitfall 2: Using a Null Comparator
When sorting collections, a common mistake is to not handle null values properly. By default, a comparator may throw a NullPointerException
if it encounters a null element unless it is designed to handle it.
Example of the Issue
List<Person> people = Arrays.asList(new Person("Alice", 25), null, new Person("Bob", 30));
Collections.sort(people); // This will throw NullPointerException
Solution: Use a Null-Safe Comparator
To address this, utilize a comparator that gracefully handles nulls. Java 8 provides the Comparator.nullsFirst()
and Comparator.nullsLast()
methods:
Comparator<Person> nullSafeComparator = Comparator.comparing(Person::getAge, Comparator.nullsLast(Comparator.naturalOrder()));
people.sort(nullSafeComparator);
With the modified comparator, null values will be handled, allowing for safe sorts.
Pitfall 3: Sorting with a State-dependent Comparison
Another common issue is when the comparison logic relies on mutable state. If the state of objects changes while sorting, it can lead to unpredictable results or even a ConcurrentModificationException
.
Example of the Issue
public class Product {
private String name;
private int price;
public Product(String name, int price) {
this.name = name;
this.price = price;
}
public void changePrice(int newPrice) {
this.price = newPrice; // Mutable state
}
}
// Sorting in the meantime
List<Product> products = new ArrayList<>();
products.add(new Product("Product1", 100));
products.add(new Product("Product2", 150));
products.get(0).changePrice(200); // Mutating
Collections.sort(products, Comparator.comparingInt(p -> p.price)); // This is risky
Solution: Use Immutable Objects
To prevent this problem, you can either make the objects immutable or ensure that their state does not change during the sorting operation. Here’s how to create an immutable Product
:
public final class Product {
private final String name;
private final int price;
public Product(String name, int price) {
this.name = name;
this.price = price;
}
public int getPrice() {
return price; // No mutator here
}
}
Using immutable objects will guard against side effects during sorting.
Pitfall 4: Forgetting to Use Stream Pipelines Properly
Java Streams offer a powerful and concise way to sort collections. However, developers often forget that streams do not modify the original collection but instead return a new one.
Common Mistake
List<Person> people = Arrays.asList(new Person("Alice", 25), new Person("Bob", 30));
people.stream().sorted(Comparator.comparing(Person::getAge)); // This does nothing to the `people` list
Solution: Collect the Results
Make sure to collect the results back into a new list or replace the original list if needed:
List<Person> sortedPeople = people.stream()
.sorted(Comparator.comparing(Person::getAge))
.collect(Collectors.toList()); // Correct usage
My Closing Thoughts on the Matter
Sorting collections in Java 8 can greatly enhance the power and conciseness of your code. However, developers must be cautious of common pitfalls such as inconsistent comparison implementations, null handling, mutable state issues, and improper stream usage. By being aware of these pitfalls and applying the solutions discussed, you can avoid sorting errors in your Java applications.
For those looking to deepen their understanding of Java collections and sorting, I recommend checking out Java 8's Official Documentation for more details on collection manipulation and sorting mechanisms.
With this knowledge at hand, you'll be well-equipped to tackle sorting challenges in your Java projects efficiently. Happy coding!
Checkout our other articles